Getting Started with Data Preprocessing

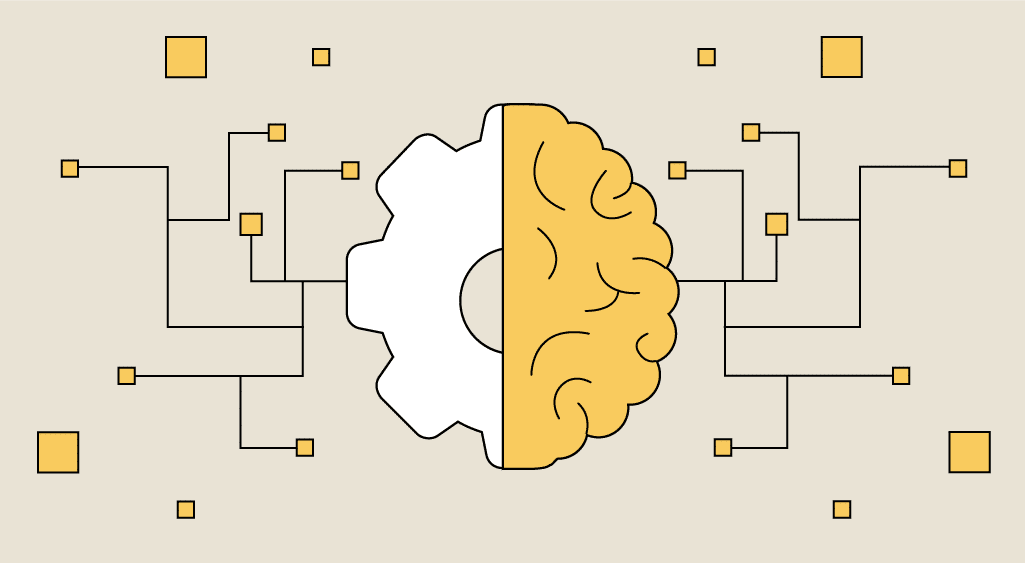
Introduction
Data preprocessing is the first and most crucial step in the Machine Learning pipeline. It involves transforming raw data into a clean and usable format, ensuring that the machine learning model can learn effectively. This step is vital as poor quality data can lead to inaccurate predictions and unreliable results. In this blog, we will explore the essential components of data preprocessing, including:
Data Cleaning
Feature Scaling
Encoding Categorical Data
Splitting Datasets
1. Data Cleaning
Data cleaning involves removing or correcting errors and inconsistencies in the data. It includes:
Handling Missing Data:
Missing data can occur due to various reasons such as user non-response, data corruption, or incomplete data entry. There are several strategies to handle missing data:
Remove Rows or Columns: Drop rows or columns with missing values if the percentage of missing data is low.
Imputation: Replace missing values with mean, median, or mode. For example, in Python:
from sklearn.impute import SimpleImputer import numpy as np imputer = SimpleImputer(missing_values=np.nan, strategy='mean') data[:, 1:3] = imputer.fit_transform(data[:, 1:3])
Predictive Imputation: Use ML models to predict missing values.
Handling Outliers:
Outliers can distort the results of a model. They can be handled by:
Removal: Removing data points beyond a certain threshold (e.g., using the Z-score method).
Transformation: Applying log transformation or scaling to reduce the impact of outliers.
Removing Duplicates:
Duplicates can cause overfitting. They can be removed using:
data = data.drop_duplicates()
2. Feature Scaling
Feature scaling standardizes the range of independent features, ensuring that all features contribute equally to the model's learning process. This is especially important for algorithms like SVM, K-NN, and Neural Networks.
Types of Feature Scaling:
Standardization: Scales the data to have a mean of 0 and a standard deviation of 1.
from sklearn.preprocessing import StandardScaler scaler = StandardScaler() data_scaled = scaler.fit_transform(data)
Normalization: Scales the data to a range between 0 and 1.
from sklearn.preprocessing import MinMaxScaler scaler = MinMaxScaler() data_normalized = scaler.fit_transform(data)
Robust Scaling: Uses the median and interquartile range, making it robust to outliers.
from sklearn.preprocessing import RobustScaler scaler = RobustScaler() data_robust = scaler.fit_transform(data)
3. Encoding Categorical Data
Categorical data must be converted into numerical form for machine learning models to process it. There are two common methods:
Label Encoding:
Converts each unique category into a number.
from sklearn.preprocessing import LabelEncoder label_encoder = LabelEncoder() data['Category'] = label_encoder.fit_transform(data['Category'])
Limitation: It can introduce ordinal relationships which may not exist.
One-Hot Encoding:
Converts categories into binary columns for each category.
from sklearn.preprocessing import OneHotEncoder from sklearn.compose import ColumnTransformer ct = ColumnTransformer([('encoder', OneHotEncoder(), [0])], remainder='passthrough') data = np.array(ct.fit_transform(data))
Advantage: Avoids ordinal relationships and is suitable for nominal categories.
Limitation: Increases dimensionality for features with many unique categories.
Choosing Between Label Encoding and One-Hot Encoding:
Use Label Encoding for ordinal categories (e.g., Low, Medium, High).
Use One-Hot Encoding for nominal categories (e.g., Color: Red, Blue, Green).
4. Splitting Datasets
Splitting the dataset is crucial for evaluating model performance. It involves dividing the data into:
Training Set: Used to train the model.
Test Set: Used to evaluate model performance.
Train-Test Split:
The most common split is 80% for training and 20% for testing.
from sklearn.model_selection import train_test_split
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
Train-Validation-Test Split:
For hyperparameter tuning, a validation set is also used:
Training Set: 60%
Validation Set: 20%
Test Set: 20%
X_train, X_temp, y_train, y_temp = train_test_split(X, y, test_size=0.4, random_state=42)
X_val, X_test, y_val, y_test = train_test_split(X_temp, y_temp, test_size=0.5, random_state=42)
Cross-Validation:
Cross-validation further improves model evaluation by splitting the data into k-folds:
k-Fold Cross-Validation: The dataset is divided into k subsets, and the model is trained k times, each time using a different subset as the test set.
from sklearn.model_selection import KFold kf = KFold(n_splits=5, shuffle=True, random_state=42) for train_index, test_index in kf.split(X): X_train, X_test = X[train_index], X[test_index] y_train, y_test = y[train_index], y[test_index]
Stratified k-Fold: Preserves the percentage of samples for each class in each fold, useful for imbalanced datasets.
Conclusion
Data preprocessing is a crucial step that significantly impacts the performance of a machine learning model. By cleaning the data, scaling features, encoding categorical data, and properly splitting datasets, we ensure that the model learns effectively and generalizes well to unseen data.
Subscribe to my newsletter
Read articles from Tushar Pant directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Tushar Pant
Tushar Pant
Cloud and DevOps Engineer with hands-on expertise in AWS, CI/CD pipelines, Docker, Kubernetes, and Monitoring tools. Adept at building and automating scalable, fault-tolerant cloud infrastructures, and consistently improving system performance, security, and reliability in dynamic environments.