Redis Caching in Spring Boot: Boosting Performance
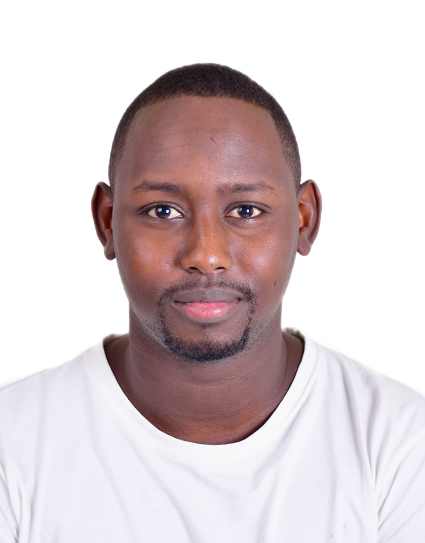

1. Introduction
Modern web applications require high performance and low latency, especially when dealing with frequent database queries. Caching is a powerful technique to improve response times and reduce database load. One of the most efficient caching solutions is Redis.
In this article, we will explore how to integrate Redis caching in a Spring Boot application and implement a GET endpoint that returns cached data.
2. What is Redis?
Redis (Remote Dictionary Server) is an open-source, in-memory data store used as a cache, message broker, or database. It is designed for ultra-fast data access with sub-millisecond latency.
Why Redis for Caching?
Blazing fast: Data is stored in-memory, making reads/writes extremely fast.
Scalable: Supports replication and clustering for distributed applications.
Flexible data structures: Supports strings, lists, hashes, and more.
Persistence options: Can persist data to disk if needed.
By integrating Redis caching, Spring Boot applications can serve frequent requests much faster, improving overall performance.
3. Example: Spring Boot GET Endpoint with Redis Caching
Let's implement a simple GET endpoint that fetches user details, with Redis caching enabled.
Step 1: Add Dependencies
Include the required dependencies in pom.xml
:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-cache</artifactId>
</dependency>
spring.cache.type=redis
spring.redis.host=localhost
spring.redis.port=6379
If Redis is not installed, start it using Docker:
docker run --name redis -p 6379:6379 -d redis
In the main application class, enable caching:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cache.annotation.EnableCaching;
@SpringBootApplication
@EnableCaching
public class RedisCachingApplication {
public static void main(String[] args) {
SpringApplication.run(RedisCachingApplication.class, args);
}
}
We use @Cacheable
to store the fetched user data in Redis.
import org.springframework.cache.annotation.Cacheable;
import org.springframework.stereotype.Service;
@Service
public class UserService {
@Cacheable(value = "users", key = "#userId")
public String getUserById(Long userId) {
simulateSlowDatabase();
return "User-" + userId;
}
private void simulateSlowDatabase() {
try {
Thread.sleep(3000); // Simulate a slow DB query
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
}
@Cacheable(value = "users", key = "#userId")
→ Caches the result under"users"
withuserId
as the key.First request takes 3 seconds, but subsequent calls return instantly from Redis.
import org.springframework.web.bind.annotation.*;
@RestController
@RequestMapping("/users")
public class UserController {
private final UserService userService;
public UserController(UserService userService) {
this.userService = userService;
}
@GetMapping("/{id}")
public String getUser(@PathVariable Long id) {
return userService.getUserById(id);
}
}
Run the application and test with curl
or Postman:
# First request (takes 3 seconds)
curl http://localhost:8080/users/1
# Second request (instant response from cache)
curl http://localhost:8080/users/1
4. Benefits of Redis Caching
Using Redis for caching provides multiple advantages:
Faster Response Times – Reduces database calls, making requests much quicker.
Reduces Load on Database – Frequently requested data is served from cache instead of hitting the DB.
Scalability – Handles high traffic efficiently without slowing down.
Persistence Options – Can store data temporarily or persist it based on use case.
5. Conclusion
Redis caching in Spring Boot is an excellent way to optimize performance and minimize database load. With just a few configurations, we can integrate fast and efficient caching into our REST APIs.
By implementing @Cacheable, we significantly reduce response times for frequently accessed data. If you're building high-performance Spring Boot applications, Redis is a must-have for caching!
Subscribe to my newsletter
Read articles from Muhire Josué directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
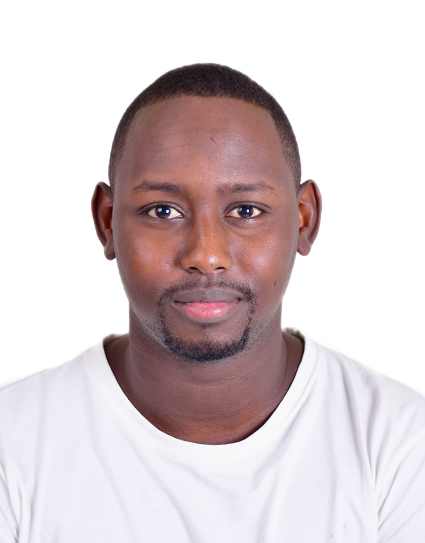
Muhire Josué
Muhire Josué
I am a backend developer, interested in writing about backend engineering, DevOps and tooling.