Learn How to Use Java Try-Catch for Optimal Exception Management
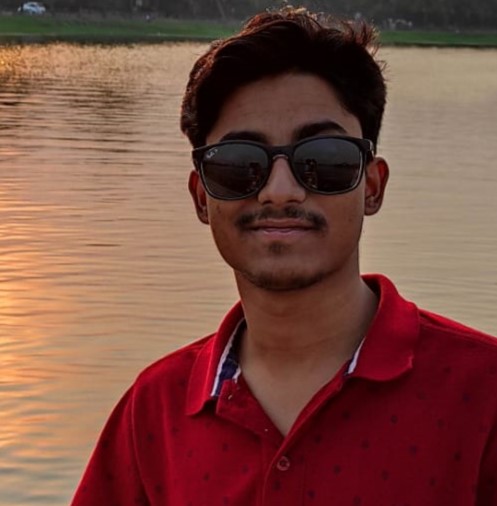

Handling exceptions is essential for writing reliable Java applications. Java offers the try-catch mechanism to catch errors and prevent program crashes. In this guide, we'll explain how try-catch works, how to manage multiple exceptions, and best practices for writing clean and efficient exception-handling code.
๐ 1. Understanding Try-Catch in Java
A try-catch block is used to detect and handle exceptions that may occur during program execution.
Basic Syntax of Try-Catch
try {
// Code that may cause an exception
} catch (ExceptionType e) {
// Handle the exception
}
Example: Handling Division by Zero
try {
int result = 10 / 0; // ๐จ ArithmeticException
} catch (ArithmeticException e) {
System.out.println("Cannot divide by zero!");
}
โ๏ธ How it works:
The code inside
try
executes first.If an exception occurs, execution jumps to the
catch
block.If no exception occurs,
catch
is skipped.
โก 2. Handling Multiple Exceptions
A program may throw different exceptions, and Java allows you to handle multiple exceptions separately using multiple catch
blocks.
Example: Handling Multiple Exceptions
try {
int[] arr = new int[5];
arr[10] = 50; // ๐จ ArrayIndexOutOfBoundsException
} catch (ArithmeticException e) {
System.out.println("Arithmetic Error");
} catch (ArrayIndexOutOfBoundsException e) {
System.out.println("Array Index Error");
} catch (Exception e) {
System.out.println("Some other error occurred");
}
โ๏ธ Key Points:
๐น More specific exceptions must be caught before the general Exception
class.
๐น If multiple exceptions are expected, use separate catch blocks for better error handling.
Alternative: Using Multi-Catch (Java 7+)
try {
String str = null;
System.out.println(str.length()); // ๐จ NullPointerException
} catch (ArithmeticException | NullPointerException e) {
System.out.println("Either Arithmetic or NullPointer Exception occurred");
}
๐น Multi-catch allows handling multiple exceptions in a single catch
block.
๐ฅ 3. Best Practices for Using Try-Catch in Java
โ๏ธ Use specific exceptions instead of Exception
class
โ Bad:
catch (Exception e) {
System.out.println("An error occurred");
}
โ Good:
catch (IOException e) {
System.out.println("File not found!");
}
โ๏ธ Log exceptions instead of printing them
โ Bad:
catch (IOException e) {
System.out.println("Error occurred");
}
โ Good:
catch (IOException e) {
e.printStackTrace(); // Logs detailed error message
}
โ๏ธ Avoid using empty catch blocks
โ Bad:
catch (Exception e) { } // Silent failure (Dangerous!)
โ Good:
catch (Exception e) {
System.out.println("An error occurred: " + e.getMessage());
}
โ๏ธ Use finally
block for cleanup (Closing files, database connections, etc.)
try {
FileReader file = new FileReader("data.txt");
} catch (FileNotFoundException e) {
System.out.println("File not found!");
} finally {
System.out.println("Execution complete.");
}
๐น The finally block always executes, whether an exception occurs or not.
๐ฏ Conclusion
try-catch
helps prevent program crashes by handling exceptions effectively.Multiple catch blocks allow handling different exceptions separately.
Best practices ensure clean, maintainable exception-handling code.
๐น Next Steps: In the next guide, weโll explore Checked vs Unchecked Exceptions in Java and when to handle them! Stay tuned. ๐
Let me know if you found this helpful! ๐
Subscribe to my newsletter
Read articles from Arkadipta Kundu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
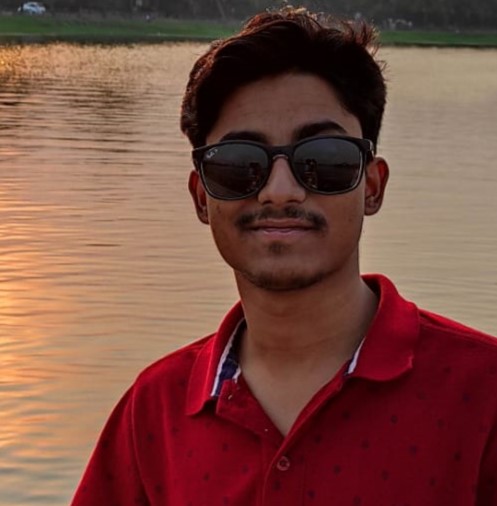
Arkadipta Kundu
Arkadipta Kundu
Iโm a Computer Science undergrad from India with a passion for coding and building things that make an impact. Skilled in Java, Data Structures and Algorithms (DSA), and web development, I love diving into problem-solving challenges and constantly learning. Right now, Iโm focused on sharpening my DSA skills and expanding my expertise in Java development.