Teleporting a Human — Understanding Serialization & Deserialization in JavaScript

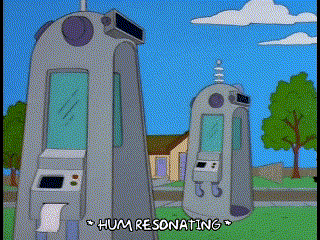
Introduction
Imagine if you could teleport anywhere instantly. One moment you're in New York, and the next you're in Tokyo. But what if, during teleportation, something went wrong? Maybe you lost an arm, or you ended up looking completely different. Pretty scary, right?
This is similar to what can happen with data when it's serialized (turned into a format for storage or transmission) and then deserialized (converted back to its original form). If not done correctly, data can be lost or misinterpreted—just like a teleportation mishap!
What is Serialization?
Serialization is the process of converting an object into a format that can be easily stored or transmitted, usually as JSON.
JavaScript Example of Serialization
const person = { name: "John Doe", age: 30, city: "New York" };
const serializedHuman = JSON.stringify(person); // Convert object to JSON
console.log(serializedHuman);
// Output: '{"name":"John Doe","age":30,"city":"New York"}'
Think of this as breaking down a person into a set of instructions for teleportation.
What is Deserialization?
Deserialization is the reverse process—reconstructing an object from its serialized format.
JavaScript Example of Deserialization
const deserializedHuman = JSON.parse(serializedHuman);
console.log(deserializedHuman.name); // John Doe
This is like reassembling the person at the destination based on the instructions.
Challenges in Teleportation (Serialization Issues)
Just as teleportation can have errors, serialization and deserialization come with their own pitfalls:
1. Data Loss (Losing an Arm in Teleportation 🚀)
Certain data types, such as functions, undefined
, and circular references, cannot be serialized using JSON.
const person = {
name: "Alice",
greet: function() { return "Hello!"; }
};
const serializedData = JSON.stringify(person);
console.log(serializedData);
// Output: '{"name":"Alice"}' (greet function is lost!)
Why? JSON does not support functions, so they get removed during serialization.
2. Corruption (Arriving at the Destination... Glitched! 😱)
If the serialized data is altered or incomplete, deserialization will fail.
try {
const brokenData = '{"name": "Bob", "age":}'; // Missing value
const parsed = JSON.parse(brokenData);
} catch (error) {
console.log("Oops! Teleportation failed: ", error.message);
}
// Output: "Oops! Teleportation failed: Unexpected token } in JSON"
Always ensure complete and valid data before deserialization.
3. Format Incompatibility (Teleporting to a Different Dimension 🌍)
Different systems may use different formats like XML instead of JSON, making it difficult to reconstruct the original data.
<!-- XML format instead of JSON -->
<person>
<name>John Doe</name>
<age>30</age>
</person>
💡 Solution: Use format converters or ensure both sender and receiver use the same format.
Handling Serialization Pitfalls in JavaScript
1. Using Custom Serialization Methods
To handle unsupported data types, we can define custom serialization rules:
const person = {
name: "Alice",
age: 25,
greet: function() { return "Hello"; }
};
const customSerialized = JSON.stringify(person, (key, value) =>
typeof value === "function" ? value.toString() : value
);
console.log(customSerialized);
// Output: '{"name":"Alice","age":25,"greet":"function() { return \"Hello\"; }"}'
Here, the function is converted into a string instead of being lost.
2. Handling Missing Data When Deserializing
Always validate and handle errors to prevent crashes.
try {
const brokenData = '{"name": "Bob", "age":}'; // Invalid JSON
const parsed = JSON.parse(brokenData);
} catch (error) {
console.log("Oops! Teleportation failed: ", error.message);
}
// Output: "Oops! Teleportation failed: Unexpected token } in JSON"
💡 Tip: Always validate JSON before parsing!
Conclusion
Serialization allows us to break down objects for transmission, just like teleportation. Deserialization reconstructs them at the destination, but if done incorrectly, data can be lost, corrupted, or misunderstood.
Best Practices for Safe "Teleportation" of Data:
Use toJSON()
methods for better control
Handle missing or corrupted data with error handling
Ensure both sender and receiver use the same format
Convert unsupported data types (functions, Dates, etc.) before serialization
Imagine the process of serialization and deserialization as a form of digital teleportation: while it allows data to be easily stored and transmitted, mistakes can lead to loss or corruption, much like a teleportation mishap. By understanding and addressing common challenges, such as handling unsupported data types and ensuring format compatibility, we can ensure data accuracy and integrity during these processes.
Final Thought:
If teleportation worked this way, would you risk using it? 🤔💭
Subscribe to my newsletter
Read articles from Ayush Bhagat directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
