Modern JavaScript (ES6+) Features You Should Know

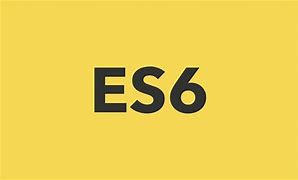
JavaScript has evolved significantly over the years, with ES6 (ECMAScript 2015) introducing major improvements that make the language more powerful and readable. Since then, newer versions have continued to add enhancements, making JavaScript more efficient and easier to use. In this blog, we'll explore the most important modern JavaScript features that every developer should know.
Let and Const
With the old JavaScript, we had only one way to declare a variable, and that was with var
, like var x = 10
. It will create a variable called x and assign a value 10 to it. Now with modern ES6 JavaScript, we have 3 different ways to declare a variable: let
, const
and var
.
var: Declares variables with function or global scope and allows re-declaration and updates within the same scope.
var name="abc" function func(){ { var age=25 } console.log(age) } var name ="xyz" console.log(name) // xyz func() // age
As we can clearly see var doesn’t get bothered by block scope and is accessible in function
func()
also it allows re-declaration ofname
variable twice.let: Declares variables with block scope, allowing updates but not re-declaration within the same block.
let name="abc" function func(){ /*{ let age=25 }*/ let age =25 console.log(age) } //let name ="xyz" name = "zyz" console.log(name) func()
const: Declares block-scoped variables that cannot be reassigned after their initial assignment.
const PI=3.14 PI=22/7 // not allowed
Arrow Functions (short hand expression)
An arrow function expression is a compact alternative to a traditional function expression, with some semantic differences and deliberate limitations in usage:
Arrow functions don't have their own bindings to
this
,arguments
, orsuper
, and should not be used as methods.Arrow functions cannot be used as constructors. Calling them with
new
throws aTypeError
. They also don't have access to thenew.target
keyword.
They are better suited for callback use.
const sum=(a,b)=>{
console.log(a+b)
}
sum(3,5) // 8
Note: Whenever we use curly brackets in arrow functions we need to mention return
for the function and if we don’t use it, then the function returns automatically.
const greet1=(name)=> `Hello ${name}`
console.log(greet1("world"))
const greet2=(name)=> {
return `Hello ${name}`
}
console.log(greet2("world"))
Here both methods returns same output but in one return is mentioned and in another it is not. Remember the syntax.
Template literals (String Interpolation)
Template literals are a feature in JavaScript that let developers work with strings in a convenient way. You denote regular strings in JavaScript using double quotes ""
or single quotes ''
.But with template literals, you denote strings using backticks ` `
. This lets you embed variables and expressions within your strings.
const name = 'abc'
const greet= `Hello ${name}`
console.log(greet) // Hello abc
Another way template literals make it easier to work with strings is when dealing with multi-line strings.
const str =`This is
multi-line string
in JS
`
console.log(str)
/*
output
This is
multi-line string
in JS
*/
Destructuring
Destructuring assignment is a special syntax that allows us to “unpack” arrays or objects into a bunch of variables, as sometimes that’s more convenient.
Array Destructuring
const arr=["John","Smith",35,"Professor"] let [firstname,surname]=arr console.log(`Name: ${firstname} ${surname}`) // skipped 2nd element let [name, ,age]=arr console.log('age: ',age) // Output Name: John Smith age: 35
- Object Destructuring
// Regular Destructuring
const person = {
name: "John Doe",
age: 30,
city: "New York",
occupation: "Software Engineer",
hobbies: ["Reading", "Coding", "Hiking"]
};
const { name, age, city } = person;
console.log(name); // John Doe
console.log(age); // 30
console.log(city); // New York
// destructuring with another name
let {name:personName,age:personAge}=person
console.log(personName) //John Doe
console.log(personAge) // 30
Default Parameters in Functions
Default parameters allow you to specify a default value for a parameter in a function. If an argument is not passed in during a function call, the default value will be used instead. The syntax for declaring default parameters in JavaScript is straightforward. Simply add an equal sign (=
) followed by the default value to the parameter in the function declaration.
function greet(name="John")
{
console.log(`Hello ${name}`)
}
greet('Smith') // Hello Smith
greet() // Hello John
As clearly visible that if we pass an argument to the function it will print the message with that name and if we do not pass anything then it will take “John” as the default value.
Rest and Spread Operator
Both are denoted by . . .
but have different use cases. The rest operator condenses elements into a single entity within function parameters or array destructuring. It collects remaining elements into a designated variable, facilitating flexible function definitions and array manipulation.
function add(...args)
{
let sum=0
for(let arg of args)
{
sum+=arg
}
return sum
}
console.log(add(1,2,3,4,5)) // 15
const arr=[1,2,3,4,5]
let [first,...rest]=arr
console.log(first) // 1
console.log(rest)// [ 2, 3, 4, 5 ]
The spread operator is primarily used for expanding iterables like arrays into individual elements. This operator allows us to efficiently merge, copy, or pass array elements to functions without explicitly iterating through them.
const arr=[1,2,3,4]
const newArr=[...arr,4,5]
console.log(newArr) // [ 1, 2, 3, 4, 4, 5 ]
Promises and Async/Await
Promises are used to deal with asynchronous operations in JS. Visit this article to learn more about asynchronous JS: https://hashnode.com/post/cm7nizvkp000309l8a24l1apm
function fetchData() {
return new Promise((resolve, reject) => {
setTimeout(() => resolve('Data Fetched!'), 2000);
});
}
const data = fetchData()
data.then((data)=> console.log(`Yay! ${data}`)) //Yay! Data Fetched!
For better readability we use async/await
function fetchData() {
return new Promise((resolve, reject) => {
setTimeout(() => resolve('Data Fetched!'), 2000);
});
}
async function getData()
{
const data = await fetchData()
console.log(`Yay! ${data}`)
}
getData()
For…Of loop
A for...of
loop operates on the values sourced from an iterable one by one in sequential order. Each operation of the loop on a value is called an iteration, and the loop is said to iterate over the iterable. Each iteration executes statements that may refer to the current sequence value.
let arr = [1,2,3,4,5]
for(let ele of arr)
{
console.log(ele)
}
// Output
1
2
3
4
5
Optional Chaining
The optional chaining ?.
is a safe way to access nested object properties, even if an intermediate property doesn’t exist. Optional chaining prevents errors when accessing deeply nested properties. The optional chaining ?.
stops the evaluation if the value before ?.
is undefined
or null
and returns undefined
.
const person={
name:"John",
city:"San Francisco",
}
console.log(person.address.city) //Cannot read properties of undefined (reading 'city')
console.log(person.address?.city) // undefined
Nullish Coalescing
The nullish coalescing operator is written as two question marks ??
.
As it treats null
and undefined
similarly, we’ll use a special term here, in this article. For brevity, we’ll say that a value is “defined” when it’s neither null
nor undefined
.
The result of a ?? b
is:
if
a
is defined, thena
,if
a
isn’t defined, thenb
.
In other words, ??
returns the first argument if it’s not null/undefined
. Otherwise, the second one.
let a = 12
let b = 13
console.log(a??b) // 12
a=undefined
console.log(a??b) // 13
Just like it works for undefined
, it also works for null
Conclusion
Modern JavaScript (ES6+) provides powerful features that make coding cleaner, more efficient, and less error-prone. Mastering these concepts will help you write better JavaScript and take full advantage of the language's capabilities.
Subscribe to my newsletter
Read articles from Koustav Chatterjee directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Koustav Chatterjee
Koustav Chatterjee
I am a developer from India with a passion for exploring tech, and have a keen interest on reading books.