Automating Git Branch Checkouts via PHP & GET Requests

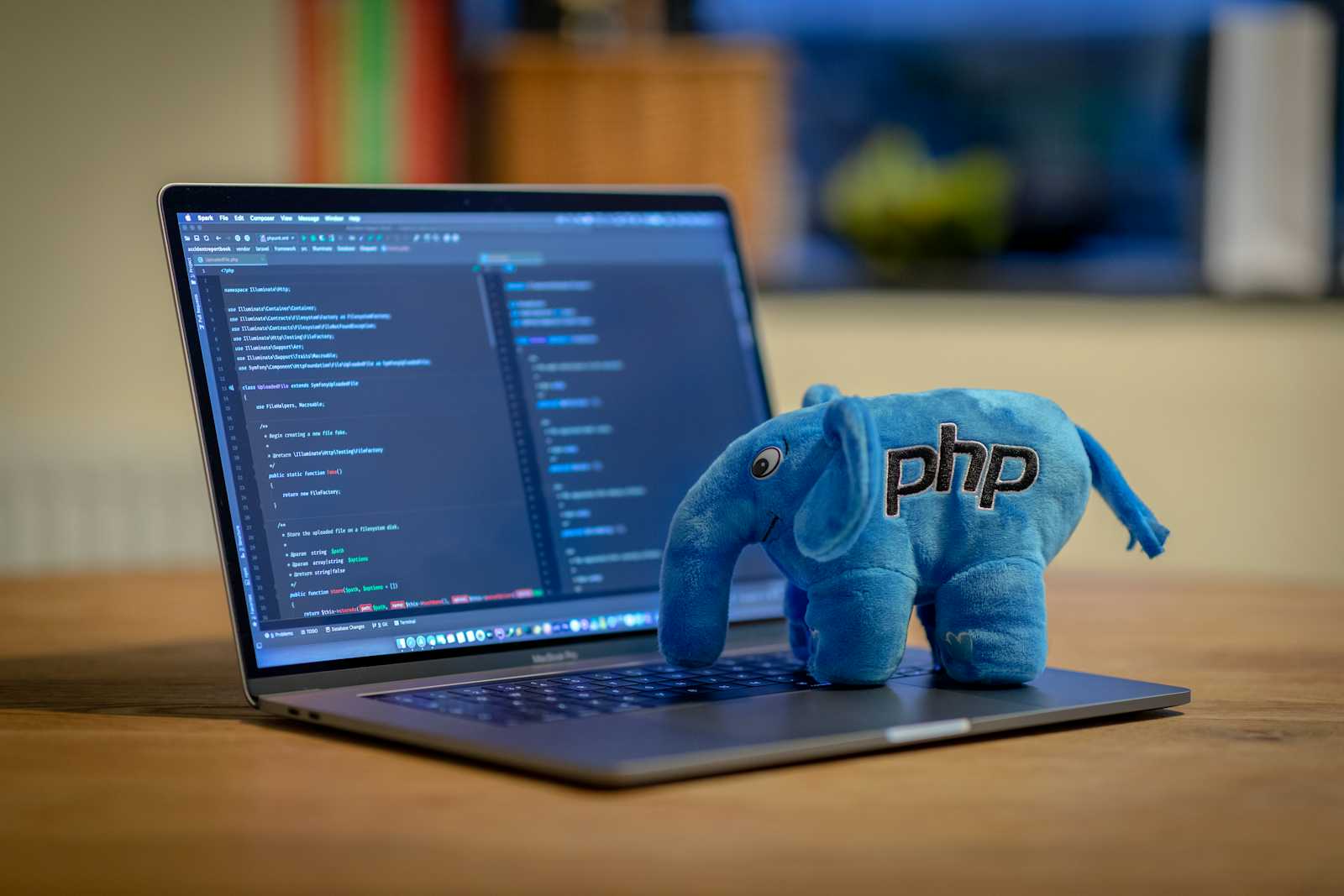
Introduction
Managing multiple branches in Git is essential for efficient software development. Different branches help in working on various features, fixes, and layout modifications simultaneously. However, managing these branches directly on a remote server can be cumbersome. The standard process involves:
SSHing into the server
Navigating to the project directory
Running
git checkout <branch>
This workflow becomes repetitive and tiring, especially when multiple developers frequently switch branches for testing or deployment.
To address this, we introduce a simple PHP-based solution that allows switching branches using a web browser. With this approach, you can perform a Git checkout operation remotely without needing SSH access every time.
The Concept
The idea is to develop a lightweight PHP script that:
Accepts a branch name as a GET request.
Uses that branch name to perform a Git checkout within the project directory.
Returns the output of the command to confirm success or failure.
With this solution, developers and team members can quickly switch branches directly from a browser by simply entering the branch name in a URL.
Why Use GET Requests?
We leverage GET requests for their simplicity and ease of use:
No need for complex forms or authentication.
Any user with access to the script can trigger a branch change.
Easy debugging since GET parameters are visible in the URL.
However, it's important to note that GET requests should be secured in a production environment to prevent unauthorized access.
Implementation
Step 1: Capturing the Branch Name
First, we capture the branch name passed via the GET request:
$branchname = $_GET['branch'];
Before proceeding, we ensure that a branch name is provided to prevent errors:
if (!$branchname) {
echo "Please enter a branch name. Example: ?branch=<name>";
return false;
}
This validation ensures that the script does not execute unnecessary commands when accessed without parameters.
Step 2: Executing the Git Checkout Command
The core of our script involves using shell_exec()
to execute the Git command:
$command = 'cd /path/to/your/repository && git checkout ' . escapeshellarg($branchname);
$output = shell_exec($command . ' 2>&1');
Key points to note:
The
cd
command ensures we are in the correct Git repository.git checkout <branch>
switches to the specified branch.escapeshellarg()
is used to sanitize user input, preventing command injection attacks.2>&1
captures both standard and error outputs, helping in debugging.
Step 3: Displaying the Output
Providing feedback is crucial so users know whether the operation was successful or not. We achieve this by echoing the output:
echo 'Checking out branch: ' . htmlspecialchars($branchname) . '...<br>';
echo nl2br(htmlspecialchars($output)) . '<br>';
Why use htmlspecialchars()
?
It prevents HTML injection, making the output safer for display.
nl2br()
converts newlines into<br>
tags for better readability in the browser.
Complete PHP Script
Here’s the full script, which you can save as checkout-git-branch.php
and upload to your server:
<?php
$branchname = $_GET['branch'] ?? '';
if (!$branchname) {
echo "Please enter a branch name. Example: ?branch=<name>";
return false;
}
$command = 'cd /path/to/your/repository && git checkout ' . escapeshellarg($branchname);
$output = shell_exec($command . ' 2>&1');
// Display the output
echo 'Checking out branch: ' . htmlspecialchars($branchname) . '...<br>';
echo nl2br(htmlspecialchars($output)) . '<br>';
?>
Security Considerations
While this script is simple and effective, it comes with potential security risks:
1. Command Injection Prevention
Never directly concatenate user input into shell commands. Instead, use escapeshellarg($branchname)
, which ensures that the input does not contain malicious code.
2. Restricting Access
Expose this script only to authorized users by implementing one of the following:
Basic Authentication: Use
.htpasswd
for authentication.IP Whitelisting: Allow only specific IP addresses to access the script.
Token-Based Security: Require a secret token in the GET request for validation.
Example of a token-based approach:
$token = $_GET['token'] ?? '';
$valid_token = 'your-secure-token';
if ($token !== $valid_token) {
echo "Unauthorized access.";
return false;
}
3. Limiting Allowed Branches
Restrict branch switching to specific branches to prevent accidental checkouts of sensitive or production branches:
$allowed_branches = ['develop', 'feature-x', 'bugfix-123'];
if (!in_array($branchname, $allowed_branches)) {
echo "Branch not allowed.";
return false;
}
Deployment and Testing
Step 1: Uploading the Script
Place checkout-git-branch.php
inside your web server directory (e.g., /var/www/html/
for Apache or /usr/share/nginx/html/
for Nginx).
Step 2: Setting Permissions
Ensure that the user running the web server has the necessary permissions to execute Git commands inside the repository:
sudo chown -R www-data:www-data /path/to/your/repository
sudo chmod -R 775 /path/to/your/repository
Step 3: Accessing the Script
To switch branches, open your browser and enter:
http://your-server.com/checkout-git-branch.php?branch=develop
If authentication or tokens are implemented, append the necessary credentials:
http://your-server.com/checkout-git-branch.php?branch=develop&token=your-secure-token
Conclusion
This PHP-based Git branch switching solution simplifies server-side branch management, reducing the need for SSH access. While convenient, ensure proper security measures are in place to avoid unauthorized access.
By implementing authentication, command sanitization, and branch restrictions, you can create a safe, efficient, and user-friendly Git workflow management tool.
Key Takeaways:
Automate Git branch switching using PHP & GET requests.
Use
shell_exec()
to rungit checkout
securely.Implement security measures to restrict unauthorized access.
Ensure proper file permissions for smooth execution.
Deploy the script on a web server for easy branch management.
With this script in place, you can switch branches instantly from your browser and make server-side Git workflows more efficient than ever!
Subscribe to my newsletter
Read articles from Ovilash Jalui directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Ovilash Jalui
Ovilash Jalui
My name is Ovilash Jalui, and I am a Full Stack Developer. I create web apps that are simple, user friendly, and help businesses grow online. My main focus is building websites for businesses and individuals who want to stand out and connect with more people. Whether it’s bringing a startup’s idea to life with apps and digital products or using AI to make them smarter, I use the latest technology and strategies to boost online presence and help businesses grow faster.