How to effectively use Map, Filter, Reduce, and Lambda in Python

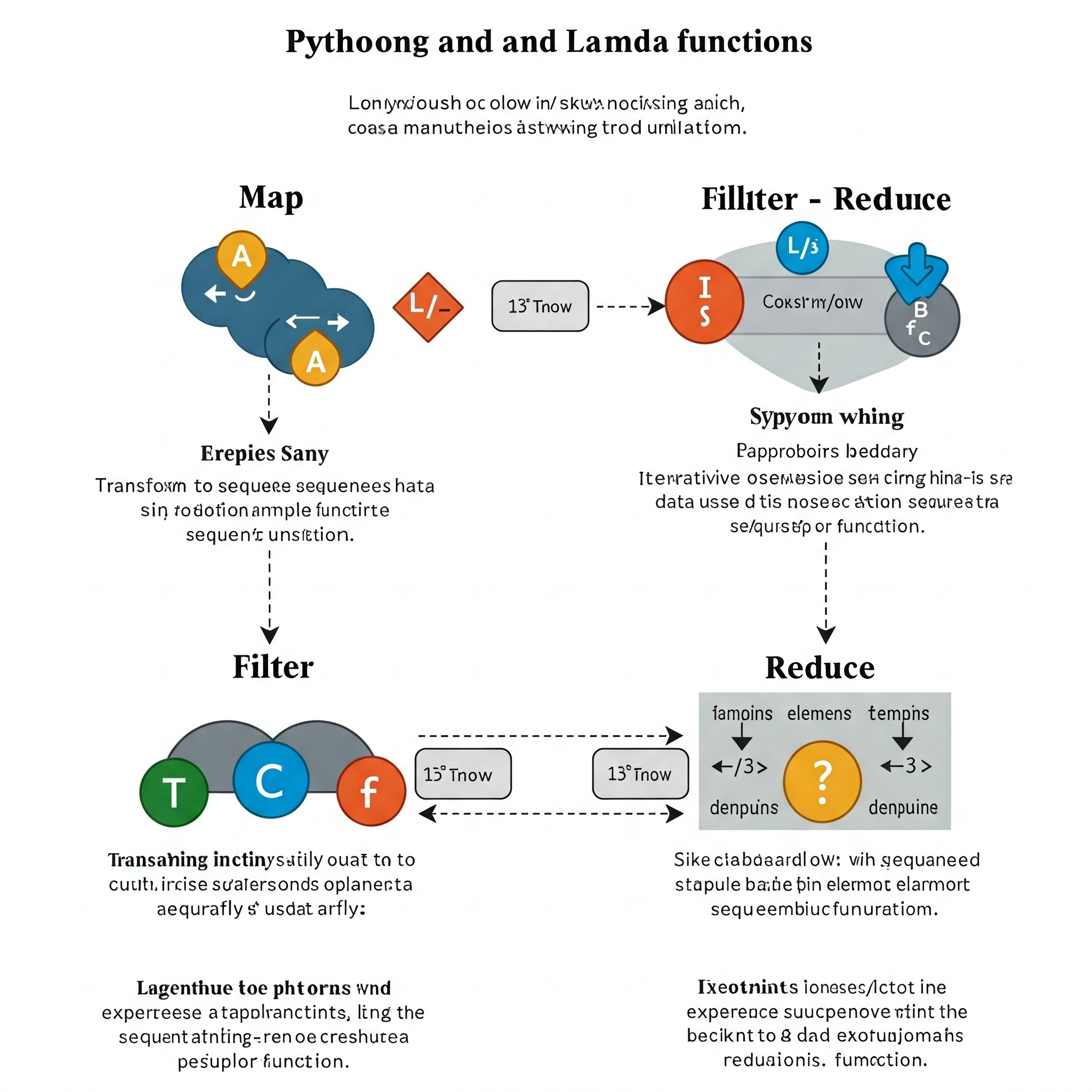
From our last Object-Oriented Programming class we explored some powerful Python functions: map, filter, reduce, and lambda. These functions help in writing clean, efficient, and concise code, making Python even more fun to work with. In this blog, I'll break them down with practical examples to understand how they work.
The Map Function: it Transforms Elements
Concept: map(F,S)
The map() function applies a given function (F) to each item in a sequence (S) and returns an iterator (which we often convert to a list for readability).
numbers = range(3, 11)
cube_function = lambda x: x ** 3
cubes = list(map(cube_function, numbers))
print(cubes)
Normally we would have generated the cubes of numbers from 3 to 10 using a for loop:
cubes = []
for i in range(3, 11):
cubes.append(i ** 3)
print(cubes)
The map()
function simplifies our code and removes the need for an explicit loop.
The Filter Function: Selects Elements Based on a condition
Concept: Filter(F,S)
The filter() function filters elements of a sequence based on a condition defined in function (F).
Example: FIltering Numbers Greater than 110
Let's say we have a list of numbers and we want to filter out only the ones greater than 110:
numbers = [50, 120, 90, 200, 115, 80, 30]
filtered_numbers = list(filter(lambda x: x > 110, numbers))
print(filtered_numbers) # Output: [120, 200, 115]
Here, we use filter()
with a lambda function to extract only numbers greater than 110.
The Reduce Function: Performs Cumulative Operations on a List
Concept: reduce(F,S)
The reduce() function from the functools
module applies a function cumulatively to the elements of a sequence.
from functools import reduce
numbers = range(1, 11)
sum_result = reduce(lambda x, y: x + y, numbers)
print(sum_result) # Output: 55
Here, reduce()
takes two elements at a time, adds them, then continues until the list is fully processed.
Computing the factorial using Reduce
factorial = reduce(lambda x, y: x * y, [1, 2, 3, 4])
print(factorial) # Output: 24
Lambda Function
Syntax:
lambda arguments: expression
Lambda functions are anonymous functions that don’t require the def
keyword.
Example: Perimeter of a rectangle
perimeter_lambda = lambda length, width: 2 * (length + width)
print(perimeter_lambda(5, 10))
Example 2: Multiplying Elements of a List by 10 Using Map
numbers = [1, 2, 3, 4, 5]
result = list(map(lambda x: x * 10, numbers))
print(result) # Output: [10, 20, 30, 40, 50]
Example 3: Filtering Odd Numbers from a List
numbers = [10, 15, 20, 25, 30, 35]
odds = list(filter(lambda x: x % 2 != 0, numbers))
print(odds) # Output: [15, 25, 35]
Example 4: Factorial Using Lambda and Reduce
from functools import reduce
factorial_lambda = reduce(lambda x, y: x * y, range(1, 6))
print(factorial_lambda) # Output: 120
Summary
✅ List -> Map -> Lambda: Used for transforming each element in a list. ✅ List -> Filter -> Lambda: Used for selecting elements based on a condition. ✅ Reduce -> Lambda: Used for cumulative operations like summing or multiplying elements.
Understanding these functions will make your Python code cleaner and more efficient! 🚀
Subscribe to my newsletter
Read articles from Lawani Elyon John directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Lawani Elyon John
Lawani Elyon John
As a student at Babcock University, I've built a foundational understanding of HTML, CSS, and JavaScript.