What REALLY Happens When You Type Localhost?
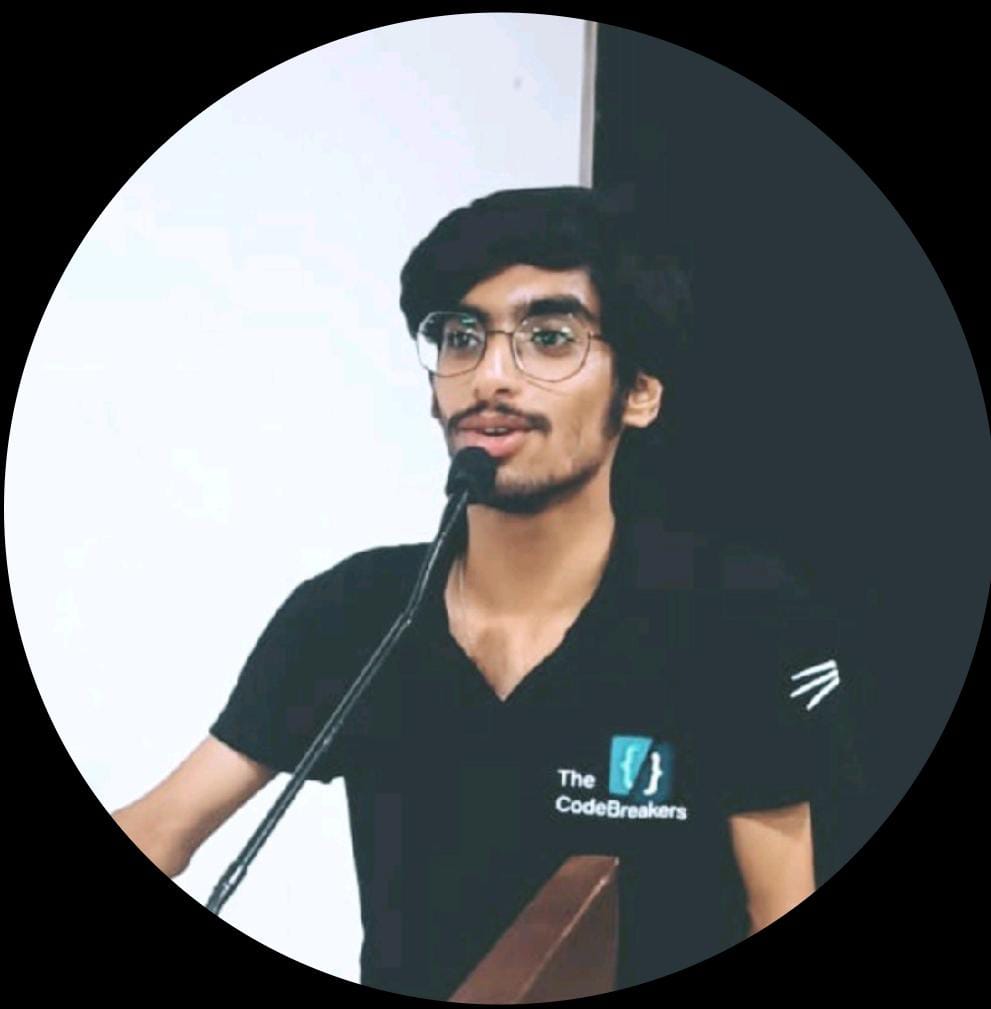
Table of contents
- What is Localhost & Why Does It Matter?
- The Loopback Interface & 127.0.0.1 Explained
- Inside the TCP/IP Stack: How Localhost Traffic Flows
- Socket Programming with Localhost
- Why Localhost is Useful for Developers
- Virtual Hosts and Multiple Projects on Localhost
- Localhost Security: It's Not as Isolated as You Think
- CORS and Localhost: Special Behavior
- IPv4 vs. IPv6: Why Both Are Important
- Containerization and Localhost: It Gets Complicated
- Localhost vs. Private & Public IPs
- Advanced Localhost Debugging Techniques
- The Power of Localhost for Developers

Whether you're a front-end developer spinning up a React app or a back-end engineer building and testing APIs, localhost is where it all starts. You type that familiar npm run dev
, and in an instant, your browser opens with http://localhost:3000
. But what exactly is going on under the hood? Let's take a deep dive into the networking magic behind Localhost and why it's such an important tool for developers.
What is Localhost & Why Does It Matter?
Localhost isn't just a random term developers made up—it's a reserved domain name that always points back to your own machine. Think of it like your home address but for your computer. No matter where you are in the world, when you type localhost
, your computer is talking to itself—digitally, of course. And here's the cool part: every machine has its own Localhost. So, whether you're on a laptop or desktop, if you type localhost
, you're accessing your own device without interfering with anyone else.
To understand Localhost better, let's break down how a website actually loads. When you type google.com
into your browser, your system checks the Domain Name System (DNS) to translate google.com
into an IP address. That address takes you to Google's servers, where your request is processed. But when you type localhost
, your computer bypasses all of that. It doesn't need to check the DNS; it already knows that localhost
means "right here," and it routes the request directly to itself.
Localhost is just a user-friendly name for the IP address 127.0.0.1
. When you type localhost
, your computer doesn't query an external DNS server. Instead, it refers to a special file on your machine called the hosts file (located at /etc/hosts
on Linux/macOS or C:\Windows\System32\drivers\etc\hosts
on Windows). Inside this file, there's an entry like this:
127.0.0.1 localhost
This entry tells the operating system that localhost
should resolve to 127.0.0.1
. This address points to your machine's loopback interface, which processes requests that never leave your computer.
The Loopback Interface & 127.0.0.1 Explained
The loopback interface is a virtual network interface that sends data back to the same device. Imagine throwing a tennis ball against a wall, and it bounces right back to you. That's how the loopback interface works. The entire 127.x.x.x
IP range is reserved for this purpose. That means 127.0.0.2
, 127.0.0.3
, and even 127.255.255.255
all point back to your machine. However, 127.0.0.1
is the standard.
In simple terms, 127.0.0.1
is a hardcoded IP that always points to your device, while localhost
is a name that gets mapped to that IP address via the hosts file.
Inside the TCP/IP Stack: How Localhost Traffic Flows
When you make a request to localhost, something fascinating happens at the TCP/IP stack level. Unlike regular network traffic that passes through all layers of the networking stack and out to physical network interfaces, localhost traffic takes a shortcut.
Here's a technical breakdown of how a request to localhost:3000
flows through the TCP/IP stack:
Application Layer: Your browser or application initiates a request to connect to localhost on port 3000.
Transport Layer: The TCP protocol establishes a connection, but instead of preparing the packet for external transmission, it recognizes the loopback address and flags it for internal routing.
Internet Layer: The IP protocol sees the
127.0.0.1
destination and routes it to the loopback interface, bypassing the normal routing table lookups for external destinations.Link Layer: Instead of converting the packet to electrical signals to be sent over physical media (like Ethernet), the packet is immediately passed back up the stack to the receiving application.
This efficient short-circuit is why localhost connections are extremely fast—the data never leaves your computer's memory, and never touches the physical network interface.
You can observe this behavior using packet capture tools like Wireshark—you won't see localhost traffic on your physical network interfaces, as it's entirely contained within the loopback interface.
Socket Programming with Localhost
From a programmer's perspective, localhost is where socket programming begins. When you bind a server to localhost, you're instructing it to only accept connections from the local machine. Here's a technical example using Node.js:
const http = require('http');
// Create an HTTP server
const server = http.createServer((req, res) => {
res.writeHead(200, {'Content-Type': 'text/plain'});
res.end('Hello World\n');
});
// Bind to localhost only - this server is not accessible from other machines
server.listen(3000, '127.0.0.1', () => {
console.log('Server running at http://127.0.0.1:3000/');
});
Contrast this with binding to all interfaces (which makes your server potentially accessible from other machines on the network):
// Bind to all available network interfaces
server.listen(3000, '0.0.0.0', () => {
console.log('Server running and accessible from the network');
});
Understanding this distinction is crucial for security. When developing, binding to localhost provides an isolated environment where your application isn't exposed to potential attackers on your network.
Why Localhost is Useful for Developers
For developers, localhost
is a vital tool. It allows you to test applications locally before pushing them to production. You can run a local web server, test APIs, or debug code without needing an internet connection. It's also a secure environment for working with sensitive applications, since requests to localhost
never leave your machine.
Additionally, the loopback interface is incredibly efficient. Since there's no need for external routing, requests are processed much faster compared to communication over a network. You can even test this efficiency by running Java or Python code to confirm that localhost
resolves to 127.0.0.1
.
Here's a simple Python example to check the IP address of localhost
:
import socket
print(socket.gethostbyname("localhost"))
In JavaScript, the DNS lookup function can also be used to resolve localhost
. The callback's family
parameter tells you whether the result is IPv4 or IPv6. Some operating systems, especially newer versions of macOS, default localhost
to IPv6 (::1
), while others continue to use the IPv4 address (127.0.0.1
).
Virtual Hosts and Multiple Projects on Localhost
Professional developers often work on multiple projects simultaneously. While you could run each on a different port (localhost:3000, localhost:3001, etc.), a more elegant solution is to use virtual hosts. This allows you to use different domain names that all resolve to localhost.
Here's how to set this up:
- Modify your hosts file to include:
127.0.0.1 project1.local
127.0.0.1 project2.local
127.0.0.1 api.project1.local
- Configure your web server (like Apache or Nginx) to handle these domains:
# Example Nginx configuration
server {
listen 80;
server_name project1.local;
root /path/to/project1;
# other configuration
}
server {
listen 80;
server_name project2.local;
root /path/to/project2;
# other configuration
}
This approach creates a development environment that more closely resembles production, where different domains handle different services.
Localhost Security: It's Not as Isolated as You Think
While localhost traffic doesn't leave your machine via the network, it's not completely isolated from security concerns. Malicious software running on your computer can still interact with services bound to localhost. This is why many sensitive services run on localhost by default—they're not exposed to the network, but they're still accessible to applications running on the same machine.
Security considerations for localhost services:
Port scanning: Malware can scan for open localhost ports to find vulnerable services.
Cross-Site Request Forgery (CSRF): Websites you visit can make requests to your localhost services, potentially triggering unwanted actions.
XSS to localhost: If your application has XSS vulnerabilities, attackers can exploit them to make requests to other localhost services.
Best practices include:
Using authentication even for localhost services
Implementing CSRF protection
Being aware of which ports are open on localhost
Using HTTPS even for localhost (with self-signed certificates)
To see which services are listening on localhost, use:
On Linux/macOS:
netstat -tuln | grep 127.0.0.1
On Windows:
netstat -an | findstr 127.0.0.1
CORS and Localhost: Special Behavior
Cross-Origin Resource Sharing (CORS) has special implications for localhost development. Modern browsers implement the Same-Origin Policy, which restricts how documents or scripts from one origin interact with resources from another origin. However, localhost often gets special treatment.
Consider this scenario: You have a front-end application running on localhost:3000
and an API server on localhost:8080
. Even though they have different ports, they're considered different origins under the Same-Origin Policy.
Your API server needs to include CORS headers to allow the front-end to access it:
// Express.js API server with CORS enabled for localhost development
const express = require('express');
const cors = require('cors');
const app = express();
// Enable CORS for the front-end
app.use(cors({
origin: 'http://localhost:3000',
methods: ['GET', 'POST', 'PUT', 'DELETE'],
credentials: true // Allow cookies to be sent with requests
}));
app.get('/api/data', (req, res) => {
res.json({ message: 'Data from API' });
});
app.listen(8080, '127.0.0.1', () => {
console.log('API running at http://localhost:8080');
});
Chrome and some other browsers have special relaxations for localhost development. Chrome, for instance, allows access to file://
URLs from pages served from localhost
, which it wouldn't allow for other domains.
IPv4 vs. IPv6: Why Both Are Important
You may be wondering, why does the internet need both IPv4 and IPv6? These are two different addressing systems that help identify devices on a network. Every device connected to a network needs a unique IP address. When IPv4 was designed in the 1980s, no one anticipated the explosion of devices connected to the internet. Today, we have smartphones, IoT devices, smart fridges, and even cars all requiring IP addresses.
IPv4 supports a maximum of around 4.3 billion unique addresses, but we've run out. IPv6 solves this issue by offering an almost infinite number of unique addresses (340 undecillion, to be precise). This ensures every device can have its own IP address without the need for Network Address Translation (NAT).
In the context of localhost, IPv6 uses ::1
as the loopback address (equivalent to IPv4's 127.0.0.1
). You can test if your system resolves localhost to the IPv6 loopback with:
ping -6 localhost
Modern operating systems will try IPv6 first, then fall back to IPv4 if necessary.
Containerization and Localhost: It Gets Complicated
Docker and other containerization technologies introduce interesting complexities to the concept of localhost. Inside a Docker container, localhost
refers to the container itself, not the host machine.
This can lead to confusion when attempting to connect to services. For example, if you have a database running on the host machine's localhost (port 5432), a containerized application cannot connect to it using localhost:5432
because that would refer to a (non-existent) database inside the container.
There are several ways to handle this:
- Use Docker's special hostname: On Docker for Mac and Windows, you can use
host.docker.internal
to refer to the host machine:
// Inside a Docker container, connecting to a service on the host
mongoose.connect('mongodb://host.docker.internal:27017/mydb');
- Use Docker networks: Creating a Docker network allows containers to communicate using container names as hostnames:
# Create a network
docker network create mynetwork
# Run containers in that network
docker run --network mynetwork --name mongodb mongo
docker run --network mynetwork --name myapp myapp-image
# Now myapp can connect to mongodb using:
# mongodb://mongodb:27017/mydb
- Use host networking mode: This shares the host's network namespace with the container, effectively removing the network isolation:
docker run --network host myapp-image
Understanding these distinctions is crucial for modern microservices development, where applications may run across multiple containers.
Localhost vs. Private & Public IPs
When discussing Localhost, it's important to differentiate between private and public IP addresses. A public IP is globally unique and assigned by your Internet Service Provider (ISP) to allow communication over the internet. For instance, Google's public DNS has the IP 8.8.8.8
. When you visit google.com, your browser connects to this public IP address via the internet.
A private IP, on the other hand, is used within a local network, like your home or office. These IPs are not directly accessible from the internet. For example, your Wi-Fi router may have the IP 192.168.1.1
, while your laptop or phone may have an IP like 192.168.1.100
.
However, localhost is neither a private nor a public IP. It is a loopback address. Unlike private IPs, localhost
never leaves your machine, and unlike public IPs, it isn't assigned by an ISP. It belongs to the 127.x.x.x
range, which is specifically reserved for loopback testing.
Advanced Localhost Debugging Techniques
Proficient developers use various techniques to debug localhost connections:
- Monitoring with tcpdump: For low-level network analysis of localhost traffic:
sudo tcpdump -i lo0 port 3000
- Socket state examination: View detailed information about localhost sockets:
ss -tlnp | grep 127.0.0.1
- Latency testing with custom tools:
import time
import socket
def measure_localhost_latency(iterations=1000):
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
sock.connect(('localhost', 3000))
total_time = 0
for _ in range(iterations):
start = time.time()
sock.send(b'ping')
response = sock.recv(4)
end = time.time()
total_time += (end - start)
sock.close()
return (total_time / iterations) * 1000 # Return average in milliseconds
print(f"Average localhost latency: {measure_localhost_latency():.6f} ms")
- Stress testing localhost services:
# Using Apache Bench to test a localhost web server
ab -n 10000 -c 100 http://localhost:3000/
These techniques can help identify performance bottlenecks and issues that might not be apparent in regular testing.
The Power of Localhost for Developers
Whether you're testing an application, analyzing network traffic, or simply curious about how the internet works, localhost
and 127.0.0.1
are always there, looping your requests back to your own machine. By understanding how it works, you can make more efficient use of this powerful tool in your development workflow.
Next time you see http://localhost:3000
in your browser, you'll have a deeper understanding of the networking magic that makes it possible. Localhost is not just a concept—it's a critical part of the developer toolkit, with layers of technical complexity that make it fascinating to explore.
By mastering the technical aspects of localhost, you're better equipped to build, debug, and secure your applications—skills that will serve you well throughout your development career. Happy coding!
Subscribe to my newsletter
Read articles from Tushar Pamnani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
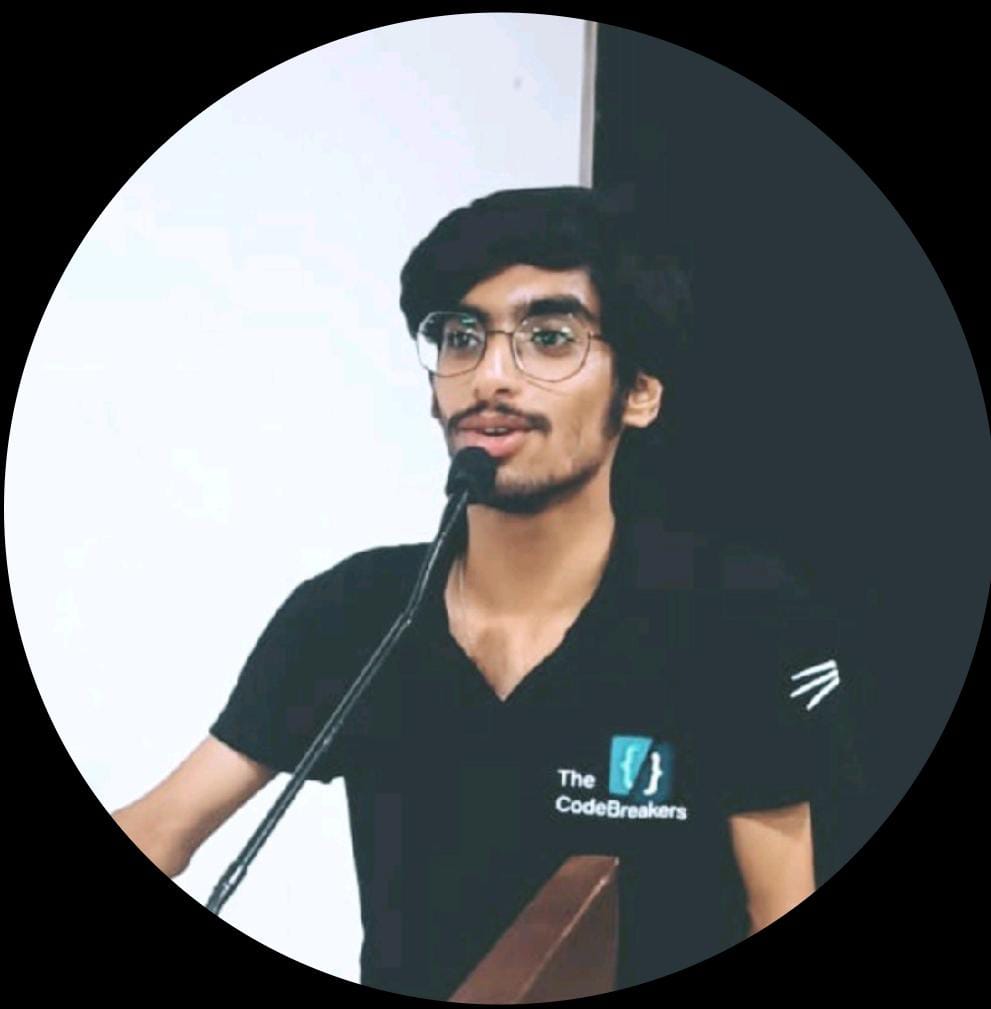
Tushar Pamnani
Tushar Pamnani
I am a MERN stack developer and a blockchain developer from Nagpur. I serve as Management Lead at ML Nagpur Community and The CodeBreakers Club, RCOEM.