How I Made AI Analyze Code Like a Seasoned Software Engineer

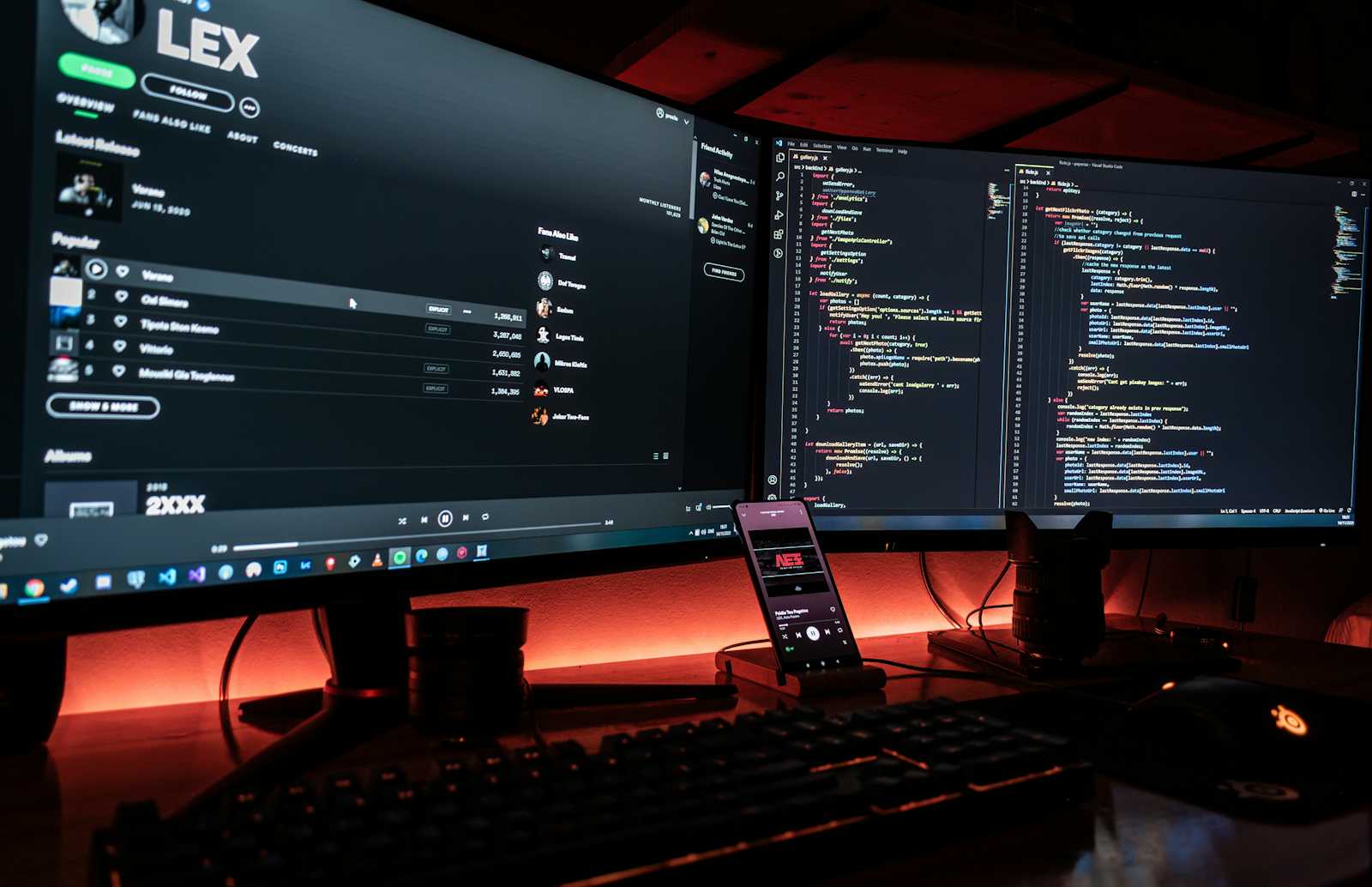
Last week, I watched our AI choke on a React codebase—again. As timeout errors flooded my terminal, something clicked. We had been teaching AI to read code like a fresh boot camp graduate, not a senior developer. Here’s what I mean:
The Bootcamp vs. Senior Developer Mindset
Think back to your first time reading a production codebase. Without experience handling mature projects, you likely got lost in the details—jumping from file to file, trying to piece things together without a clear strategy.
Now, contrast that with how a senior developer reviews a massive pull request:
They jump straight to the core files—focusing on the most critical changes first.
They group changes by feature (e.g., all authentication updates, all database modifications).
They build a mental model of the architecture first before diving into specific implementations.
Only then do they go deep into individual lines of code.
This is obvious in hindsight, right? But our AI wasn’t following this pattern at all.
The Experiment: Teaching AI to Think Like a Senior Dev
Instead of processing files in a linear fashion, we built a context-aware grouping system that mimics how experienced developers review code.
Structuring Code Analysis Like a Pro
We defined a structure that categorizes files based on their functionality and importance:
interface FileGroup {
files: ProjectFile[];
totalSize: number;
groupContext: string; // e.g., 'auth', 'database', etc.
}
export const groupFiles = (files: ProjectFile[]): FileGroup[] => {
// Group files by related functionality and size
const fileInfos = files.map(file => ({
file,
size: file.content?.length || 0,
context: getFileContext(file.path)
}));
// Process larger, more important files first
fileInfos.sort((a, b) => b.size - a.size);
const groups: FileGroup[] = [];
let currentGroup = createEmptyGroup();
for (const { file, size, context } of fileInfos) {
if (shouldStartNewGroup(currentGroup, size, context)) {
groups.push(currentGroup);
currentGroup = createNewGroup(file, size, context);
} else {
addFileToGroup(currentGroup, file, size);
}
}
return groups;
};
Giving AI Context First, Not Just Code
Instead of a naive “analyze this file” approach, we structured our AI prompts to provide system-level context before asking for analysis:
const buildGroupPrompt = (group: FileGroup): string => {
return `
Analyzing authentication system files:
- Core token validation logic
- Session management
- Related middleware
Focus on:
1. How these integrate with existing auth patterns
2. Security implications
3. Performance impact on other systems
Files to analyze:
${formatFiles(group.files)}
`;
};
The “Holy Shit” Moment
The results shattered our expectations. We initially thought we had broken our testing scripts.
Before this experiment, AI-generated insights looked like this:
"This file contains authentication logic using JWT tokens."
After implementing our new system, AI outputs transformed into:
Warning: This auth change could impact websocket connections. The token refresh logic shares patterns with the notification service (added last month), suggesting a potential race condition during high-traffic socket reconnects.
Related PR: #1234 (merged last week) modified the same retry logic. Consider adding backoff.
That’s senior developer-level awareness. The AI was now catching cross-service connections we hadn’t explicitly taught it about.
What Actually Changed?
The breakthrough wasn’t about fancy machine learning techniques or scaling up the model. The improvements came from teaching AI to think like a senior developer:
Context First: Load the system architecture into memory before analyzing code.
Pattern Matching: Group related files together to identify broader trends.
Impact Analysis: Understand how a change in one part of the codebase affects the entire system.
Historical Understanding: Track previous changes and why the code evolved a certain way.
Unexpected Side Effects
Beyond our initial goals, the AI started catching things we didn’t specifically train it for:
Spotting copy-pasted code across different features.
Flagging inconsistent error handling patterns.
Detecting potential performance bottlenecks.
Suggesting architectural improvements based on real-world usage patterns.
Why This Matters
Every week, a new “AI-powered coding assistant” pops up on Product Hunt. But they all seem to miss the point. Code suggestions without deep context are useless.
It’s like having a brilliant junior dev who just joined the team—they write syntactically perfect code that subtly breaks everything because they don’t understand the system.
The real problem isn’t making AI generate better code. It’s making AI understand code the way experienced engineers do.
Open Questions
We’re still experimenting with:
When to refresh vs. preserve historical context in AI’s understanding.
How to handle conflicting patterns in different parts of the system.
Whether to expose uncertainty in AI analysis (e.g., “I'm 80% confident this will break”).
What’s Next?
If we can teach AI to read code like a senior developer, could we push it even further?
Future Possibilities
Identifying tech debt before it becomes a problem.
Suggesting architectural improvements based on long-term patterns.
Catching security vulnerabilities by analyzing how authentication flows interact with other services.
Understanding unwritten team conventions and enforcing best practices automatically.
The real future of AI in software development isn’t about writing more code. It’s about teaching AI to think about code like a human expert.
Hashtags
#AI #MachineLearning #SoftwareEngineering #CodeReview #SeniorDev #ArtificialIntelligence #Programming #TechInnovation
Subscribe to my newsletter
Read articles from Ovilash Jalui directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Ovilash Jalui
Ovilash Jalui
My name is Ovilash Jalui, and I am a Full Stack Developer. I create web apps that are simple, user friendly, and help businesses grow online. My main focus is building websites for businesses and individuals who want to stand out and connect with more people. Whether it’s bringing a startup’s idea to life with apps and digital products or using AI to make them smarter, I use the latest technology and strategies to boost online presence and help businesses grow faster.