Comprehensive Guide to Angular Signals: Reactive State Management Made Simple

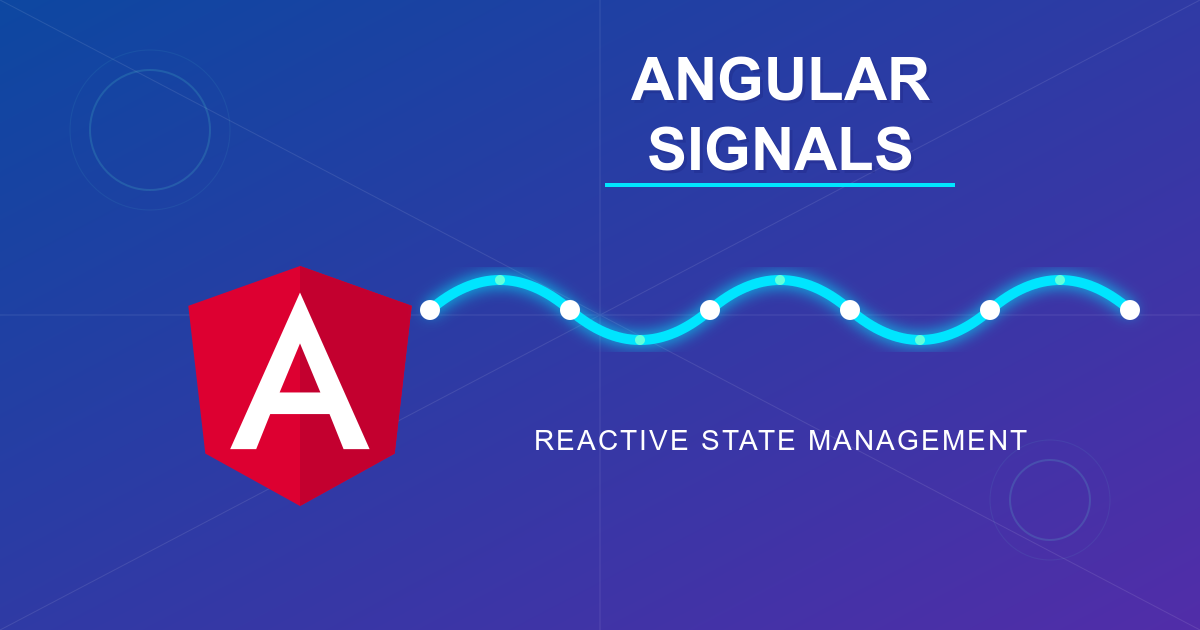
Introduction
What Are Angular Signals?
Angular Signals are a revolutionary reactivity primitive introduced in Angular v16. They enable granular tracking of state changes, allowing the framework to efficiently update only the parts of your application affected by those changes. Consider signals as "smart variables" that automatically notify dependent components, templates, or services when value changes—like a network of walkie-talkies broadcasting updates to subscribed listeners.
Role in Angular’s Reactivity Model
Signals replace coarse-grained change detection (e.g., zone.js
-driven checks) with a targeted approach. Instead of checking the entire component tree, Angular updates only what’s necessary when a signal changes.
Key Benefits Over Traditional Change Detection
🎯 Granular Updates: No unnecessary re-renders.
⚡ Performance: Reduced overhead in large apps.
📊 Explicit Dependencies: Clear tracking of state relationships.
Analogy: Imagine a spreadsheet cell. When one cell (signal) changes, only dependent cells (computed values, effects) recalculate automatically.
Core Concepts
1. Signal Creation (signal()
)
Create reactive values with signal(initialValue)
:
typescript
Copy
import { signal } from '@angular/core';
// Create a writable signal
const count = signal(0);
// Update value
count.set(5);
count.update((value) => value + 1);
2. Computed Signals (computed()
)
Derive reactive values from other signals:
typescript
Copy
const doubleCount = computed(() => count() * 2);
3. Effects (effect()
)
React to signal changes (e.g., logging, side effects):
typescript
Copy
effect(() => {
console.log(`Count is now: ${count()}`);
});
4. Signal Dependencies Graph
A text-based diagram showing relationships:
Copy
count (source signal)
│
└─ doubleCount (computed signal)
│
└─ effect (logging)
Lifecycle & Best Practices
Signal Update Lifecycle
Signal Change:
count.set(10)
Mark Dependencies: Angular detects
doubleCount
depends oncount
.Notify Consumers: Update
doubleCount
and trigger effects.
Text-Based Flowchart:
Copy
Signal Updated → Notify Dependencies → Recompute Computed Values → Run Effects
Signals vs RxJS Observables
Use Signals: For granular state management (e.g., UI state).
Use Observables: For event streams (e.g., HTTP responses, user events).
Interoperate with toSignal()
and toObservable()
:
typescript
Copy
const count$ = toObservable(count); // Signal → Observable
const dataSignal = toSignal(data$); // Observable → Signal
Common Pitfalls
🚫 Avoid modifying signals inside computed values.
🚫 Avoid infinite loops (e.g., updating a signal inside its own effect).
Performance Tips:
Use
untracked()
to read signals without creating dependencies.Limit expensive computations in
computed()
.
Real-World Example: Todo List Component
State Management with Signals
typescript
Copy
// todo.component.ts
import { signal, computed } from '@angular/core';
export class TodoComponent {
todos = signal<Todo[]>([]);
// Computed derived state
unfinishedCount = computed(() =>
this.todos().filter(todo => !todo.completed).length
);
addTodo(title: string) {
this.todos.update(todos => [...todos, { title, completed: false }]);
}
toggleTodo(index: number) {
this.todos.update(todos =>
todos.map((todo, i) =>
i === index ? { ...todo, completed: !todo.completed } : todo
)
);
}
}
Template Integration
<!-- todo.component.html -->
<div>
<input #newTodo>
<button (click)="addTodo(newTodo.value)">Add</button>
</div>
<div *ngFor="let todo of todos(); index as i">
<input type="checkbox" [checked]="todo.completed" (change)="toggleTodo(i)">
{{ todo.title }}
</div>
<!-- Display computed state -->
<p>{{ unfinishedCount() }} items remaining</p>
Advanced Patterns
Signal Composition
Combine multiple signals:
const user = signal({ name: 'Alice', age: 30 });
const isAdult = computed(() => user().age >= 18);
Zone.js Integration
Opt out of automatic change detection with ngZone: 'noop'
:
bootstrapApplication(AppComponent, { providers: [
provideZoneChangeDetection({ ngZone: 'noop' })
]});
Visual Aids
Signal Propagation Diagram
[Source Signal]
→ [Computed Signal]
→ [Effect]
→ [Template Binding]
Signals vs Traditional Change Detection
Feature | Signals | Traditional (zone.js) |
Granularity | Per-signal updates | Full tree check |
Performance | Optimized | Overhead in large apps |
Dependency Tracking | Automatic | Manual (async/await) |
Practical Tips
Start Small: Refactor localized state (e.g., form controls) first.
Combine with RxJS: Use signals for UI state and observables for async operations.
Avoid Overuse: Not all state needs to be reactive!
Conclusion
Angular Signals simplify reactivity with a declarative, performant approach. Use them for:
Complex state management
Performance-critical UIs
Fine-grained reactivity in components
By embracing signals, you’ll write cleaner, more efficient Angular code. 🚀
Subscribe to my newsletter
Read articles from Zakir Hussain Parrey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Zakir Hussain Parrey
Zakir Hussain Parrey
Driven by a passion for tackling complex challenges, I excel in high-pressure environments that demand rapid learning and adaptation. My goal is to join a forward-thinking company where I can apply my robust problem-solving skills and continue to grow professionally. I bring a diverse skill set, including proficiency in JavaScript, HTML5, CSS3, Bootstrap, React.js, Angular, and more, complemented by a strong foundation in domain-driven design, responsive principles, website optimization, and digital marketing. I am eager to further expand my programming expertise and contribute to innovative solutions.