Creating a Basic HTTP Server with Node.js
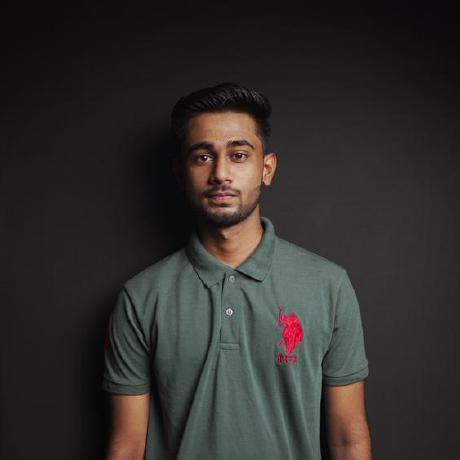

When I first started working with Node.js, one of the most exciting things I learned was how to create a simple HTTP server.
It felt like magic just a few lines of code, and I had a server responding to requests!
If you’re just starting out, this guide will walk you through the basics of setting up an HTTP server, handling different request types, working with request and response objects and sending responses.
Setting Up the Server
Node.js provides a built-in http
module that makes it easy to create an HTTP server. First, ensure you have Node.js installed, then create a new JavaScript file (e.g server.js
) and add the following code:
const http = require('http');
const server = http.createServer((req, res) => {
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end('Hello, World!');
});
const PORT = 3000;
server.listen(PORT, () => {
console.log(`Server running at http://localhost:${PORT}/`);
});
Explanation:
We import the
http
module.http.createServer
creates an HTTP server that listens for requests.The callback function receives two objects:
req
(request) andres
(response).We set the response header with
res.writeHead(200, { 'Content-Type': 'text/plain' });
.We end the response with
res.end('Hello, World!');
.The server listens on port 3000.
Run this script using:
node server.js
Visit http://localhost:3000/
in your browser and you should see "Hello, World!".
QR Code Suyash Chandrakar
Handling Different HTTP Methods (Routing)
A basic server should be able to handle multiple HTTP methods like GET, POST, PUT and DELETE.
Let’s modify our server to add simple routing:
const http = require('http');
const server = http.createServer((req, res) => {
res.setHeader('Content-Type', 'application/json');
if (req.method === 'GET' && req.url === '/') {
res.writeHead(200);
res.end(JSON.stringify({ message: 'Welcome to the homepage!' }));
} else if (req.method === 'POST' && req.url === '/data') {
let body = '';
req.on('data', chunk => {
body += chunk.toString();
});
req.on('end', () => {
res.writeHead(201);
res.end(JSON.stringify({ message: 'Data received', data: JSON.parse(body) }));
});
} else if (req.method === 'PUT' && req.url === '/update') {
res.writeHead(200);
res.end(JSON.stringify({ message: 'Resource updated successfully!' }));
} else if (req.method === 'DELETE' && req.url === '/delete') {
res.writeHead(200);
res.end(JSON.stringify({ message: 'Resource deleted successfully!' }));
} else {
res.writeHead(404);
res.end(JSON.stringify({ message: 'Not Found' }));
}
});
const PORT = 3000;
server.listen(PORT, () => {
console.log(`Server running at http://localhost:${PORT}/`);
});
Explanation:
We check
req.method
andreq.url
to determine the type of request.GET /
returns a welcome message.POST /data
accepts JSON data and responds with it.PUT /update
andDELETE /delete
send success messages.If the route doesn’t match, we return a
404 Not Found
response.
Working with Request and Response Objects
The req
(request) and res
(response) objects are central to handling HTTP requests.
Let's break them down:
The Request Object (req
)
req.method
: Returns the HTTP method (e.g GET, POST, etc.).req.url
: Returns the requested URL.req.headers
: Returns an object containing request headers.req.on('data')
: Listens for incoming data chunks (useful for POST requests).req.on('end')
: Signals the end of the request body.
The Response Object (res
)
res.writeHead(statusCode, headers)
: Sets the status code and response headers.res.write(data)
: Writes data to the response body.res.end()
: Ends the response.
Example of handling headers and request details:
const server = http.createServer((req, res) => {
console.log(`Received ${req.method} request for ${req.url}`);
console.log('Headers:', req.headers);
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end('Request details logged!');
});
Sending and Receiving Data
To test the POST
request, use a tool like Postman or curl
:
curl -X POST http://localhost:3000/data -H "Content-Type: application/json" -d '{"name": "John"}'
The server will respond with:
{"message":"Data received","data":{"name":"John"}}
Similarly, you can send PUT
and DELETE
requests:
curl -X PUT http://localhost:3000/update
curl -X DELETE http://localhost:3000/delete
Error Handling and Server Improvements
To improve our server, we can add error handling:
server.on('error', (err) => {
console.error('Server error:', err);
});
We can also use environment variables for port configuration:
const PORT = process.env.PORT || 3000;
Conclusion
Creating an HTTP server in Node.js is a great way to understand how web servers work.
With just a few lines of code, you can set up routing, handle different HTTP methods and send/receive data.
As you continue learning, you can explore frameworks like Express.js to simplify server development.
Subscribe to my newsletter
Read articles from Suyash Chandrakar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
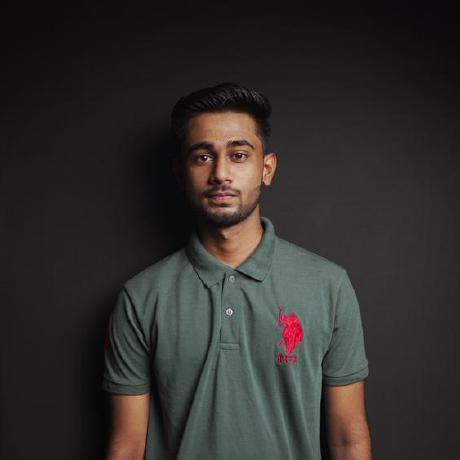