Introduction to MSB, Efficient Algorithms, and Gas Optimization in Blockchain Development
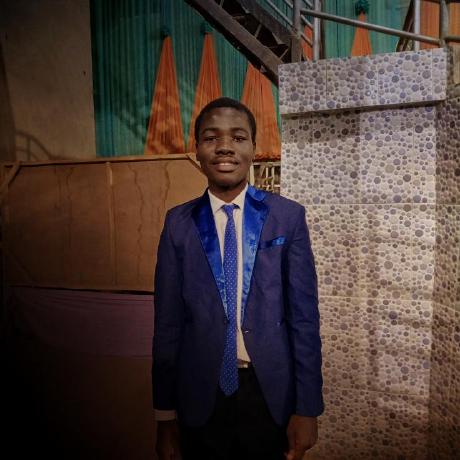

Introduction
In blockchain technology, the Most Significant Bit (MSB) refers to the highest-order bit in a binary number, representing its largest value. In the context of computing and data storage, understanding MSB helps optimize operations, as it plays a role in determining the magnitude and efficiency of data processing, especially when dealing with large numbers.
Efficient algorithms are crucial in blockchain development because they ensure that transactions and smart contracts can be processed swiftly and securely. Blockchains often handle vast amounts of data and complex computations, making the use of optimized algorithms necessary for reducing latency, ensuring scalability, and minimizing resource consumption.
Gas optimization in smart contracts is equally important. Gas represents the computational effort required to execute operations on a blockchain, particularly in Ethereum. By optimizing gas usage, developers can reduce transaction costs and improve the overall performance of decentralized applications (dApps). Efficient smart contracts ensure users and developers can interact with the blockchain in a cost-effective and seamless manner, increasing adoption and long-term sustainability.
What is the Most Significant Bit (MSB)?
The Most Significant Bit (MSB) is the leftmost 1 in the binary representation of a number. It represents the highest value bit in a binary number and determines the overall magnitude of the number.
Example:
For the number 13, its binary representation is 1101. The MSB is at position 3 (counting from 0 on the right), as it is the leftmost 1 in the binary string.
Why the MSB is Useful in Computing and Blockchain:
In Computing: The MSB helps in determining the size or magnitude of a number. It is crucial in tasks like integer comparisons, data storage, and optimizing algorithms for speed and memory efficiency.
In Blockchain: The MSB is important for verifying cryptographic signatures, transaction validation, and optimizing data structures, ensuring secure and efficient operations within decentralized systems. Understanding the MSB can also help in minimizing computational costs and reducing transaction fees in blockchain networks.
Why Does the MSB Matter in Blockchain?
In blockchain systems, the Most Significant Bit (MSB) plays a critical role in computational efficiency, directly impacting gas costs and overall performance. Gas refers to the computational effort required to execute operations on the blockchain, particularly in Ethereum and similar platforms. Each operation consumes a certain amount of gas, and users are charged based on the complexity of the task. Understanding how the MSB influences computational costs is essential for optimizing blockchain operations.
Gas Costs and Inefficient Algorithms
The gas cost of computations in blockchain is closely tied to the complexity of the operations. Inefficient algorithms can significantly increase gas consumption because they require more steps, iterations, and computations to achieve the same result. For instance, if a smart contract or transaction requires processing large numbers or involves complex calculations (like scaling token amounts or adjusting mining difficulty), the MSB will determine how much computational effort is required. Algorithms that fail to account for the MSB may perform unnecessary steps, leading to higher gas fees.
How Inefficient Algorithms Lead to High Gas Fees and Scalability Issues
When blockchain algorithms are inefficient, they require more resources to process data, resulting in higher gas fees. This increases the cost of performing operations on the network and can also lead to scalability issues. As more users interact with the blockchain, these inefficiencies become magnified, causing network congestion and slower transaction processing times. By optimizing algorithms to reduce reliance on excessive computations, especially those involving the MSB, blockchain platforms can become more cost-effective and scalable.
By optimizing how algorithms interact with the MSB, blockchain platforms can reduce unnecessary computational costs, ensure fair pricing for users, and improve scalability for widespread adoption.
Linear Search vs. Binary Search: A Comparison
Linear Search:
How it works: Linear search checks each bit one by one to find the desired value.
Steps for uint256 number: For a 256-bit number, linear search could require up to 256 iterations, as it checks each bit sequentially.
Gas Cost: Linear search results in high gas costs due to its inefficient nature, involving many operations. Each iteration consumes gas, and when checking every bit individually, it leads to excessive computation.
Binary Search:
How it works: Binary search divides the search space into halves, repeatedly narrowing down the problem and focusing on the half that contains the desired value. This approach leverages the concept of logarithmic time complexity.
Steps for uint256 number: For a 256-bit number, binary search only takes 8 steps (log₂(256) = 8) because it continuously divides the search space in half.
Gas Cost: Binary search has a low gas cost compared to linear search because it drastically reduces the number of operations required to find the value, making it far more efficient.
Key Differences:
Efficiency: Binary search is exponentially more efficient than linear search in terms of both time and gas usage.
Scalability: As numbers get larger (e.g., increasing from uint256 to uint512), linear search's computational cost grows linearly, while binary search remains logarithmic, making it far more scalable and cost-effective for large datasets.
In blockchain development, using binary search over linear search is a smart way to optimize gas consumption and improve overall efficiency in smart contract operations.
Feature | Linear Search | Binary Search | |
Time Complexity | O(n) (256 steps) | O(log n) (8 steps) | |
Gas Cost | High | Low | |
Scalability | Poor | Excellent |
This table highlights the key differences between Linear Search and Binary Search in terms of performance, gas costs, and scalability, emphasizing the significant advantages of Binary Search in blockchain development.
Gas Savings in Action: Real-World Example
Let's calculate the gas cost of finding the Most Significant Bit (MSB) using both Linear Search and Binary Search in a blockchain context. To illustrate the savings, we will use Solidity code for both methods.
Linear Search (Finding MSB)
In linear search, we check each bit of the number sequentially, one by one, from the least significant to the most significant bit. For a uint256, this approach could take up to 256 iterations, resulting in significant gas consumption.
// Linear Search to find MSB in Solidity
pragma solidity ^0.8.0;
contract MSBLinearSearch {
function findMSB(uint256 number) public pure returns (uint256) {
uint256 msb = 0;
// Iterate through all bits (256 bits for uint256)
for (uint256 i = 255; i >= 0; i--) {
if (number & (1 << i) != 0) {
msb = i;
break; // Return as soon as the MSB is found
}
}
return msb;
}
}
Gas Cost of Linear Search:
- This approach requires 256 iterations for a uint256 number. Each iteration involves a bitwise operation and a comparison, leading to higher gas costs due to the excessive number of computations.
Binary Search (Finding MSB)
In binary search, the problem is divided in half repeatedly, narrowing down the search space. For a uint256 number, binary search will only take 8 steps (log₂(256) = 8). This reduces gas consumption significantly.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract MSB {
function findMSB(uint256 number) public pure returns (uint8) {
require(number > 0, "Input must be greater than zero");
uint8 pos = 0;
if (number >= 2**128) { number >>= 128; pos += 128; }
if (number >= 2**64) { number >>= 64; pos += 64; }
if (number >= 2**32) { number >>= 32; pos += 32; }
if (number >= 2**16) { number >>= 16; pos += 16; }
if (number >= 2**8) { number >>= 8; pos += 8; }
if (number >= 2**4) { number >>= 4; pos += 4; }
if (number >= 2**2) { number >>= 2; pos += 2; }
if (number >= 2**1) { pos += 1; }
return pos;
}
}
Gas Cost of Binary Search:
- Binary search requires only 8 steps to find the MSB for a uint256 number. Each step involves fewer computations, making it far more efficient and significantly reducing gas costs.
Walkthrough the Binary Search Algorithm
Step | Condition | Action | number | pos | |
1 | number >= 2^128 | False | 13 | 0 | |
2 | number >= 2^64 | False | 13 | 0 | |
3 | number >= 2^32 | False | 13 | 0 | |
4. | number >= 2^16 | False | 13 | 0 | |
5. | number >= 2^8 | False | 13 | 0 | |
6. | number >= 2^4 | False | 13 | 0 | |
7. | number >= 2^2 | True: Shift right by 2, pos +=2 | 3 | 2 |
8. |
| True: Shift right by 1, | 1 | 3 |
9. | Terminate | Return | 1 | 3 |
For number = 13
, the binary search algorithm correctly determines that the MSB is at position 3
.
Comparison of Gas Savings:
Linear Search: Requires up to 256 iterations, resulting in high gas costs because each iteration consumes computational resources.
Binary Search: Requires only 8 iterations, leading to a drastic reduction in gas consumption. This efficiency makes it ideal for use in smart contracts, particularly when dealing with large numbers.
Example Gas Savings:
For a smart contract that finds the MSB, the Linear Search could result in hundreds of gas units used per operation, while the Binary Search would consume only a fraction of that, leading to significant savings—especially as the network scales with more users and transactions.
By using binary search, you not only save hundreds of iterations but also drastically reduce the computational load on the network, improving scalability and reducing overall costs for users.
Challenges and Trade-offs
While efficient algorithms like binary search provide significant gas savings and performance improvements, they often come with their own set of challenges.
Complexity of Code
Efficient algorithms can require more complex code compared to simpler, brute-force approaches. For example, while binary search reduces gas consumption and computational steps, implementing it requires careful handling of conditions, iterative steps, and proper handling of edge cases (e.g., ensuring the correct range for a uint256 number). This increases the difficulty of writing and maintaining the code.
Trade-off Between Development Time and Gas Savings
There is a trade-off between the time spent on optimizing an algorithm and the gas savings achieved. While the benefits of gas savings are significant, particularly in production environments with high transaction volumes, the upfront development time may increase due to the need for more sophisticated algorithms and their testing. Developers must balance the immediate cost of implementing an efficient algorithm with the long-term benefits of reduced gas costs and improved scalability.
Short-term: Simple algorithms may be faster to implement, but they will likely lead to higher gas costs.
Long-term: Optimized algorithms will save on gas, making the application more cost-efficient in the long run, even though they require additional development and testing.
The Importance of Testing and Benchmarking
Efficient code must be tested rigorously. Testing and benchmarking are essential steps to ensure that the optimized algorithm performs as expected without introducing new issues, such as bugs or unexpected behavior. With blockchain transactions involving real money or assets, any inefficiency or mistake could lead to significant financial losses.
Use unit tests and gas cost benchmarks to compare different implementations and ensure that the optimized solution delivers the expected gas savings.
Performance testing is crucial to verify that the optimizations actually scale well as the blockchain network grows.
Conclusion
In blockchain development, the Most Significant Bit (MSB) and efficient algorithms like binary search are powerful tools for enhancing performance and reducing gas costs. These optimizations play a critical role in improving scalability, lowering transaction costs, and enhancing the overall user experience on blockchain networks.
Encouraging developers to prioritize gas efficiency and optimize their smart contracts is crucial for the long-term sustainability of the ecosystem. By improving the underlying algorithms, developers can build blockchain systems that are not only faster and cheaper to use but also more scalable, creating a more robust environment for decentralized applications.
Call to Action:
Optimize your smart contracts, save gas, and build a better blockchain ecosystem!
Reach out to me for more questions or clarifications , need for collaboration and more…
Subscribe to my newsletter
Read articles from Olumide Adeola directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
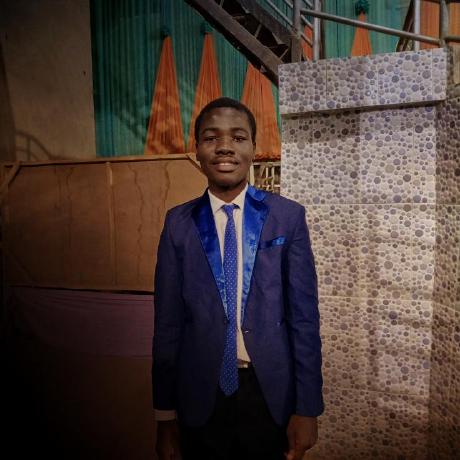
Olumide Adeola
Olumide Adeola
Classical | Health | Academics | Services et Solutions | Finance | Agriculture | Technology This is what my passion revolves round....