Creating A Rock-Paper-Scissors Game Using Bashscript.
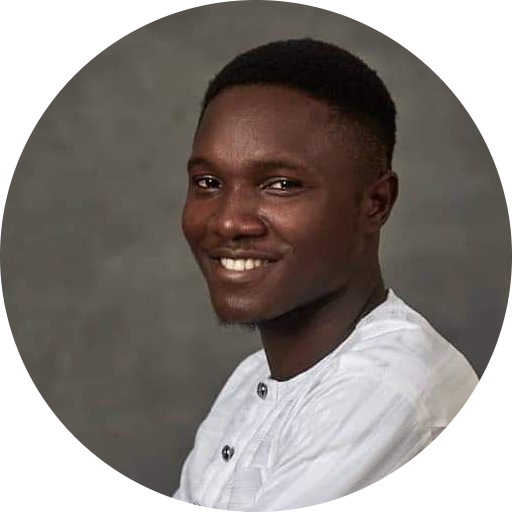
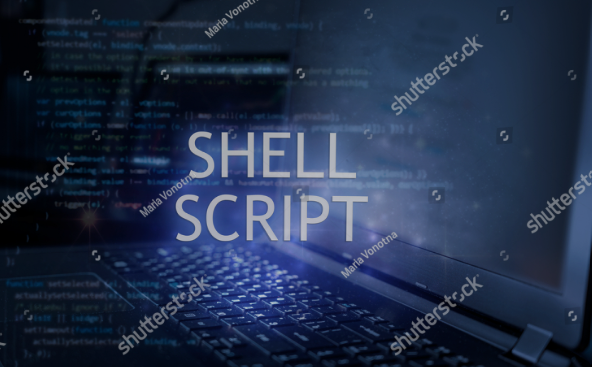
Introduction
Are you curious about how simple games can be created using Bashscript?
Before that, let’s see what Bashscript is :
Bashscript is a script that contains a series of Linux commands used for automating repetitive or complex set of tasks making the daily life of a system administrator easy and efficient.
Note that the very first step to writing a Bashscript is defining your shebang (#!/bin/bash).
Also note that in your script, comments are written with the hashtag (#) sign.
Why Bashscript?
Automation – Reduces repetitive manual work
Efficiency – Speeds up command execution
Ease of Use – Simple syntax, no need for compilation
Universality – Works across all Unix-like systems
Note : Before writing a script, it is important to know the in and out of what you’re trying to automate. This means that you should have a clear idea of what you’re developing before you write your script.
In this article, we’ll create a simple Rock-Paper-Scissors game using two approaches:
If and else statement.
Case statements.
As said earlier, it’s best practice to understand what you’re developing before writing your script for automation. Therefore, the rules of the game will be needed to carry out the automation process.
The rules :
Rock beats Scissors
Paper beats Rock
Scissors beat Paper
Same choices result in a tie
With these rules, it is clear that our script should make use of a decision-making logic. This is essential because the game involves comparing choices to determine the winner.
Writing The Rock-Paper-Scissors Game In A Bashscript.
To create a Bash script, we need to first create a file with a .sh
extension. The .sh
extension helps to identify the file as a shell script, making it easier to identify and execute.
Creating our file
Our file is named rock-paper-scissors.sh. It is best practice that we give our script file a name that best suits our project.
In the terminal, do:
touch rock-paper-scissors.sh
This creates an empty file named rock-paper-scissors.sh
.
Editing the Script Using a Text Editor (VIM)
You can open and edit the script using any text editor like nano
, vim
, or Visual Studio Code
but we’ll be using the vim
text editor.
To open the file using vim :
vim rock-paper-scissors.sh
Once within the vim
text editor, press letter ‘i’ on your keyboard to enter insert mode.
To save and exit the script file, press the ‘esc’ keypad, type :wq
and press Enter. This then takes us back to our terminal.
Understanding the Script
In this script, we’ll be making use of ‘arrays’.
An Array is used to store a collection of elements ( rock, paper, scissors ) under a single variable name.
Below is an example of how to define an array :
#Defining an array
choices=("rock" "paper" "scissors")
Each element in an array is accessed using an index which starts from 0. Example below:
#To access elements in an array one by one
echo "${choices[0]}" # Outputs rock
echo "${choices[1]}" # Outputs paper
echo "${choices[2]}" # Outputs scissors
#The more the choices, increase the index(numbers)
In this script we tried to access all elements. This is done using the ‘@’ symbol instead of an index.
#To access all elements
echo "${choices[@]}" # Outputs rock, paper, scissors
Steps to writing our script:
Define the choices.
The game consists of three choices which are rock, paper, and scissors. These choices will be stored in an array as described above and ensure that the user’s choice is valid.
#!/bin/bash
# Rock, Paper, Scissors Game
#Store valid choices
choices=("rock" "paper" "scissors")
read -p "Enter your choice (rock, paper, scissors): " user_choice
# Check if the user choice is valid
if [[ ! " ${choices[@]} " =~ " ${user_choice} " ]]; then
echo "Invalid choice"
exit 1
fi
The read
command is a Bash command used to take user input.
-p "Enter your choice (rock, paper, scissors): "
Here, the -p
flag allows to display a prompt message before taking input.
user_choice
: This is the variable where the user's input or choice is stored.
if [[ ... ]]; then
: This is an ‘if statement’ conditional block. An ‘if statement’ is used to check if the condition within the statement block is true. In this script, we’re trying to validate if the user choice is valid or true. Therefore, if the condition is true, the commands inside will execute.
!
: This command means negation. It reverses the condition set within the if statement block. Thus, if the condition is true, the negation symbol !
makes it false and vice versa.
" ${choices[@]} "
This access all elements within the array. It also expands an array into a string with spaces between the elements.
=~
This is used for pattern matching in Bash.
" ${choices[@]} " =~ " ${user_choice} "
This command checks if the user choice exists within the specified choices.
Therefore, the first part of the script checks if the user choice is found in the array where the choices are defined. If the user choice is not found, the script prints “Invalid choice” and exits with the exit 1
command which is an exit code that signals an error.
Generate a Random Choice for the Computer.
The game has to be dynamic and the computer’s choice should be unpredictable. It’s there fore logical to write a command to randomly generates a choice for the computer.
# Generate a random choice for the computer
computer_choice=${choices[$((RANDOM % 3))]}
echo "Computer choice: $computer_choice"
Here, ${choices[$((RANDOM % 3))]}
is assigned to a variable named ‘computer_choice’.
${choices[$((RANDOM % 3))]}
This command retrieves the elements in the variable name ‘choices’. Unllike the user choice where the player enters their selection, this command randomly picks one from the array called choices
and assigns it to the variable names computer_choice.
RANDOM
This is a special built-in Bash variable that generates a random number.
% 3
makes sure that the random number is between 0 and 2 (the %
is a modulus operator, which gives the remainder when dividing a number).
echo "Computer chose: $computer_choice" : This command prints the computer's random choice (rock, paper, or scissors) to the screen.
Determine the winner.
Now, we need to get or determine the winner of the game based on the rules of the game as stated above.
The winner will be determined using two different approaches.
i. If statement
ii. case statement
You can use either of the two approaches based on your preference.
Using the IF statement :
# Get the winner
if [[ "$user_choice" == "$computer_choice" ]]; then
echo "It's a tie"
elif [[ "$user_choice" == "rock" && "$computer_choice" == "scissors" ]] ||
[[ "$user_choice" == "paper" && "$computer_choice" == "rock" ]] ||
[[ "$user_choice" == "scissors" && "$computer_choice" == "paper" ]]; then
echo " You win"
else
echo "You lose, Computer wins"
fi
This part of the script makes use of logical operators in Bash Scripting. &&
which means AND and ||
which means OR are the logical operators used within the script for ‘comparisons and conditional execution.
In Bash scripting, &&
runs a command only if both conditions are true, while ||
runs a command if at least one condition is true.
In the Rock-Paper-Scissors script, &&
checks if the user’s choice beats the computer’s, and ||
links multiple winning cases. This helps decide the winner fairly based on the rules of the game.
if [[ "$user_choice" == "$computer_choice" ]]; then
echo "It's a tie"
This is a condition in an ‘If conditional block’ that checks if the user and the computer selected the same choice. If true, the game ends in a tie.
Else if as described in the script as elif
the user has chosen a winning choice based on the game rules, the script prints “You win”. Else
, the scripts moves to the final statement within the If conditional block, meaning the user has lost, and prints ”You lose, Computer wins”
.
Using Case Statement :
Using a case
statement is an alternative to using the if
statement. As a system administrator, cloud engineer etc, we focus on making life easier through automation. Therefore, a case
statement can or should be used instead of the if statement for better readability, efficiency and easier maintenance especially when handling multiple choices than in bash Scripting.
It is important to note that a case
statement starts with case
and ends with esac
. Moreso, In a case
statement, )
separates the condition from the action, ;;
tells Bash to stop checking after a match is found and *
tells how unexpected inputs or errors are handled.
Below is a case
statement that also determines the winner of the rock-paper-scissors game based on the game rules:
# Get the winner using case statement
case "$user_choice-$computer_choice" in
rock-rock|paper-paper|scissors-scissors)
echo "It's a tie" ;;
rock-scissors|paper-rock|scissors-paper)
echo "You win" ;;
rock-paper|paper-scissors|scissors-rock)
echo "You lose, Computer wins" ;;
*)
echo "Error" ;;
esac
In case
statement, a hyphen (-) is used as a separator to join two values into one string to match patterns.
case "$user_choice-$computer_choice" in
This joins the user choice and the computer choice in a single string which then allows the case statement to match patterns e.g as stated in the script we have, rock-scissors
, paper-rock
, etc.
Therefore, instead of having to run multiple checks, the case
statement groups all possible outcomes in one place. This ensures a cleaner, easier to read and more efficient script compared to the if
statement.
The script is the defined by the rules of the game using the matching patterns which compares the user choice and the computer choice to determine the winner or loser. And if there’s an unexpected input ( a choice not specified in the variable named choices
) it prints Error
.
Run the Script
Once done writing and editing our script, save and exit the text editor. As mentioned above, we used the vim
text editor to edit our script. Therefore, save and exit the script file by pressing the ‘esc’ keypad, type :wq
and press Enter.
More so, to run our script, we need to add executable permission to our script file. This ensures that we can execute the script directly from the terminal without needing to specify the Bash interpreter each time.
To add executable permission to the script file :
chmod +x rock-paper-scissors.sh
To run the script and ensure it’s working properly :
./rock-paper-scissors.sh
Our script works:
Conclusion
By using if
and case
statements, we successfully implemented decision-making in our Bash game. This simple exercise is a great way to practice scripting and user interaction in Bash.
Subscribe to my newsletter
Read articles from Seyitan Oluwaseitan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
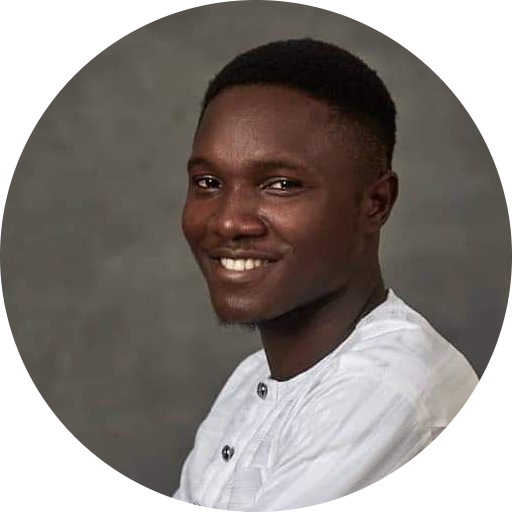
Seyitan Oluwaseitan
Seyitan Oluwaseitan
I am on my way to being a world class DevOps engineer.