π Day 2: JavaScript Functions β Regular vs. Arrow Functions π

Table of contents
- π Welcome to the 30-Days JavaScript Learning Journey!
- π Regular (Function Declarations & Expressions)
- 1οΈβ£ π What is a Function in JavaScript?
- 2οΈβ£ π Regular Functions (Function Declarations & Expressions)
- 3οΈβ£ Arrow Functions (ES6 Feature)
- 4οΈβ£ The this Keyword in Regular vs. Arrow Functions
- 5οΈβ£ Arrow Functions in Event Listeners
- 6οΈβ£ When to Use Regular vs. Arrow Functions?
- 7οΈβ£ Key Differences Between Regular and Arrow Functions:
- 8οΈβ£ Final Thoughts
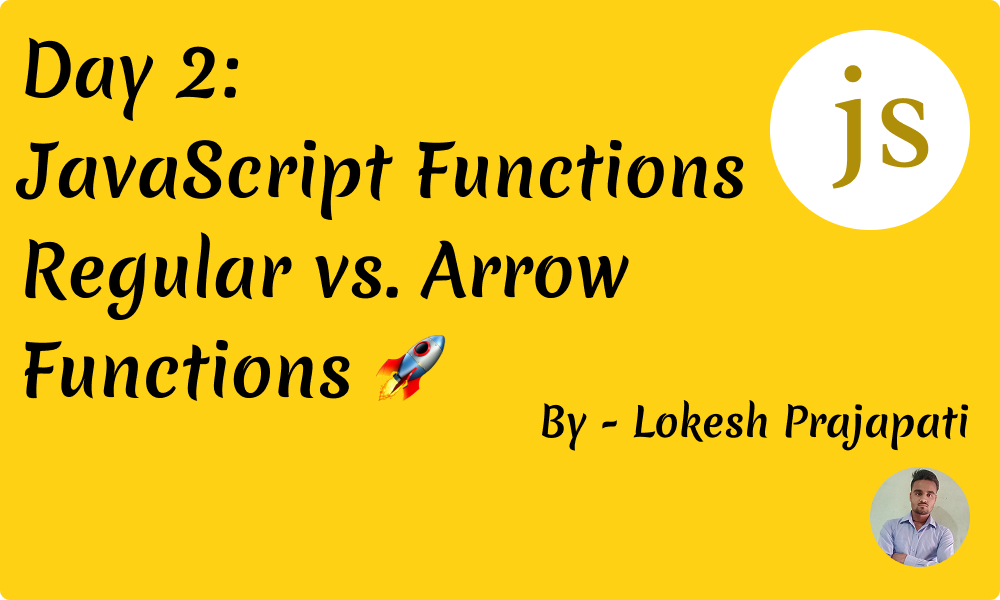
π Welcome to the 30-Days JavaScript Learning Journey!
JavaScript functions are the backbone of programming, allowing us to write reusable and maintainable code. There are two primary ways to define functions in JavaScript:
π Regular (Function Declarations & Expressions)
π Arrow Functions (ES6 Feature)
Today, weβll deep dive into these two types, compare their behavior, and understand when to use each. Letβs get started! π₯
β β β β β β β β β β β β β β β β β β β β β β β β β β β β β β β β
1οΈβ£ π What is a Function in JavaScript?
A function is a block of reusable code that performs a specific task. Instead of writing the same code multiple times, we can define a function once and call it whenever needed.
A function in JavaScript can be created using:
Function Declarations
Function Expressions
Arrow Functions (ES6)
Now, letβs explore them in detail.
β β β β β β β β β β β β β β β β β β β β β β β β β β β β β β β β
2οΈβ£ π Regular Functions (Function Declarations & Expressions)
β 2.1) Function Declaration (Named Function)
A function declaration is defined using the function
keyword and can be called before its definition (due to hoisting).
function helloGreeting(name) {
return `Hello, ${name}!`;
}
console.log(helloGreeting("User")); // β
"Hello, User!"
β Function declarations are hoisted, meaning they can be used before they are defined in the code.
β β β β β β β β β β β β β β β β β β β β β β β β β β β β β β β β
β 2.2 Function Expression (Anonymous Function)
A function expression is stored in a variable and is NOT hoisted, meaning it cannot be called before its definition.
const helloGreeting = function(name) {
return `Hello, ${name}!`;
};
console.log(helloGreeting("Bob")); // β
"Hello, Bob!"
β Hoisting Limitation Example:
console.log(sayHello()); // β ReferenceError: Cannot access 'sayHello' before initialization
const sayHello = function() {
return "Hello!";
};
π Fix: Use function declarations if you need hoisting.
β β β β β β β β β β β β β β β β β β β β β β β β β β β β β β β β
3οΈβ£ Arrow Functions (ES6 Feature)
Arrow functions were introduced in ES6 (ECMAScript 2015) to provide a shorter and cleaner syntax for writing functions.
const greet = (name) => `Hello, ${name}!`;
console.log(greet("Charlie")); // β
"Hello, Charlie!"
π₯ Arrow Function Syntax Variations
β 3.1 Single Parameter (No Parentheses Needed)
const square = num => num * num;
console.log(square(4)); // β
16
β 3.2 Multiple Parameters (Parentheses Required)
const add = (a, b) => a + b;
console.log(add(5, 10)); // β
15
β
3.3 Multi-line Body (Use {}
and return
)
const multiply = (a, b) => {
return a * b;
};
console.log(multiply(3, 4)); // β
12
β β β β β β β β β β β β β β β β β β β β β β β β β β β β β β β β
4οΈβ£ The this
Keyword in Regular vs. Arrow Functions
One of the biggest differences between regular and arrow functions is how this
is handled.
β
4.1 Regular Functions β this
is Dynamic : Regular functions have their own this
, which depends on how the function is called.
β
Regular functions work correctly with this
in objects.
const person = {
name: "Alice",
greet() {
console.log(`Hello, I am ${this.name}`);
}
};
person.greet(); // β
"Hello, I am Alice"
β 4.2 Arrow Functions β No Own this:
Arrow functions do not have their own this
and instead inherit it from their surrounding scope.
π Fix: Use a regular function inside objects to correctly reference this
.
const person = {
name: "Alice",
greet: () => {
console.log(`Hello, I am ${this.name}`);
}
};
person.greet(); // β "Hello, I am undefined"
β β β β β β β β β β β β β β β β β β β β β β β β β β β β β β β β
5οΈβ£ Arrow Functions in Event Listeners
Arrow functions do not bind this
, which can lead to unexpected results in event listeners.
document.querySelector("button").addEventListener("click", () => {
console.log(this); // β `this` is undefined
});
β
Use Regular Function for Correct this
Behavior
document.querySelector("button").addEventListener("click", function () {
console.log(this); // β
`this` refers to the clicked button
});
β β β β β β β β β β β β β β β β β β β β β β β β β β β β β β β β
6οΈβ£ When to Use Regular vs. Arrow Functions?
β Use Regular Functions When:
You need hoisting.
You need
this
to refer to the object (e.g., inside methods).You need access to the
arguments
object.
β Use Arrow Functions When:
You want a shorter syntax for simple functions.
You do not need
this
inside the function.You are using callback functions like
.map()
,.filter()
,.reduce()
.
β β β β β β β β β β β β β β β β β β β β β β β β β β β β β β β β
7οΈβ£ Key Differences Between Regular and Arrow Functions:
β β β β β β β β β β β β β β β β β β β β β β β β β β β β β β β β
8οΈβ£ Final Thoughts
Understanding the differences between regular functions and arrow functions will make you a better JavaScript developer. π
π‘ Key Takeaways:
Arrow functions are shorter, but they do not have their own
this
.Regular functions work better in objects and event listeners.
Function declarations are hoisted, but function expressions and arrow functions are not.
π¬ Which one do you use the most? Let me know in the comments! β¬οΈ
π Follow me for daily JavaScript posts from Basic to Advanced! π»π₯
β β β β β β β β β β β β β β β β β β β β β β β β β β β β β β β β
Subscribe to my newsletter
Read articles from Lokesh Prajapati directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Lokesh Prajapati
Lokesh Prajapati
π JavaScript | React | Shopify Developer | Tech Blogger Hi, Iβm Lokesh Prajapati, a passionate web developer and content creator. I love simplifying JavaScript, React, and Shopify development through easy-to-understand tutorials and real-world examples. Iβm currently running a JavaScript Basics to Advanced series on Medium & Hashnode, helping developers of all levels enhance their coding skills. My goal is to make programming more accessible and practical for everyone. Follow me for daily coding tips, tricks, and insights! Letβs learn and grow together. π‘π #JavaScript #React #Shopify #WebDevelopment #Coding #TechBlogger #LearnToCode