Backend Developer Roadmap 2025: The Complete Guide π
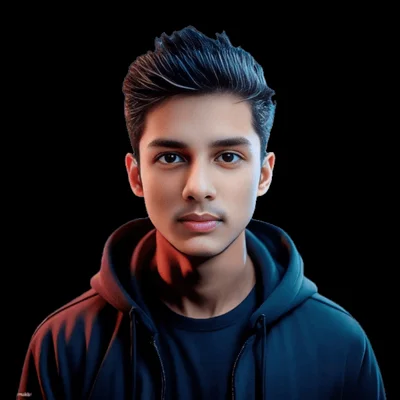
Table of contents
- 1. Understanding How the Web Works π (Timeline: 1-2 Weeks)
- 2. Learning a Programming Language π₯οΈ (Timeline: 3-4 Weeks)
- 3. Mastering Your Language's Ecosystem π (Timeline: 2-3 Weeks)
- 4. Version Control and Repo Hosting π§βπ» (Timeline: 1 Week)
- 5. Databases: Relational & Non-Relational πΎ (Timeline: 2-3 Weeks)
- 6. Working with APIs π (Timeline: 2-3 Weeks)
- 7. API Protocols: WebSockets, gRPC, SSE π (Timeline: 2 Weeks)
- 8. Authentication and Authorization π (Timeline: 2 Weeks)
- 9. Bonus Section: Advanced Concepts π
- 10. Application Deployment π (Timeline: 3-4 Weeks)
- 11. Performance Optimization & Scaling β‘ (Timeline: Ongoing)
- Conclusion π―

The world of backend development is constantly evolving, and keeping up with the latest trends, technologies, and tools is essential for any developer aiming to build scalable, reliable, and efficient web applications. If you're looking to break into backend development or level up your skills, this guide is tailored for you. Whether youβre just starting or already have some experience, weβll walk you through each key aspect of backend development in 2025. π»π§
1. Understanding How the Web Works π (Timeline: 1-2 Weeks)
Before diving into the core aspects of backend development, itβs important to understand how the web works at a basic level. This will help you as you begin working with APIs, servers, and databases.
Key Concepts:
Client-Server Model: The client sends a request to the server, which processes the request and returns a response.
HTTP/HTTPS Protocol: The protocol that governs how data is transferred over the web.
DNS: The system that translates human-readable domain names into IP addresses.
Web Browsers and Web Servers: How browsers request resources and how servers respond with data (HTML, CSS, JS).
Suggested Resources:
Code Example:
<!-- Basic HTTP request example in HTML -->
<form action="https://example.com" method="GET">
<input type="text" name="query">
<button type="submit">Search</button>
</form>
2. Learning a Programming Language π₯οΈ (Timeline: 3-4 Weeks)
Backend development relies on robust programming languages. Your choice of language will dictate your approach to building backend systems. Some popular options in 2025 include:
Node.js: Great for building fast, event-driven apps using JavaScript.
Python: Popular for data-heavy applications and web frameworks like Django and Flask.
Java: Well-suited for enterprise applications with great community support.
C#: Ideal for Windows-based applications and ASP.NET Core.
Rust: Emerging as a low-level, high-performance language for backend services.
Recommended Resources:
Code Example (Node.js):
const http = require('http');
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader('Content-Type', 'text/plain');
res.end('Hello, World!\n');
});
server.listen(3000, '127.0.0.1', () => {
console.log('Server running at http://127.0.0.1:3000/');
});
3. Mastering Your Language's Ecosystem π (Timeline: 2-3 Weeks)
Once you've picked your backend language, it's time to learn its ecosystem. This includes libraries, frameworks, and tools that are essential for building modern web applications.
For example:
Node.js: Learn Express.js, a lightweight web framework.
Python: Master Django or Flask for web app development.
Java: Learn Spring Boot for scalable applications.
C#: Explore ASP.NET Core for backend APIs.
Suggested Resources:
4. Version Control and Repo Hosting π§βπ» (Timeline: 1 Week)
Version control is an essential skill for every developer. Git is the most widely used version control system, and platforms like GitHub, GitLab, and Bitbucket are used for repository hosting.
Key Concepts:
Branches: Create branches for different features or bug fixes.
Commits: Save changes with descriptive commit messages.
Pull Requests: Review and merge code changes.
Code Example:
# Clone a repository
git clone https://github.com/username/repository.git
# Create a new branch
git checkout -b feature-new-feature
# Stage changes
git add .
# Commit changes
git commit -m "Added new feature"
# Push changes
git push origin feature-new-feature
5. Databases: Relational & Non-Relational πΎ (Timeline: 2-3 Weeks)
Understanding databases is crucial in backend development. You'll work with both relational (SQL) and non-relational (NoSQL) databases.
Key Concepts:
Relational Databases: Use structured tables (e.g., MySQL, PostgreSQL).
Non-Relational Databases: Store data in flexible formats (e.g., MongoDB, Redis).
Database Comparison Table:
Feature | Relational Databases | Non-Relational Databases |
Structure | Tables with rows & columns | Key-value pairs, documents, graphs |
Example | MySQL, PostgreSQL | MongoDB, Redis |
Query Language | SQL | NoSQL Query Language |
Suggested Resources:
6. Working with APIs π (Timeline: 2-3 Weeks)
A major part of backend development is working with APIs. You'll deal with REST APIs, GraphQL, and API documentation.
Key Concepts:
REST API: Stateless APIs that follow the CRUD operations (Create, Read, Update, Delete).
GraphQL: A flexible query language for APIs, allowing clients to request exactly what they need.
Example (Node.js + Express - REST API):
const express = require('express');
const app = express();
// Sample API route
app.get('/api/users', (req, res) => {
res.json([{ name: "John Doe", age: 30 }]);
});
app.listen(3000, () => {
console.log("API server running at http://localhost:3000");
});
7. API Protocols: WebSockets, gRPC, SSE π (Timeline: 2 Weeks)
In addition to HTTP-based APIs, there are several API protocols youβll encounter:
WebSockets: Full-duplex communication for real-time apps.
gRPC: High-performance communication for microservices.
Server-Sent Events (SSE): One-way real-time communication from server to client.
Suggested Resources:
8. Authentication and Authorization π (Timeline: 2 Weeks)
Security is vital in backend development, particularly when it comes to authentication (verifying identity) and authorization (defining what users can do).
Key Concepts:
JWT (JSON Web Tokens): A widely used method for handling authentication.
OAuth: An authorization framework for third-party apps.
9. Bonus Section: Advanced Concepts π
Once you've mastered the basics, dive into more advanced backend concepts:
CORS (Cross-Origin Resource Sharing): Controls resource sharing across different domains.
SSL/TLS: Secures communications over the web.
XSS (Cross-Site Scripting): Protect against malicious script injections.
CSRF (Cross-Site Request Forgery): Prevent unauthorized requests from attackers.
10. Application Deployment π (Timeline: 3-4 Weeks)
When your application is ready, deploying it is the final step. Learn about Docker, web services, and cloud platforms like AWS, Azure, and Google Cloud.
Key Concepts:
Docker: Containerize your applications to make them portable and scalable.
Cloud Platforms: AWS, GCP, and Azure provide scalable services for deployment.
Suggested Resources:
11. Performance Optimization & Scaling β‘ (Timeline: Ongoing)
To handle a large number of users and data, you'll need to optimize your applicationβs performance and scale it efficiently.
Key Techniques:
Caching: Use Redis or Memcached to store frequently accessed data.
Load Balancing: Distribute traffic across multiple servers.
Database Indexing: Speed up query execution.
Conclusion π―
Becoming a skilled backend developer requires a combination of mastering essential concepts, choosing the right technologies, and continuously improving your knowledge. The Backend Developer Roadmap 2025 is your guide to staying ahead in a constantly evolving field. π‘
Additional Resources:
By following this roadmap, youβll gain the technical skills needed to build scalable, secure, and efficient backend systems. Stay committed, keep learning, and youβll be ready for any challenges that come your way! π
Subscribe to my newsletter
Read articles from Lakshay Dhoundiyal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
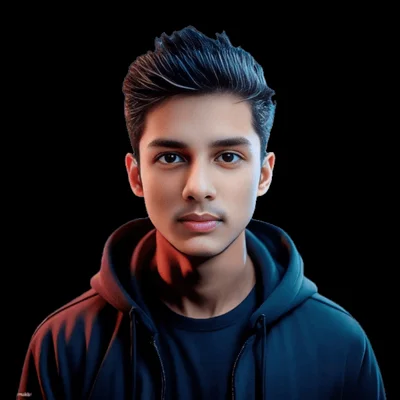
Lakshay Dhoundiyal
Lakshay Dhoundiyal
Being an Electronics graduate and an India Book of Records holder, I bring a unique blend of expertise to the tech realm. My passion lies in full-stack development and ethical hacking, where I continuously strive to innovate and secure digital landscapes. At Hashnode, I aim to share my insights, experiences, and discoveries through tech blogs.