React Essentials: JSX, Babel, Bundlers & Source Maps β A Beginnerβs Guide

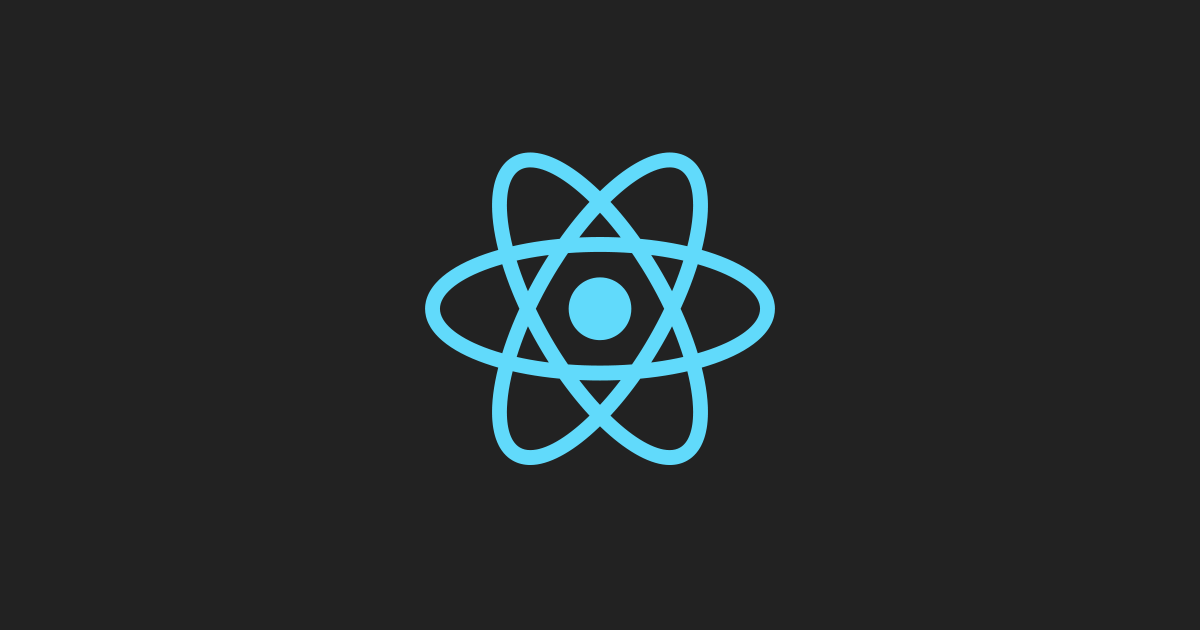
React Quick Notes π
1. What is a React Element?
A React Element is the smallest unit in React, like a DOM node but lightweight.
It is immutable (can't be changed after creation).
Created using JSX or
React.createElement()
.
π Example:
const element = <h1>Hello, React!</h1>; // JSX
const element2 = React.createElement('h1', {}, 'Hello, React!'); // Without JSX
2. What is JSX? And What is Babel?
JSX (JavaScript XML) allows writing HTML-like syntax inside JavaScript.
JSX is not valid JavaScript, so it needs to be compiled into pure JS.
Babel is a JavaScript compiler that converts JSX into normal JavaScript.
π Example JSX (before Babel):
const element = <h1>Hello, JSX!</h1>;
π After Babel (pure JavaScript):
const element = React.createElement('h1', {}, 'Hello, JSX!');
β JSX makes writing UI easier but must be transpiled by Babel before the browser understands it.
3. What is Source Map and Why is it Important?
A Source Map is a file that maps minified/bundled code back to the original source code.
Helps debugging by showing the original code instead of the minified version in the browserβs DevTools.
π Example:
Without Source Map β Shows
main.js:1:2344
(hard to understand).With Source Map β Shows
App.js:12
(actual line in source code).
β Important for debugging, but should be disabled in production for security reasons.
4. What is a Bundler? Why Do We Need It?
A Bundler takes multiple JS, CSS, and asset files and merges them into optimized files for the browser.
It improves performance, reduces file size, and makes code browser-friendly.
π Why We Need a Bundler?
β
Browsers can't understand import
from node_modules
.
β
Reduces HTTP requests by combining files.
β
Performs minification, compression, and tree shaking (removes unused code).
β
Supports CSS, images, and assets imports in JS.
5. How a Bundler Works?
1οΈβ£ Entry Point β Reads the main file (index.js
).
2οΈβ£ Dependency Resolution β Finds and processes import
statements.
3οΈβ£ Compilation & Transformation β Converts modern JS (ES6+) to older versions.
4οΈβ£ Optimization β Minifies, compresses, and removes unused code.
5οΈβ£ Output β Creates a single or few optimized files for the browser.
π Popular Bundlers:
Webpack β Most configurable.
Vite β Faster and modern (best for React).
Parcel β Zero-config, easy to use.
esbuild β Super fast.
β In React apps, Vite/Webpack makes everything smooth and optimized. π
π₯ Key Takeaways
β React Element β Smallest UI building block.
β JSX β Looks like HTML but compiles to JavaScript.
β Babel β Converts JSX into browser-compatible JS.
β Source Map β Helps debug minified code.
β Bundler β Packs, optimizes, and makes the app production-ready.
π For React projects, using Vite/Webpack is essential for performance & efficiency!
Subscribe to my newsletter
Read articles from Mohd Raza directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
