Implement a Credits System with Stripe (my messy notes)
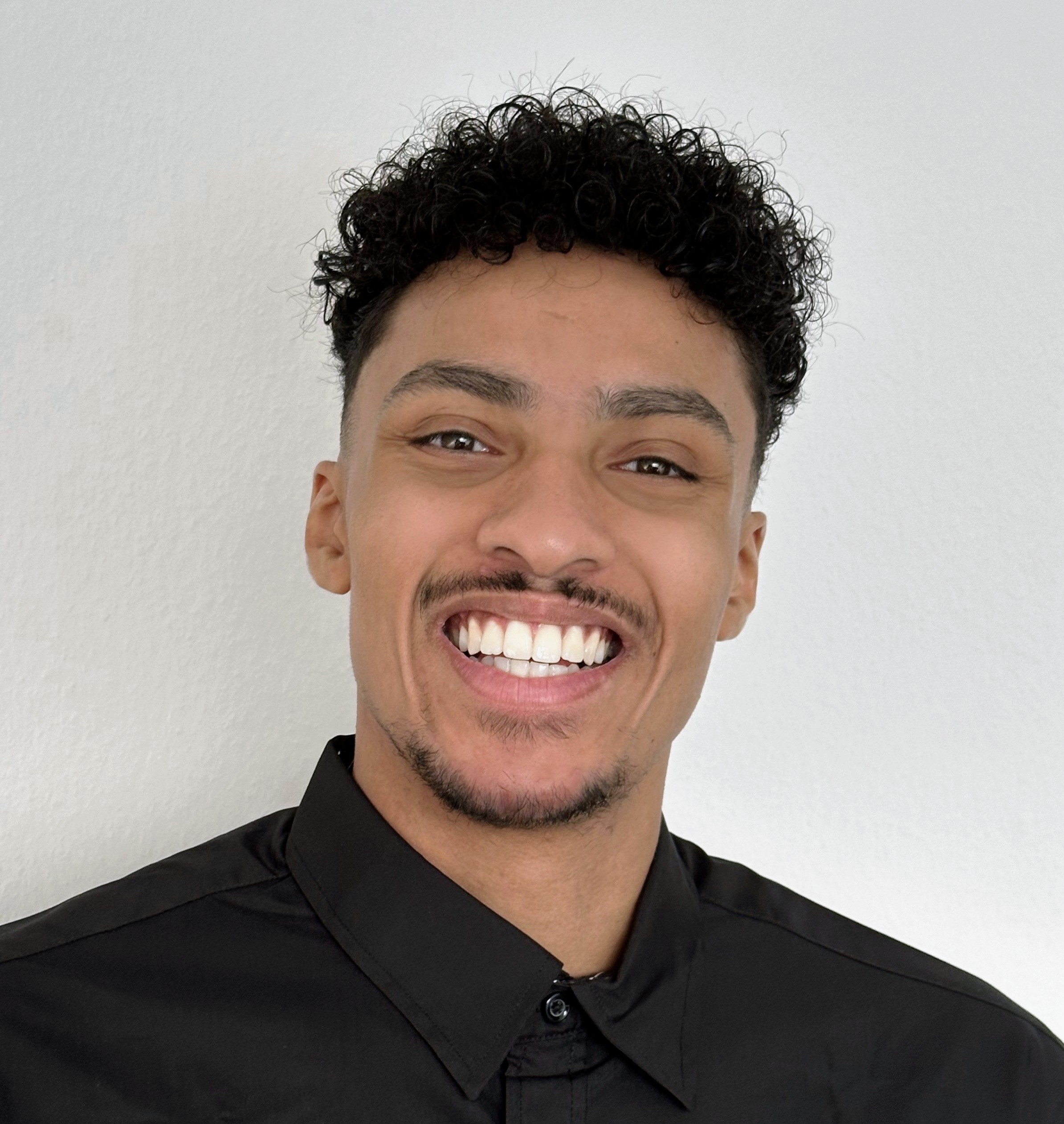
2 min read
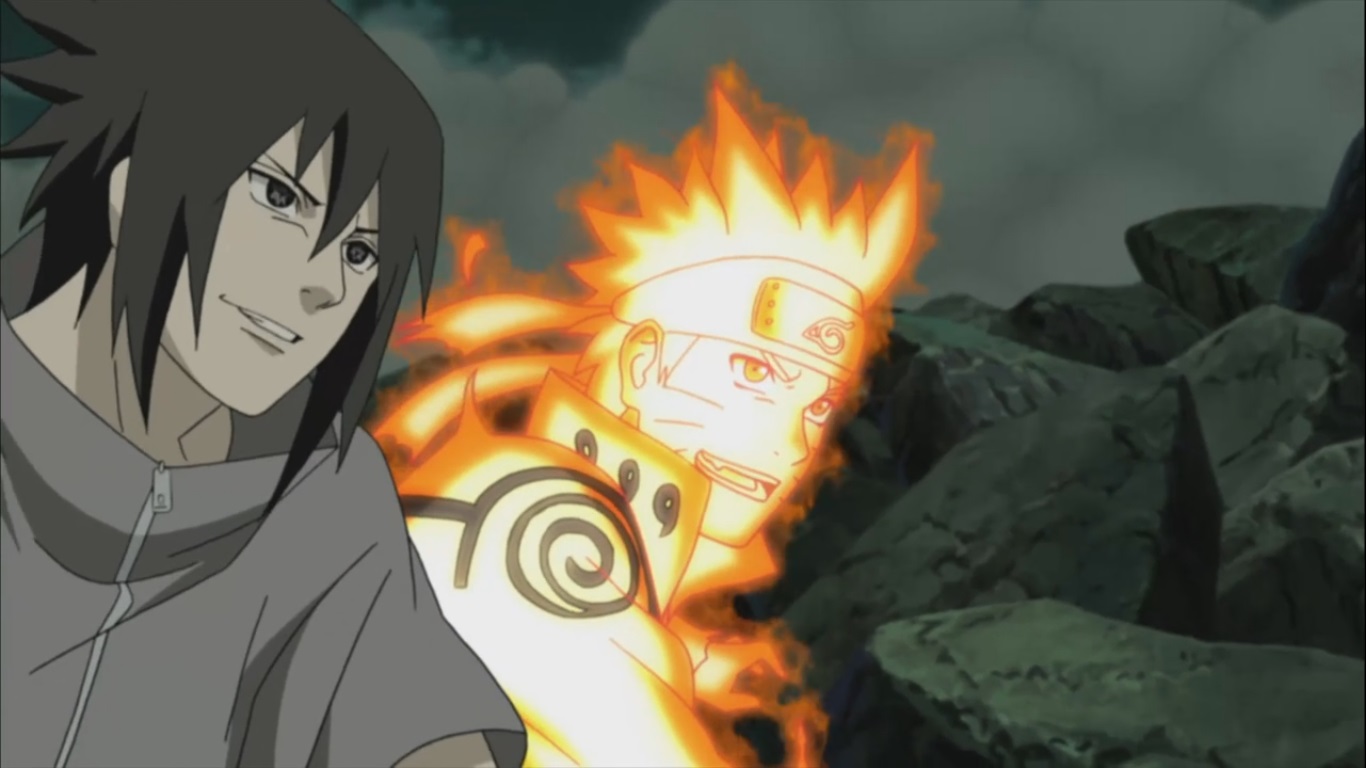
Key Components
1. User Model
interface User {
id: string;
credits: number;
// ... other fields
}
2. Stripe Product Setup
const PRICE_IDS = {
"100": process.env.PRICE_ID_100, // 100 credits
"250": process.env.PRICE_ID_250, // 250 credits
"500": process.env.PRICE_ID_500, // 500 credits
} as const;
// Map credits to their price IDs
type Credits = keyof typeof PRICE_IDS;
3. Checkout Session Creation
async function createCheckoutSession(userId: string, credits: Credits) {
const priceId = PRICE_IDS[credits];
if (!priceId) throw new Error("Invalid credits amount");
const session = await stripe.checkout.sessions.create({
mode: "payment",
metadata: {
userId,
credits, // Store credits in metadata for webhook
},
line_items: [
{
price: priceId,
quantity: 1,
},
],
success_url: `${process.env.HOST_URL}/dashboard`,
cancel_url: `${process.env.HOST_URL}/pricing`,
});
return session;
}
4. Webhook Handler
async function handleStripeWebhook(body: unknown, signature: string) {
const event = stripe.webhooks.constructEvent(
body,
signature,
process.env.STRIPE_WEBHOOK_SECRET!
);
if (event.type === "checkout.session.completed") {
const session = event.data.object as Stripe.Checkout.Session;
const { userId, credits } = session.metadata;
// Add credits to user
await updateUserCredits(userId, parseInt(credits));
}
return { success: true };
}
5. Credit Management
// Add credits (after purchase)
async function updateUserCredits(userId: string, amount: number) {
const user = await getUser(userId);
const newCredits = user.credits + amount;
await updateUser(userId, { credits: newCredits });
}
// Use credits (before action)
async function useCredits(userId: string, amount = 1) {
const user = await getUser(userId);
if (user.credits < amount) {
throw new Error("Insufficient credits");
}
const newCredits = user.credits - amount;
await updateUser(userId, { credits: newCredits });
}
Usage Pattern
User clicks "Buy Credits" button
Create checkout session with metadata
Redirect to Stripe checkout
Webhook triggers on successful payment
Parse metadata and add credits to user
Check credits before expensive operations:
async function generateSomething(userId: string) {
try {
await useCredits(userId);
// Do expensive operation
} catch (error) {
throw new Error("Please purchase more credits");
}
}
Tips
Always use database transactions when modifying credits
Add credits field to your auth token/session for quick checks
Consider adding a credit history/log for transparency
Make credit operations idempotent using session IDs
0
Subscribe to my newsletter
Read articles from Tiger Abrodi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
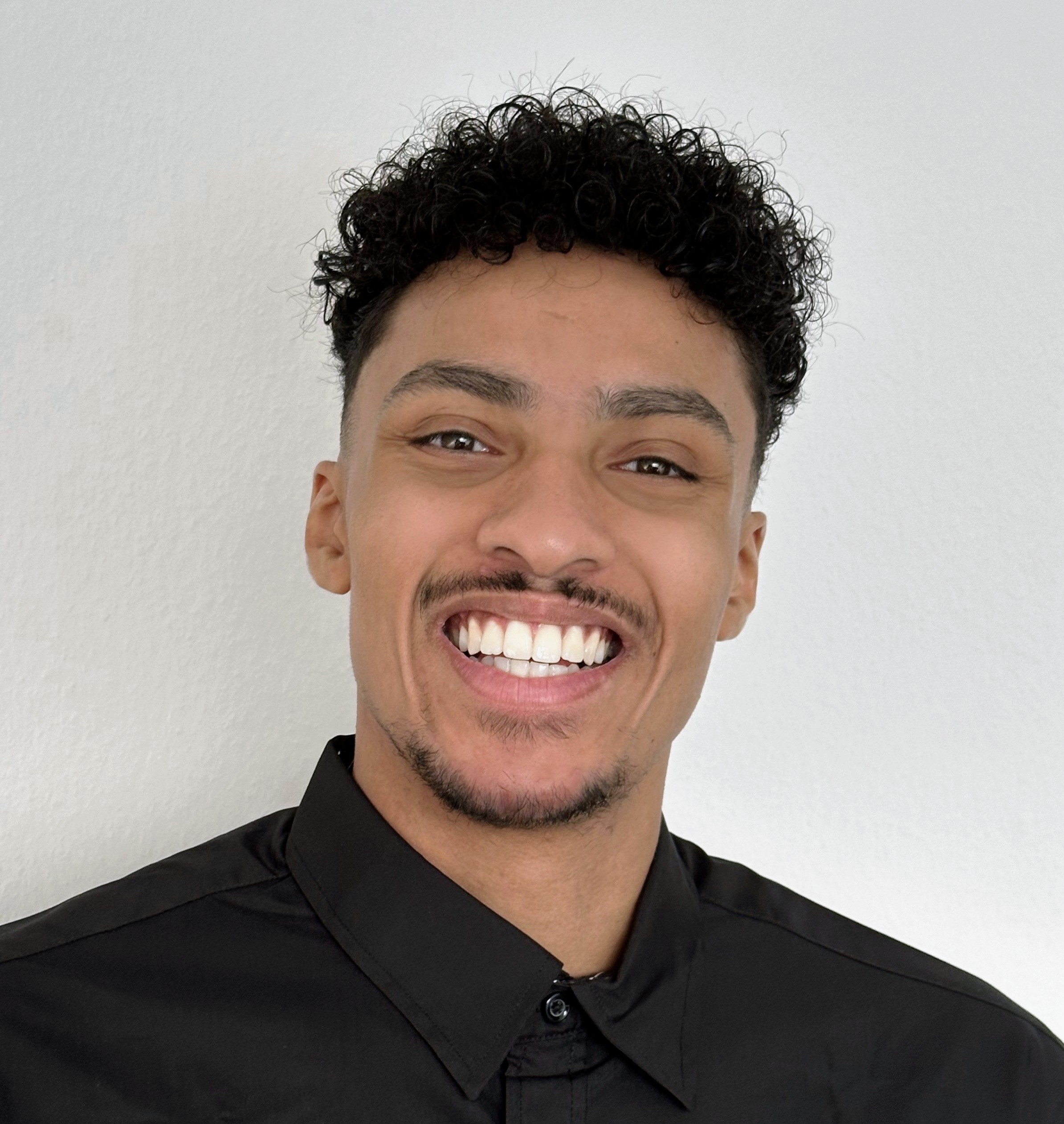
Tiger Abrodi
Tiger Abrodi
Just a guy who loves to write code and watch anime.