Optimizing Responsive Component Rendering in React: Solving the Mounting Problem
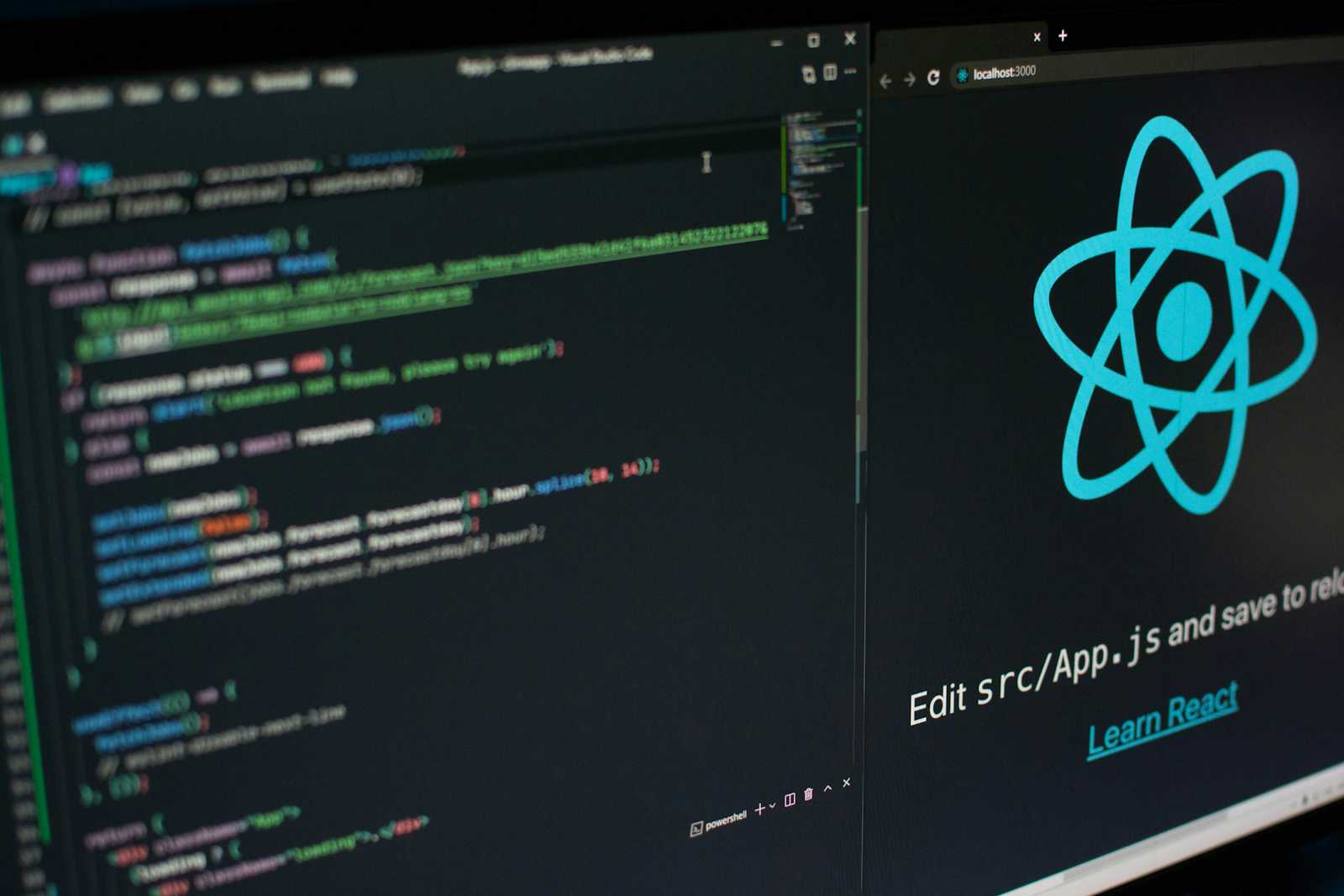
In modern web development, building responsive applications is a must. However, ensuring optimal performance while handling responsive designs can be challenging. One common issue developers face is the mounting problem, where unnecessary components are rendered in the DOM, even if they’re not visible. This can lead to performance bottlenecks, especially on low-end devices.
In this blog, we’ll explore how to solve this problem by leveraging conditional rendering, lazy loading, and the Resize Observer API. We’ll also walk through a practical implementation in a React project using Tailwind CSS for styling.
The Problem: Unnecessary Mounting of Components
When building responsive applications, developers often use CSS (e.g., display: none
or hidden
classes) to hide components that aren’t needed for a specific screen size. While this approach works, it has a significant drawback: all components are still mounted in the DOM, even if they’re not visible. This leads to:
Unnecessary Resource Usage: Hidden components still consume memory and processing power.
Increased Bundle Size: All components are loaded upfront, even if they’re not immediately needed.
Poor Performance: Frequent mounting and unmounting of components can cause performance issues, especially on low-end devices.
The Solution: Conditional Rendering, Lazy Loading, and Resize Observer
To solve this problem, we can use a combination of conditional rendering, lazy loading, and the Resize Observer API. Here’s how these techniques work together:
1. Conditional Rendering
Conditional rendering ensures that only the required component is rendered based on the current screen size. Instead of hiding components with CSS, we use a state variable (e.g., isMobile
) to determine which component to render.
Example:
{isMobile ? <MobileGrid data={data} /> : <DesktopTable data={data} />}
Benefits:
Reduced DOM Size: Only one component is rendered at a time.
Improved Performance: Avoids unnecessary rendering of hidden components.
2. Lazy Loading
Lazy loading ensures that components are only loaded when they’re needed. This is achieved using React.lazy
and Suspense
.
Example:
const DesktopTable = React.lazy(() => import('./DesktopTable'));
const MobileGrid = React.lazy(() => import('./MobileGrid'));
<Suspense fallback={<div>Loading...</div>}>
{isMobile ? <MobileGrid data={data} /> : <DesktopTable data={data} />}
</Suspense>
Benefits:
Reduced Initial Bundle Size: Components are split into separate chunks and loaded on demand.
Faster Initial Load: Only the necessary components are loaded upfront.
3. Resize Observer API
The Resize Observer API detects changes in the size of a container or viewport. This allows the application to dynamically switch between mobile and desktop views without relying on global window.resize
events.
Example:
useEffect(() => {
const container = containerRef.current;
const handleResize = debounce((entries) => {
for (let entry of entries) {
const { width } = entry.contentRect;
setIsMobile(width < 768); // Mobile/tablet breakpoint
}
}, 100); // Debounce to avoid excessive updates
const resizeObserver = new ResizeObserver(handleResize);
if (container) {
resizeObserver.observe(container);
}
return () => {
if (container) {
resizeObserver.unobserve(container);
}
};
}, []);
Benefits:
Granular Control: Observes specific elements rather than the entire viewport.
Efficient Updates: Only triggers re-renders when the container size changes.
Debouncing: Limits the frequency of updates to improve performance.
Implementation in a React Project
Let’s walk through how to implement this solution in a React project using Tailwind CSS for styling.
Step 1: Refactor Components
Divide your existing component into two separate components: one for mobile/tablet view (MobileGrid
) and one for desktop view (DesktopTable
).
Example:
// DesktopTable.jsx
const DesktopTable = ({ data }) => {
return (
<table className="min-w-full bg-white border border-gray-200">
{/* Table content */}
</table>
);
};
// MobileGrid.jsx
const MobileGrid = ({ data }) => {
return (
<div className="grid grid-cols-1 sm:grid-cols-2 gap-4">
{/* Grid content */}
</div>
);
};
Step 2: Use Conditional Rendering and Lazy Loading
In your main component, use React.lazy
and Suspense
to conditionally render the appropriate component.
Example:
const DesktopTable = React.lazy(() => import('./DesktopTable'));
const MobileGrid = React.lazy(() => import('./MobileGrid'));
function App() {
const [isMobile, setIsMobile] = useState(false);
const containerRef = useRef(null);
useEffect(() => {
// Resize Observer logic
}, []);
return (
<div ref={containerRef}>
<Suspense fallback={<div>Loading...</div>}>
{isMobile ? <MobileGrid data={data} /> : <DesktopTable data={data} />}
</Suspense>
</div>
);
}
Step 3: Replace Tailwind Media Queries
Replace your existing Tailwind media queries with the new logic to ensure only the required component is rendered.
Benefits of This Approach
Reduced DOM Size: Only one component is rendered at a time.
Smaller Bundle Size: Components are loaded on demand.
Efficient Updates: Resize Observer and debouncing ensure updates are triggered only when necessary.
Improved User Experience: Faster load times and smoother transitions between views.
Conclusion
By leveraging conditional rendering, lazy loading, and the Resize Observer API, we can solve the mounting problem and optimize the performance of responsive applications. This approach ensures that only the necessary components are rendered and loaded, resulting in a more efficient and scalable solution.
If you’re currently using Tailwind CSS media queries to handle responsive behavior, consider refactoring your components and adopting this approach for better performance and maintainability.
Let me know your thoughts in the comments! Have you faced similar challenges in your projects? How did you solve them? 🚀
Subscribe to my newsletter
Read articles from Roshan Singh bhadauriya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Roshan Singh bhadauriya
Roshan Singh bhadauriya
Hi, I'm Roshan Singh Bhadauriya. A passionate Front-end Developer based in Dewas, India.