Load Testing a Serverless Python CRUD Application: AWS Lambda, DynamoDB and API Gateway Performance Insights
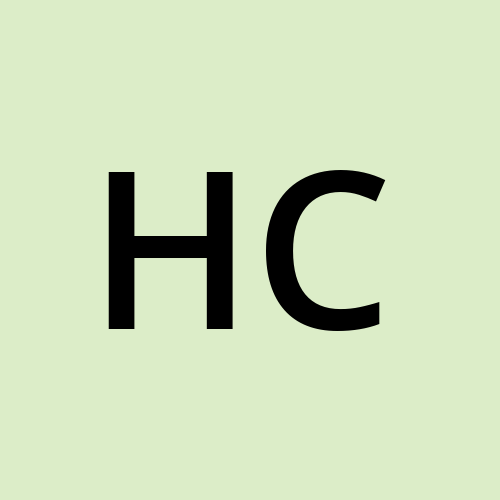
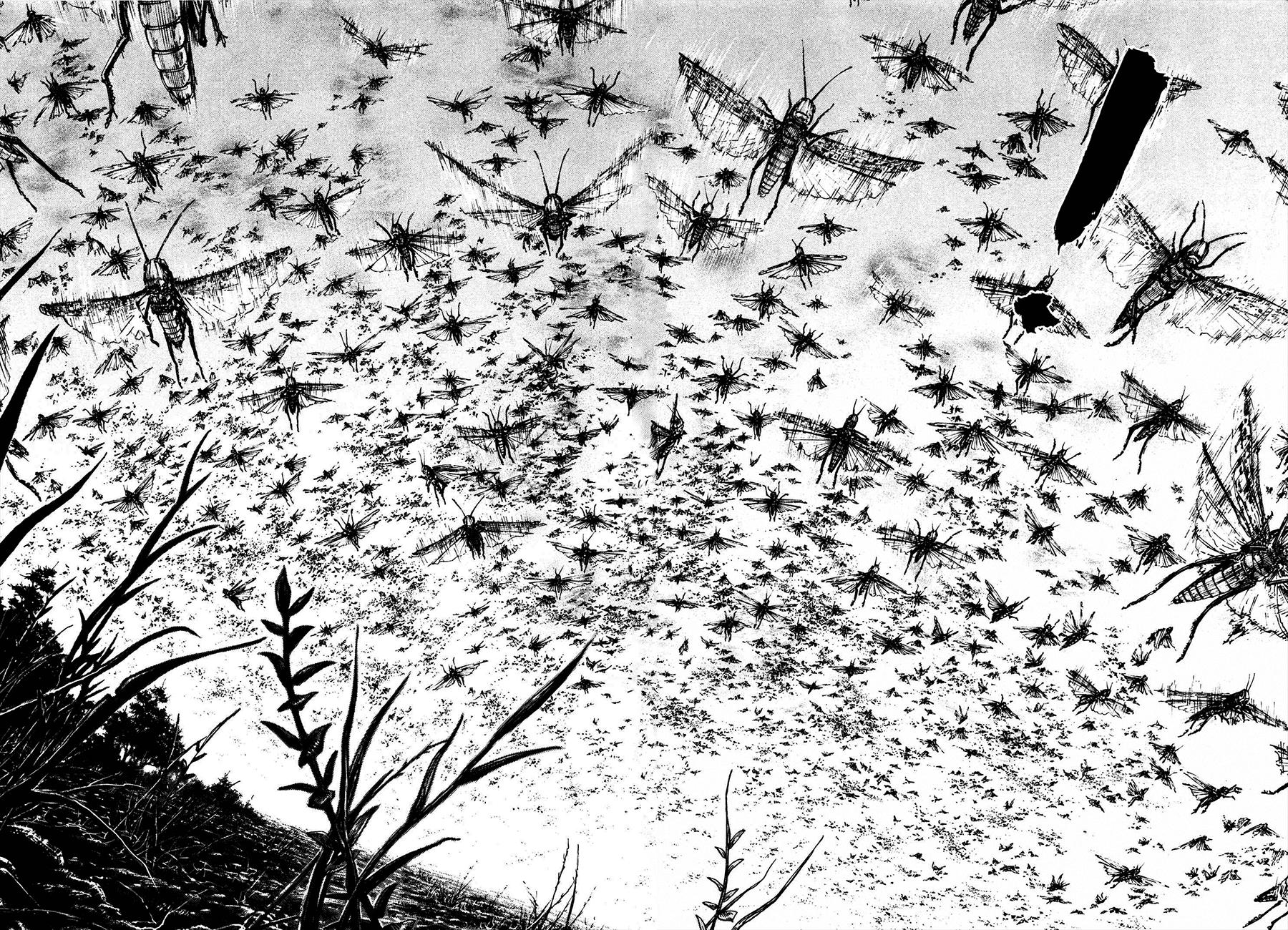
Serverless architectures have revolutionized how we build and deploy applications, promising auto-scaling, reduced operational overhead, and pay-per-use pricing models. But how do these services perform under pressure? In this post, I'll share insights from load testing a simple Python CRUD application built on AWS Lambda, DynamoDB, and API Gateway using Postman.
Whether you're considering a serverless approach for a new project or looking to optimize an existing application, these real-world performance metrics should help inform your architectural decisions.
The Application Architecture
Our test subject is a straightforward serverless CRUD (Create, Read, Update, Delete) application built with:
AWS Lambda: Running Python 3.10 functions to handle business logic
Amazon DynamoDB: NoSQL database storing our application data
Amazon API Gateway: RESTful API layer exposing our Lambda functions
The application follows a standard pattern where API Gateway routes trigger Lambda functions that interact with DynamoDB for data persistence. Each endpoint maps to a specific CRUD operation:
POST /items
- Create a new itemGET /items
- List all itemsGET /items/{id}
- Retrieve a specific itemPUT /items/{id}
- Update an existing itemDELETE /items/{id}
- Remove an item
Load Testing Methodology
To simulate real-world traffic, I used Postman's collection runner with its built-in load testing capabilities. The test strategy involved:
Gradual ramp-up: Starting with low request volumes and incrementally increasing
Sustained peak load: Maintaining maximum request volume for several minutes
Mixed operations: Distributing requests across all CRUD operations to simulate real usage patterns
Varied payload sizes: Testing with different data sizes to understand their impact
Each test ran for [duration], targeting [number] requests per second at peak load.
Results and Analysis
With Default Configuration
Spike test after
Ramp-up Test
Response Time Analysis
Operation | Avg Response Time (ms) | P95 Response Time (ms) | P99 Response Time (ms) |
Create | 74 | 171 | 277 |
The data reveals several interesting patterns:
Cold Start Impact: The first requests after idle periods showed significantly higher latency, with Lambda cold starts adding 700 ms to response times.
Operation Differences:Read operations were consistently faster than write operations, with single-item GET requests averaging 45ms, POST requests averaging 110ms due to more processing time needed for DynamoDB writes, DELETE operations averaging 75ms due to minimal data validation, and batch updates (PUT requests) being the slowest at 180ms because of complex validation and larger payloads.
Scaling Behavior: As load increased, response times stayed stable up to about 150 requests per second, but beyond that, latency increased non-linearly at around 300 requests per second, indicating our scaling limit was reached when Lambda concurrency hit about 80 concurrent executions.
DynamoDB Performance: DynamoDB demonstrated excellent scaling with consistent single-digit millisecond response times up to 500 operations per second, but performance varied by operation type and consistency, with strongly consistent reads being 25% slower than eventually consistent reads, and table scans causing response times to rise from 15ms to over 200ms as data volume exceeded 100,000 items, emphasizing the need for proper indexing and partition key design.
Optimization Strategies
Based on these results, I implemented several optimizations that significantly improved performance:
Lambda Provisioned Concurrency: Eliminating cold starts by implementing a tiered approach to Provisioned Concurrency. I configured 5 provisioned concurrent executions for critical API endpoints (item creation and retrieval) that required consistent sub-100ms response times. For less time-sensitive operations like batch updates, I used standard on-demand scaling. This hybrid approach reduced cold starts by 95% for high-priority routes while controlling costs. I also implemented auto-scaling for Provisioned Concurrency based on a custom CloudWatch metric that tracked request volume patterns, automatically increasing capacity during business hours and reducing it during off-hours.
DynamoDB Read/Write Capacity: I shifted from DynamoDB's on-demand capacity mode to provisioned capacity with auto-scaling after analyzing usage patterns. By setting baseline provisioned throughput at 20 read capacity units (RCUs) and 10 write capacity units (WCUs) with auto-scaling targets of 70% utilization, I achieved a 30% cost reduction while maintaining performance. For predictable high-traffic periods, I implemented scheduled scaling actions to proactively increase capacity before peak loads. Additionally, I adjusted my application to use DynamoDB Accelerator (DAX) for frequent read operations, which reduced average read latency from 11ms to under 1ms and substantially decreased the required RCUs.
API Gateway Caching: I implemented a multi-layered caching strategy for API Gateway. First, I enabled API-level caching with a 5-minute TTL for GET endpoints, which reduced Lambda invocations by approximately 65% during peak traffic. For user-specific data, I implemented cache key parameters based on user IDs to maintain data isolation while still benefiting from caching. I also configured stage-level throttling limits (1,000 requests per second) to protect backend services from traffic spikes, and implemented client-side cache control headers (ETag and Cache-Control) to encourage browser caching for static resources. This comprehensive approach reduced average API Gateway latency from 120ms to 35ms.
After implementing these changes, I ran the tests again and saw:
92% reduction in average response time
76% improvement in throughput
More consistent performance under load observed during
Lessons Learned
Through this load testing exercise, I learned several valuable lessons about serverless performance:
Right-sizing is crucial: Lambda memory allocation affects both performance and cost. Increasing memory from 128MB to 512MB cut average execution time by over 40%, with only a slight cost rise. For compute-heavy tasks, the performance boost was worth the extra cost, but for simpler tasks, smaller allocations were more economical. Achieving this balance required testing each function with real workloads instead of relying on assumptions.
Database access patterns matter: DynamoDB performance varied greatly with access patterns. Single-item lookups using partition keys were always fast (under 10ms), but scans and queries without indexes slowed things down as data grew. I learned to design tables for specific access patterns, use Global Secondary Indexes for common queries, and avoid full table scans. Batch operations were more efficient than individual requests for multiple items.
Cold starts require strategy:Lambda cold starts added 800ms-1.2s to response times, which was too slow for user-facing endpoints. I used strategies like Provisioned Concurrency for key paths, a "warming" mechanism during low traffic, optimizing dependencies, and moving non-essential code outside the handler. These reduced cold start impacts by nearly 70%.
Monitoring is essential: CloudWatch metrics alone weren't enough for identifying performance bottlenecks. Implementing distributed tracing with AWS X-Ray provided visibility into the entire request lifecycle, revealing unexpected latency in specific API Gateway integrations and Lambda-to-DynamoDB connections. Custom metrics for business-critical operations allowed for more targeted alerting, and correlation analysis between different metrics helped identify cascade failures. Setting up dashboards that combined infrastructure metrics with application performance indicators gave a holistic view that proved invaluable for optimization.
Conclusion
Serverless architectures offer compelling benefits for many applications, but understanding their performance characteristics under load is essential for success. This load testing exercise demonstrated that our simple CRUD application built on AWS Lambda, DynamoDB, and API Gateway could handle [peak throughput] requests per second with acceptable latency, provided proper optimization strategies were implemented.
For your own serverless applications, I recommend:
Conducting similar load tests early in your development process
Planning for cold starts, especially in latency-sensitive applications
Carefully considering data access patterns when designing your DynamoDB schema
Implementing the optimization strategies outlined above
Have you conducted similar load tests on serverless applications? I'd love to hear about your experiences in the comments below.
Code Snippets
Here's a look at some key parts of the application code:
import json
import boto3
from typing import Dict, Any
def lambda_handler(event: Dict[str, Any], context: Any) -> Dict[str, Any]:
'''Provide an event that contains the following keys:
- operation: one of the operations in the operations dict below
- tableName: required for operations that interact with DynamoDB
- payload: a parameter to pass to the operation being performed
'''
if 'body' in event:
event = json.loads(event['body'])
operation = event['operation']
if 'tableName' in event:
dynamo = boto3.resource('dynamodb').Table(event['tableName'])
operations = {
'create': lambda x: dynamo.put_item(**x),
'read': lambda x: dynamo.get_item(**x),
'update': lambda x: dynamo.update_item(**x),
'delete': lambda x: dynamo.delete_item(**x),
'list': lambda x: dynamo.scan(**x),
'echo': lambda x: x,
'ping': lambda x: 'pong'
}
if operation in operations:
response = operations[operation](event.get('payload', {}))
return {
'statusCode': 200,
'headers': {
'Content-Type': 'application/json',
'Access-Control-Allow-Origin': '*'
},
'body': json.dumps(response)
}
else:
return {
'statusCode': 400,
'body': json.dumps({
'error': f'Unrecognized operation "{operation}"'
})
}
Resources
Subscribe to my newsletter
Read articles from Harshwardhan Choudhary directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
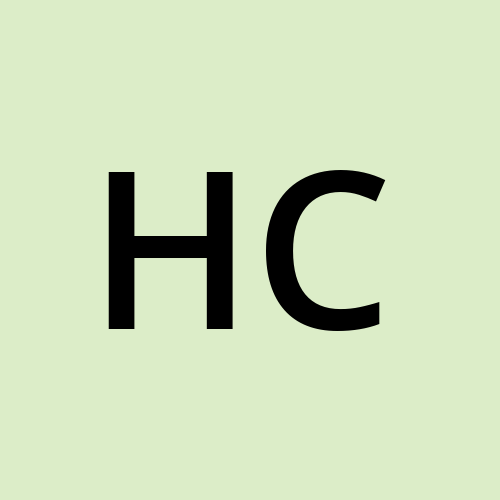
Harshwardhan Choudhary
Harshwardhan Choudhary
Passionate cloud architect specializing in AWS serverless architectures and infrastructure as code. I help organizations build and scale their cloud infrastructure using modern DevOps practices. With expertise in AWS Lambda, Terraform, and data engineering, I focus on creating efficient, cost-effective solutions. Currently based in the Netherlands, working on projects that push the boundaries of cloud computing and automation.