Mastering the DOM: A Hands-On Guide with Examples
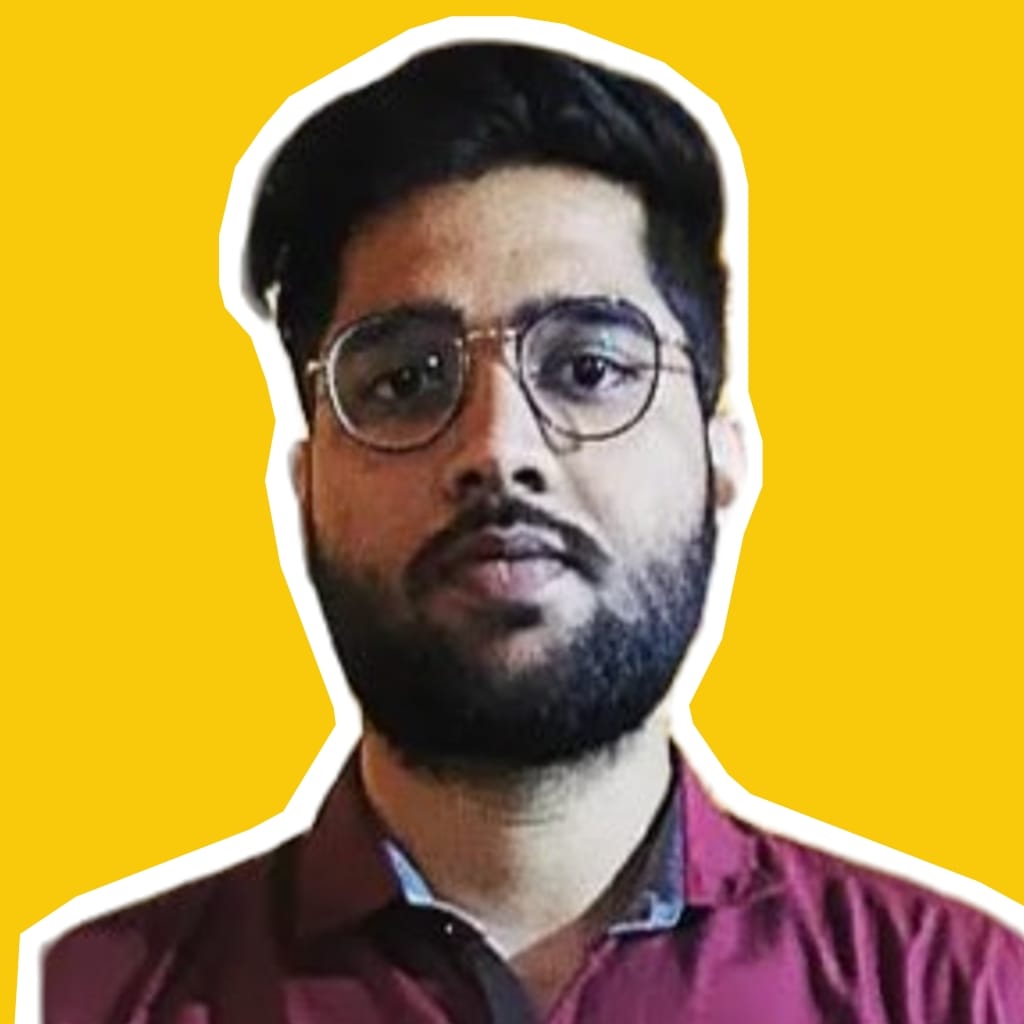

In this article, I will share my learnings from completing DOM challenges. This will help you understand how the DOM works and introduce you to commonly used methods and event listeners for DOM manipulation. Let’s get started!
What is DOM ?
The DOM (Document Object Model) is a representation of the HTML structure of a webpage. However, the main problem of HTML is that it cannot add interactivity or dynamic behavior to the webpage. For example, if there's a button on the webpage, HTML by itself cannot handle what happens when the button is clicked.
This is where the DOM acts as a bridge between the webpage and scripts like JavaScript, allowing us to manipulate the page dynamically. With JavaScript, we can modify the DOM by adding, removing, or updating elements in response to user interactions, making the webpage more interactive and functional.
Now that we understand the DOM, let's explore the methods and events I used while completing the DOM challenges.
addEventListener()
addEventListener is a method in javascript that is used to attach event handler to an element. It listens for a specified event like “click”, ”button” and runs a function when the event occurs
element.addEventListener(event, function, useCapture);
event : the type of event (input,button… etc)
function : the function event that occurs
useCapture (optional) : A boolean value that determines event propagation (default is false)
const button = document.getElementById("myButton");
button.addEventListener("click", () => {
alert("Button clicked!");
});
// when the button with id="myButton" is clicked, an alert pops up
Real Time DOM Manipulation
In one of the challenges, I had to update the DOM in real-time as the user typed into an input field. If no value was entered, the text "Not Provided" should be displayed by default. To achieve this, I explored the input
event.
The input
event fires whenever the value of an <input>
field changes due to user interaction, such as typing in a text box. Unlike the change
event, which only triggers when the input loses focus, the input
event updates the DOM instantly as the user types.
name.addEventListener("input",()=>{
if(name.value.length > 0){
displayName.innerText = name.value
}
else {
displayName.innerText = "Not Provided"
}
})
Every time the user types in the input field, the event listener updates the
displayName
content in real-time.If the input field is empty or contains only spaces,
"Not Provided"
is displayed instead.
Creating and Appending Elements
One of the most powerful features of JavaScript is its ability to dynamically create and insert elements into the DOM. This allows us to build interactive user interfaces
1. Creating Elements with document.createElement
In JavaScript, we use the document.createElement("li")
method to create a new element.
const li = document.createElement("li");
li.classList.add("task-item")
here what i did is i have created a element li where i have add class name task-item using classlist.add property but this is just the element created there is no change in webpage yet so what we have to do is now use appendChild method
2. Appending Elements with appendChild
Once we create an element, we need to attach it to an existing part of the DOM. The appendChild()
method is used to insert the new element as a child of another element.
tasks.appendChild(li)
suppose now when we have to remove the li item from the cart so now we have to manipulate the dom such that our li element gets removed from the tasks element
3. Removing Elements with removeChild
deleteButton.addEventListener("click", () => {
taskList.removeChild(li); // Removes the <li> when the delete button is clicked
});
Using Browser APIs to Manipulate the DOM
Modern web browsers provide built-in APIs that allow us to interact with the DOM dynamically. One such API is the setInterval
function, which helps us execute code repeatedly after a specified time interval.
Image Carousel with Auto-Play
One of the challenges I worked on involved building an image carousel with an autoplay feature that moves to the next image every 5 seconds. This required the use of setInterval
to switch images automatically and clearInterval
to stop autoplay when needed.
Understanding setInterval
for Autoplay
The setInterval(callback, time)
method allows us to repeatedly execute a function after a given time interval (in milliseconds).
function callTimer(time){
clearInterval(interval)
interval = setInterval(()=>{
if(time > 0)
{
timer.innerText= `Next slide in ${time}s`
time--;
}
else
{
clearInterval(interval)
timer.innerText= ``
if(i<images.length-1){
nextBtn.click()
}
else {
previousBtn.click()
}
callTimer(5)
}
},1000)
}
Clearing the Interval (clearInterval
)
To stop autoplay, we use clearInterval(intervalId)
. This was needed when manually navigating images or pausing the carousel.
Working with Arrays to Manage State in the Shopping Cart
One of the most important aspects of this shopping cart implementation was effectively managing the cart’s state using arrays. Instead of directly modifying the DOM for every action, I stored all cart items in an array called allCartItems
, which acted as the single source of truth for the cart's data.
This approach allowed me to manipulate the cart dynamically and update the UI efficiently. Here’s how I managed different cart operations using array methods:
Finding Items in the Cart (find()
)
Before adding an item to the cart, I needed to check if it was already present. To do this, I used the find()
method, which searches the array for an item that matches a given condition.
let existingItem = allCartItems.find(cartItem => cartItem.name === item);
if(existingItem){
existingItem.quantity++;
return updateCart();
}
Why?
This prevents duplicate entries.
If an item already exists, I just increase its quantity instead of adding it again.
💡 This ensures that the cart reflects real-world behavior, where the same product is grouped instead of being added multiple times separately.
Adding New Items (push()
)
If the item was not found in the cart, I created a new object representing the item and pushed it into the array:
let newItem = {
name: item,
price: price,
quantity: 1
};
allCartItems.push(newItem);
updateCart();
Why?
This makes it easy to store multiple items in a structured way.
Each item is represented as an object containing
name
,price
, andquantity
.
💡 Using objects in arrays made it easy to manage item details dynamically.
Updating Item Quantity (map()
)
When a user increases or decreases the quantity of an item, instead of searching through the DOM, I updated the state in the array:
incrementQuantity.addEventListener("click", () => {
cartItem.quantity++;
updateCart();
});
Removing Items (splice()
)
When the user removes an item, I used the splice()
method to delete it from the array based on its index:
allCartItems.splice(index, 1);
updateCart();
Why?
The
splice()
method removes the item at the specified index, preventing unnecessary looping.The cart updates instantly, reflecting the correct items.
💡 This made removing items more efficient instead of looping through the entire array and filtering it manually.
What I Learned From Memory Card Game
This was a challenging tasks but i got to learned about different concepts of js i had refere some website and yt tutorial for learning how to built the memory card game
Visit this link for your reference: https://www.geeksforgeeks.org/build-a-memory-card-game-using-html-css-and-javascript/
Shuffling Cards Using sort()
To make the game challenging, I randomized the card order using sort()
with a random comparator:
cardValuesCopy.sort(() => Math.random() - 0.5); // little hack but fisher yates algo is used most often
- This provides an equal probability of swapping positions, ensuring the cards are shuffled each time the game starts.
Game Logic: Matching Cards
To check if the flipped cards match, I compared their inner text values:
jsCopyEditfunction checkForMatch(){
if(firstCard.children[1].innerText !== secondCard.children[1].innerText){
setTimeout(() => {
firstCard.classList.remove("flipped");
secondCard.classList.remove("flipped");
resetBoard();
}, 500);
} else {
winnerMoves++;
if (winnerMoves === 8) {
setTimeout(() => {
alert(`Congratulations🔥 You won the game with ${moves.innerText} moves and ${time.innerText} taken`);
restartGame();
}, 300);
}
resetBoard();
}
}
Conclusion
These DOM challenges have truly helped me level up my JavaScript skills. I explored various methods, properties, event listeners, and DOM manipulation techniques, which deepened my understanding of how the browser dynamically updates the UI. From handling user interactions to working with timers, arrays, and state management, each challenge has enhanced my problem-solving abilities.
You can check out the GitHub repository and live demo of these projects here:
🔗 Live Link: https://srvjha.github.io/DOM-Challenges
🔗 Github Repo Link: https://github.com/srvjha/DOM-Challenges
If you learned something new, do give it a like and share it with your peers! 🚀
Subscribe to my newsletter
Read articles from Saurav Jha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
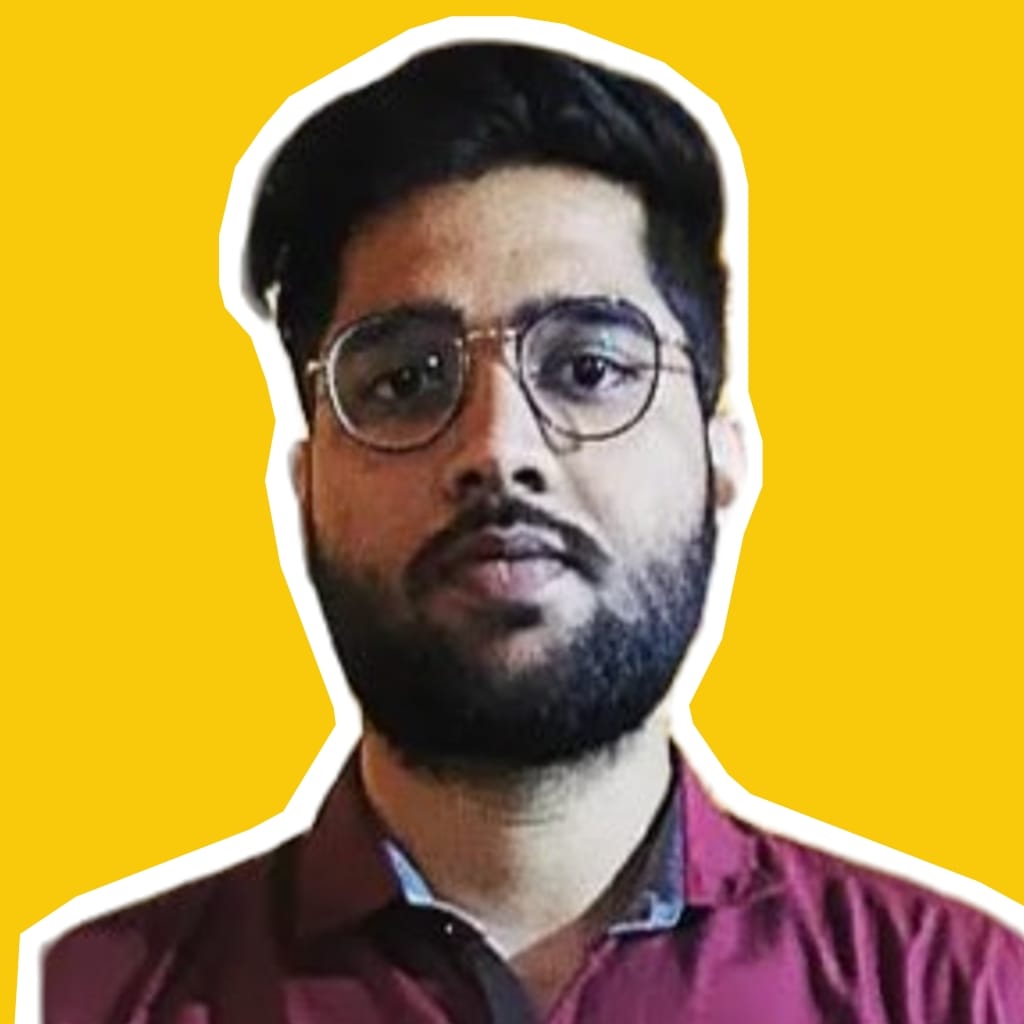
Saurav Jha
Saurav Jha
Software Developer | Full Stack | Testing | API Documentation