Naming Variables in Programming: Best Practices and Examples for TypeScript and JavaScript
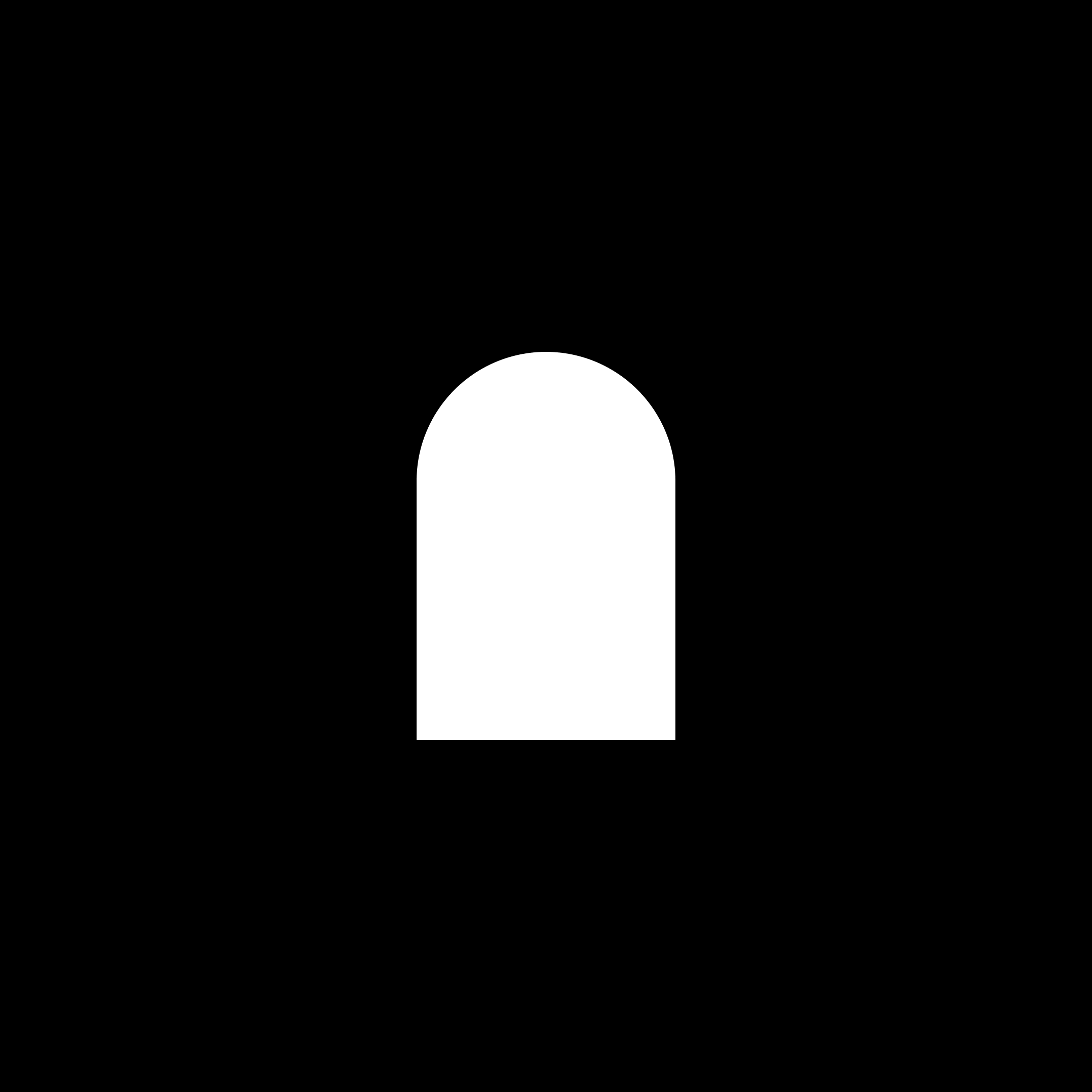
Variable naming is a foundational skill in programming that significantly affects how clear, maintainable, and readable your code is. A well-named variable communicates its purpose instantly, while a poorly named one can lead to confusion for you or your team. In this post, we’ll cover best practices for naming variables in TypeScript and JavaScript, explore common naming conventions with their specific uses, and share examples to help you write cleaner, more effective code.
Why Variable Naming Matters
Good variable names do more than label data—they make your code easier to understand, debug, and share. Whether you’re troubleshooting a bug or collaborating with others, clear naming saves time and reduces mistakes. Let’s see how to do it right in TypeScript and JavaScript.
Common Naming Conventions in TypeScript and JavaScript
Naming conventions bring consistency to your codebase. While TypeScript and JavaScript don’t enforce strict rules, certain styles are widely used:
camelCase: The go-to convention for variables and functions in JavaScript and TypeScript. It starts with a lowercase letter, and each subsequent word begins with an uppercase letter.
let userName: string = "Alice"; let itemCount: number = 10;
Application: Use camelCase for most variables and function names in TypeScript and JavaScript projects.
snake_case: Less common in these languages, but occasionally used for constants or in specific frameworks. Words are separated by underscores.
let user_age: number = 25; let total_items: number = 100;
Application: Snake_case might appear in constants or when working with libraries that favor this style, though it’s rare in mainstream JavaScript/TypeScript codebases.
PascalCase: Commonly used for class names, interfaces, and types in TypeScript. Every word starts with an uppercase letter.
class ShoppingCart {} interface UserData {}
Application: Reserve PascalCase for classes, interfaces, and custom types to distinguish them from variables and functions.
Best Practices for Naming Variables
Be Descriptive
Choose names that reveal what the variable represents. Avoid vague terms like data
or value
unless their meaning is obvious from context.
Bad Example:
let x: number = 42;
Good Example:
let userScore: number = 42;
Avoid Ambiguity
Steer clear of unclear abbreviations. Use full words or standard shorthand like num
for "number" instead of cryptic terms like usr
or cnt
.
Bad Example:
let usrNm: string = "Bob";
Good Example:
let userName: string = "Bob";
Naming Constants
Constants—values that won’t change—often use uppercase letters with underscores to signal their fixed nature.
Example:
const MAX_USERS: number = 100;
const API_KEY: string = "xyz123";
Reflect Scope and Context
A variable’s name can hint at its scope or purpose:
- Short names like
i
are fine for simple loop iterators:for (let i = 0; i < 10; i++) { console.log(i); }
- For variables with broader scope (e.g., across functions), use more descriptive names:
let totalParticipants: number = 0;
In complex scenarios, even loop variables can benefit from clarity:
for (let columnIndex = 0; columnIndex < columns.length; columnIndex++) {
console.log(columns[columnIndex]);
}
Stay Consistent
Pick a naming style and stick with it. If you use userId
in one place, don’t switch to userID
elsewhere.
Follow Language Norms
In TypeScript and JavaScript, camelCase is standard for variables and functions, while PascalCase is used for classes and interfaces.
More Examples
- Bad:
let t: string = "2023-10-15"; let arr: number[] = [1, 2, 3];
- Good:
let eventDate: string = "2023-10-15"; let numberList: number[] = [1, 2, 3];
Benefits of Good Naming
- Readability: Clear names make your code self-explanatory, reducing the need for comments.
- Maintainability: Descriptive names help you quickly grasp code logic later.
- Collaboration: Teammates can follow your work without decoding confusing names.
Conclusion
Effective variable naming is an easy way to improve your TypeScript and JavaScript programming. By using descriptive names, adhering to consistent conventions like camelCase for variables and PascalCase for classes, and aligning with language norms, you create code that’s easier to read, debug, and maintain. It’s a small step that pays off big—whether you’re working solo or with a team. So, give your variables names that make their purpose crystal clear.
References
Microsoft TypeScript Coding Guidelines
Official guidelines for writing TypeScript code, including naming conventions.MDN Web Docs: JavaScript Naming Conventions
Mozilla’s best practices for naming variables in JavaScript.Clean Code: A Handbook of Agile Software Craftsmanship by Robert C. Martin
Chapter 2 covers meaningful naming practices in programming.Google JavaScript Style Guide
Google’s official style guide for JavaScript, including naming recommendations.
Subscribe to my newsletter
Read articles from Dhruv Suthar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
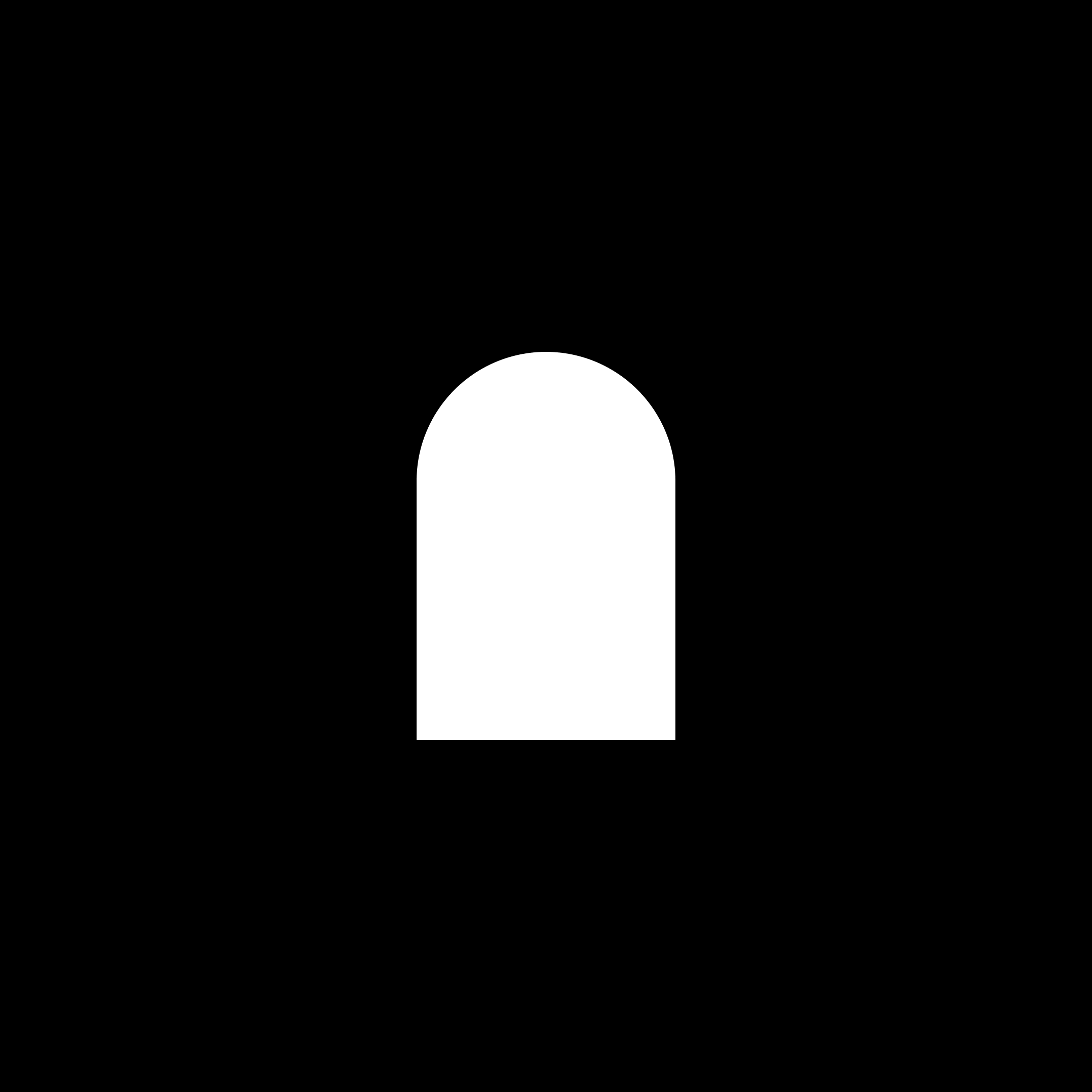
Dhruv Suthar
Dhruv Suthar
Building low-level, and minimal web experiences. Focusing on minimalism and monochromes.