Building an Image Classifier Using Deep Learning
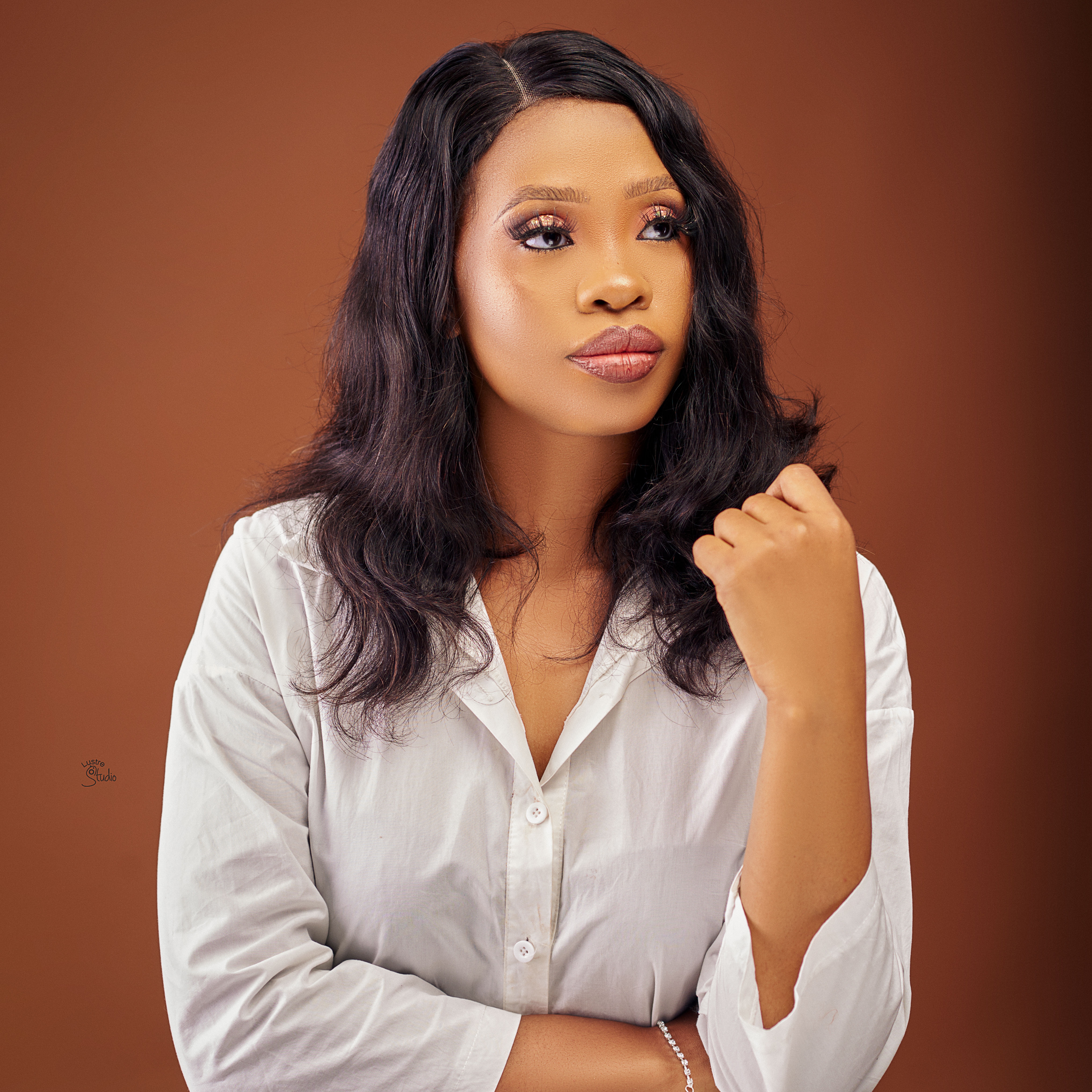
Ever wondered how online stores like Amazon or ASOS instantly recognize and categorize clothing items? Or how your phone can identify objects in photos? The magic behind this is Deep Learning – a powerful branch of AI that enables machines to "see" and understand images just like humans do.
In this project, we’ll build a Convolutional Neural Network (CNN) that can classify images of clothing from the Fashion MNIST dataset. By the end of this tutorial, you'll not only understand how image classification works but also how AI is transforming industries like fashion, retail, and e-commerce.
Let’s dive in and teach a computer how to recognize fashion items!
Step 1: Importing Required Libraries
First, we need to import some Python libraries that will help us build our model.
from tensorflow.keras import layers, models, Sequential
from tensorflow.keras.layers import Conv2D, Flatten, Dense
from tensorflow.keras.utils import to_categorical
import numpy as np
import matplotlib.pyplot as plt
TensorFlow/Keras – Helps us create and train the model.
NumPy – Handles numerical data.
Matplotlib – Displays images.
Step 2: Loading the Fashion MNIST Dataset
We will now load the dataset, which contains images of clothing.
# Load the Fashion MNIST dataset
train_images, train_labels, test_images, test_labels = (np.load('data/fashion_mnist.npz')[key]
for key in ['train_images', 'train_labels', 'test_images', 'test_labels'])
- Each image in the dataset is 28x28 pixels in grayscale (black and white).
Step 3: Viewing Some Sample Images
Before training, let’s look at some sample images.
plt.figure(figsize=(5,5))
for i in range(25):
plt.subplot(5,5,i+1)
plt.xticks([])
plt.yticks([])
plt.grid(False)
plt.imshow(train_images[i]/255.0, cmap='gray')
plt.show()
We divide pixel values by 255.0 to scale them between 0 and 1.
The Matplotlib library is used to display a 5x5 grid of images.
Step 4: Preparing the Data for Training
Before training, we need to preprocess the images so that the model can learn efficiently.
# Normalize images (scale pixel values to 0-1)
train_images = train_images / 255.0
test_images = test_images / 255.0
# Convert labels to one-hot encoding
train_labels_1h = to_categorical(train_labels)
test_labels_1h = to_categorical(test_labels)
# Get the number of classes
num_classes = len(set(train_labels))
# Image size
img_size = train_images[0].shape[0]
Understanding the Concepts:
Normalization: Converts pixel values from 0-255 to a range between 0 and 1, which helps the model learn faster and perform better.
One-hot encoding: Converts labels into a format the model can understand. Instead of a single number representing a class (e.g., 3 for a shirt), we use a vector where only the correct class is marked with a 1 (e.g.,
[0, 0, 1, 0, 0, 0, 0, 0, 0, 0]
).
Step 5: Building the CNN Model
Now, we create the deep learning model.
# Define the model
model = Sequential()
# First layer: Extracts features from the image
model.add(Conv2D(32, kernel_size=3, input_shape=(img_size, img_size, 1), activation='relu'))
# Second layer: Detects more complex features
model.add(Conv2D(16, kernel_size=3, activation='relu'))
# Flatten the image to feed it into the final layer
model.add(Flatten())
# Final layer: Classifies the image into one of 10 categories
model.add(Dense(num_classes, activation='softmax'))
Understanding the Layers:
Convolutional Layers (
Conv2D
) – These layers detect important features, such as edges and textures, in an image. The first layer detects simple patterns, while the second layer detects more complex ones.ReLU Activation (
relu
) – Helps the model learn by keeping only positive values and discarding negative ones.Flatten Layer (
Flatten
) – Converts the multi-dimensional output of the convolutional layers into a single one-dimensional array to be processed by the final classification layer.Dense Layer (
Dense
) – The last layer contains 10 neurons (one for each clothing category). It predicts the probability of the image belonging to each category.
Step 6: Compiling the Model
Before training, we tell the model how to learn.
model.compile(optimizer='adam',
loss='categorical_crossentropy',
metrics=['accuracy'])
Understanding the Compilation Process:
Adam Optimizer – Adjusts the learning process to make training more efficient
Categorical Crossentropy Loss – Measures how well the model’s predictions match the correct labels. (It gives lower loss when predictions are closer to the actual class)
Accuracy Metric – Helps track how well the model is performing.
Step 7: Training the Model
Now, we train the model using our dataset.
# Train the model
model.fit(train_images, train_labels_1h,
validation_split=0.2,
epochs=10,
batch_size=100)
validation_split=0.2
– Uses 20% of the training data to check progress.epochs=10
– Trains the model 10 times on the dataset.batch_size=100
– Processes 100 images at a time.
Step 8: Testing the Model
After training, we test the model to see how well it performs on new images.
# Evaluate the model on test data
scores = model.evaluate(test_images, test_labels_1h, batch_size=100)
test_accuracy = scores[-1]
print(f'Test accuracy: {test_accuracy:.2f}')
- The test accuracy tells us how well the model performs on unseen images.
We have successfully built a deep learning model that can classify fashion images! By refining this model with more data, additional layers, and advanced training techniques, it can become even more accurate and robust. The future of AI in fashion and beyond is exciting!
E-commerce platforms like ASOS, H&M, and Zara can use similar models to automatically tag and categorize clothing items, making product searches more efficient.
Impact Beyond Fashion
This technology isn’t just for fashion—it’s also used in:
Healthcare: Identifying diseases from medical images like X-rays.
Agriculture: Recognizing plant diseases to help farmers take action early.
Security: Detecting objects and people in surveillance footage.
Subscribe to my newsletter
Read articles from Ifeoma Okafor directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
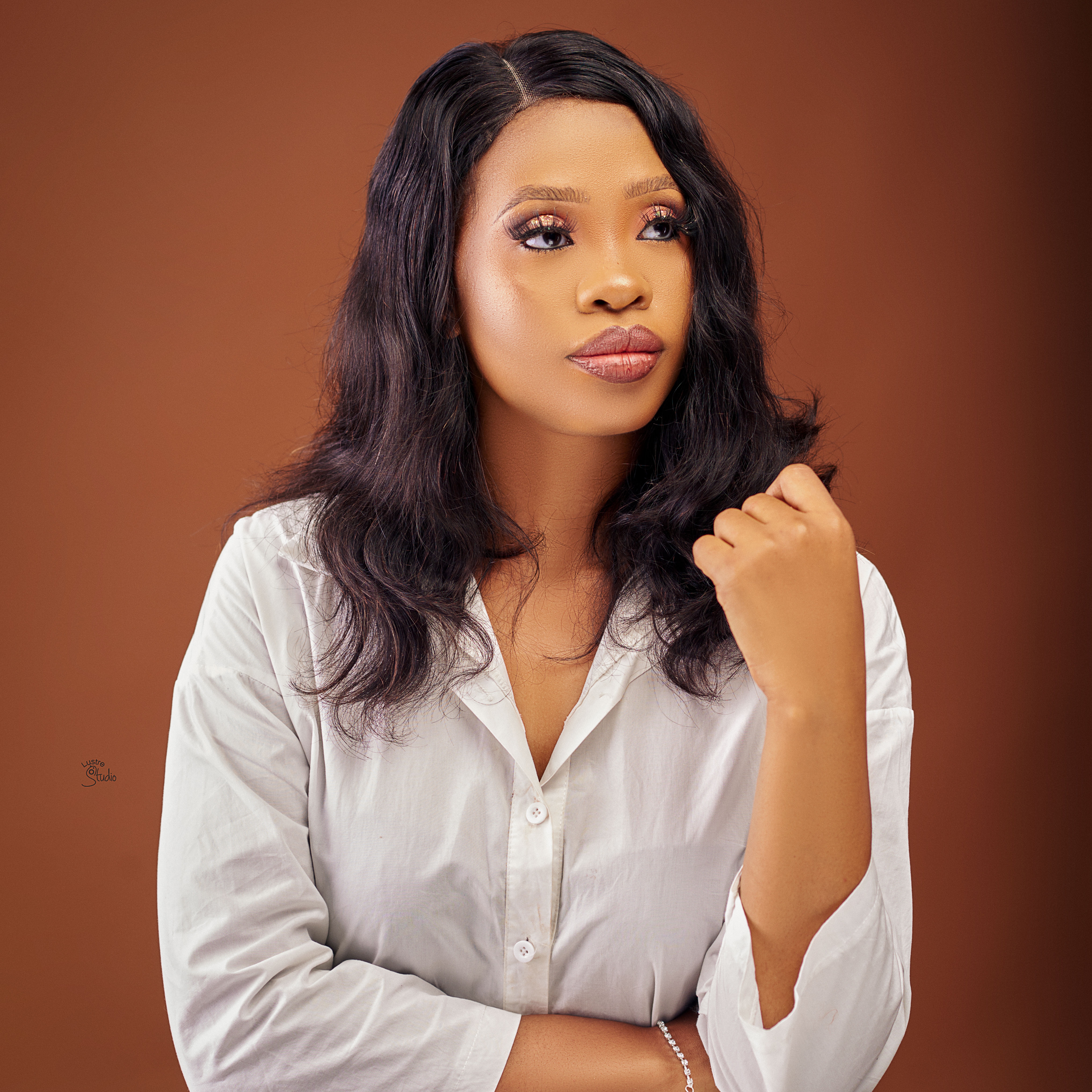
Ifeoma Okafor
Ifeoma Okafor
I am passionate about technology, a pharmacist and a passionate advocate for public health. I firmly believe that leveraging technology is pivotal in advancing healthcare and ensuring its accessibility to all individuals. When I am not immersed in the activities stated above, I find solace in indulging in life's simple pleasures. Reading captivating books, watching thought-provoking movies, and cherishing invaluable moments with loved ones are among the many ways I find joy outside of my professional pursuits. Read, follow and leave a comment ❤️