Analyzing and Optimizing Node Modules for Performance
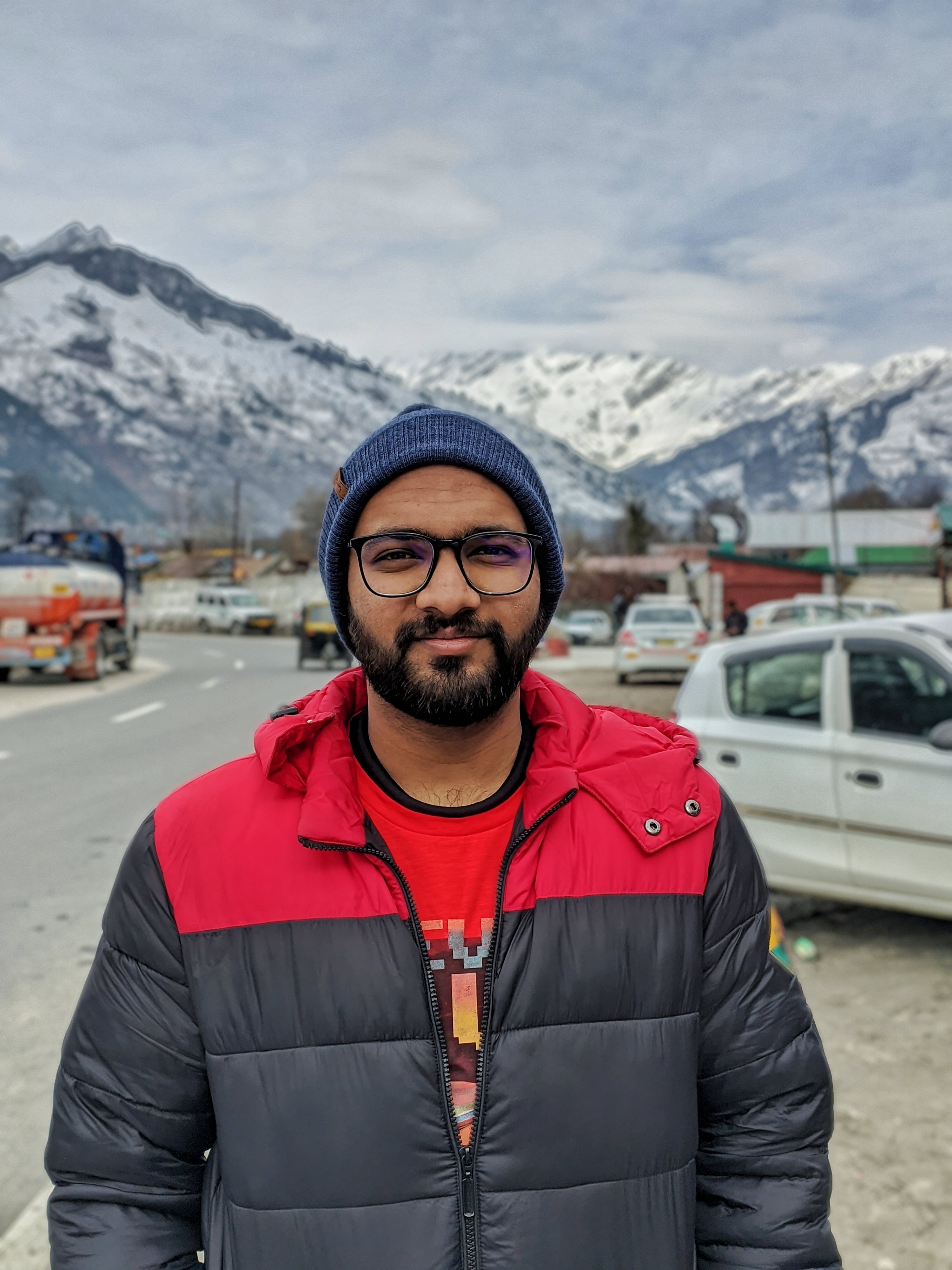

When it comes to Node projects, managing and optimizing the node_modules
folder is essential for improving performance, speeding up builds, and reducing bundle sizes. Large dependencies can slow down applications, extend deployment times, and even cause memory issues.
In this blog, we’ll explore how to analyze, check the size of, and optimize node_modules
effectively.
Why Analyze node_modules?
Performance: Bulky dependencies can slow down your application.
Faster Builds: Removing unnecessary dependencies speeds up the build process.
Smaller Bundles: Optimizing dependencies helps shrink frontend bundles, especially when using tools like Webpack or Vite.
Security: Eliminating unused or outdated packages reduces potential vulnerabilities.
1. Checking the Size of node_modules
Using the du Command (Disk Usage)
To quickly check the size of your node_modules
folder, run:
du -sh node_modules/
This command will display the total size of the node_modules
directory.
Using npm ls
To list the top-level dependencies installed, use:
npm ls --depth=0
This helps identify outdated or unnecessary dependencies.
Using package-size
The package-size
tool lets you check package sizes before installing them:
npx package-size <package-name>
This is useful for comparing alternative packages before adding them to your project.
2. Analyzing Dependencies with Tools
size-limit
size-limit
helps analyze JavaScript bundles and dependencies:
npm install --save-dev size-limit
Then, add this script to your package.json:
"scripts": {
"size": "size-limit"
}
Run the analysis with:
npm run size
This will show how much each dependency contributes to your bundle size.
webpack-bundle-analyzer
For frontend projects using Webpack, you can visualize module sizes by installing:
npm install --save-dev webpack-bundle-analyzer
Then, add it to your Webpack configuration:
const { BundleAnalyzerPlugin } = require('webpack-bundle-analyzer');
module.exports = {
plugins: [new BundleAnalyzerPlugin()]
};
Run your build process and open the generated report to analyze your bundle.
3. Optimizing node_modules
1. Remove Unused Dependencies
Find unused dependencies with:
npx depcheck
Then, remove unnecessary packages:
npm uninstall <package-name>
2. Use Lighter Alternatives
Replace heavy libraries with lightweight alternatives. Examples:
lodash
→lodash-es
(tree-shakable)moment.js
→date-fns
ordayjs
3. Enable Tree Shaking
If using Webpack, ensure tree shaking is enabled:
mode: 'production'
Also, use ES module imports instead of require()
, for example:
import { debounce } from 'lodash';
4. Run npm dedupe
To remove duplicate packages, run:
npm dedupe
5. Compress node_modules with pnpm
pnpm
optimizes module storage using symlinks:
npm install -g pnpm
pnpm install
This reduces node_modules
size significantly.
6. Optimize Docker Builds
If you're using Docker, improve caching by copying only package.json
before installing dependencies:
COPY package.json package-lock.json ./
RUN npm ci
COPY . .
This speeds up Docker builds by caching dependencies efficiently.
4. Optimizing node_modules for Production
1. Use NODE_ENV=production
Ensure development dependencies are not installed in production:
NODE_ENV=production npm install --omit=dev
2. Minify and Bundle Code
For frontend apps, minify and bundle your code using Webpack or esbuild
:
npm install --save-dev terser-webpack-plugin
Update your Webpack configuration:
const TerserPlugin = require('terser-webpack-plugin');
module.exports = {
optimization: {
minimize: true,
minimizer: [new TerserPlugin()]
}
};
3. Optimize Server Dependencies
For backend applications, use pkg
to bundle Node.js apps into standalone executables:
npm install -g pkg
pkg .
4. Remove Source Maps and Unnecessary Files
Reduce deployment size by removing source maps and other unnecessary files:
find node_modules -name "*.map" -type f -delete
5. Use npm ci for Consistent Installs
For reproducible and clean installs in production, use:
npm ci --only=production
Conclusion
Optimizing node_modules
is essential for performance and efficiency. Using tools like size-limit
, webpack-bundle-analyzer
, and depcheck
can help identify and remove bloat. Switching to lightweight dependencies and using package managers like pnpm
can further enhance project efficiency.
For production, keep dependencies minimal, minify and bundle code, and remove unnecessary files to ensure lean and efficient deployments. By following these best practices, you can keep your project fast, scalable, and maintainable.
Image Credits: ChatGPT.
Subscribe to my newsletter
Read articles from Akshay Raichur directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
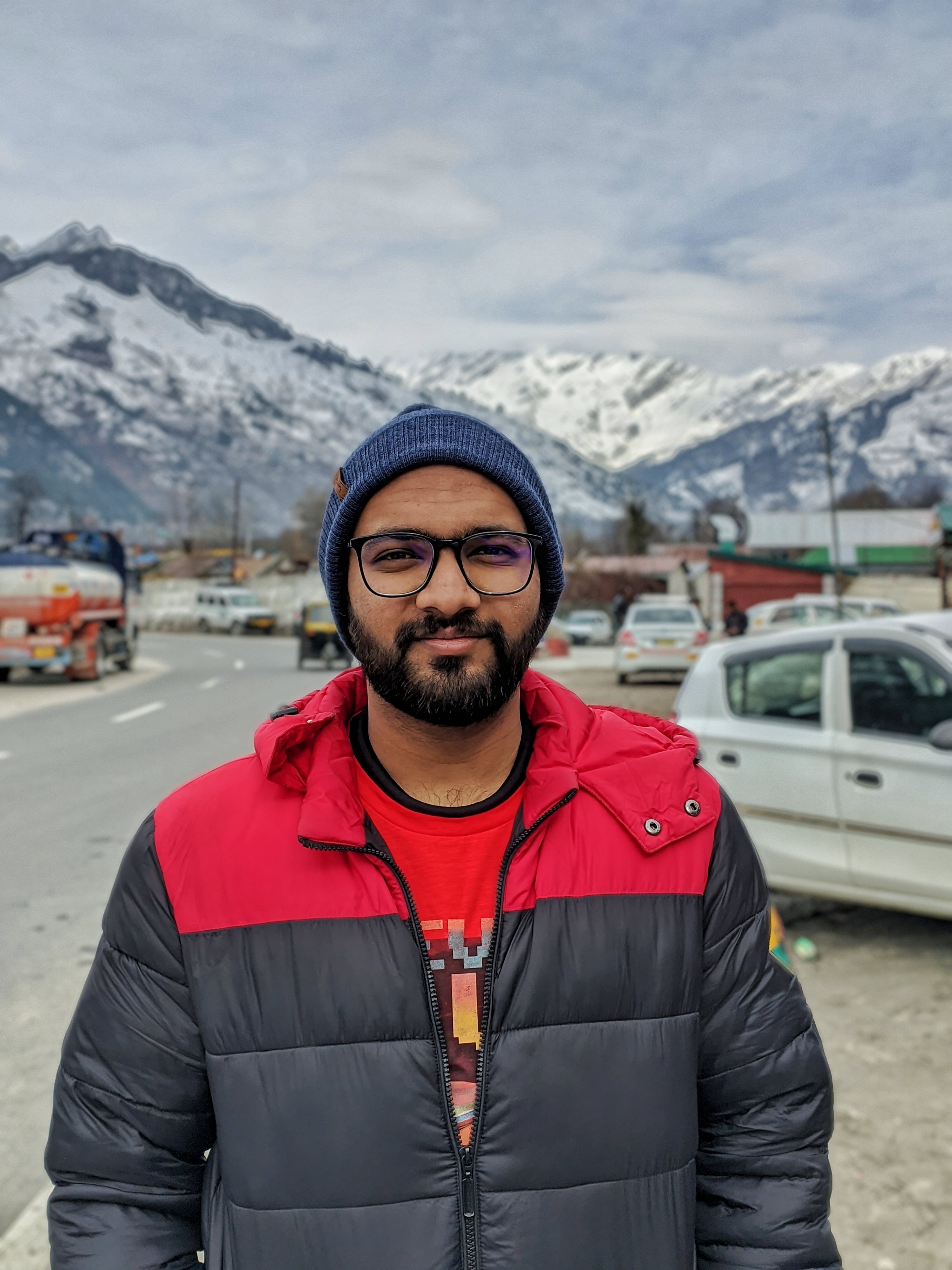
Akshay Raichur
Akshay Raichur
Software Engineer at Meesho, who loves JavaScript & web ❤️ Startup Enthusiast | Travel 🛫 | Photography 📸 | Badminton 🏸 | Tech 💻