Simple Drag-and-Drop Sorting for React
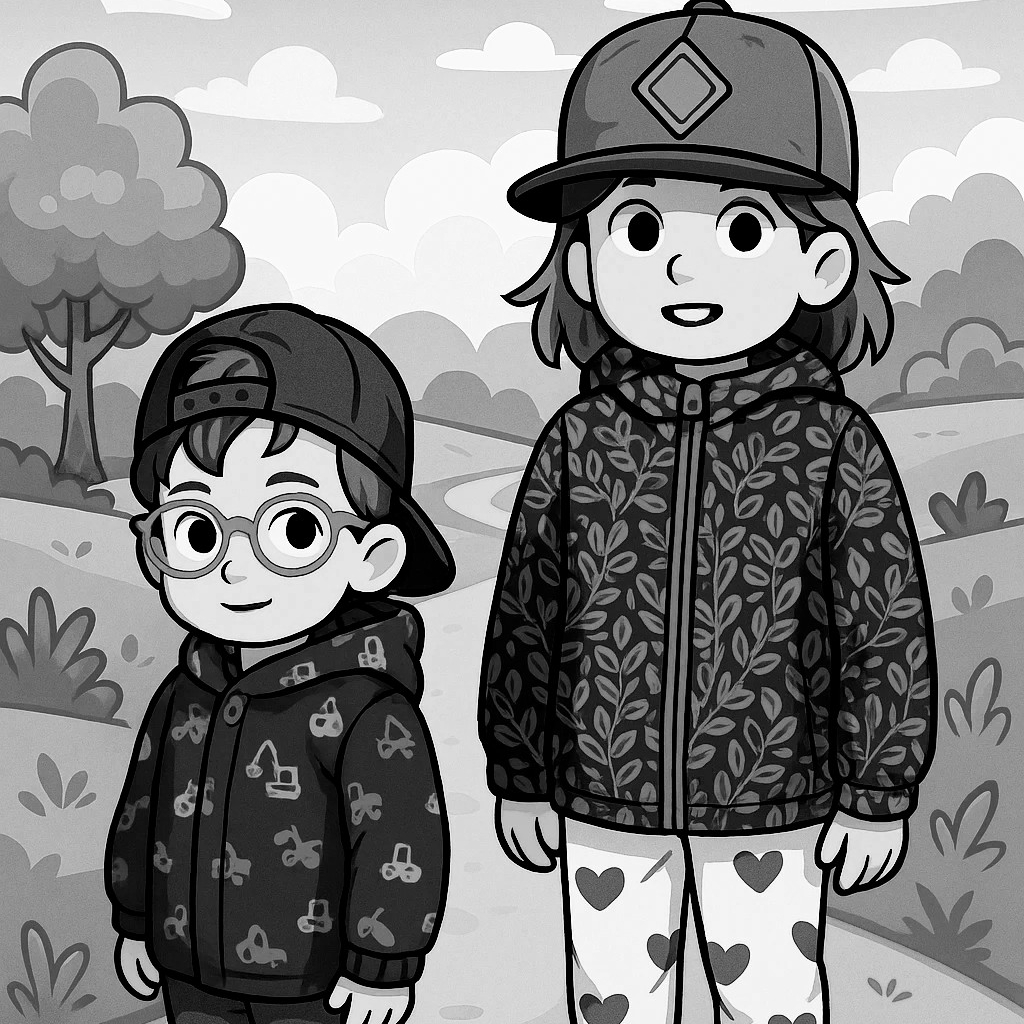

Have you ever needed a simple drag-and-drop sorting feature in your React app, but got overwhelmed by large libraries with unnecessary features? If you're like me, especially when working on SPFx projects, you just want users to rearrange list items—nothing complicated. That's why I created @spfxappdev/sortable, a lightweight and easy solution for basic sorting needs.
The Problem: Overkill Libraries
There are a variety of drag-and-drop libraries for React. But many of them have too much extra stuff: complex animations, lots of settings and large file sizes. For simple tasks, like reordering a list of elements in an SPFx component, these libraries can be too much.
The Solution: @spfxappdev/sortable
- Keep It Simple
@spfxappdev/sortable
is designed to be the opposite of these feature-rich libraries. It's a minimalist React component that provides basic drag-and-drop sorting functionality without any extras. Here's what makes it stand out:
Lightweight: Small bundle size, ensuring your application stays lean (unpacked ~62kb).
Simple API: Easy to use and integrate into your existing projects.
No Dependencies: Zero external dependencies, minimizing potential conflicts (except React, of course).
Basic Functionality: Focuses on the core task of reordering elements.
Why I Built It: SPFx and Beyond
My main reason for creating @spfxappdev/sortable
was my frequent need for simple sorting in SharePoint Framework (SPFx) projects. I often found myself wanting to give users the ability to customize the order of items in a list, but I didn't want to add a large and complex library to my project.
However, @spfxappdev/sortable
isn't just for SPFx. It's a flexible solution for any React project where you need basic drag-and-drop sorting without the extra weight.
How to Use @spfxappdev/sortable
Using @spfxappdev/sortable
is very easy. Here's a quick example:
import * as React from "react";
import {
Sortable
} from "@spfxappdev/sortable";
interface Item {
id: number;
text: string;
}
const SimpleList: React.FunctionComponent = () => {
const [items, setItems] = React.useState<Item[]>([
{ id: 1, text: "Item 1" },
{ id: 2, text: "Item 2" },
{ id: 3, text: "Item 3" },
]);
const handleOnChange = (
items: Item[],
changedItem?: Item,
oldIndex?: number,
newIndex?: number
) => {
// Update your state here. This example assumes you know which list changed.
// In a real app, you'd likely need to identify the list.
setItems([...items]);
};
return (
<div>
<Sortable
items={items}
onChange={handleOnChange}
>
{items.map((item: Item): JSX.Element => {
return (
<div key={item.id} className="list-item">
{item.text}
</div>
);
})}
</Sortable>
</div>
);
};
export default SimpleList;
As you can see, the API is straightforward:
Pass your list of items to the
items
prop.Provide a
onChange
callback to update the order of the items.Render your items as children of the
Sortable
component.
Installation
To install @spfxappdev/sortable
, simply run:
npm install @spfxappdev/sortable
When to Use @spfxappdev/sortable
@spfxappdev/sortable
is ideal for cases where:
You need basic drag-and-drop sorting.
You want to keep your bundle size low.
You prefer a simple and easy-to-use API.
You don't need fancy animations or complex features.
When to Look Elsewhere
If your project needs advanced features like:
Complex animations.
more complex drag and drop behaviors.
Then you may want to look for a more detailed library.
Conclusion
@spfxappdev/sortable
shows the power of simplicity. It's a lightweight and easy-to-use solution for basic drag-and-drop sorting in React. If you're tired of big libraries and just want a simple way to reorder your list items, give @spfxappdev/sortable
a try.
Demo
In the GitHub project repository in the “samples” folder you will find some examples that you can run locally.
Additionally you can find samples on codesandbox
Subscribe to my newsletter
Read articles from $€®¥09@ directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
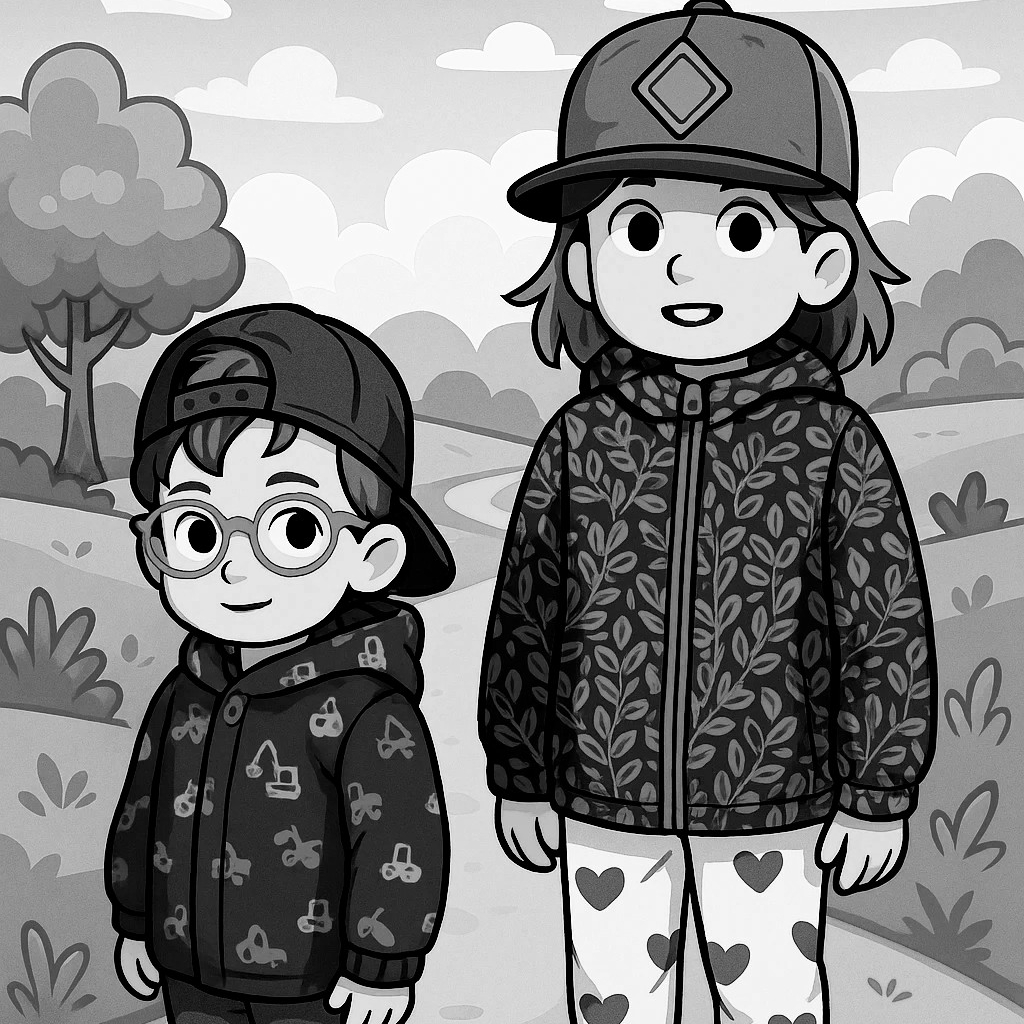
$€®¥09@
$€®¥09@
I am Sergej and I am a Software Architect from Germany (AURUM GmbH). I have been developing on Microsoft technologies for more than 14 years, especially in SharePoint / Microsoft 365. With this blog, I want to share my knowledge with you and also improve my English skills. The posts are not only about SPFx (SharePoint Framework) but also about tips & tricks around the M365 world & developments of all kinds. The posts are about TypeScript, C#, Node.js, Vue.js, Visual Studio/ VS Code, Quasar, PowerShell, and much more. I hope you will find some interesting posts. I would also be happy if you follow me. Greetings from Germany Sergej / $€®¥09@