Beginner JavaScript Guide 2025: Discover the Magic of Coding #1

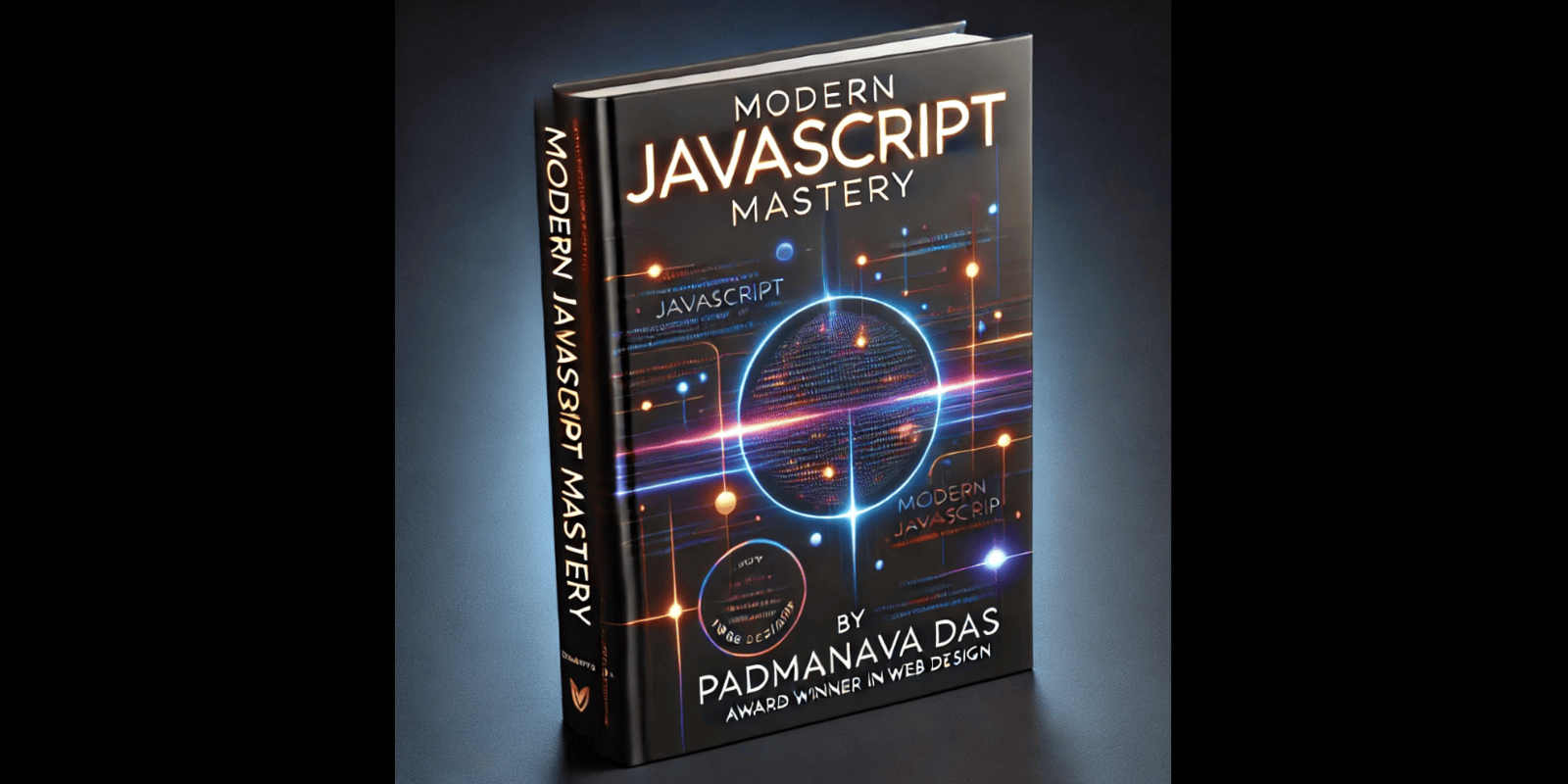
Friends!
Kamon acho?
Welcome to the very first post in our JavaScript series. Whether you are new to coding or have been programming for a long time, this article is crafted in a simple and enjoyable manner. Think of JavaScript as that magic wand which transforms ordinary web pages into interactive experiences, captivating everyone from a little child to an experienced developer!
What Exactly Is JavaScript?
JavaScript is a powerful programming language that brings interactivity to websites. Imagine it as a recipe book full of instructions that tell your web browser how to behave—whether it is reacting to a click, animating an image, or even performing a little digital dance. In short, JavaScript converts a static page into a lively, dynamic experience.
Setting Up Your Very Own JavaScript Playground
Before you start casting coding spells, you need a proper stage—a simple HTML file where you can run your JavaScript code. Here is a basic example that demonstrates how to embed JavaScript into your webpage:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>My JavaScript Playground</title>
</head>
<body>
<h1>Welcome to My Coding Playground!</h1>
<p>Get ready to witness some magic!</p>
<!-- Here is our inline JavaScript -->
<script>
// This line logs a friendly greeting to your browser's console.
console.log("Hello, Universe! JavaScript is working its magic.");
</script>
</body>
</html>
When you open this file in your browser, the code within the <script>
tag will run automatically, and you will see the greeting in the browser’s console. (Tip: Press F12 or right-click and select "Inspect" to view the console.)
Inline Scripts vs. External Scripts
For small experiments, writing your JavaScript code directly in the HTML file (as shown above) is perfectly acceptable. However, as your projects become larger, it is advisable to maintain your JavaScript code in separate files. This approach not only keeps your HTML tidy but also allows the browser to cache your code for quicker loading on subsequent visits.
Using Inline Scripts
The previous example demonstrates an inline script, where JavaScript code is directly embedded within the HTML document.
Using External Scripts
Below is an example of how you can link an external JavaScript file to your HTML:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>External JavaScript Magic</title>
<!-- Linking to an external JavaScript file named "app.js" -->
<script src="app.js" defer></script>
</head>
<body>
<h1>Magic from an External File!</h1>
<p>This page gets its magic from a separate file.</p>
</body>
</html>
And in your app.js
file, you may write:
console.log("Hello from the external file! JavaScript is doing wonders.");
The defer
attribute ensures that the script executes only after the HTML has been fully parsed.
you can use type="module"
instead of defer
in your script tag. When you specify a script as a module, it is deferred by default, which means it will run after the HTML is parsed. Plus, using modules allows you to use ES6 import/export features. However, note that module scripts have some additional rules, like being in strict mode automatically and not supporting inline scripts (you need separate files).
Below are two examples that show how to use defer
and type="module"
and explain their differences.
Example 1: Using defer
HTML File (index-defer.html):
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Defer Example</title>
<!-- This script will load after the HTML is parsed -->
<script src="defer-script.js" defer></script>
</head>
<body>
<h1>Defer Example</h1>
</body>
</html>
JavaScript File (defer-script.js):
// This is a normal deferred script that runs in the global scope.
console.log("This is a deferred script.");
// Variables declared here become properties of the global window object.
window.myDeferVar = "Hello from defer script!";
Key Points for defer
:
Execution Timing: The script is downloaded in parallel but executed only after the HTML document is fully parsed.
Global Scope: The script runs in the global scope, meaning variables and functions are added to the
window
object.No Module Features: You cannot use ES6 module features like
import
/export
in a deferred script.
Example 2: Using type="module"
HTML File (index-module.html):
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Module Example</title>
<!-- This script is treated as an ES6 module -->
<script src="module-script.js" type="module"></script>
</head>
<body>
<h1>Module Example</h1>
</body>
</html>
JavaScript File (module-script.js):
// This is a module script. It automatically runs in strict mode and in its own scope.
console.log("This is a module script.");
// Variables here do NOT automatically become global.
const myModuleVar = "Hello from module script!";
// You can export items from a module:
export const greeting = "Hello, module world!";
// Note: To use the exported items, another module must import them.
Key Points for type="module"
:
Execution Timing: Module scripts are deferred by default; they execute after the HTML is fully parsed.
Module Scope: The script runs in its own module scope. Variables and functions are not added to the global scope unless explicitly attached.
Strict Mode: Modules run in strict mode by default.
Module Features: You can use ES6 module syntax such as
import
andexport
to manage dependencies between files.
Summary of Differences
Scope:
Defer: Runs in the global scope. Variables become properties of
window
.Module: Runs in module scope. Variables are local unless exported.
Strict Mode:
Defer: Not automatically in strict mode (unless you add
"use strict";
).Module: Always in strict mode.
Module Syntax:
Defer: Cannot use
import
/export
.Module: Supports
import
/export
for modular code structure.
Execution Order:
- Both wait until after the HTML is parsed, but module scripts inherently support the modular system and extra features.
Using defer
is great when you simply want your script to run after the document loads without altering the global scope significantly, while type="module"
is ideal if you want to structure your code using modern JavaScript modules with better encapsulation and dependency management.
Getting to Know the Building Blocks
JavaScript code consists of individual instructions known as statements—each one like a sentence that tells the computer exactly what to do. Let’s look at some basics:
Statements: These are commands such as
console.log("Hello!");
that instruct the computer step by step.Semicolons: These act like full stops at the end of a sentence, marking the end of each statement. Although JavaScript can sometimes infer them, using semicolons is a good practice.
Comments: These are your personal notes in the code which help explain what you are doing. They are ignored by the computer. Use
//
for single-line comments and/* ... */
for multi-line comments.
For example:
// This is a single-line comment
console.log("Hello, Universe!"); // This prints a greeting
/*
This is a multi-line comment.
Use this to explain more complex parts of your code.
*/
let magicNumber = 42; // Declare a variable named magicNumber
console.log("The magic number is: " + magicNumber);
Let’s Have Some Fun with Interactivity
Now, let’s add a little interactivity to our page. How about a button that displays a friendly message when clicked? Have a look at this example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Interactive JavaScript Fun</title>
</head>
<body>
<h1>Click the Button for Some Magic!</h1>
<button id="magicBtn">Click Me!</button>
<script>
// Locate the button by its ID and attach a click event listener.
const magicBtn = document.getElementById('magicBtn');
magicBtn.addEventListener('click', function() {
// When the button is clicked, show a magical alert.
alert("Abracadabra! You have just unleashed the magic of JavaScript.");
});
</script>
</body>
</html>
In this example, clicking the button triggers an alert with a fun message. This clearly demonstrates how JavaScript can make a webpage interactive and engaging. If you don’t understand anything don’t worry! we’ll explain everything in detail in the upcoming blog. Just play with it!
Your First Coding Challenge
Now, it is your turn! Create a simple HTML page that:
Contains a heading and a paragraph.
Includes a button that, when clicked, displays a custom message (using
alert
orconsole.log
).
Try out both inline and external scripts. Once you have it working, experiment by changing the message or the button text. You may share your version with friends!
Wrapping Up
Today, we have uncovered the basics of JavaScript:
What JavaScript is and why it is the magic ingredient of the web.
How to set up your own coding playground using a simple HTML file.
The differences between inline and external scripts*.*
Fundamental concepts like statements, semicolons, and comments*.*
A simple interactive example that demonstrates JavaScript’s potential.
This is just the beginning of your journey into the world of JavaScript. In our upcoming posts, we will explore variables, functions, and many other exciting features of this versatile language.
Happy coding, and remember: every expert coder started exactly where you are now, exploring the wonders of JavaScript one step at a time!
Subscribe to my newsletter
Read articles from Padmanava Das directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Padmanava Das
Padmanava Das
From Republic of Bharat