The Hidden JavaScript Trick That Helped Kajal Ace Her Interview
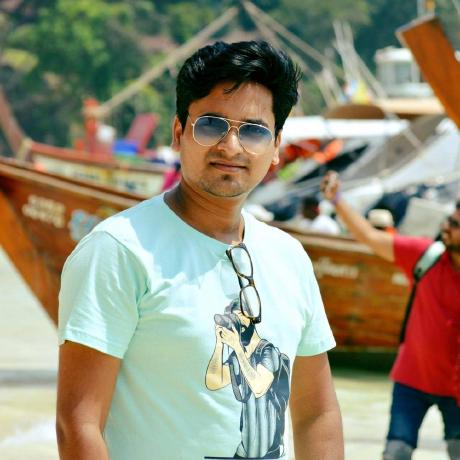
JavaScript coding interviews are a test of both technical skills and problem-solving ability. Let’s explore an inspiring interview scenario between Chirag, an experienced interviewer, and Kajal, a talented frontend developer eager to land her dream job.
The Interview Begins
Interviewer (Chirag): "Welcome, Kajal! Today, we’ll work on a frontend JavaScript problem to assess your problem-solving approach and coding skills. Are you ready?"
Candidate (Kajal): "Absolutely, Chirag. Let’s do this!"
Chirag: "Great! Here’s a challenge: Imagine you are implementing a search feature where users type in a search box, and an API request is made for each input change. How would you optimize this to ensure we don’t send too many requests?"
The Thought Process
Kajal starts analyzing the problem:
She recognizes that making a request for every keystroke would be inefficient.
She considers debouncing, which helps limit API calls but doesn’t prevent outdated responses from overriding newer ones.
She also thinks about how to cancel unnecessary requests.
Kajal: "I would use debouncing to reduce the number of API calls, but we also need a way to prevent outdated responses from interfering with newer ones. Maybe we can track ongoing requests?"
Chirag: "That’s a great thought! But how would you ensure that when a new request is made, any previous request is properly stopped?"
After a moment of reflection, Kajal recalls a JavaScript feature she had read about.
Kajal: "Oh! We can use AbortController
! It allows us to cancel an ongoing fetch request when a new one is triggered."
Chirag: "Exactly! Let’s see how you would implement that."
The Implementation
Kajal writes her solution:
let controller;
async function fetchData(query) {
if (controller) {
controller.abort(); // Cancel the previous request
}
controller = new AbortController();
const signal = controller.signal;
try {
const response = await fetch(`https://api.example.com/search?q=${query}`, { signal });
const data = await response.json();
console.log("Fetched Data:", data);
} catch (error) {
if (error.name === "AbortError") {
console.log("Fetch request was aborted");
} else {
console.error("Fetch failed:", error);
}
}
}
// Simulating user input triggering multiple requests
fetchData("JavaScript");
setTimeout(() => fetchData("React"), 500);
setTimeout(() => fetchData("Next.js"), 1000);
Challenges and Refinements
While her solution works, Chirag asks a few more questions:
What if the user types the same query again?
How can we ensure only the latest response is used?
What happens if there’s a network error?
Kajal enhances her code:
let controller;
let lastQuery = "";
async function fetchData(query) {
if (query === lastQuery) return; // Prevent duplicate requests
lastQuery = query;
if (controller) {
controller.abort();
}
controller = new AbortController();
const signal = controller.signal;
try {
const response = await fetch(`https://api.example.com/search?q=${query}`, { signal });
if (!response.ok) throw new Error("Network response was not ok");
const data = await response.json();
console.log("Fetched Data:", data);
} catch (error) {
if (error.name === "AbortError") {
console.log("Previous request aborted successfully");
} else {
console.error("Fetch failed:", error);
}
}
}
The Feedback Session
Chirag: "Fantastic job, Kajal! Let’s go over your solution."
Strengths
✅ Clear understanding of AbortController
and request cancellation. ✅ Implemented a clean approach to prevent stale requests. ✅ Handled errors gracefully, including network failures and aborted requests.
Areas for Further Enhancement
⚡ Consider using a debounce function to further optimize rapid API calls. ⚡ Explore using third-party libraries like Axios, which has built-in support for request cancellation. ⚡ Think about integrating UI feedback, like showing a loading indicator when fetching data.
Key Takeaways
🔹 Efficient API Calls
Use
AbortController
to cancel unnecessary requests and improve performance.Prevent race conditions where old responses overwrite new ones.
🔹 Optimized User Experience
Implement debouncing or throttling to reduce API calls.
Provide clear UI indicators for loading and errors.
🔹 Robust Error Handling
Catch and handle network failures.
Log and debug issues effectively.
Final Words from Chirag
Chirag: "Kajal, you have a strong problem-solving mindset and a solid grasp of JavaScript. With your ability to think ahead and optimize solutions, I’m happy to say—welcome to the team! Congratulations!"
Frontend interviews aren’t just about coding—they’re about designing efficient and user-friendly solutions. Keep learning, practicing, and optimizing! 🚀
Looking to take your coding skills to the next level? kodekarma.dev is your go-to platform for practicing frontend coding challenges. With curated problems, real-world scenarios, and guidance tailored for all levels, KodeKarma helps you prepare smarter and faster. Start your journey to interview success today!
Subscribe to my newsletter
Read articles from Chirag goel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
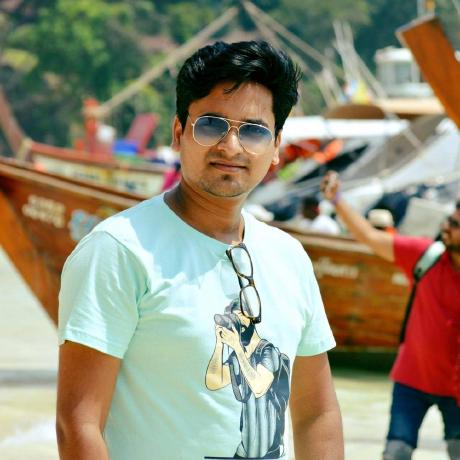