Method Overloading in Java
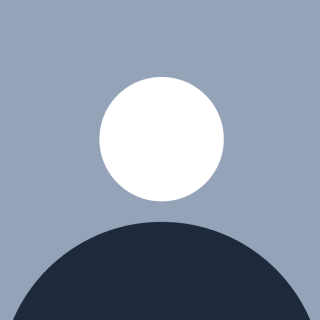

In Java, method overloading allows a class to have multiple methods with the same name. However, each must have a different set of parameters, either in the number of parameters, their data types, or both. This powerful feature enhances code readability, reduces redundancy, and improves maintainability by allowing methods to handle different input types while keeping their purpose clear.
With method overloading, developers can write more flexible and reusable code, making their programs adaptable to various scenarios. This article will explain what method overloading is, how it works, and why it’s useful in Java.
What is Method Overloading in Java?
Method overloading occurs when a class has multiple methods with the same name but different parameters varying in number, type, or both. This allows methods to perform similar functions while handling different inputs.
How to perform method overloading in Java?
To create a method overload in Java, define multiple methods with the same name inside the same class, but with different parameter lists. The parameters must differ in number, data type, or order to ensure uniqueness.
Let’s create a class that demonstrates method overloading:
class Calculator {
public int add(int a, int b) {
return a + b;
}
public double add(double a, double b) {
return a + b;
}
public int add(int a, int b, int c) {
return a + b + c;
}
}
public class Main {
public static void main(String[] args) {
Calculator calc = new Calculator();
System.out.println(calc.add(5, 10)); // Calls add(int, int)
System.out.println(calc.add(5.5, 2.5)); // Calls add(double, double)
System.out.println(calc.add(1, 2, 3)); // Calls add(int, int, int)
}
}
Code Explanation
The
Calculator
class defines three overloaded methods namedadd()
, each performing an addition operation but accepting different parameters:add(int, int)
: Accepts two integers and returns their sum.add(double, double)
: Accepts two double values and returns their sum.add(int, int, int)
: Accepts three integers and returns their sum.
The
main()
method creates an instance of theCalculator
class and calls theadd()
method three times, passing different types of arguments.Java determines which method to call based on the number and type of arguments provided:
calc.add(5, 10);
callsadd(int, int)
calc.add(5.5, 2.5);
Callsadd(double, double)
calc.add(1, 2, 3);
Callsadd(int, int, int)
This example explains how Java chooses the appropriate method at compile-time based on the provided arguments.
How Method Overloading Works
Java determines which overloaded method to call at compile-time based on the arguments passed when calling the method. This is why method overloading is also known as compile-time polymorphism.
When a method is invoked, Java follows a set of rules to determine the correct procedure:
Exact Match Preference:
When a method has the same name and its parameters exactly match the number and type of values you pass when calling it, Java will choose that method.
Let’s create a Printer
class with two overloaded methods, each having different parameter data types:
class Printer {
public void print(String message) {
System.out.println("Message: " + message);
}
public void print(int number) {
System.out.println("Number: " + number);
}
}
public class Main {
public static void main(String[] args) {
Printer printer = new Printer();
printer.print("Hello"); // Calls print(String message)
printer.print(10); // Calls print(int number)
}
}
Here, Java selects print(String)
for a string input and print(int)
for an integer input because they match exactly.
Type Promotion:
This is also known as widening conversion. If an exact match is not found, Java tries to promote smaller data types to larger ones.
class Calculator {
public void add(double a, double b) {
System.out.println("Sum: " + (a + b));
}
}
public class Main {
public static void main(String[] args) {
Calculator calc = new Calculator();
calc.add(5, 10); // Calls add(double, double) (int -> double conversion)
}
}
Since 5
and 10
are integers, but no add(int, int)
method exists, Java automatically promotes them to double
, selecting add(double, double)
.
Method Selection Based on Parameter Order:
If two overloaded methods have the same parameter types but in different order, Java distinguishes them based on the sequence of arguments passed in the method call.
class Display {
public void show(int a, String b) {
System.out.println("Integer: " + a + ", String: " + b);
}
public void show(String a, int b) {
System.out.println("String: " + a + ", Integer: " + b);
}
}
public class Main {
public static void main(String[] args) {
Display display = new Display();
display.show(5, "Hello"); // Calls show(int, String)
display.show("World", 10); // Calls show(String, int)
}
}
Java differentiates between the two overloaded methods based on the order of arguments.
Conclusion
In this article, we explored method overloading in Java, a powerful feature that allows multiple methods to share the same name but differ in parameters. We learned how to implement method overloading with practical examples, demonstrating how Java selects the appropriate method at compile-time based on exact matches, type promotion, and parameter order. By mastering method overloading, developers can write more efficient and reusable code, improving both functionality and structure in their Java applications.
Thank you for reading and Happy Coding!
Subscribe to my newsletter
Read articles from Dennis Temoye directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by