Naive Bayes – A Probabilistic Classifier with Powerful Simplicity

Table of contents
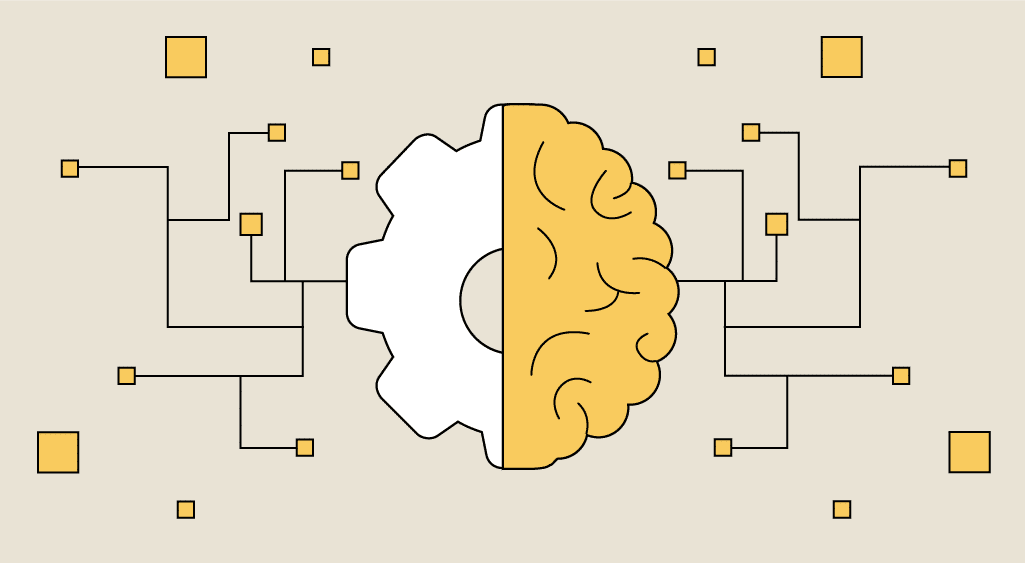
Introduction
Naive Bayes is a family of probabilistic algorithms based on Bayes' Theorem. It is known for its simplicity, speed, and effectiveness, particularly in text classification tasks like spam detection, sentiment analysis, and document categorization.
Despite its "naive" assumption of feature independence, Naive Bayes performs surprisingly well in many real-world applications. In this blog, we will explore:
1. What is Naive Bayes?
Naive Bayes is a probabilistic classifier that uses Bayes' Theorem to predict the probability of a class given input features. It assumes that all features are independent of each other (naive assumption), which simplifies calculations but is rarely true in practice.
1.1 Bayes' Theorem
The foundation of Naive Bayes is Bayes' Theorem, which calculates the posterior probability of a class CC given evidence XX:
Where:
P(C∣X) = Posterior probability of class C given predictor X
P(X∣C) = Likelihood of predictor X given class C
P(C) = Prior probability of class C
P(X) = Marginal probability of predictor X
1.2 Why Use Naive Bayes?
Simplicity and Speed: Fast to train and predict, even on large datasets.
Probabilistic Output: Provides probabilities for class membership.
Effective for Text Classification: Excels in spam detection and sentiment analysis.
2. How Does Naive Bayes Work?
Let's break down the working of Naive Bayes into simple steps:
Step 1: Calculate Prior Probabilities
Calculate the prior probability for each class from the training data:
Step 2: Calculate Likelihoods
Calculate the likelihood of each feature given each class. This is done differently for different types of Naive Bayes (Gaussian, Multinomial, Bernoulli).
Step 3: Apply Bayes' Theorem
For a new data point, calculate the posterior probability for each class using Bayes' Theorem:
- Since P(X)P(X) is constant across classes, it can be ignored for classification.
Step 4: Classification
Assign the class with the highest posterior probability:
3. Types of Naive Bayes Classifiers
There are three main types of Naive Bayes classifiers, each suitable for different data distributions:
3.1 Gaussian Naive Bayes
In Gaussian Naive Bayes, continuous values associated with each feature are assumed to be distributed according to a Gaussian distribution. A Gaussian distribution is also called Normal distribution When plotted, it gives a bell shaped curve which is symmetric about the mean of the feature values
Used for: Continuous data
Assumption: Features are normally distributed.
Likelihood Calculation:
- Applications: Image classification, medical diagnosis.
3.2 Multinomial Naive Bayes
Multinomial Naive Bayes is used when features represent the frequency of terms (such as word counts) in a document. It is commonly applied in text classification, where term frequencies are important.
Used for: Discrete data (e.g., word counts)
Assumption: Features represent frequencies or counts.
Likelihood Calculation:
- Applications: Text classification, document categorization.
3.3 Bernoulli Naive Bayes
Bernoulli Naive Bayes deals with binary features, where each feature indicates whether a word appears or not in a document. It is suited for scenarios where the presence or absence of terms is more relevant than their frequency. Both models are widely used in document classification tasks
Used for: Binary data (0 or 1)
Assumption: Features are binary (present or absent).
Likelihood Calculation:
- Applications: Binary text classification (e.g., spam detection).
4. Advantages and Disadvantages
4.1 Advantages:
Simple and Fast: Easy to implement and quick to train.
Scalable: Efficient for high-dimensional datasets.
Less Data Requirement: Works well with smaller datasets.
Handles Missing Data: Ignores missing features during classification.
4.2 Disadvantages:
Naive Assumption of Independence: Assumes all features are independent, which is rarely true.
Zero Frequency Problem: Assigns zero probability to unseen features.
Poor Performance on Correlated Features: Struggles when features are highly correlated.
Continuous Data Limitation: Gaussian Naive Bayes assumes normal distribution.
5. Naive Bayes Assumptions and Limitations
Feature Independence: Assumes all features are conditionally independent.
Class Conditional Independence: Assumes independence among features given the class label.
Real-world Limitation: In practice, features are often correlated, affecting performance.
6. Implementation of Naive Bayes in Python
Let's implement a Naive Bayes classifier using Scikit-learn:
# Import necessary libraries
import numpy as np
from sklearn import datasets
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler
from sklearn.naive_bayes import GaussianNB
from sklearn.metrics import accuracy_score, classification_report, confusion_matrix
import seaborn as sns
import matplotlib.pyplot as plt
# Load the Iris dataset
iris = datasets.load_iris()
X = iris.data
y = iris.target
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# Feature scaling
scaler = StandardScaler()
X_train = scaler.fit_transform(X_train)
X_test = scaler.transform(X_test)
# Instantiate the Gaussian Naive Bayes classifier
model = GaussianNB()
model.fit(X_train, y_train)
# Make predictions
y_pred = model.predict(X_test)
# Evaluate the model
print("Accuracy:", accuracy_score(y_test, y_pred))
print("Classification Report:\n", classification_report(y_test, y_pred))
# Confusion matrix visualization
conf_matrix = confusion_matrix(y_test, y_pred)
sns.heatmap(conf_matrix, annot=True, cmap='Blues')
plt.title('Confusion Matrix')
plt.xlabel('Predicted')
plt.ylabel('True')
plt.show()
7. Real-world Applications
Spam Detection: Classifying emails as spam or not spam.
Sentiment Analysis: Determining sentiment polarity in social media posts.
Text Classification: Document categorization and news classification.
Medical Diagnosis: Predicting diseases based on symptoms.
Recommender Systems: Suggesting products based on user preferences.
8. Tips for Better Performance
Feature Selection: Select relevant features to reduce noise and improve accuracy.
Smoothing Techniques: Use Laplace or Lidstone smoothing to handle zero probabilities.
Hybrid Models: Combine with other algorithms for better accuracy.
Handling Correlated Features: Use PCA or other dimensionality reduction techniques.
9. Conclusion
Naive Bayes is a powerful probabilistic classifier known for its simplicity, speed, and efficiency in text classification tasks. Despite its naive assumption of feature independence, it delivers competitive performance in many real-world scenarios.
Subscribe to my newsletter
Read articles from Tushar Pant directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
