How to Insert an Image in Word Documents with Python [Step-by-Step Guide]

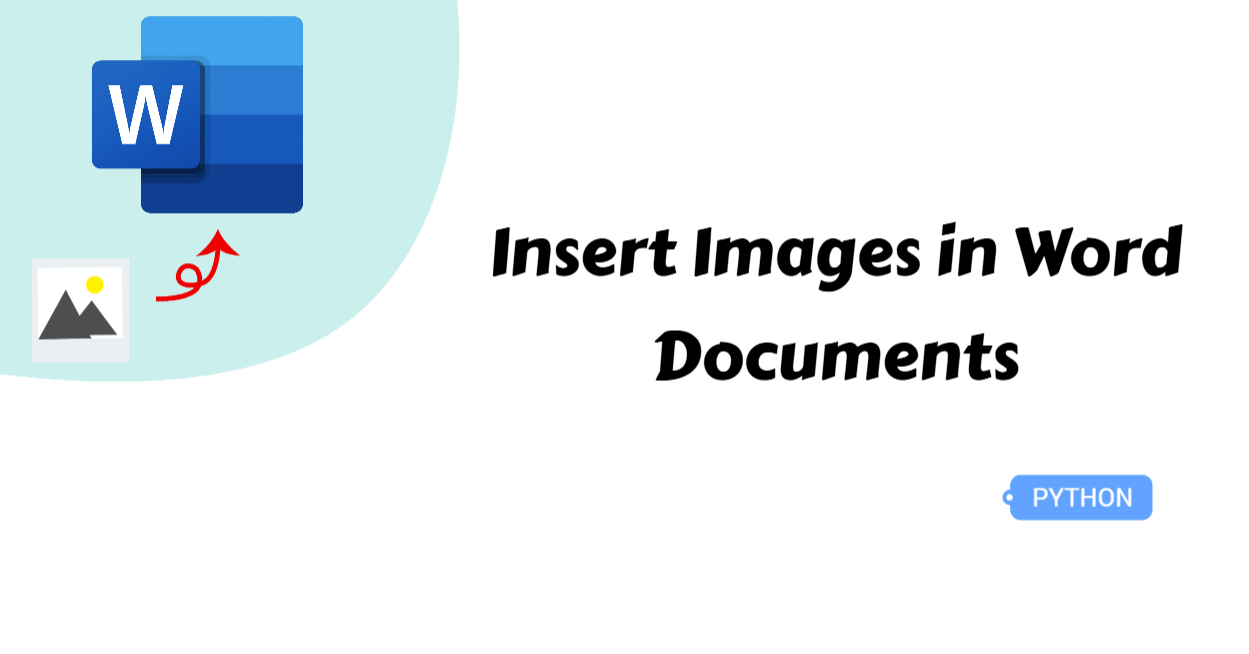
Word, the most widely used document editor, enables users to seamlessly integrate text and images for more engaging and visually appealing content. Whether you need to insert logos, charts, or other visual elements, adding images enhances clarity and improves the overall presentation of reports and documents. With Python, you can automate this process efficiently, saving time and effort. In this article, we’ll walk you through how to insert images in Word using Python, helping you create well-structured and professional-looking documents.
Python Library to Insert Images in Word Documents
To quickly add images to Word documents, using a third-party library is highly recommended. When considering Python Word APIs, python-docx and win32com might come to mind. While both allow users to insert images into Word files, they come with limitations—python-docx does not support precise image positioning, and win32com requires MS Word to be installed and only works on Windows. Instead, Spire.Doc and Aspose.Words offer more flexibility. These standalone libraries do not depend on Microsoft Office and can run on any system. Given its intuitive API and rich features, this article will use Spire.Doc as an example to demonstrate how to insert images into a Word file efficiently. You can install it using the pip command: pip install Spire.Doc
.
How to Insert an Image in a Word Document: The Basic Method
With the preparation done, let’s dive into the process of adding an image to a Word document using Python. This section covers the basic approach—using the Paragraph.AppendPicture() method to insert an image into a paragraph. The process involves creating a new document, adding sections and paragraphs, and then inserting the image into a specific paragraph. Let’s go through the steps in detail.
Steps to insert an image in Word documents:
Create an object of the Document class.
Add a new section with the Document.AddSection() method, and add new paragraphs with the Section.AddParagraph() method.
Add text to the paragraphs and customize the formatting.
Load an image and place it in a paragraph through the Paragraph.AppendPicture() method.
Configure the width of the image with the DocPicture.Width property and the height with the DocPicture.Height property.
Set its text wrapping style using the DocPicture.TextWrappingStyle property.
Save the resulting Word document.
Here is the Python example of inserting an image into a new Word document:
from spire.doc import *
from spire.doc.common import *
# Create a Document object
document = Document()
# Add a seciton
section = document.AddSection()
# Add a paragraph
paragraph1 = section.AddParagraph()
# Add text to the paragraph and set formatting
tr = paragraph1.AppendText("This is sample text showing how to add images in Word documents with Spire.Doc for Python.")
tr.CharacterFormat.FontName = "Calibri"
tr.CharacterFormat.FontSize = 11
paragraph1.Format.LineSpacing = 18
paragraph1.Format.BeforeSpacing = 10
paragraph1.Format.AfterSpacing = 10
# Add another paragraph
paragraph2 = section.AddParagraph()
tr = paragraph2.AppendText("This is sample text showing how to insert an image in Word files.")
# Add text to the paragraph and set formatting
tr.CharacterFormat.FontName = "Calibri"
tr.CharacterFormat.FontSize = 11
paragraph2.Format.LineSpacing = 18
# Add an image to the specified paragraph
picture = paragraph1.AppendPicture("/Logo1.png")
# Set image width and height
picture.Width = 50
picture.Height = 50
# Set text wrapping style for the image
picture.TextWrappingStyle = TextWrappingStyle.Square
# Save the result document
document.SaveToFile("/InsertImageinWord.docx", FileFormat.Docx)
document.Close()
How to Add an Image in a Word Document at a Specific Position
After learning the basics of inserting an image into a paragraph, let's take it a step further and explore how to precisely position an image within a Word document. By using the DocPicture.HorizontalPosition and DocPicture.VerticalPosition properties, you can control the exact placement of the image, ensuring it appears exactly where you need it.
Steps to add an image in a Word document at a specific position:
Create an instance of the Document class.
Add a section with the Document.AddSection() method, and add paragraphs with the Section.AddParagraph() method.
Insert an image into the Word document using the Paragraph.AppendPicture() method.
Configure the width of the image with the DocPicture.Width property and the height with the DocPicture.Height property.
Customize the specific position of the image through the DocPicture.HorizontalPosition and DocPicture.VerticalPosition properties.
Set the text wrapping style of the image.
Save the new Word document to files.
Here’s a code example demonstrating how to insert an image into a Word document and set its exact position:
from spire.doc import *
from spire.doc.common import *
# Create a Document object
doc = Document()
# Add a section
section = doc.AddSection()
# Add a paragraph to the section
paragraph = section.AddParagraph()
# Add text to the paragraph and set formatting
paragraph.AppendText("The sample demonstrates how to insert an image at a specified location in a Word document.")
paragraph.ApplyStyle(BuiltinStyle.Heading2)
# Add an image to the paragraph
picture = paragraph.AppendPicture("/Logo1.png")
# Set image position
picture.HorizontalPosition = 150.0
picture.VerticalPosition = 60.0
# Set image size
picture.Width = 40.0
picture.Height = 60.0
# Set a text wrapping style for the image (note that the position settings are not applicable when the text wrapping style is Inline)
picture.TextWrappingStyle = TextWrappingStyle.Through
# Save the result document
doc.SaveToFile("/InsertImage-SpecificPosition.docx", FileFormat.Docx)
doc.Close()
The Bottom Line
This guide covers how to insert an image in Word documents using Python and further customize its position. To simplify the process, it provides step-by-step instructions and code examples. By the end of this article, you'll see that adding images to Word documents is quick and effortless!
Subscribe to my newsletter
Read articles from Casie Liu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
