Building a Drag-and-Drop Image Uploader with JavaScript
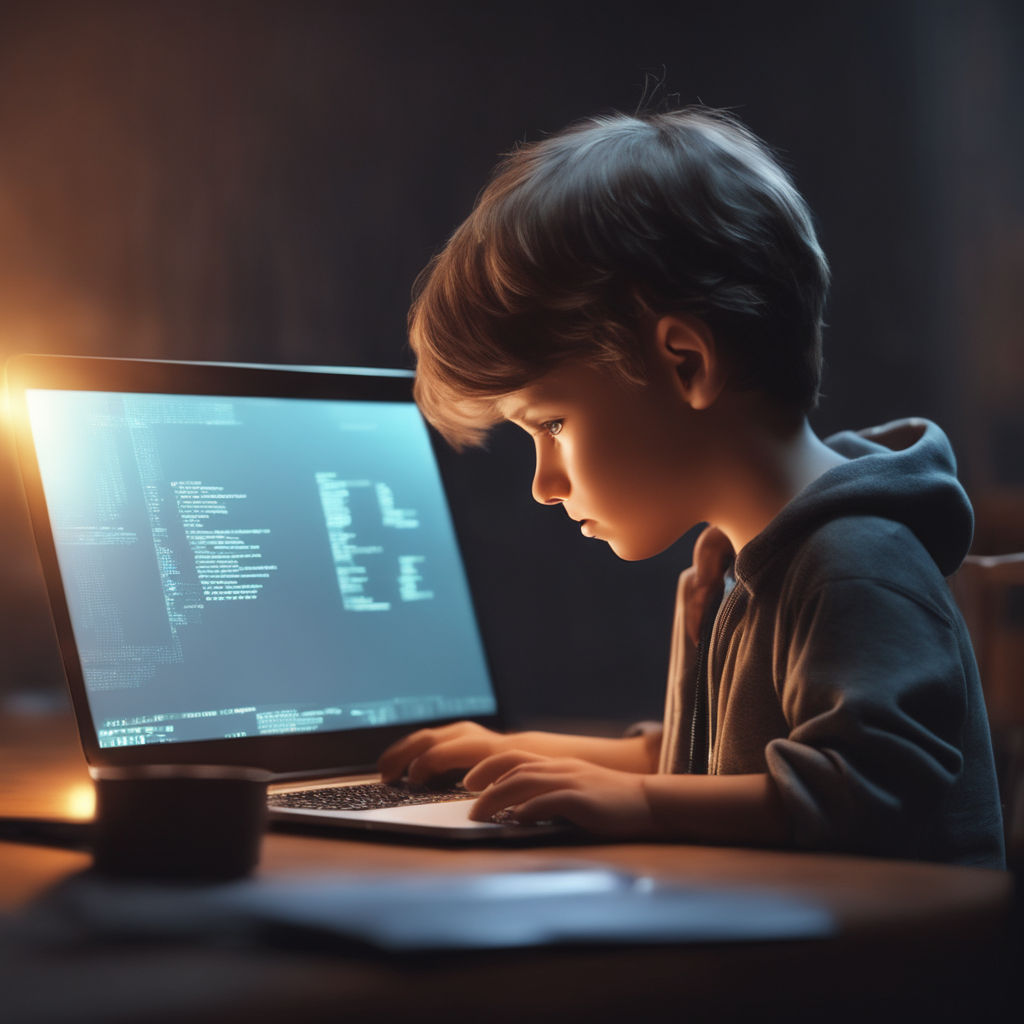
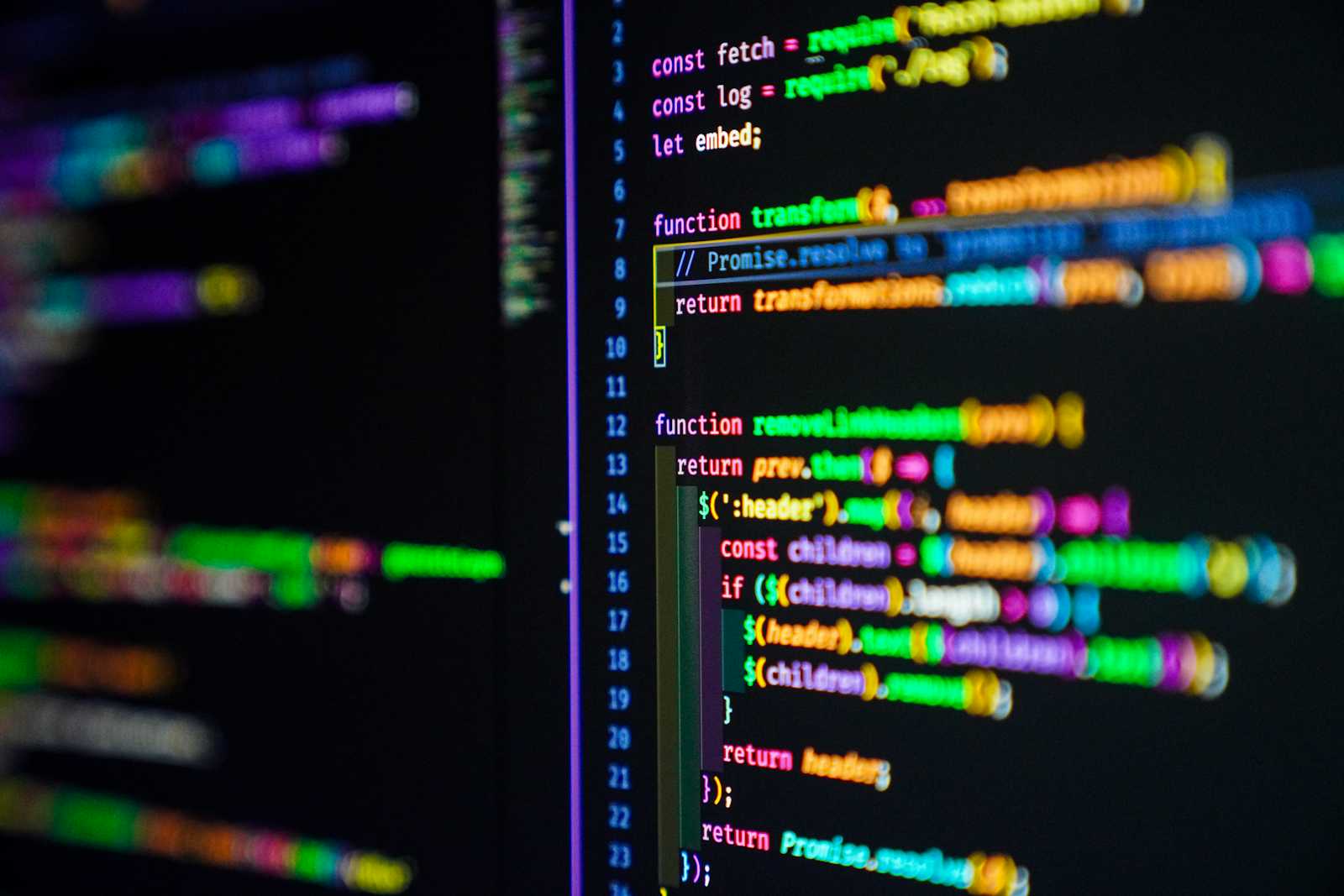
Creating an intuitive image uploader that supports both clicking to select files and drag-and-drop functionality is an excellent way to enhance user experience on your website. In this tutorial, we’ll break down a practical example where you can drag images into designated areas to preview them before any upload process begins. We’ll explore how the code handles drag events, file validations, and image previewing using the FileReader API.
Overview
The project consists of three main parts:
JavaScript – Handles drag-and-drop events, file validations, and image previews.
HTML – Defines the drag-and-drop areas, a file input for fallback, and containers for displaying images.
CSS – Provides basic styling to visually differentiate the upload zones and highlight drag interactions.
Let’s take a closer look at the implementation.
HTML Structure
The HTML file organizes the drag-and-drop zones and the file input:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="./src/style.css" />
<title>Drag and Drop</title>
</head>
<body>
<div>
<h1 style="text-align: center;">Drag and Drop</h1>
<div class="drag-upload">
<div class="drag-area green" id="img-show">
<label for="file-upload" id="upload-label">
<span>Drag & Drop Images</span>
<span>Or</span>
<span>Click to browse Images...🖼️</span>
<input type="file" id="file-upload" accept="image/*" multiple />
</label>
</div>
</div>
<div class="container">
<div class="drag-area orange">Item Drop Area 2</div>
<div class="drag-area red">Item Drop Area 3</div>
<div class="drag-area blue">Item Drop Area 4</div>
</div>
</div>
<script type="module" src="/src/main.js"></script>
</body>
</html>
Key points:
The main drag area (with class
green
) hosts both the file input and the image display container.Additional drop zones (orange, red, blue) allow you to move the previewed images between different areas.
CSS Styling
The accompanying CSS styles ensure a clean, modern interface:
body {
display: flex;
align-items: center;
justify-content: center;
flex-direction: column;
background-color: #181616d0;
}
.container {
display: flex;
max-width: 1240px;
margin: auto;
align-items: center;
padding: 0 10px;
gap: 10px;
flex-wrap: wrap;
}
.drag-area {
width: 200px;
min-height: 250px;
padding: 10px;
border: 1px solid rgba(181, 170, 170, 0.338);
border-radius: 10px;
box-shadow: 5px 0px 18px rgba(64, 64, 64, 0.573);
display: flex;
}
.green {
background-color: rgba(255, 255, 255, 0.911);
position: relative;
min-width: 250px;
font-size: 18px;
}
.green input[type="file"] {
width: 100%;
visibility: hidden;
}
.green label {
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
border: dashed;
}
.currentBox {
background-color: rgba(102, 102, 102, 0.744);
transition: background-color 0.2s ease-in-out;
}
.drag-img {
max-width: 100px;
object-fit: contain;
}
The styles:
Create a flexible layout for multiple drop zones.
Hide the native file input, while its label serves as a clickable area.
Highlight drop areas during drag events with the
.currentBox
class.
JavaScript: Handling Drag Events and File Reading
The code begins by selecting the key elements from the DOM:
const draggableArea = document.querySelectorAll(".drag-area");
const imageShow = document.querySelector("#img-show");
const dragUploadFile = document.getElementById("file-upload");
const label_Onupload = document.getElementById("upload-label");
// A variable to track the element currently being dragged.
let isDragged;
File Input Change Event
When the user selects files via the file input, an event listener validates the number of files and triggers image display:
dragUploadFile.addEventListener("change", (e) => {
if (e.target.files.length > 3) {
label_Onupload.style.display = "flex";
alert("upload not more than 3 images...");
} else if (e.target.files.length <= 3) {
displayMultipleImages(e.target.files);
label_Onupload.style.display = "none";
return;
}
});
This check prevents uploading more than three images. If the count is acceptable, the displayMultipleImages
function processes each file.
Drag and Drop Event Listeners
Each element with the class .drag-area
receives listeners for several drag events:
draggableArea.forEach((area) => {
area.addEventListener("dragover", onDragOver);
area.addEventListener("dragenter", onDragEnter);
area.addEventListener("dragleave", onDragLeave);
area.addEventListener("drop", onDragDrop);
area.addEventListener("dragend", onDragEnd);
});
The purpose of these events is to:
onDragOver: Prevent default behavior and indicate that the element is a valid drop target.
onDragEnter/onDragLeave: Visually highlight the drop area.
onDragDrop: Handle dropped files, display images, and if dragging an existing image, move it to a new area.
onDragEnd: Finalize the drag operation and update the UI accordingly.
Here’s an example of the onDragOver
function:
function onDragOver(e) {
e.stopPropagation();
e.preventDefault();
e.dataTransfer.dropEffect = "move";
e.target.classList.add("currentBox");
}
By calling preventDefault()
and adding a CSS class (here, currentBox
), the code ensures that the browser doesn’t execute its default file handling (like opening the image) and gives visual feedback to the user.
Dropped Files Processing
When a drop event occurs, the onDragDrop
function runs:
function onDragDrop(e) {
e.preventDefault();
e.target.classList.remove("currentBox");
const dropTarget = e.target.closest(".drag-area");
if (!dropTarget) return;
for (let file of e.dataTransfer.files) {
if (file.type === "image/*") {
displayMultipleImages(file);
}
if (isDragged) {
if (dropTarget !== isDragged.parentElement) {
dropTarget.appendChild(isDragged);
}
}
}
}
The function:
Prevents default behavior.
Checks that the drop target is a valid drag area.
Iterates over the dropped files; if the file is an image, it calls
displayMultipleImages
to create a preview.If an element is being dragged (for example, if the user is reordering images), it moves the dragged element to the new drop area.
Reading Files and Displaying Images
The displayMultipleImages
function uses the FileReader API to create a data URL for each image:
function displayMultipleImages(files) {
for (let file of files) {
let reader = new FileReader();
reader.readAsDataURL(file);
reader.onload = () => {
let image = new Image();
image.src = reader.result;
image.style.display = "block";
image.className = "drag-img";
image.setAttribute("draggable", "true");
image.addEventListener("dragstart", function (e) {
e.dataTransfer.setData("text/plain", image.src);
isDragged = e.target;
});
imageShow.appendChild(image);
};
}
}
Here’s what happens step by step:
A new FileReader object reads the file as a Data URL.
Once loaded, an image element is created, its
src
set to the result, and styled accordingly.The image is made draggable by setting the
draggable
attribute.An event listener is added for
dragstart
to track which image is being moved.Finally, the image is appended to the
#img-show
container, making it visible to the user.
This drag-and-drop uploader demonstrates how to combine native browser events with the FileReader API to create a user-friendly interface. By monitoring drag events and leveraging dynamic file reading, you can offer a seamless experience—allowing users to preview images immediately before any server upload occurs.
Whether you plan to extend this functionality with real-time upload progress or integrate it into a larger application, this example serves as a strong foundation for building modern web interfaces. Happy coding!
Subscribe to my newsletter
Read articles from Ronak Mathur directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
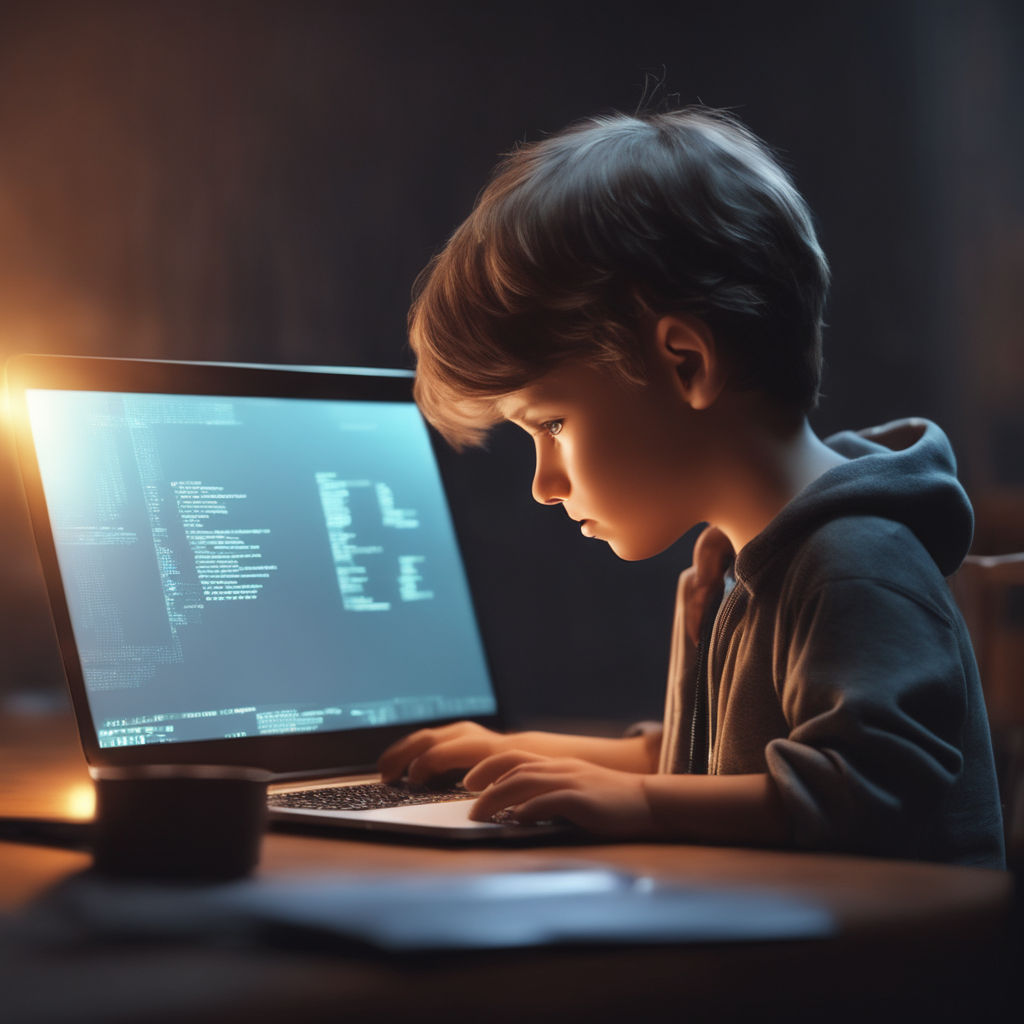