How to Select an Option from a React-Select Dropdown Using Playwright

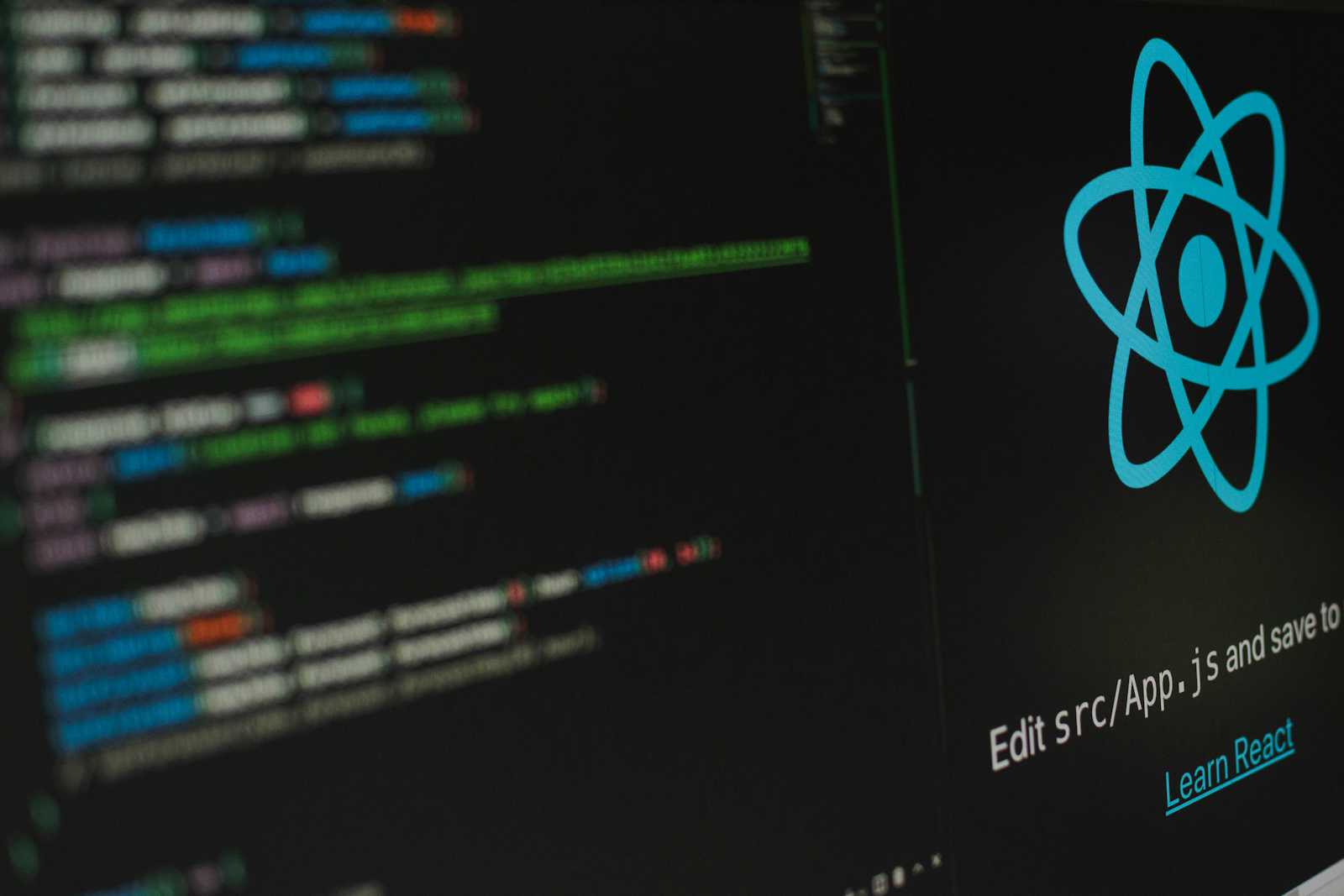
Introduction
Testing dropdowns in Playwright is straightforward if you’ve worked with standard HTML <select>
elements. However, React-Select, a popular dropdown library, behaves differently because it doesn’t use native <select>
elements. Instead, it creates a custom dropdown with divs and spans, requiring a different approach.
In this guide, we’ll focus on:
Advantages of using React-Select.
Understanding how React-Select differs from standard dropdowns.
Selecting an option from a React-Select dropdown when you already know the option.
Selecting the first available option dynamically.
Advantages of React-Select
React-Select offers several advantages over standard dropdowns:
Searchable Dropdowns: Users can type to filter options.
Asynchronous Data Loading: Options can be fetched dynamically.
Custom Styling: Supports theming and styling customization.
Multi-Select & Tagging: Allows selecting multiple options.
These features make React-Select a powerful alternative, but they also require different testing techniques, as shown above.
How React-Select Differs from Standard Dropdowns
Unlike a traditional HTML <select>
element, React-Select:
Uses
<div>
elements instead of<option>
and<select>
tags.Requires interactions through clicks instead of
select
events.Supports advanced features like async loading, search, and multi-select.
Because of these differences, selecting an option requires Playwright locators targeting the right elements.
Selecting a Specific Option
If you know the exact option to select, use the following approach:
import { test, expect } from '@playwright/test';
test('Select a specific option from React-Select dropdown', async ({ page }) => {
// Navigate to page with dropdown
await page.goto('https://example.com');
// Open the dropdown
await page.click('.react-select__control');
// Select an option by text
const optionText = 'Option 1';
//select the option matching the text
await page.getByRole("option", { name: optionText, exact: true }).click();
// Verify selection
await expect(page.locator('.react-select__single-value')).toHaveText(optionToSelect);
});
This ensures:
The dropdown opens before selecting an option.
The specified option is clicked.
Selection is verified after the interaction.
Selecting the First Available Option
If the option to select isn’t predefined, you may need to pick the first available option dynamically:
import { test, expect } from '@playwright/test';
test('Select the first available option from React-Select dropdown', async ({ page }) => {
//Navigate to page with dropdown
await page.goto('https://example.com');
// Open the dropdown
await page.click('.react-select__control');
// Select the first option
await page.locator("div[id^='react-select'] div[role='option']").first().click();
});
The above code ensures the dropdown is opened and the first option is selected. You do not have to know which value it is.
If you’re used to testing standard dropdowns, React-Select may initially seem confusing due to its custom implementation. However, Playwright provides powerful tools to:
Select a known option by text.
Dynamically pick the first available option.
Adapt to React-Select’s unique structure.
By understanding these differences, you can write more reliable tests for applications using React-Select!
🔗 Further Reading
Subscribe to my newsletter
Read articles from Mayimuna Kizza Lugonvu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Mayimuna Kizza Lugonvu
Mayimuna Kizza Lugonvu
Hi, I’m Mayimuna, but you can call me Muna. I am a Software Engineer from Uganda with a passion for solving real-world problems through code, creativity, and storytelling. I've started my writing journey, and I hope to write about systems design, development workflows, what it’s like building tech in emerging markets, and everything software-related. Currently, I’m exploring cloud-native technologies, digital empowerment in agriculture, and AI. My other interests include digital marketing and language learning (안녕하세요 — I’m learning Korean!).