Exploring Configuration Changes in .NET Framework and .NET Core
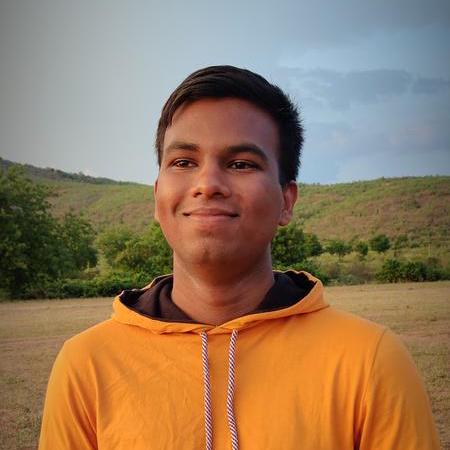
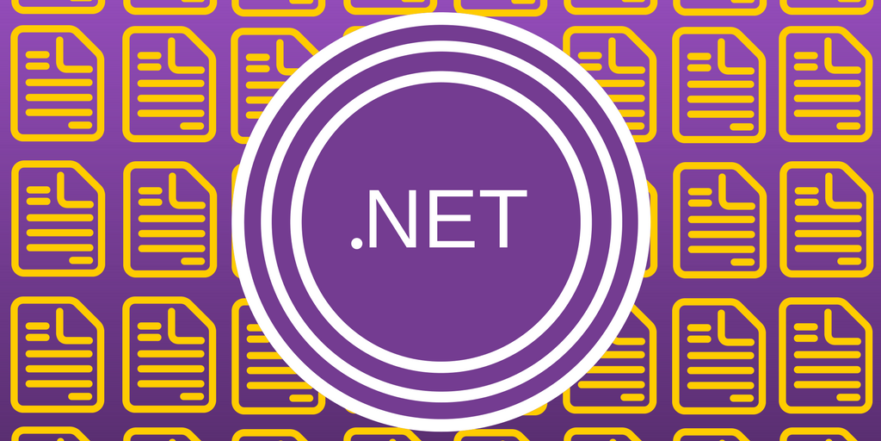
Configuration management is a cornerstone of application development, allowing flexibility and scalability across environments. While .NET Framework and .NET Core (now .NET) share similarities, their configuration paradigms are quite distinct due to fundamental differences in architecture and design philosophy. This blog explores these differences through hands-on examples using two simple C# projects.
Adding and Using Configuration in .NET Framework
- Create a new Console Application targeting .NET Framework using Visual Studio 2019.
Console App targeting .NET Framework
2. You can see an App.config
file created, under the project.
3. If it’s not already created then add App.config
file and open the file, you can add few custom-made settings, inside the App.config
file.
<?xml version="1.0" encoding="utf-8" ?>
<configuration>
<startup>
<supportedRuntime version="v4.0" sku=".NETFramework,Version=v4.7.2" />
</startup>
<appSettings>
<add key="firstName" value="Jane"/> <!-- Custom added values -->
<add key="lastName" value="Doe"/> <!-- Custom added values -->
</appSettings>
</configuration>
NOTE:
WhileappSettings
is the most commonly used section, there are many other sections available, or you can create your own custom sections.
4. Read Configuration in the code, using ConfigurationManager
from the App.config
.
using System;
using System.Configuration;
namespace Old_Configuration
{
class Program
{
static void Main(string[] args)
{
string fName = ConfigurationManager.AppSettings["firstName"];
string lName = ConfigurationManager.AppSettings["lastName"];
Console.WriteLine($"First Name: {fName}");
Console.WriteLine($"Last Name: {lName}");
Console.ReadLine();
}
}
}
NOTE:
In the above code, while compiling if you get the following error:
“The name ‘ConfigurationManager’ does not exist in the current context Old_Configuration”Then under the project section, go to references, and add reference to System.Configuration , this should fix the issue
5. Compile and Run !! you should see the following output…
Console output
Adding and Using Configuration in .NET Core
- Create a new Console Application targeting .NET Core using Visual Studio 2022.
2. Add an appsetting.json
file to the project.
{
"firstName": "Jane",
"LastName": "Doe"
}
complexSetting.json
(multiple json files)
{
"Address": {
"State": {
"Capital": "Moonland",
"District": "South Moon"
},
"Location": {
"Galaxy": "Milky way"
}
},
"Telephone": "Not Available"
}
3. Add the following NuGet packages Microsoft.Extensions.Configuration
and Microsoft.Extensions.Configuration.Json
to the project.
4. Use the ConfigurationBuilder
to read the JSON configuration.
using System;
using Microsoft.Extensions.Configuration;
namespace NewConfiguration
{
class Program
{
static void Main(string[] args)
{
var config = new ConfigurationBuilder()
.AddJsonFile("appsetting.json")
.AddJsonFile("complexSetting.json") // Adding the other json file also to the config
.Build();
string fName = config["firstName"];
string lName = config["lastName"];
string capital = config["Address:State:Capital"];
string galaxy = config["Address:Location:Galaxy"];
Console.WriteLine($"First Name: {fName}");
Console.WriteLine($"Last Name: {lName}");
Console.WriteLine($"Capital : {capital}");
Console.WriteLine($"Galaxy : {galaxy}");
}
}
}
NOTE:
Once complied might run into error, that the “**.json” file is not found.
Copy the respective “**.json” files to the generated exe path
5. Run the console app !! you should see the following output..
Console Output
GitHub Link: Blogs/Exploring Configuration Changes in .NET Framework and .NET Core at main · reddymahendra52/
Subscribe to my newsletter
Read articles from Mutukundu Mahendra Reddy directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
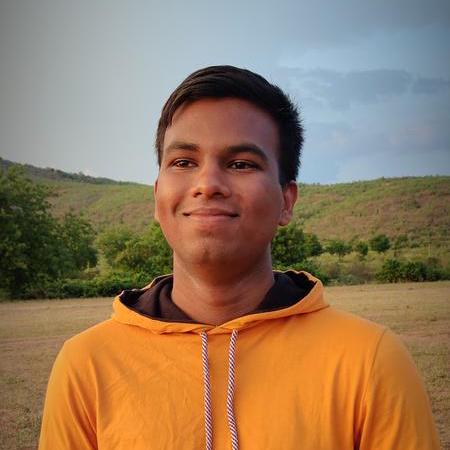