Callbacks in JavaScript
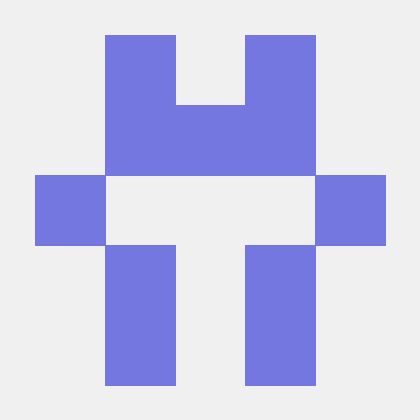
hey folks let’s understand callbacks in a easy way. we use callbacks to save ourselves from DRY(don’t repeat yourself) problem like we can understand from a example:
LIFE BEFORE CALLBACK
function square(n){
return n*n;
}
function cube(n){
return n*n*n;
}
function quad(n){
return n*n*n*n;
}
function sumOfSquare(a,b){
let square1 = square(a);
let square2 = square(b);
return square1 + square2;
}
function sumOfCube(a,b){
let cube1 = cube(a);
let cube2 = cube(b);
return cube1 + cube2;
}
let ans = sumOfSquare(2,3);
console.log(ans);
ans = sumOfCube(2,3);
console.log(ans);
if you look in the code you find i use separate functions for cube and square if i want quad then i have to call one another function which calculate sum of quad this repetition of functions is a problem called DRY.
To overcome this problem callback introduced as a solution in JavaScript.
function square(n){
return n*n;
}
function cube(n){
return n*n*n;
}
function quad(n){
return n*n*n*n;
}
function sumOfSomething(a,b,cb){
let operation1 = cb(a);
let operation2 = cb(b);
return operation1 + operation2;
}
let ans = sumOfSomething(2,3,cube); // Line A
console.log(ans);
//Output:35
here ‘‘cb’’ is callback which takes function as a argument so that i don’t have to take separate functions for cube , square and quad we just have to change the argument in “Line A” from cube to square or quad and you are done now you don’t have to make other functions for each operations like we use earlier.
Subscribe to my newsletter
Read articles from aditya kushwaha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
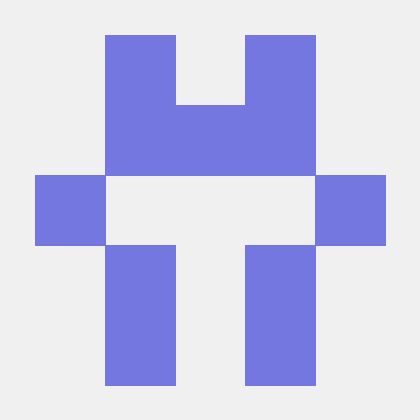