JavaScript Functions Aur Function Expression Kya Hain? Chaliye Jaante Hain

Table of contents
- Function Kya Hai?
- Function Parameters aur Data Types
- Alag-Alag Data Types ke Saath Function Call
- Default Parameters
- Chalo Function pass karte hain
- Function Expression Kya Hota Hai?
- Anonymous (Benaam) Function
- Hoisting aur Function Overriding
- Callback Function
- Arrow Functions
- Functions as Objects
- Extra Concept: Immediately Invoked Function Expression (IIFE)
- Function Rest Parameter
- Arguments Passing: By Value vs. By Reference
- this Keyword
- Summary
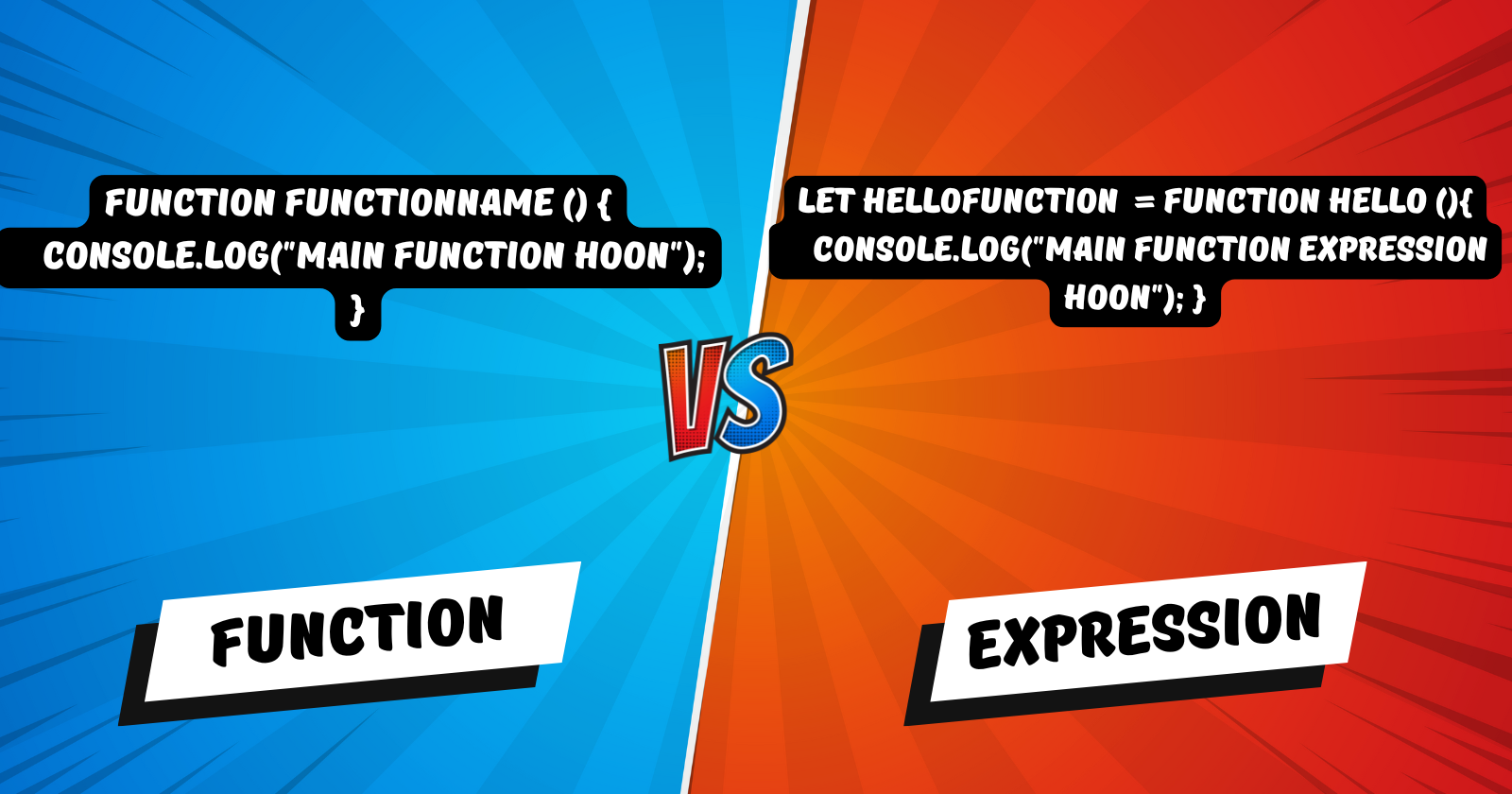
Function Kya Hai?
JavaScript mein function ek reusable code block hota hai jo specific task perform karta hai. Function declare karne ke liye hum function
keyword ka use karte hain, uske baad function ka naam, parentheses (jismein parameters ho sakte hain) aur curly braces {}
ke andar code likhte hain aur isko hum FUNCTION DECLARATION kehte hain.
Example
function functionname () {
console.log("Main function hoon");
}
functionname();
Yeh aap ka function hogaya, function keyword, function ka naam, parentheses and curly braces open and close and iske andaar apna logic likhege, yahan par function console log mein print kar raha hai and neeche hum usko call kar rahe hain uske naam se.
Function Parameters aur Data Types
Jab hum function ko call karte hain, toh hum parameters (arguments) pass karte hain. JavaScript mein parameters ke data type call time par decide hote hain. Agar missing arguments diye jayein, toh wo undefined ho jate hain.
Example
function add (num1, num2){
console.log(num1 + num2);
}
add(4, 5);
add("Syed", "Ahmed");
Yahan par function define kiya with keyword function aur uska naam hai add and aur wo do parameters le raha hai, num1 and num2 , iska datatype kya hai, number hai, magar kaise pata chala, jab add function call kiya toh humne 4, 5 pass kiya toh yeh num1 and num2 number hogaya, next line mein humne two strings pass kiye hain, toh num1 and num2 ab strings hogaye, toh pata chala ke jab hum function ko call karte hain and input pass karte hain toh call time pe datatype ka pata chalta hai, yahan pe number, strings, array, object, function bhi as parameter pass kar sakte hain
Alag-Alag Data Types ke Saath Function Call
Aap function ko alag-alag data types ke arguments ke saath bhi call kar sakte hain:
function add(num1, num2){
console.log(num1 + num2);
}
add(4,5); //number pass kiya
add("Syed", "Ahmed"); //strings pass kiya
add([1,2], [3,4]); //arrays pass kiya
add({firstname:"Syed"}, {lastname:"Ahmed"}); //key value pair pass kiya yani object
Toh above examples humne numbers paas kiya, toh num1 and num2 numbers datatype honge, phir next call mein humne string pass kiya, phir next call mein array pass kiya, phir next call object pass kiya, toh brain.exe use karo aur batao console log mein kya aayega?
Default Parameters
Agar aap function ko kam arguments ke saath call karte hain (declared parameters se kam), toh missing values by default undefined set ho jati hain. Kabhi-kabhi yeh acceptable hai, lekin kai baar aap chahte hain ki agar koi argument na diya gaya ho toh uska ek default value ho. ES6 se aap function parameters ke liye default values specify kar sakte hain.
Default Parameter Example
function myFunction(x, y = 10) {
return x + y;
}
console.log(myFunction(5)); // Output: 15, kyunki y default value 10 use hui
Yahaan, agar y
argument pass nahi hota ya undefined
hota hai, toh automatically y = 10
set ho jata hai.
Chalo Function pass karte hain
JavaScript mein functions bhi ek data type hote hain. Aap function ko argument ke roop mein dusre function mein pass kar sakte hain.
Function as Parameter Example
function add(num1, num2){
console.log(num1() + num2());
}
add(function () {
return 5;
},
function () {
return 6;
});
Above code mein function as parameter pass kiya hai, toh num1, num2 yahan par functions hain, aur console log mein humne num1() and num2() ko call kiya jo respective values return kar raha hai.
Function Expression Kya Hota Hai?
Function Expression mein hum function ko ek variable mein store kar dete hain. Is tarah ke functions ko hum variable ke naam se call karte hain, na ki function ke naam se, isiko hum FUNCTION EXPRESSION kehte hain, chalo jaante hain code se
Function Expression Example
let hellofunction = function hello (){
console.log("main function expression hoon");
}
//hello() aise call nahi kar sakte kyun ki function expression hai toh variable ke naam se
//usko bulayenge na ki function ke naam se
//variable ke naam se call karna padega
hellofunction();
Function Expression with Parameters
//function expression parameters kaise pass kare
Let storefunction = function add (num1, num2){
console.log(num1 + num2);
}
//add(4,5) jaise maine upar kaha function ke naam se call nahi kar sakte
//kyun ki function expression hai toh variable ke naam se usko bulayenge na ki function
//ke naam se
storefunction(4,5);
Above code mein humne hellofunction variable mein function hello store kiya hai and function kar kya raha hai, woh kuch string print kar raha hain console mein, ab tumme lage ga isko call kaise kare kya hello() se call kare, nahi, kyun ke hellofunction variable hai aur usme humne function ko store kiya hai, toh humme variable ke naam se call karna padega, variable ka naam aur parentheses, toh hellofunction() aise call karte hain.
Isi tarah storefunction variable mein add naam ka function store kiya hai with parameters num1 and num2, yahan par dekh sakhte hain humne function call karne ke liye variable ka naam use nahi kiya, balke humne storefunction variable se call kiya, par jo function humme call karna hai usko two parameters(input) chahiye, toh woh pass karna padega, toh likhte hain variable ka naam parentheses aur uske andar parameters pass kardenge, toh storefunction(4.5) aise call karenge
Ummid hai aapko Function aur function ka expression ka basic syntax samaj aagaya hoga, chalo next topic pe chalte hain
Anonymous (Benaam) Function
Jab function expression define karte hain aur function ko koi naam nahi dete, toh use Anonymous Function kehte hain. Agar naam dete hain to use Named Function Expression kehte hain, chaliye code se jaante hain
Anonymous Function Example
//named function
let functionname = function hello(){
console.log("Mein named hoon");
}
functionname();
//Anonymous function
let benaamfunciton = function (){
console.log("Mein benaam hoon");
}
benaamfunction();
Hoisting aur Function Overriding
JavaScript mein hoisting ka matlab hai ki function declarations ko program run hone se pehle memory mein allocate kar diya jata hai. Is wajah se aap function declaration ko call kar sakte hain, chahe woh call se pehle likha ho ya baad mein. Lekin, agar same naam se multiple function declarations ho, toh last wali declaration sab par override kar jaati hai.
Example Code 1
Chalo ab itna sikh liya hai toh ab kuch tricky code dekhte hain
sayHello();
function sayHello() {
console.log("Hello from function declaration!");
console.log("Works even before the function is declared");
}
sayHello();
function sayHello() {
console.log("Hello from function declaration!");
console.log("Works after the function is declared");
}
sayHello();
function sayHello() {
console.log("Hello from function declaration!");
console.log("this is the end function declaration");
}
sayHello();
Is example mein, sayHello()
function ke teen declarations hain. Global execution context ke hisaab se, sabse last wali declaration override kar degi baaki ko, chalo detail se jaante hain aur samajte hain.
Function declare karne se pehle hi function call hogaya, kya yeh execute karega ya error dega, aap ko lag raha hoga yeh error dega, per nahi yeh error nahi dega, kyun, kyunki function saare jab bhi define hote hain woh GLOBAL EXCECUTION CONTEXT mein hote hain, kya matlab, jab program run hone se pehle aapke code ko execution engine padta hai aur jo bhi function declaration(naki function expression) hai unko global context deta hai, yani memory allocate kar deta hai, isi concept ko HOISTING kehte hain, magar variables ka aisa nahi hota, usko memory jab hi milti hai jab code acutally top to bottom execute hota hai, ek aur cheez agar same naam se multiple function honge toh jo sab se last function hai woh execute hoga
Above code mein 3 sayHello() function hai same naam se, par execute niche wala hoga, kyun same function sayHello() 3 baar repeat hora hai, toh dusra wala pehle ko override kardega, aur theesra dusre ko override kardega, Ab socho output kya aayega aur comment karo.
Example Code 2
Iska output kya hoga, brain.exe lagao aur comment karo, socho aur comment karo
sayHello(); //Kya output hoga
function sayHello() {
console.log("Function Declaration: Initial");
}
{
let sayHello = "Main Kaun hoon";
console.log(sayHello); //Kya output hoga
}
sayHello(); //Kya output hoga
function sayHello() {
console.log("Function Declaration: Final");
}
sayHello(); //Kya output hoga
Example Code 3 (Function Expression ke saath)
Chalo samaj liya above code, ab kuch aur dimag ki daahi kare, below code ka kya output aageya, comment karo, Global execution concept (HOISTING) use karo aur function expression jo hum ne seekha hai use karo, maine function expression ko kuch define nahi kiya hai, jaise LET, CONST, VAR, tum add karke khelo aur samjho ki code phatega ya chalega
sayHello();
function sayHello() {
console.log("Function Declaration");
}
sayHello = function () {
console.log("Function Expression");
};
sayHello();
function sayHello() {
console.log("Function Declaration 2");
}
sayHello();
Note: Function expressions ko hoisting nahi milti (agar variable ko let
ya const
se declare kiya gaya ho) aur agar same naam se function declarations ho, toh last wali declaration hi effective rahegi.
Example Code 4 (Mix of Declaration and Expression)
Chalo niche wala function execute hoga aur kya output aayega, comment karo
sayHello();
function sayHello() {
console.log("Initial Declaration");
}
const sayHello = function () {
console.log("Function Expression");
};
sayHello();
Callback Function
Callback Function woh function hota hai jo kisi dusre function ko argument ke roop mein diya jata hai, aur us dusre function ke andar baad mein call kiya jata hai. Callback ka use asynchronous operations ya kisi event ke response mein hota hai, chaliye code se samajte hain
Callback Function Example
sum(3, 5, displaySum);
function displaySum(result) {
console.log("Result is : " + result);
}
function sum(num1, num2, mycallback) {
let sum = num1 + num2;
mycallback(sum);
}
Above code mein displaySum
ko callback function ke roop mein sum
mein pass kiya gaya hai. displaySum
ko callback function kaha jata hai kyunki woh as argument or parameter pass kiya hai sum function mein, aur jab bhi hum function as an argument pass karte hain parentheses nahi lagate hain, sum function ko humne call kiya with 3, 5 and displaySum as arguments, 3, 5 numbers hain aur displaySum as function argument pass kiya hai.
Arrow Functions
Arrow function kya hai, arrow function ek short form hai function expression ko define karne ke liye, aur yeh concise syntax hai function expression ko likhne ka. Ye anonymous functions create karne mein kaafi useful hote hain, aur inka this
binding lexical hota hai, chaliye code se jaante hain
let functionExpression = function (a, b){
return a+b
}; //yeh function expression hai
let arrowfunction = (a,b) => a+b; //arrow function
// Arrow Function (with multiple statements)
let arrowfunction1 = (a,b) => {
let sum = a + b;
return sum;
};
Humne above code likha dono correct hain aur dono execute honge, phela function expression hai aur ussi function expression ko short form mein likha hai ussko arrow function kehte hain, bina koi function keyword use kare bina sirf parentheses aur usme parameters, uske baad => symbol aur phir curly braces and code logic.
Functions as Objects
JavaScript mein functions ko objects ke roop mein bhi samjha ja sakta hai. Halanki typeof
operator functions ke liye "function" return karta hai, asal mein functions objects hi hote hain. Inke paas properties aur methods dono hote hain.
Properties:
- arguments.length: Jab function call hota hai, toh
arguments.length
property se yeh pata chalta hai ki kitne arguments pass kiye gaye.
- arguments.length: Jab function call hota hai, toh
Methods:
- toString(): Is method se function ka pura source code string ke roop mein mil jata hai.
Example Code
function greet(name) {
console.log("Hello, " + name);
console.log("Arguments received: " + arguments.length);
}
greet("Syed");
// Output:
// Hello, Syed
// Arguments received: 1
console.log(greet.toString());
// Output: Function 'greet' ka pura source code as a string
Is example se aap dekh sakte hain ki functions ke paas kitni flexibility hai—yeh na sirf executable code hote hain balki unke paas apni properties aur methods bhi hoti hain.
Extra Concept: Immediately Invoked Function Expression (IIFE)
IIFE ek aisa function hota hai jo define hote hi turant execute ho jata hai. Ye mainly variable scope ko limit karne ke liye use hota hai.
IIFE Example
(function() {
console.log("Yeh IIFE turant execute ho gaya!");
})();
IIFE se aap global scope ko pollute hue bina temporary variables ya logic execute kar sakte hain.
Function Rest Parameter
Jab ek function ko zyada arguments ke saath call kiya jata hai (declared parameters se zyada), toh ES6 ka rest parameter (...
) use karke aap in extra arguments ko ek array ke roop mein capture kar sakte hain. Ye feature functions ko flexible banata hai, jahan aap indefinite number of arguments handle kar sakte hain.
Rest Parameter Example
function multiply(multiplier, ...numbers) {
return numbers.map(num => num * multiplier);
}
console.log(multiply(2, 1, 2, 3)); // Output: [2, 4, 6]
Agar aap purane tarike se kaam karna chahte hain, toh ES5 mein aap arguments object ka use karke bhi extra arguments access kar sakte hain:
function showArguments() {
for (let i = 0; i < arguments.length; i++) {
console.log(arguments[i]);
}
}
showArguments(1, 2, 3, 4);
// Output: 1, 2, 3, 4
Arguments Passing: By Value vs. By Reference
Arguments are Passed by Value
JavaScript mein, function call ke dauran jo parameters diye jate hain, unhe arguments kehte hain. Primitive values (jaise numbers, strings, booleans) by value pass hoti hain. Iska matlab hai ki function sirf us value ka copy receive karta hai. Agar function ke andar value change hoti hai, toh original value affect nahi hoti.
Example: Pass by Value
function changeValue(a) {
a = 10;
}
let x = 5;
changeValue(x);
console.log(x); // Output: 5, kyunki x ka original value change nahi hua
Objects are Passed by Reference
JavaScript mein, objects ke case mein, unke references values hote hain. Isliye, agar aap function ke andar kisi object ke property ko change karte hain, toh woh change original object mein reflect hota hai.
Example: Pass by Reference
function modifyObject(obj) {
obj.name = "Changed";
}
let person = { name: "Original" };
modifyObject(person);
console.log(person.name); // Output: "Changed", kyunki object ke reference se original value update hui
this Keyword
JavaScript mein this keyword ka behavior alag-alag contexts par depend karta hai. Iska use function ya object ke context ko refer karne ke liye hota hai. Chaliye, alag-alag scenarios ke saath samajhte hain:
Global Context
Global scope mein, browser environment mein this window
object ko refer karta hai aur Node JS kya refer karta hai, VS code mein run karke dekho
console.log(this); // Browser mein: window object
Function Context
Normal function ke andar, agar aap this use karte hain:
Non-strict mode:
this
global object ko refer karta hai (browser meinwindow
).Strict mode:
this
undefined hota hai.
function showThis() {
console.log(this);
}
showThis(); // Non-strict mode mein: window; Strict mode mein: undefined
Object Method Context
Jab koi function object ke method ke roop mein likha jata hai, toh this us object ko refer karta hai jismein woh method defined hai.
const person = {
name: 'Syed',
greet: function() {
console.log("Hello, " + this.name);
}
};
person.greet(); // Output: Hello, Syed
Arrow Functions
Arrow functions ka apna this nahi hota. Ye parent (lexical) scope ka this inherit karte hain.
const person2 = {
name: 'Ahmed',
greet: () => {
console.log("Hello, " + this.name);
}
};
person2.greet(); // Output: "Hello, undefined" — kyunki arrow function apna alag this bind nahi karta
Arrow Function ko Sahi Context Mein Use Karne Ka Example
Agar aap arrow function ka fayda uthana chahte hain aur correct this reference chahte hain, toh aap parent function ke andar arrow function define kar sakte hain:
const person3 = {
name: 'Ali',
greet: function() {
// yahan parent function ka this (person3) arrow function mein inherit ho jayega
const inner = () => {
console.log("Hello, " + this.name);
};
inner();
}
};
person3.greet(); // Output: Hello, Ali
Summary
Function Declaration:
function
keyword ke saath defined reusable code blocks, jo hoisting se benefit uthate hain.Parameters aur Data Types: Function call ke dauran pass hone wale arguments, jinhe runtime par evaluate kiya jata hai.
Function Expression: Functions ko variables mein store karke likhna, jismein naam optional hota hai.
Anonymous Functions: Naam ke bina likhe gaye functions, jo aksar function expressions mein use hote hain.
Hoisting: Function declarations ko run hone se pehle memory mein allocate kar diya jata hai; last declaration hi effective hoti hai.
Callback Functions: Dusre functions ke argument ke roop mein pass hone wale functions, jo asynchronous ya event-driven scenarios mein use hote hain.
Arrow Functions: Concise syntax ke saath functions likhne ka tareeka, jismein lexical
this
binding hota hai.Functions as Objects: Functions objects jaise properties (jaise
arguments.length
) aur methods (jaisetoString()
) rakhte hain.IIFE: Immediately Invoked Function Expressions, jo define hote hi turant execute ho jate hain.
Default Parameters: Missing arguments ke liye default value set karne ki suvidha.
Rest Parameters & Arguments Object: Extra arguments ko array ke roop mein handle karna ya traditional
arguments
object ka use karna.Pass by Value vs. Reference: Primitives by value pass hote hain, jabki objects unke references ke through pass hote hain.
this Keyword: Different contexts mein
this
ka behavior (global, function, object, arrow functions).
Is comprehensive guide se aap JavaScript functions ke sabhi important aspects ko detail mein samajh sakte hain. Har section mein diye gaye examples ko aap khud run karke inke behavior ko explore kar sakte hain. Happy coding!
Subscribe to my newsletter
Read articles from Syed Ahmed SM directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
