Recurrent Neural Networks (RNN): A Detailed Guide

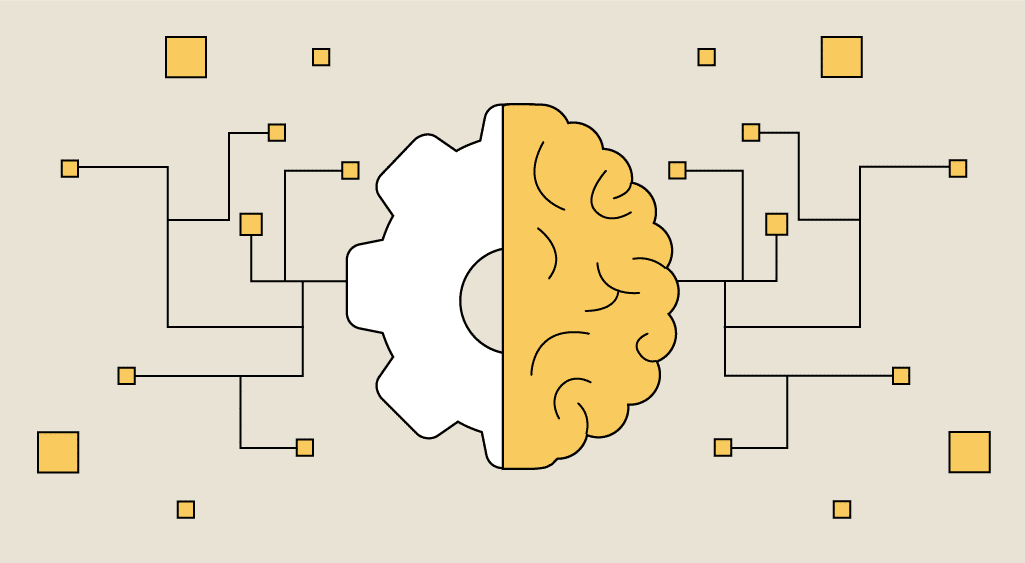
Introduction
Recurrent Neural Networks (RNNs) are a class of neural networks designed to process sequential data. Unlike traditional neural networks, which assume inputs are independent, RNNs have connections that allow them to retain information across sequence steps, making them ideal for tasks where context and order are important, such as natural language processing, speech recognition, and time series forecasting.
This blog will cover:
What is an RNN?
Why use RNNs for sequential data?
How do RNNs work?
Types of RNNs:
Vanilla RNN
Long Short-Term Memory (LSTM)
Gated Recurrent Unit (GRU)
Applications of RNNs
Advantages and Limitations
Implementation Example in Python using TensorFlow/Keras
What is a Recurrent Neural Network (RNN)?
A Recurrent Neural Network (RNN) is a type of neural network specifically designed to handle sequential data. RNNs maintain a hidden state that captures information about previous inputs, enabling them to learn temporal dependencies and context.
Key Characteristics:
Sequential Information Retention: RNNs retain context from previous time steps, allowing them to understand sequences.
Shared Weights: The same weights are applied at every time step, reducing the number of parameters.
Feedback Loops: Hidden states are fed back into the network, influencing future outputs.
Architecture Overview
An RNN processes input sequences one step at a time, maintaining a hidden state that is updated at each step.
Input Sequence → Hidden State → Output Sequence
Why Use RNNs for Sequential Data?
Contextual Learning: RNNs remember previous inputs, making them suitable for tasks where order and context matter (e.g., language translation).
Variable Input Length: RNNs can handle input sequences of varying lengths, unlike traditional neural networks.
Sequential Output: They generate outputs at each time step, making them ideal for tasks like time series prediction.
Temporal Dependency: RNNs capture temporal dependencies, essential for speech recognition and video analysis.
How Do RNNs Work?
RNNs have loops in their architecture, enabling them to store information from previous time steps in a hidden state. At each time step:
The current input and the previous hidden state are combined.
An activation function (e.g., tanh) is applied.
The new hidden state is calculated.
An output is generated.
Mathematical Formulation
Given input sequence X={x1,x2,...,xT}, the RNN computes:
- Hidden State Update:
ht = Hidden state at time t
xt = Input at time t
Wxh = Weight matrix for input to hidden state
Whh = Weight matrix for hidden to hidden state
bh = Bias term
f = Activation function (e.g., tanh)
- Output Calculation:
yt = Output at time t
Why = Weight matrix for hidden to output
g = Activation function (e.g., softmax for classification)
Example Architecture
Input x_t → Hidden State h_t → Output y_t
↑ ↓
Hidden State h_{t-1}
Types of RNNs
1. Vanilla RNN
Vanilla RNNs are the simplest form of RNNs. They have a single hidden layer and use an activation function like tanh or ReLU.
Limitations:
Vanishing Gradient Problem: Gradients become very small, leading to poor learning of long-term dependencies.
Exploding Gradient Problem: Gradients become very large, leading to unstable training.
2. Long Short-Term Memory (LSTM)
LSTM networks are designed to solve the vanishing gradient problem by introducing a more complex memory mechanism.
Key Components:
Cell State (Ct): Stores long-term memory.
Hidden State (ht): Stores short-term memory.
Input Gate: Controls how much new information is added to the cell state.
Forget Gate: Controls how much of the previous memory to keep.
Output Gate: Controls the output and hidden state update.
LSTM Equations:
- Forget Gate:
- Input Gate:
- Cell State Update:
- Output Gate:
Advantages:
Handles long-term dependencies effectively.
Mitigates vanishing gradient issues.
3. Gated Recurrent Unit (GRU)
GRU is a variant of LSTM with a simpler architecture. It combines the input and forget gates into a update gate and merges the hidden and cell states.
Key Components:
Update Gate: Controls how much of the past information to keep.
Reset Gate: Controls how much of the past information to forget.
GRU Equations:
- Update Gate:
- Reset Gate:
- Hidden State Update:
Advantages:
Simpler than LSTM with fewer parameters.
Faster training while maintaining similar performance.
Applications of RNNs
Language Modeling and Text Generation
Speech Recognition
Machine Translation
Time Series Prediction
Video Analysis and Captioning
Implementation Example in Python (TensorFlow/Keras)
import tensorflow as tf
from tensorflow.keras import layers, models
# Sample Sequential Data (e.g., Time Series)
X_train, y_train = ..., ...
X_train = X_train.reshape((X_train.shape[0], X_train.shape[1], 1))
# Define RNN model
model = models.Sequential([
layers.SimpleRNN(50, activation='tanh', input_shape=(X_train.shape[1], 1)),
layers.Dense(1)
])
# Compile Model
model.compile(optimizer='adam', loss='mse')
# Train Model
model.fit(X_train, y_train, epochs=20)
Conclusion
RNNs are powerful models for sequential data but suffer from vanishing gradients. LSTM and GRU variants overcome this limitation and are widely used in NLP and time series tasks.
Would you like to explore advanced architectures like Bidirectional RNNs or Attention Mechanisms?
Subscribe to my newsletter
Read articles from Tushar Pant directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
