Kaggle's Intro to Machine Learning Course
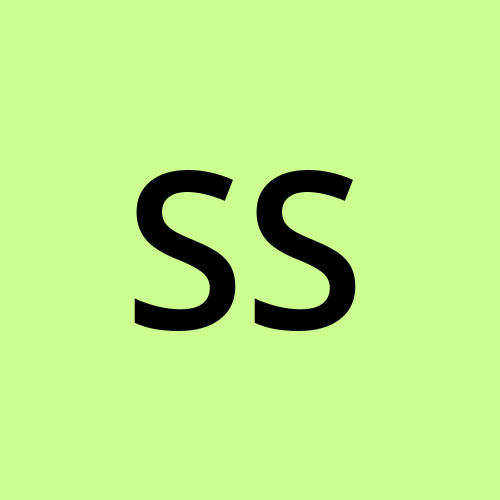
Starting Out on Machine Learning
Machine learning (ML) has become an essential tool in various fields, from healthcare to finance, and learning how to implement ML models has never been more important. Recently, I completed the Intro to Machine Learning course on Kaggle, which has been an eye-opening experience. In this blog, I will take you through the lessons I learned, the hands-on exercises, and the key takeaways from the course.
Lessons
The course is structured in a way that introduces machine learning from the ground up. Here’s a brief overview of the lessons:
1. How Models Work
This was the first lesson, and it covered the basics of machine learning, which is perfect for beginners. The lesson introduced the concept of models and how they learn from data. It gave me a solid foundation to understand how algorithms work and the importance of data in training those models.
2. Basic Data Exploration
Before jumping into creating machine learning models, it's crucial to understand your data. This lesson taught me the importance of loading, cleaning, and exploring the data. It also introduced the various methods to visualize data and identify patterns that would be useful in model training.
# Basic Exploration
print(df.head())
print(df.describe())
print(df.isnull().sum())
3. Your First Machine Learning Model
This lesson was the most exciting one! I built my very first machine learning model. The course provided a practical exercise where I applied the concepts learned in the previous lessons to create a model. I felt like a real data scientist!
from sklearn.tree import DecisionTreeClassifier
from sklearn.model_selection import train_test_split
from sklearn.datasets import load_iris
# Load data and split
data = load_iris()
X_train, X_test, y_train, y_test = train_test_split(data.data, data.target, test_size=0.3)
# Train and evaluate decision tree
model = DecisionTreeClassifier()
model.fit(X_train, y_train)
print(model.score(X_test, y_test))
4. Model Validation
Once you’ve built a model, it’s important to validate its performance. In this lesson, I learned how to measure the accuracy of a model and test its predictions. It was fascinating to see how various metrics such as precision, recall, and accuracy can help determine how well the model is performing.
from sklearn.model_selection import cross_val_score
from sklearn.ensemble import RandomForestClassifier
from sklearn.datasets import load_iris
# Load data
data = load_iris()
X, y = data.data, data.target
# Cross-validation
model = RandomForestClassifier()
cv_scores = cross_val_score(model, X, y, cv=5)
print(cv_scores.mean())
5. Underfitting and Overfitting
A critical lesson in machine learning is understanding underfitting and overfitting. This lesson explained the concepts and the delicate balance needed to achieve optimal performance. I learned how to fine-tune my models to avoid overfitting, where the model is too complex and performs well on training data but poorly on unseen data, and underfitting, where the model is too simple and misses key patterns in the data.
# Underfitting model
underfit_model = DecisionTreeClassifier(max_depth=1)
underfit_model.fit(X_train, y_train)
# Overfitting model
overfit_model = DecisionTreeClassifier(max_depth=10)
overfit_model.fit(X_train, y_train)
# Evaluate models
print(f"Underfit Accuracy: {underfit_model.score(X_test, y_test)}")
print(f"Overfit Accuracy: {overfit_model.score(X_test, y_test)}")
6. Random Forests
This lesson introduced a more sophisticated algorithm: Random Forests. I learned how this ensemble method uses multiple decision trees to make predictions. Random Forests are powerful because they reduce the risk of overfitting and increase the model's accuracy by aggregating predictions from multiple trees.
from sklearn.ensemble import RandomForestClassifier
from sklearn.model_selection import train_test_split
from sklearn.datasets import load_iris
# Load data and split
data = load_iris()
X_train, X_test, y_train, y_test = train_test_split(data.data, data.target, test_size=0.3)
# Random forest model
model = RandomForestClassifier(n_estimators=100)
model.fit(X_train, y_train)
# Evaluate model
print(model.score(X_test, y_test))
7. Machine Learning Competitions
The final lesson encouraged me to apply the knowledge I gained to Kaggle’s real-world machine learning competitions. This was a fantastic opportunity to test my skills against other data enthusiasts and keep improving by learning from the competition results. It was inspiring to see how others approached similar problems.
import pandas as pd
# Load Kaggle competition dataset
train_data = pd.read_csv('train.csv')
# Preprocess (e.g., basic cleaning)
train_data = train_data.fillna(train_data.mean())
# Example model building (e.g., logistic regression)
from sklearn.linear_model import LogisticRegression
X_train = train_data.drop(columns=['target'])
y_train = train_data['target']
model = LogisticRegression()
model.fit(X_train, y_train)
# Make predictions
predictions = model.predict(X_train)
# Save predictions to a submission file
submission = pd.DataFrame({'Id': train_data['Id'], 'Prediction': predictions})
submission.to_csv('submission.csv', index=False)
Exercises and Hands-on Learning
One of the key features of the course is the hands-on exercises, which allowed me to directly apply the concepts to real-world datasets. I worked on various projects that helped me gain practical experience in implementing machine learning algorithms. The exercises were challenging but manageable, providing a perfect balance between learning and application.
Key Takeaways
Data is Key: No matter how powerful an algorithm is, the quality of your data matters more.
Model Performance Metrics: Understanding and choosing the right metrics for validation is crucial in building effective models.
Balancing Underfitting and Overfitting: Finding the right level of complexity for a model is a fundamental skill.
Machine Learning Is an Iterative Process: Building a successful model requires continuous learning, adjustments, and fine-tuning.
Kaggle as a Platform: The platform’s competitions and datasets were extremely valuable in pushing my skills further.
Happy learning!
Subscribe to my newsletter
Read articles from Shivankur Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
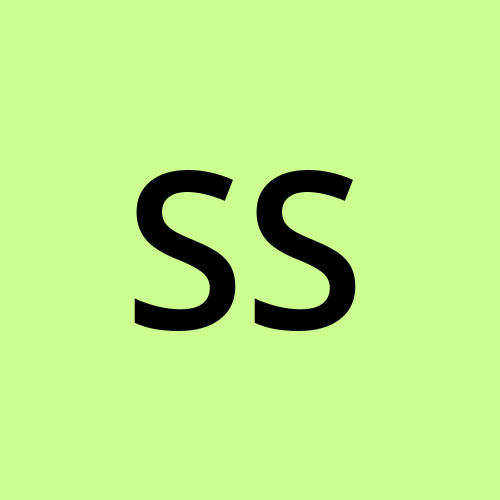