Develop Your ASP.NET .NET And Nuxt Web Application using HTTPS
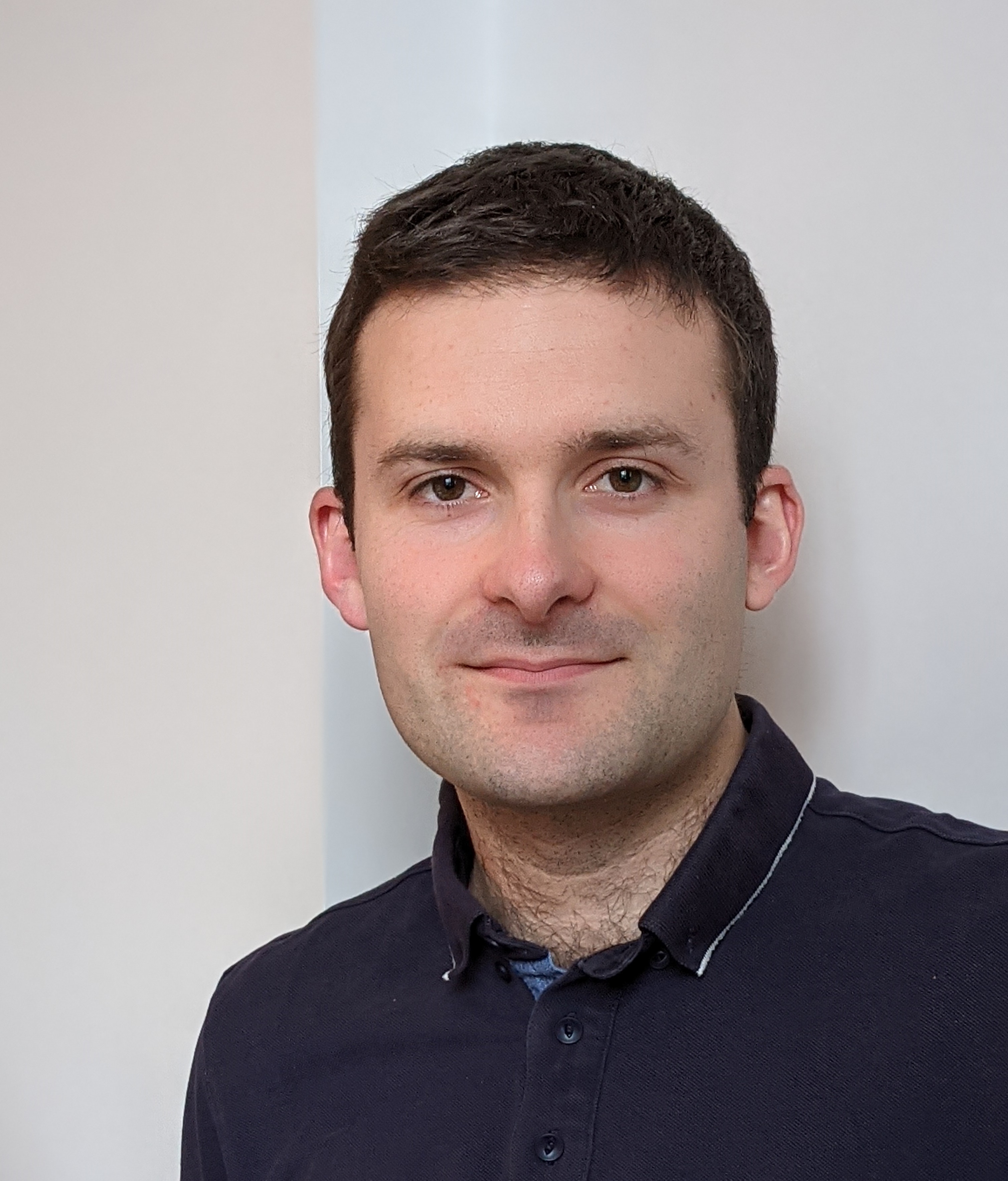
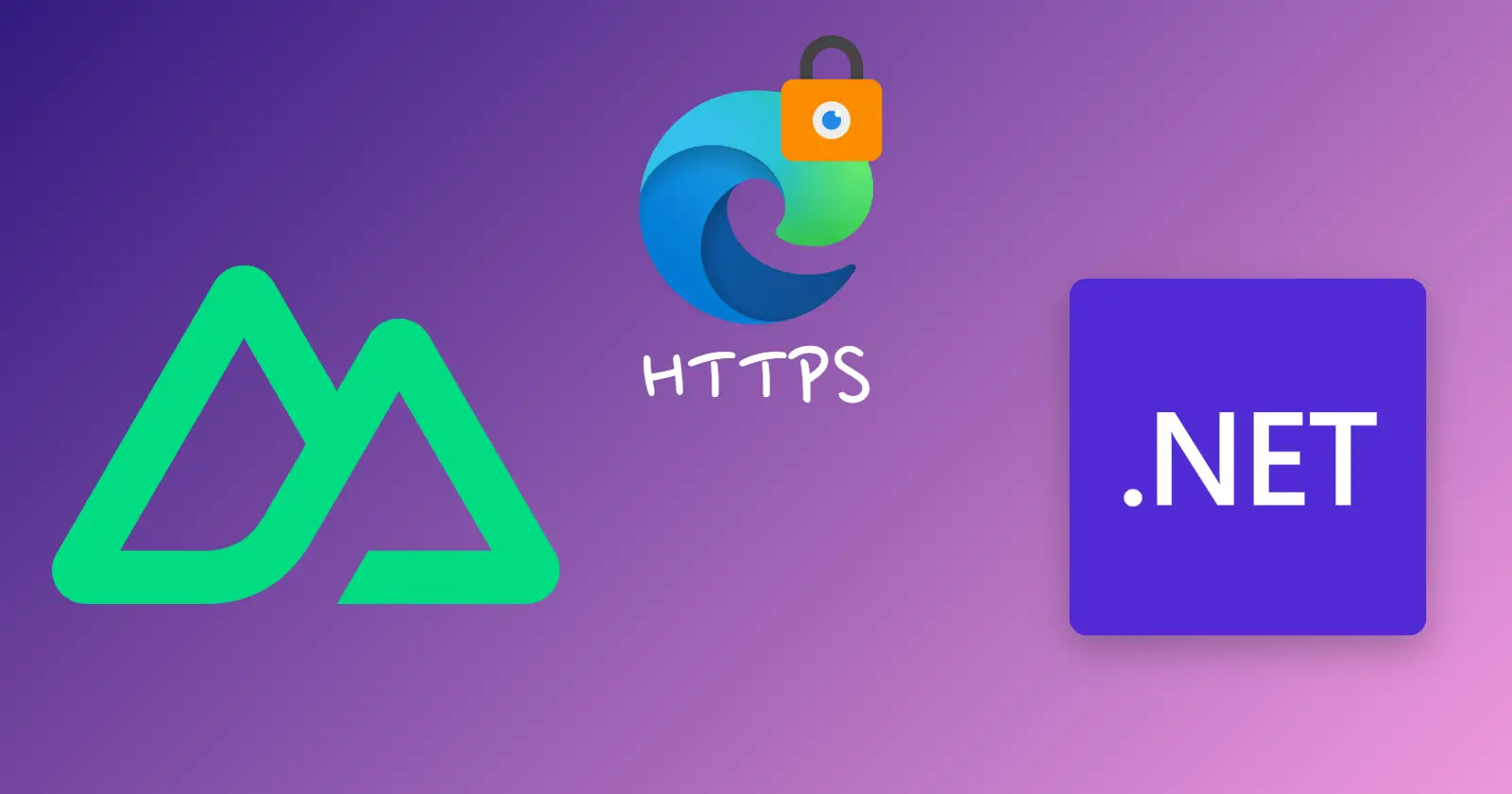
In my last article, we explored how to integrate an ASP.NET Core API with a Nuxt.js front end. However, we were using HTTP to debug both the API and the front end locally.
There are a lot of reasons why we would want to use HTTPS instead of HTTP for local development, but mainly it's about having a local setup that closely matches the production environment. Our web application will of course use HTTPS when deployed to production and other environments, so it's better to identify any potential issues related to HTTPS as early as possible.
So let’s switch to HTTPS for the ASP.NET Core and Nuxt.js application we previously created.
Use HTTPS for the ASP.NET Core API
To make HTTPS work, we need to generate a self-signed certificate and trust it on our local environment. There are different ways to do that, but to keep things simple we can use the built-in command of the .NET CLI dev-certs
that will install an ASP.NET Core HTTPS Development certificate:
dotnet dev-certs https --trust
In the launchsettings.json
file of the WebAPI
project, you can see there are 2 profiles:
{
"$schema": "https://json.schemastore.org/launchsettings.json",
"profiles": {
"http": {
"commandName": "Project",
"dotnetRunMessages": true,
"launchBrowser": false,
"applicationUrl": "http://localhost:5096",
"environmentVariables": {
"ASPNETCORE_ENVIRONMENT": "Development"
}
},
"https": {
"commandName": "Project",
"dotnetRunMessages": true,
"launchBrowser": false,
"applicationUrl": "https://localhost:7238;http://localhost:5096",
"environmentVariables": {
"ASPNETCORE_ENVIRONMENT": "Development"
}
}
}
}
By default, the first profile is used when you run the project without specifying a profile:
dotnet run --project WebApi\WebApi.csproj
To use the https
profile, we can run this command:
dotnet run --project WebApi\WebApi.csproj -lp https
And now the WebApi
runs in HTTPS locally:
Configure the Nuxt front end to work with the HTTPS API endpoint
Because we changed the WebApi
to HTTPS, the endpoint has changed, so we have to change the URL for the API on the WebApp
.
We can do that in the nuxt.config.ts
:
routeRules: {
'/api/**': {
proxy: 'https://localhost:7238/**',
}
},
As indicated in Nuxt documentation, “to call an (external) HTTPS URL with a self-signed certificate in development, you will need to set NODE_TLS_REJECT_UNAUTHORIZED=0
in your environment.” We can set this environment variable directly in the dev
script in package.json
(as it is explained in this article) but the syntax would differ depending on the shell used. So instead we will set it directly in an .env
file:
NODE_TLS_REJECT_UNAUTHORIZED=0
Now, the WebApp
is working again, this time by using the WebApi
HTTPS endpoint.
Yet, we are still using HTTP for the front itself, let’s change that.
Use HTTPS for the Nuxt front end
In the Nuxt configuration, there is an https
section with key
and cert
properties to specify the private key and certificate to use. We already have an ASP.NET Core HTTPS Development certificate, so we can use it for the WebApp
by exporting the necessary files with the dotnet dev-certs
command:
dotnet dev-certs https --export-path ./WebApp/dev-cert.pem --format PEM -np
This will generate a dev-cert.pem
file and a dev-cert.key
file. Make sure to configure properly your .gitignore
to avoid committing these files.
Vue
, React
or Angular
, generating a certificate using dotnet dev-certs
and exporting the corresponding files is exactly what the code of the vite.config.ts
file in the templates does.Then, we can modify the nuxt.config.ts
file:
$development: {
routeRules: {
'/api/**': {
proxy: 'https://localhost:7238/**',
}
},
devServer: {
https: {
key: 'dev-cert.key',
cert: 'dev-cert.pem'
}
}
},
And here is the result, both the front end and the API using HTTPS:
To create a production-like environment during local development, it's a good idea to use HTTPS. Using tools like the .NET CLI to generate and trust self-signed certificates makes it easier to set up HTTPS for both the ASP.NET Core API and the Nuxt.js front end.
Subscribe to my newsletter
Read articles from Alexandre Nedelec directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
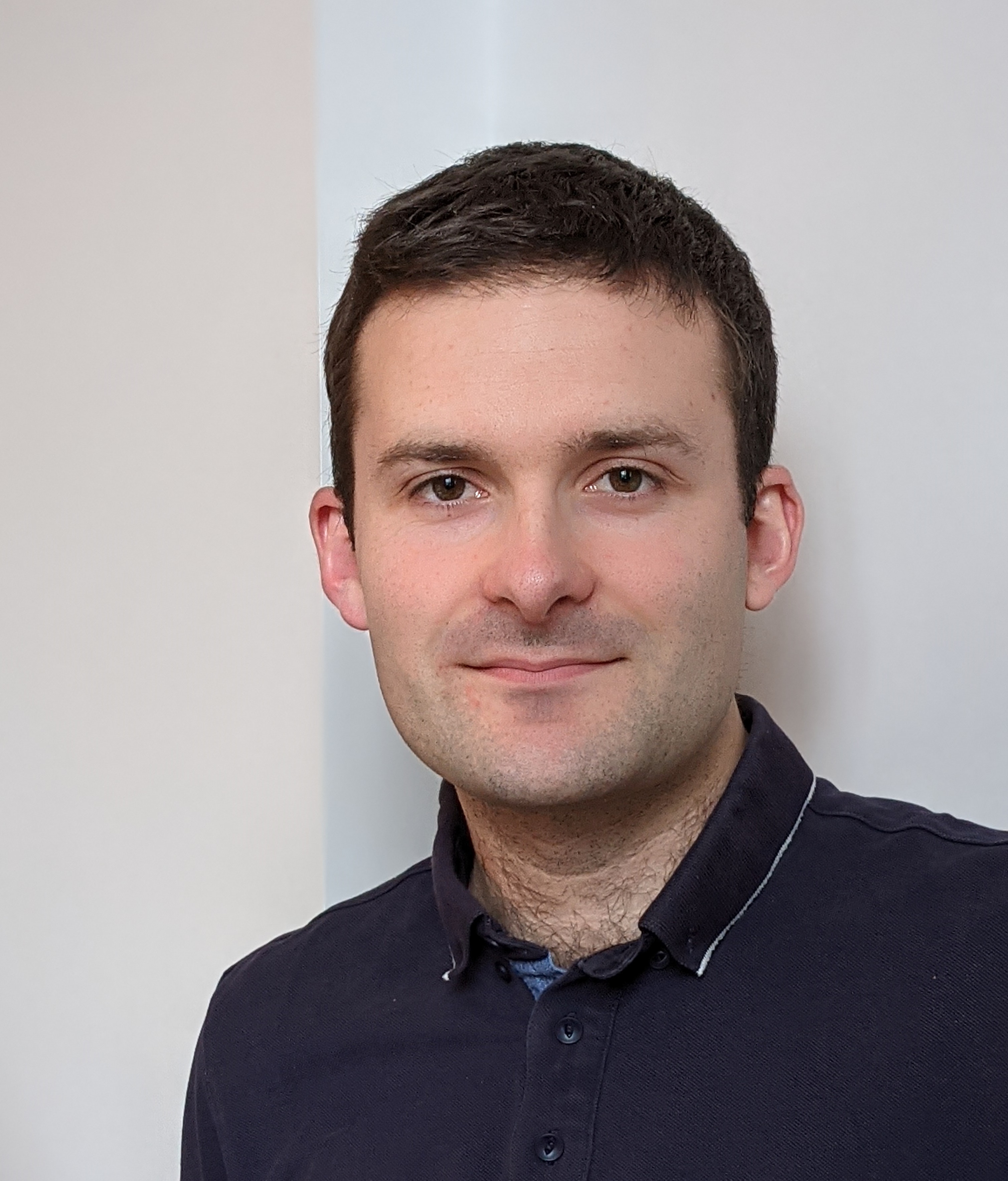
Alexandre Nedelec
Alexandre Nedelec
I am a software developer living in Bordeaux. I am a .NET and Azure enthusiast, primarly coding in C# but I like to discover other languages and technologies too.