Flutter Testing : The 100% test coverage myth
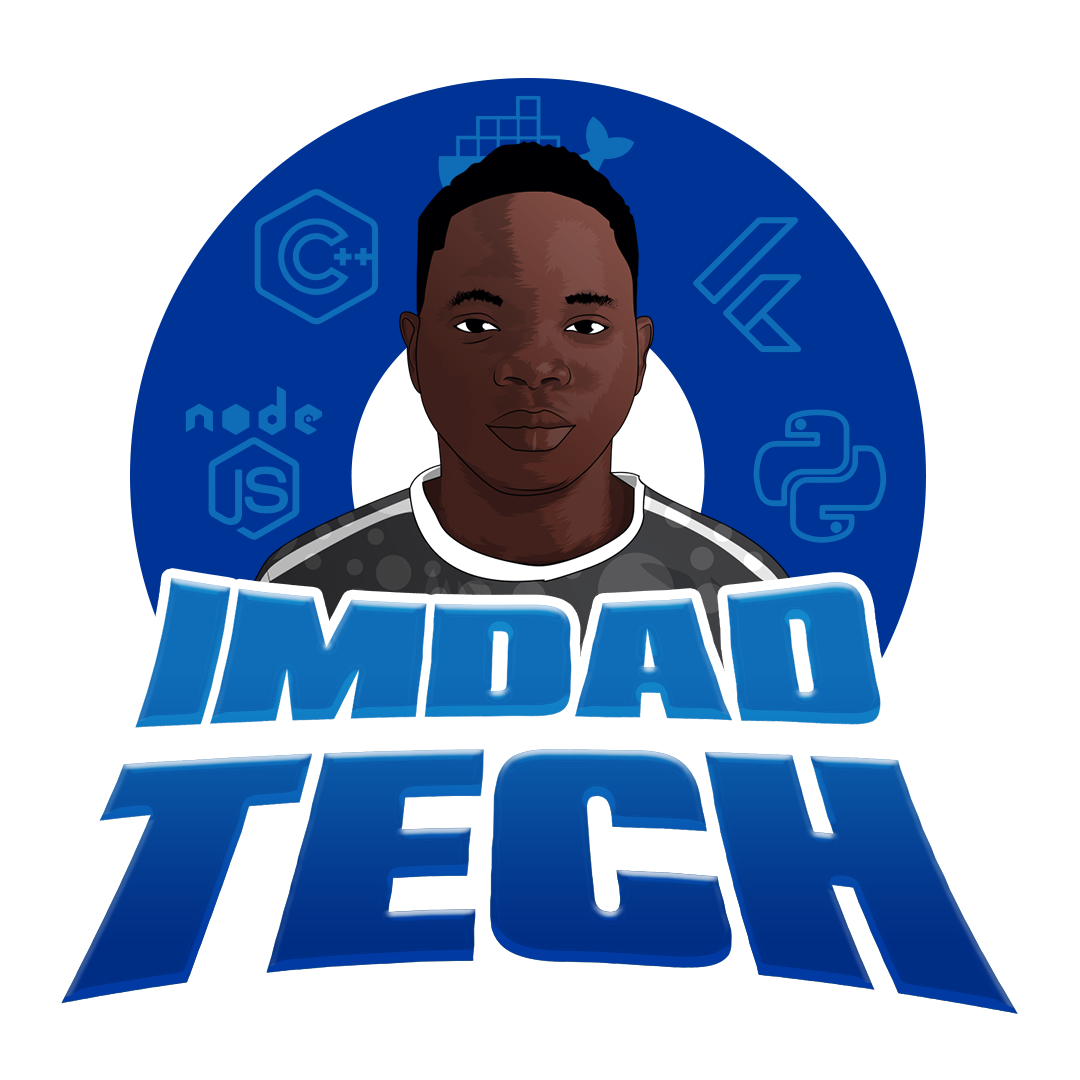
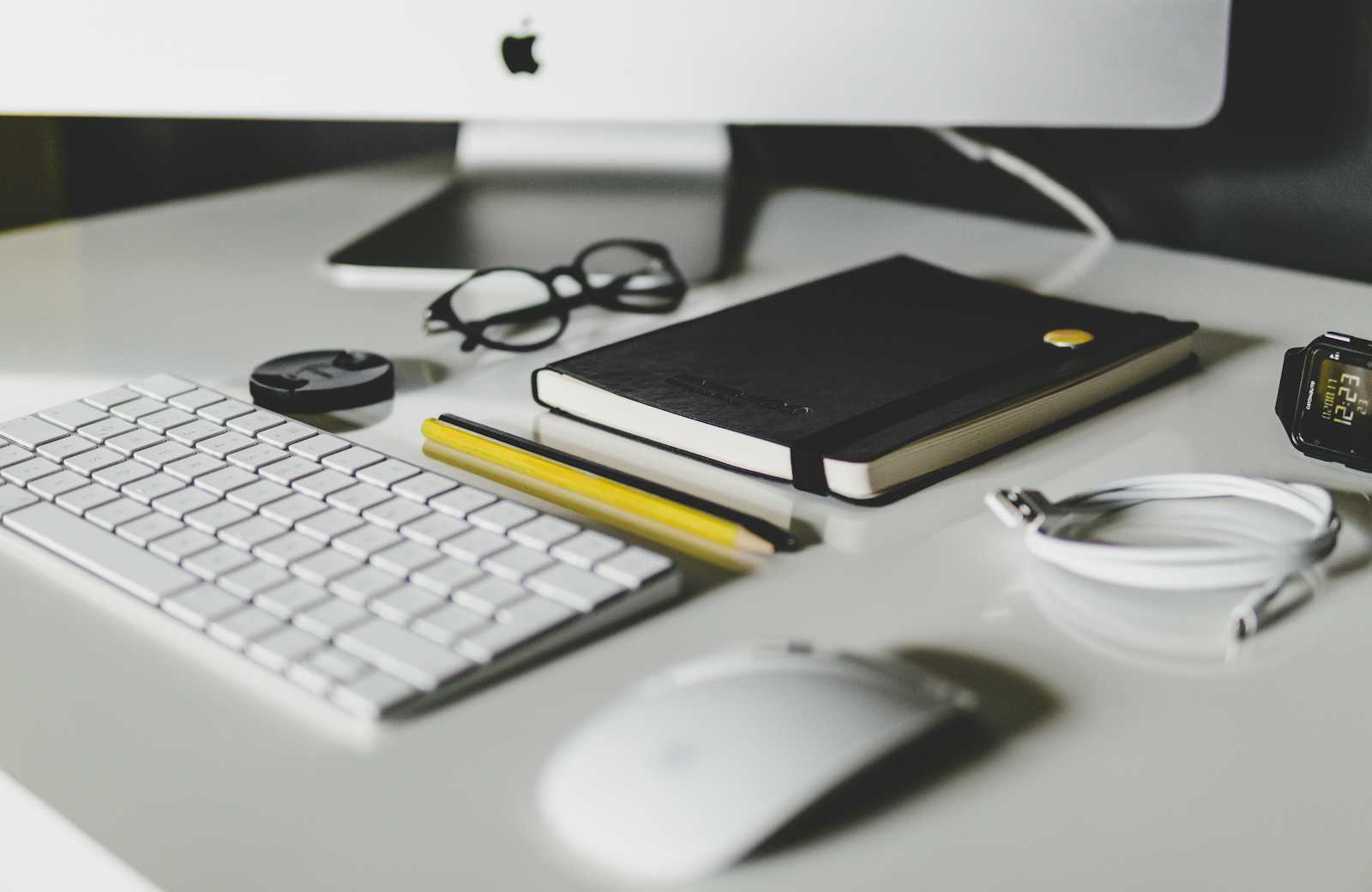
Introduction
I recently worked on a mobile application during a hackathon. At the beginning of this hackathon, I aim to cover my codebase with 100% test code coverage. With this goal in mind, I prioritized writing tests for each feature I implemented. By doing this way, I realized that I spent few time when manually testing the app and I was able to find and fix quickly a bug. I built a solid foundation for my code base and I was able to modify an existing code with confidence because I can rely on the unit tests to notify me when I mistakenly broke something. There are many advantages to writing tests and I wasn’t able to reach my goal of 100% test coverage. Why? I will answer the why in this article after exposing the architecture of my codebase and How I test my codebase so you can learn at the same time how to use available tools to test your flutter codebase.
During the hackathon, I built a mobile app that mainly generates a list of recipes for a user based on his preferences. I built the whole mobile application with the Flutter framework. To keep the code clean, I followed the DDD (Domain Driven Development) concept and a clean architecture to separate everything and to avoid having the UI and business logic together. The DDD leads us to understand how the business works, which problems it resolves also how it earns money so that we can focus on what matters, on features that bring value to the business. And logically, test the important thing, the customer experience. The code is divided into several layers. The presentation, application, domain, and infrastructure layer.
Presentation : The presentation layer contains the UI (User Interface). But it also contains the controller of the view. A controller is a bridge between the UI and the other layer. It contains the functions that are executed when the user interacts with the UI.
Application : The application layer contains the services and use cases used inside a controller. A service is a collection of functions related to a feature. We can for example have the authentification service to manage the login, and register of a user and the notification service to handle notifications. A use case is generally a class with one public function to accomplish a specific task. The use case has access to all the needed services or repositories to accomplish this task.
Domain : The domain layer contains the class model and the interfaces of the repositories. A model is used to represent an object and an interface is an abstract class that contains the declaration of a function without its body. So, a service for example depends on the interface of a repository and not directly on the implementation of this repository.
Infrastructure : The infrastructure layer contains the functions to interact with third parties. For example, an API, a database, etc …
Let’s illustrate the previous explanation with an example. The following image shows how the register feature was implemented in my project :
The RegisterView represents the UI. It listens to a state (RegisterControllerState) emitted by the controller (RegisterController). The view can use the received state to display anything on the user screen or just do some action. In my case, I use the RegisterControllerState to display a snack bar with an error message when the registration fails or to redirect the user to the next screen when the registration is successful. The RegisterController has one public function and depends on the RegisterUsecase. All the logic to register a user is coded inside the RegisterUsecase. The controller's role is to call the public function of the use case and emit a state. The controller has to be as light as possible. The RegisterUsecase depends on the IUserPersonnalInfoRepository, IAuthUserService, and IAuthService.
Now, we can move on to the unit test section. I use some packages to write and run the unit tests.
flutter_test: installed by default by the framework.
bloc_test: provide necessary methods to test bloc/cubit controller.
Mocktail: used to mock functions and have control of the return value of a function.
The first step to test the bloc controller is to create an instance of the controller. For that, I used the buildController method.
RegisterController buildController() {
return RegisterController(
registerUsecase,
);
}
Then, we have to mock the use case used by the controller by using the mocktail package. In our example, I mock the RegisterUsecase. To create a mock of the use case, we need to create a new class that inherits from the Mock class and implements the real RegisterUsecase implementation. You'll find an example below ⬇ :
class RegisterUsecaseMock extends Mock implements RegisterUsecase {}
And then, we have to create an instance of the previous mock class as follow ⬇ :
late RegisterUsecase registerUsecase;
const String email = 'test@gmail.com';
const String password = 'password';
const String name = 'test';
const String errorMessage = 'Register failed';
RegisterController buildController() {
return RegisterController(
registerUsecase,
);
}
setUp(() {
registerUsecase = RegisterUsecaseMock();
});
The setUp
method is executed before each test is run. Now, we can test the controller by using the blocTest
method as follows:
blocTest<RegisterController, RegisterControllerState?>(
'should emit RegisterControllerSuccess when register is successful',
build: () => buildController(),
setUp: () {
when(
() => registerUsecase.register(
email: email,
password: password,
name: name,
),
).thenAnswer(
(_) async => true,
);
},
act: (bloc) => bloc.register(
email: email,
password: password,
name: name,
),
expect: () => <RegisterControllerState?>[
RegisterControllerSuccess(),
],
);
Between the <>
, we first have the name of the controller to test and his cubit type. Each test should have a short description. The build
property takes as value a function that returns an instance of the controller to be tested. Inside the setUp
, we generally call the mock methods and do some initialization. The setUp
of the blocTest has any effect outside of this bloc and it called before the test is executed. The act
is useful when you want to call a public function of a controller during the test execution. In my case, I called the register method. After calling the register, I expected that the controller emits a certain state. We use the expect
to verify if the current state of the bloc matches with the expected state. If yes, the test has passed else it fails.
Apart from the controllers, I also test the services and the use cases by following the same logic. To test for example this use case:
test(
'should register a user',
() async {
final registerUsecase = RegisterUsecase(
authService,
authUserService,
userPersonnalInfoRepository,
);
const userPersonnalInfo = UserPersonnalInfo(
uid: EntityId('uid'),
email: email,
name: name,
);
when(
() => authService.register(
email: email,
password: password,
),
).thenAnswer((_) => Future.value(true));
when(
() => authUserService.currentUser,
).thenAnswer(
(_) => const AuthUser(
email: email,
uid: EntityId(
'uid',
),
),
);
when(
() => userPersonnalInfoRepository.save(userPersonnalInfo),
).thenAnswer(
(_) => Future.value(),
);
final result = await registerUsecase.register(
email: email,
password: password,
name: name,
);
verify(
() => userPersonnalInfoRepository.save(
userPersonnalInfo,
),
).called(1);
expect(result, true);
},
);
}
I first created an instance of the use case to test. Then I mock all external services used by the use case. Then, I specified the returned value of each function used inside the use case to pass my test. I called the use case method. And I verify, if the user's info is correctly saved on the database by using the verify().called()
. I also verify if the register passes by comparing the result of the use case with true
.
The only layer that wasn’t tested on my codebase was the infrastructure layer. The infrastructure layer isn’t intended to contain complex logic and most of the time it interacts with third-party packages. So, there is no plus value to test this part if you keep this layer as simple as possible. I was able to reach 91 / 100 % of test coverage due to this reason.
In conclusion, writing useful tests can reduce the risks of bugs and also help the developer to be productive. It is important to test the necessary things and just not be obsessed with reaching a certain number of code coverage. We learn about the DDD and clean architecture concept and also about the way to test bloc controller and code use case.
Thanks for reading 👨🏽💻.
Subscribe to my newsletter
Read articles from imdad.tech directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
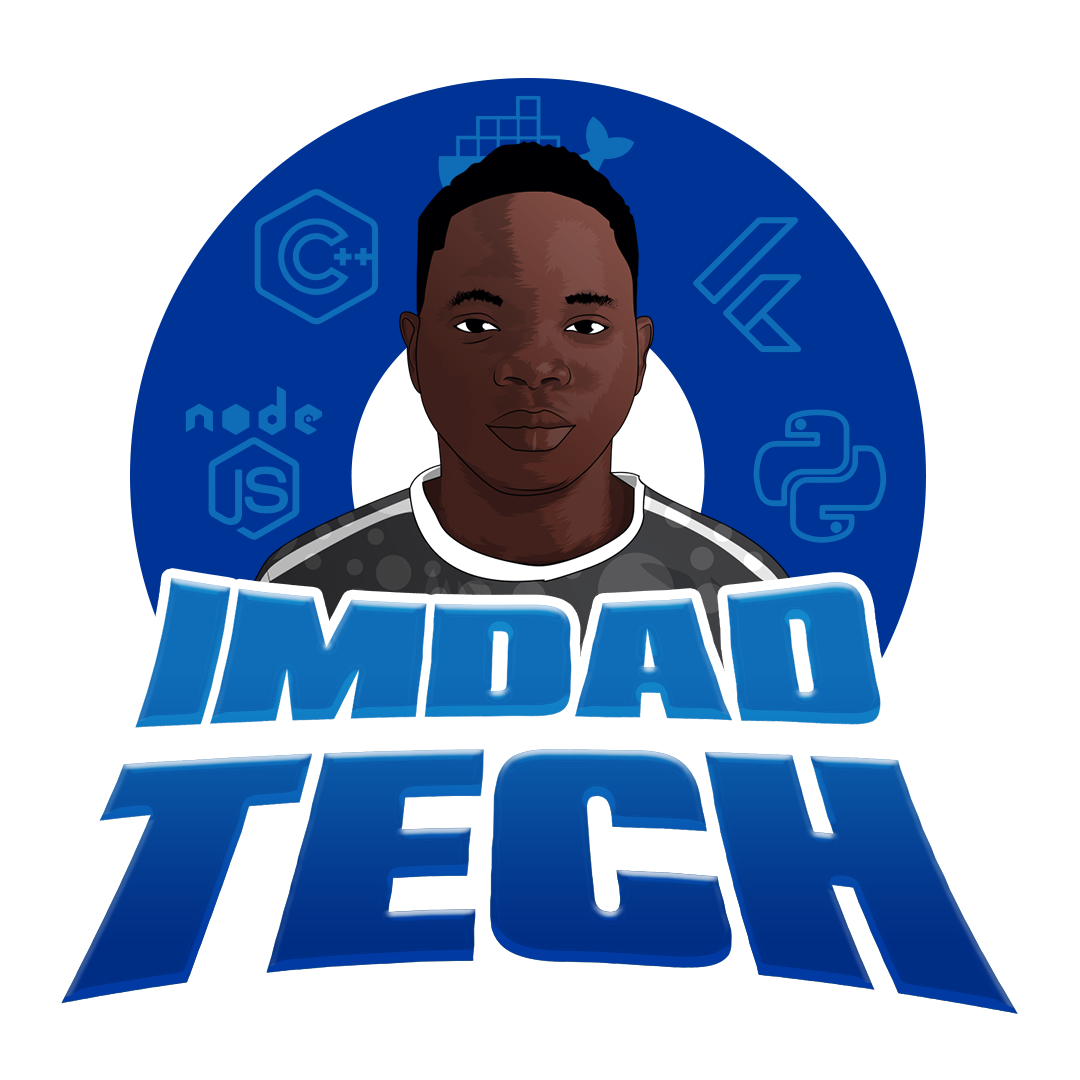
imdad.tech
imdad.tech
Hi, my name is Imdad, FullStack developer who likes to use technology to create cool, useful and fun stuffs. Daily I use MEVN Stack to build Web App and Flutter to build mobile App. Sometimes I use Python 🐍 to automate and create game agents. Currently I am interested in blockhain and web3.