Control Flow in JavaScript: if-else and switch

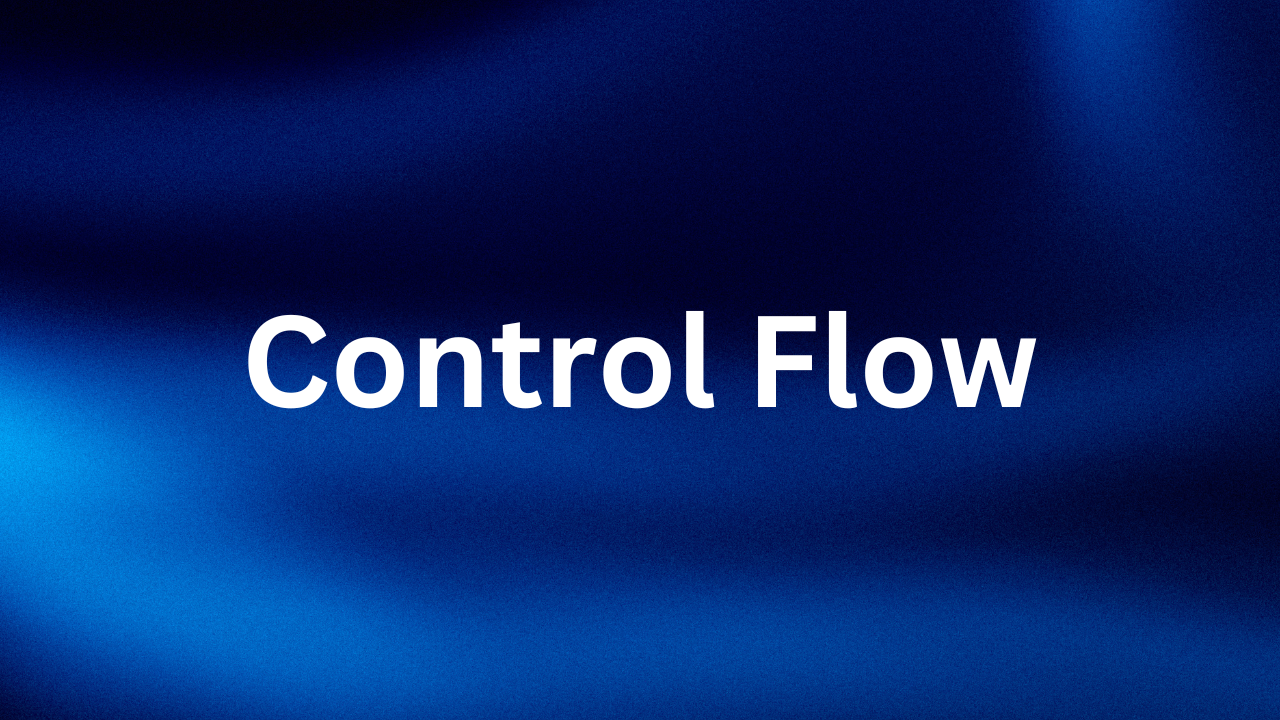
Control flow in JavaScript determines how code executes based on different conditions. The most common ways to control the flow of a program are if-else
statements and switch
statements.
1. if-else Statement
The if-else
statement allows you to execute code based on a condition. If the condition is true
, the block inside if
runs. If it’s false
, the else
block runs (if provided).
Syntax:
if (condition) {
// Code runs if condition is true
} else {
// Code runs if condition is false
}
Example:
let age = 18;
if (age >= 18) {
console.log("You are eligible to vote.");
} else {
console.log("You are not eligible to vote.");
}
Output:
You are eligible to vote.
if-else-if Ladder
If you have multiple conditions, you can use else if
.
let marks = 75;
if (marks >= 90) {
console.log("Grade: A");
} else if (marks >= 75) {
console.log("Grade: B");
} else {
console.log("Grade: C");
}
Output:
Grade: B
2. switch Statement
The switch
statement is used when there are multiple possible conditions for a single variable. Instead of using multiple if-else
, a switch
provides a cleaner approach.
Syntax:
switch (expression) {
case value1:
// Code executes if expression === value1
break;
case value2:
// Code executes if expression === value2
break;
default:
// Code executes if no case matches
}
Example:
let day = 3;
switch (day) {
case 1:
console.log("Monday");
break;
case 2:
console.log("Tuesday");
break;
case 3:
console.log("Wednesday");
break;
default:
console.log("Invalid day");
}
Output:
Wednesday
Why Use switch Instead of if-else?
Better readability when dealing with multiple conditions.
More efficient in some cases as it directly jumps to the matching case.
Conclusion
Use
if-else
when checking conditions that involve comparisons (>
,<
,>=
, etc.).Use
switch
when comparing a single variable against multiple possible values.
Understanding these control flow statements will help you write efficient and clean JavaScript code!
Subscribe to my newsletter
Read articles from Chaitanya Sonawane directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
