How to Add Background Image and Color in Word: Python Guide

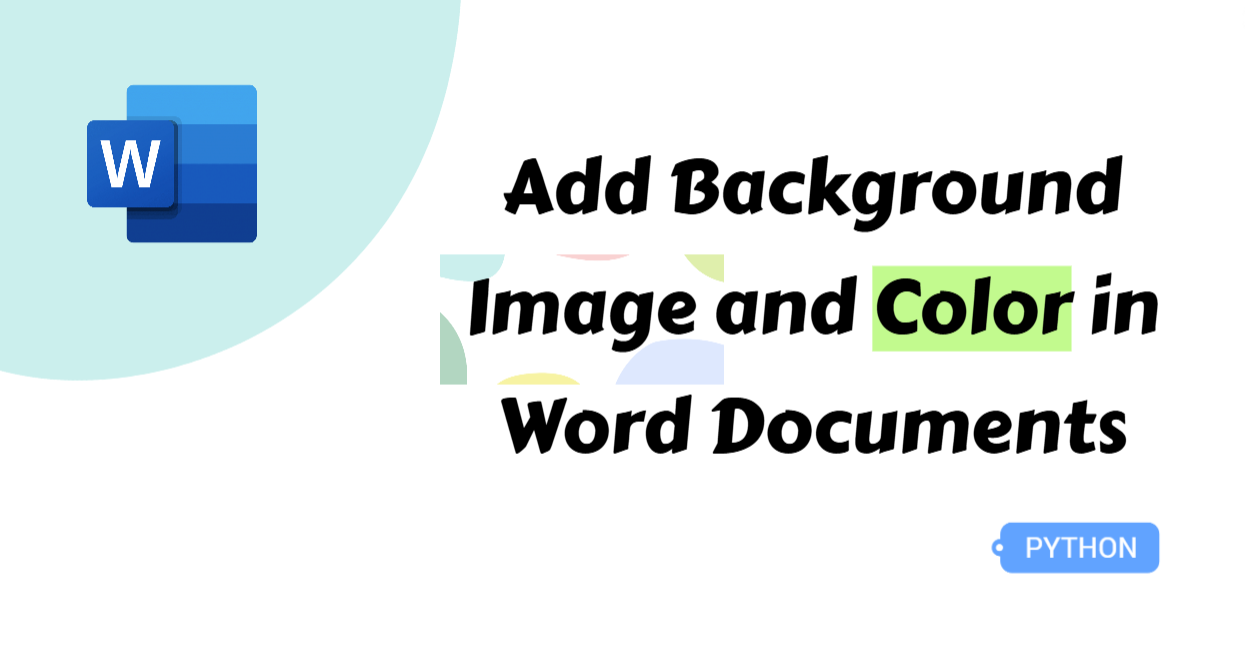
If you want to make your Word documents stand out and reflect a sense of ownership, adding a unique background image or color can be an excellent solution. Not only does it elevate the document's visual appeal, but it also adds a professional touch to reports, presentations, or any formal document. Repeating this process manually across multiple files can be time-consuming, but with Python, you can automate it quickly and efficiently. In this article, we’ll walk you through how to add both background images and colors in Word using Python, helping you create distinctive, polished documents in no time.
Python Library to Add Background Image and Color in Word
When it comes to setting the background of a Word document using Python, choosing the right library is crucial. Common libraries like python-docx do not support direct background image or color insertion. Instead, you would have to simulate the background color using shapes. On the other hand, pydocx can only set the background color when converting HTML to Word, but it cannot operate on existing Word documents.
Given the limitations of these open-source libraries, for more advanced functionality, you might want to consider enterprise-level libraries like Spire.Doc or Aspose.Words. These libraries provide complete support for setting both background images and colors in existing Word documents. Additionally, they offer a wide range of features, such as inserting images, text find-and-replace, and other advanced document operations.
In this article, we will demonstrate how to set the background image and color in Word documents using Spire.Doc, thanks to its intuitive API and rich features. You can easily install it using the pip command: pip install Spire.Doc.
How to Insert Background Images in Word Documents
A background image can also serve as a watermark, such as one featuring a company or organization logo. Even when used purely for visual enhancement, a background image can significantly improve the aesthetics of a Word document. With Spire.Doc, you can easily insert a background image using the Background.Type property and the Background.SetPicture() method, allowing for seamless integration of your custom background image into Word documents.
Steps to insert background image in a Word document:
Create an instance of the Document class.
Read a source Word document from files using the Document.LoadFromFile() method.
Access its background through the Document.Background property.
Set the background type as Picture through the Background.Type property.
Set the background image with the Background.SetPicture() method.
Save the modified Word file as a new document using the Document.SaveToFile() method.
Here is the Python example of adding an image as the background in a Word document:
from spire.doc import *
from spire.doc.common import *
# Create a Document object
document = Document()
# Load a Word document
document.LoadFromFile("/AI-Generated Art.docx")
# Get the document's background
background = document.Background
# Set the background type as Picture
background.Type = BackgroundType.Picture
# Set the background picture
background.SetPicture("/background1.png")
#save the resulting document
document.SaveToFile("/AddBackgroundImage.docx", FileFormat.Docx2016)
document.Close()
How to Add Solid Background Color in Word Files
A solid color background is ideal for maintaining readability, as it ensures the reader’s focus remains undistracted while adding a touch of visual appeal. With the Background.Type property and the Background.Color() method, you can easily customize the background color of your Word document. Follow the steps below to create a unique document!
Steps to add a solid background color in a Word document:
Create an object of the Document class.
Load a sample Word document using the Document.LoadFromFile() method.
Access the background through the Document.Background property.
Set the background type as Color through the Background.Type property.
Choose a color and set it as the background using the Background.Color property.
Save the updated Word document.
Below is the code example for setting AliceBlue as the solid background color in a Word document:
from spire.doc import *
from spire.doc.common import *
# Create a Document object
document = Document()
# Load a Word document
document.LoadFromFile("/AI-Generated Art.docx")
# Get the document's background
background = document.Background
# Set the background type as Color
background.Type = BackgroundType.Color
# Set the background color
background.Color = Color.get_AliceBlue()
#save the resulting document
document.SaveToFile("/AddBackgroundColor.docx", FileFormat.Docx2016)
document.Close()
How to Add Gradient Background Color in Word Documents
Sometimes you may find solid colors boring. You can easily set a gradient background to add more depth and interest. This can be achieved by using the Background.Type property to define the background as a gradient and then select two colors to create the effect. Below, let’s dive into the detailed steps and the code example to help you implement this.
Steps to add a gradient background color in Word documents:
Create a Document object.
Load a sample Word document from files using the Document.LoadFromFile() method.
Access the background of the document through the Document.Background property.
Set the background type as Gradient through the Background.Type property.
Configure the two colors with the Background.Gradient.Color1 and Background.Gradient.Color2 properties.
Customize gradient shading variant and style using Background.Gradient.ShadingVariant and Background.Gradient.ShadingStyle properties.
Save the resulting Word document.
The following code example demonstrates how to set a gradient background color from AliceBlue to LightBlue in a Word document:
from spire.doc import *
from spire.doc.common import *
# Create a Document object
document = Document()
# Load a Word document
document.LoadFromFile("/AI-Generated Art.docx")
# Get the document's background
background = document.Background
# Set the background type as Gradient
background.Type = BackgroundType.Gradient
# Set two gradient colors
background.Gradient.Color1 = Color.get_AliceBlue()
background.Gradient.Color2 = Color.get_LightBlue()
# Set gradient shading variant and style
background.Gradient.ShadingVariant = GradientShadingVariant.ShadingDown
background.Gradient.ShadingStyle = GradientShadingStyle.Horizontal
#Save the resulting document
document.SaveToFile("/AddGradientBackground.docx", FileFormat.Docx2016)
document.Close()
The Conclusion
This explores the process of adding a background image and background color to a Word document using Python, covering both solid and gradient color backgrounds. To make the process easier to grasp, we've included detailed steps and code examples. By the end of this guide, you'll be able to effortlessly create Word documents with customized, eye-catching backgrounds!
Subscribe to my newsletter
Read articles from Casie Liu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
