Breaking the Silence
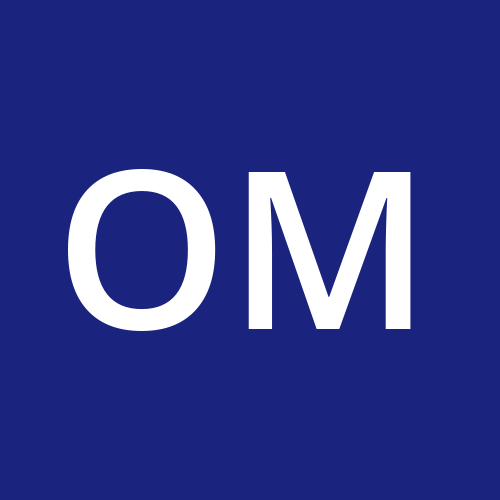
My Journey into Golang
For months, I've been contemplating writing about my experiences with Golang. Each time I sat down to document what I've learned, the words never seemed quite right. I'd start typing, delete everything, and close my editor in frustration. The blank page became an intimidating adversary, and the perfect introduction to my Golang journey remained elusive.
Today, I've had an epiphany: there will never be "perfect" words. No matter how long I wait or how many drafts I create, I'll never feel fully satisfied with how I express my coding journey. So I've made a decision - I'm going to start documenting my Golang learning process on GitHub, one piece at a time. Rather than striving for polished perfection, I'll focus on sharing authentic insights and practical knowledge as I acquire them.
Beginning this week, I commit to publishing at least three articles weekly about different aspects of Golang that I'm exploring. Each article will delve into specific concepts I've encountered, challenges I've overcome, and the practical applications I've discovered. My first piece examines a simple Rock Paper Scissors implementation that demonstrates Golang fundamentals like package structure, imports, constants, variables, and functions. Through these regular posts, I hope to not only solidify my own understanding but also help others who might be on similar learning paths.
Rock Paper Scissors: A Simple Introduction to Golang's Basics
Let's break down this Rock Paper Scissors implementation to understand some fundamental Golang concepts:
package main
// always start a file with package main
import(
"fmt" // import fmt for printing to the console
"math/rand" //the equivalent of import random.randint in python to generate random numbers
)
//consts can never be changed after they are declared, unlike variables which can change value
const(
Rock = 0
Paper = 1
Scissor = 2
)
//var are subject to change and can hold values of different types
var actions = map[int] string{
Rock: "Rock",
Paper: "Paper",
Scissor: "Scissors",
}
//func is our function that takes no arguments but returns nothing (void). It has access to all other functions and variables within this
func main(){
// Println prints out the specified values and automatically appends a newline character at the end.
fmt.Println("Welcome to My Rock Paper Scissors")
fmt.Println("Like it says you have 3 Choices Rock Paper Scissors")
fmt.Println("Rock = 0\nPaper = 1\nScissors = 2")
var userInput int
for {
fmt.Print("Choose your move: ")
_, err := fmt.Scan(&userInput)
if err == nil && (userInput >= Rock && userInput <= Scissor){
break
}else{
fmt.Println("Invalid Input. Please enter either 0 or 1 or 2")
}
}
computerInput := rand.Intn(3) // rand.Intn returns a random number
var result string //var always suggest to change
if userInput == Rock && computerInput == Scissor{
result = "Congratulations! You Win!"
}else if userInput == Paper && computerInput == Rock{
//elif python == else if in go
result = "Congratulations! You Win!"
}else if userInput == Scissor && computerInput == Paper{
result = "Congratulations! You Win!"
}else if userInput == computerInput{
result = "It's a Tie!"
}else{
result = "You Lost"
}
computerAction := actions[computerInput]
// so this was the most interesting one := you get to declare and assign which is a short form of it by giving it a new variable
userAction := actions[userInput]
// fmt.Println(computerAction)
// fmt.Println(computerInput)
fmt.Println(result ,"\nUser's action was",userAction,"\nComputer's action was",computerAction)
}
Key Takeaways:
Package Declaration: Every Go file begins with a package declaration. For executable programs, the main package is required.
Imports: Go uses explicit imports for external packages. The
fmt
package provides formatting functions, whilemath/rand
gives us random number generation.Constants vs Variables:
Constants (
const
) are immutable values that cannot change after declarationVariables (
var
) can be modified throughout program execution
Data Structures: The program uses a map (similar to dictionaries in Python) to associate numeric choices with their string representations.
Function Structure: The
main()
function serves as the entry point for execution.Input Handling: Go provides robust error handling for user input through the
fmt.Scan()
function.Variable Declaration Shorthand: The
:=
operator provides a concise way to declare and initialize variables in one step.Control Flow: The program demonstrates conditional logic with
if/else if/else
structures and loops withfor
.
In future articles, I'll explore more complex Go concepts like goroutines, channels, interfaces, and building web applications. Stay tuned as I continue documenting my Golang journey!
Follow my learning journey on GitHub and connect with me on LinkedIn.
Subscribe to my newsletter
Read articles from Onesmus Dzidzai Maenzanise directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
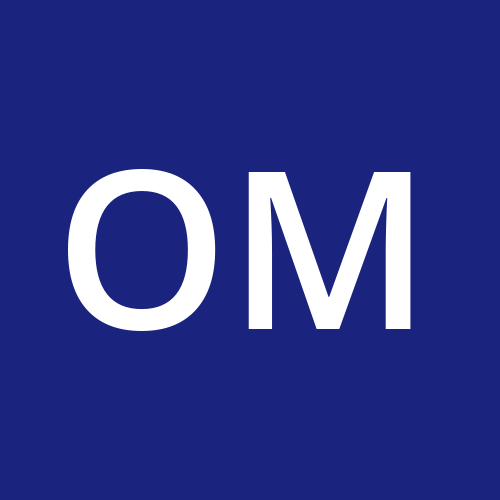