Boost Your JavaScript Skills: Strict Mode, Modern Variables, and Key Practices #2

Table of contents
- Today, we'll explore essential JavaScript concepts that can make your coding life easier and more robust. We’ll dive into Strict Mode , understand how to work with modern variables using let and const, and learn why the old var is now mostly a relic of the past. Along the way, I'll share some valuable tips and best practices to help you write cleaner, safer code.
- 1. Strict Mode: Code with Confidence
- 2. Modern Variables: Using let & const
- 3. var: The Old Timer of JavaScript
- 4. Tips & Best Practices
- 5. Bringing It All Together: A Sample Code
- Conclusion
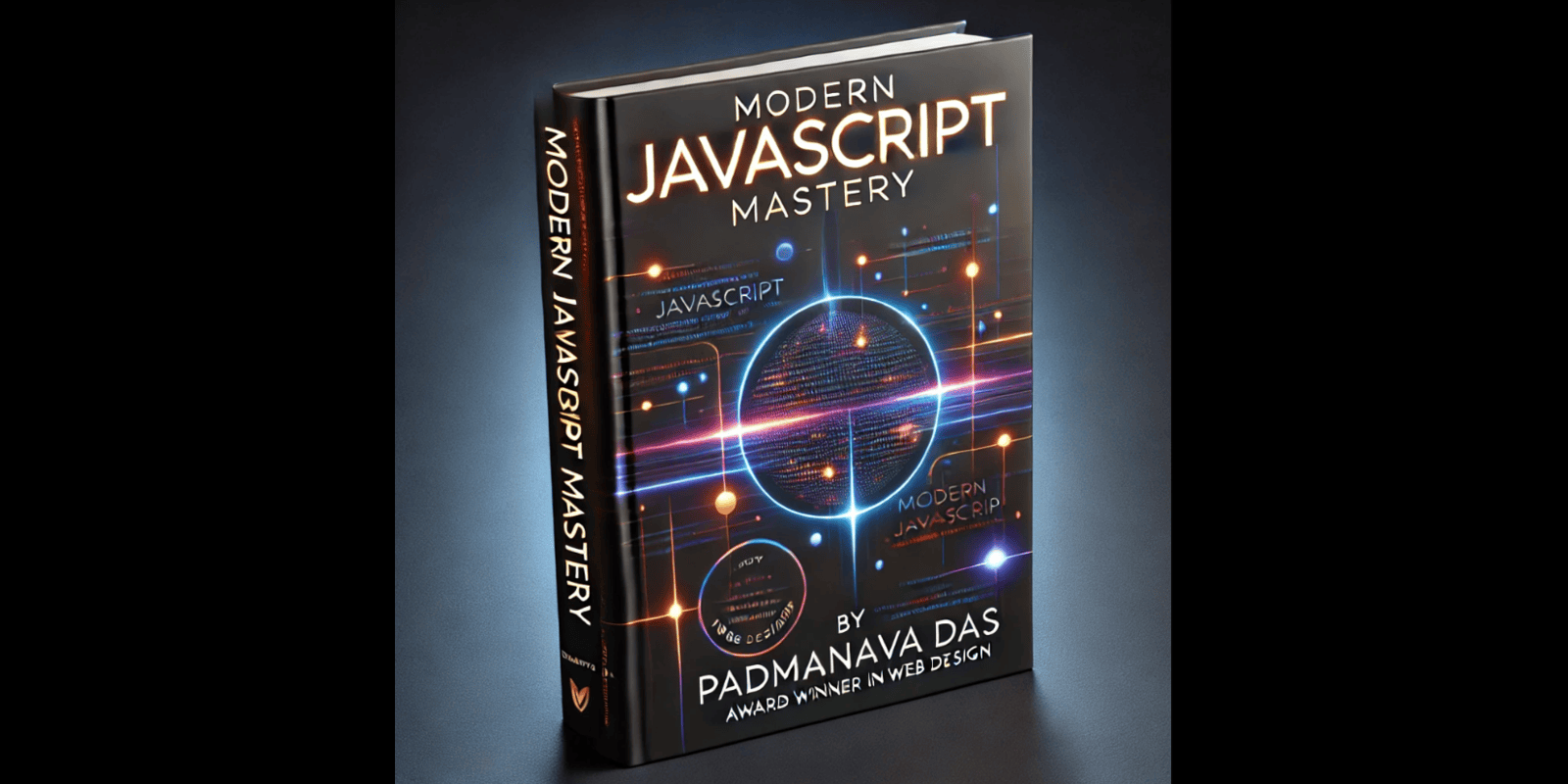
Hi Bondhura!
Kamon Acho?
Today, we'll explore essential JavaScript concepts that can make your coding life easier and more robust. We’ll dive into Strict Mode , understand how to work with modern variables using let
and const
, and learn why the old var
is now mostly a relic of the past. Along the way, I'll share some valuable tips and best practices to help you write cleaner, safer code.
1. Strict Mode: Code with Confidence
What is Strict Mode?
Imagine playing a board game with clear, well-defined rules. There’s no guessing what’s allowed or what isn’t. That’s what strict mode does in JavaScript—it enforces a cleaner, more secure way to write code by catching common mistakes early on.
How to Enable Strict Mode
To activate strict mode, simply add the following line at the very top of your script or within a function:
"use strict"; // Enabling strict mode
function greet() {
console.log("Hello, world!");
}
greet();
Remember:
Top Position:
"use strict";
must appear at the very beginning of your script or function (apart from comments) to work correctly.Scoped Application: You can apply strict mode globally for your script or locally within a specific function.
Irreversible: Once enabled in a scope, strict mode stays in effect for that scope.
2. Modern Variables: Using let & const
What Are Variables?
Think of variables as labeled boxes where you can store your favorite toys (or data). They hold values like strings, numbers, or objects, and you can change what's inside as needed.
Declaring Variables with let and const
The let Keyword
Use let
when you expect the variable’s value to change.
"use strict";
let message = "Hello, world!";
console.log(message); // Outputs: Hello, world!
// Updating the value is perfectly fine with let
message = "Hello, JavaScript!";
console.log(message); // Outputs: Hello, JavaScript!
The const Keyword
Use const
for values that you don't want to change after they’re set.
"use strict";
const OUR_PLANET = "Earth";
console.log("We live on " + OUR_PLANET);
// The following line would cause an error because OUR_PLANET is a constant:
// OUR_PLANET = "Mars";
Why use let and const?
Block Scope: They are limited to the block (i.e., within curly braces
{}
) in which they are defined, reducing unexpected behavior.Clarity: Use
const
by default, and only uselet
when you know the variable's value will change. This makes your code more predictable.
3. var: The Old Timer of JavaScript
Before let
and const
, var
was the standard way to declare variables. However, it comes with quirks that modern JavaScript aims to avoid.
Issues with var:
Function Scope vs. Block Scope:
var
is scoped to the entire function, not just the block. This can lead to unexpected results.if (true) { var greeting = "Hello with var!"; } console.log(greeting); // Outputs: "Hello with var!" even though it's inside an if-block.
Hoisting: Variables declared with
var
are “hoisted” (moved to the top of their scope) during compilation. This can cause confusion if you try to use them before they're defined.console.log(hoistedVar); // Outputs: undefined, not an error var hoistedVar = "I was hoisted!";
Why Prefer let and const Over var?
Predictability: With block-scoping,
let
andconst
ensure variables only exist where you intend them to.Fewer Bugs: The pitfalls of hoisting and scope leakage with
var
can lead to subtle bugs that are hard to track down.Modern Best Practices: Most modern coding standards and linters recommend avoiding
var
for cleaner, more maintainable code.
4. Tips & Best Practices
Use Strict Mode for Better Code Quality
Always Start with
"use strict";
: Place it at the top of your scripts to catch common mistakes early.Function-Specific Strict Mode: If you’re working on a legacy codebase, consider enabling strict mode for new functions to gradually improve code quality.
Choose the Right Variable Declaration
Default to const: Use
const
unless you know the variable’s value will change.Switch to let When Necessary: If the value must change (like counters in loops or user input), then use
let
.Avoid var: Minimize or eliminate the use of
var
to prevent scope and hoisting issues.
Write Readable and Maintainable Code
Descriptive Names: Use meaningful names for variables (e.g.,
currentUser
rather than justuser
).Keep Your Scope Clear: Declare variables in the smallest scope possible to avoid unintended side effects.
Comment When Needed: Brief comments can help others (or future you) understand why certain choices were made.
5. Bringing It All Together: A Sample Code
Here's a little example that combines strict mode, modern variable declarations, and a cautionary note on var:
"use strict"; // Enable strict mode
// Modern variable declarations using let and const
let currentUser = "Rahul"; // This might change over time
const OUR_PLANET = "Earth"; // Our planet's name is constant
function greetUser() {
console.log("Namaste " + currentUser + ", welcome to our beautiful " + OUR_PLANET + "!");
}
greetUser(); // Outputs: Namaste Rahul, welcome to our beautiful Earth!
// Example showing a pitfall of var:
function legacyVarExample() {
if (true) {
var oldVar = "I belong to the entire function!";
}
console.log(oldVar); // This works, but it's risky since oldVar is accessible anywhere in the function.
}
legacyVarExample();
Conclusion
JavaScript is a versatile language that has evolved significantly over the years. By embracing strict mode and using modern variable declarations (let
and const
), you can write safer, more predictable code. Understanding the quirks of var
reminds us of the progress we've made and why it's best to stick with modern practices.
I hope this guide, helps you feel confident in writing robust JavaScript code. Happy coding, and keep exploring the amazing world of JavaScript!
Feel free to share your thoughts or ask questions in the comments below. Let's learn and grow together!
Subscribe to my newsletter
Read articles from Padmanava Das directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Padmanava Das
Padmanava Das
From Republic of Bharat