TypeScript's Edge in Object-Oriented Programming Over JavaScript
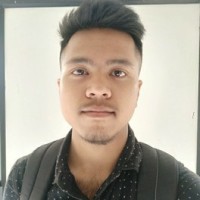
Table of contents
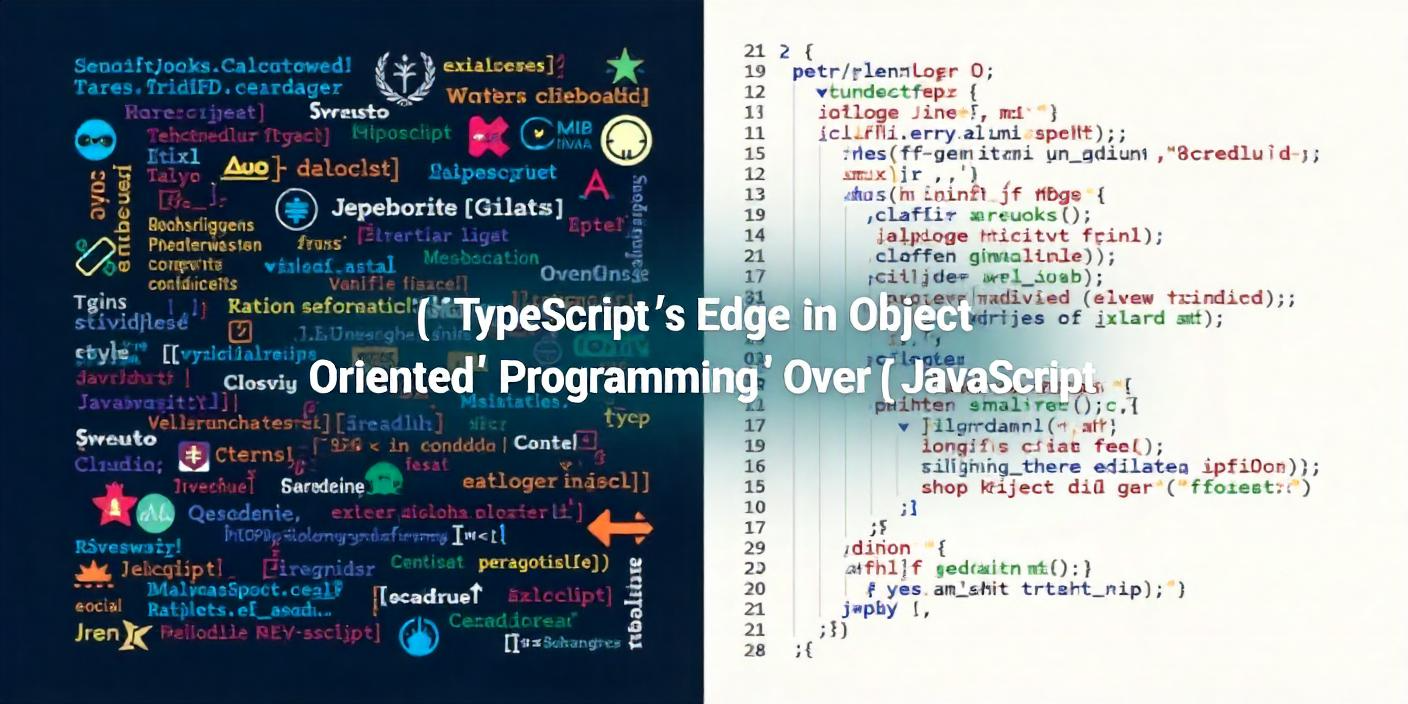
Javascript provides classes, objects, inheritance so does typescript. So how exactly typescript does it better than Javascript in context of OOP?
Lets look into the below table for a more clear understanding of the features that enhances OOPs in JS vs TS
Feature | Javascript | Typescript |
Classes | ✅ | Enhances it through types |
Inheritance | ✅ | Enhances it through type checking |
Interfaces | ❌ | Pure Typescript Feature |
Access Modifiers | ‘Private’ ✅, ‘protected’ ❌ | Provides ‘Private’ and ‘Protected’ access modifiers |
Static Members | ✅ | Adds type checking |
Abstract Classes | ❌ | Availble only in typescript |
Generics | ❌ | Availble in Typescript |
Getters & Setters | ✅ | Enhaced with type safety |
Method Overriding | ✅ | Enforced through type checks |
Encapsulation | ✅ | ✅ |
Typescript with interfaces, abstract classses, access modifiers and generics provides a better ecosystem for object oriented programming than Javascript.
Lets look into some of the features Typescript provides briefly:
Interfaces
There is no built in interface in Javascript, Typescipt we can use interface as follow:
Interface Bike{
model: string
price: number
}
const introduceBike =(bike:Bike)=> {
return console.log(`The ${bike.model} comes at a price of ${bike.price}.`)
}
introduceBike({model:'CB350', price: 350000}) //The CB350 comes at a price of 350000.
introduceBike({model:'GS310'}) // type error
Access Modifiers
In ES2020, private fields are introduced in Javascript we can use them using ‘#’.
Unlike TS, it also enforces in the runtime as well. In TS, these modifiers gets removed during runtime.
class Person {
#name; // Private field
constructor(name) {
this.#name = name;
}
getName() {
return this.#name; // Allowed inside the class
}
}
const person = new Person("John Cena"); // just imagine him cause you cant see him
console.log(person.getName()); // "John Cena"
console.log(person.#name); // SyntaxError: Private field is not accessible
Typescript provides ‘private’ and ‘protected’ access modifiers , which are enforced in compile time and removed in run time.
class Animal {
protected name: string; // Protected field
constructor(name: string) {
this.name = name;
}
}
class Dog extends Animal {
bark() {
console.log(`${this.name} bhow bhow.`); // Accessible in subclass
}
}
const dog = new Dog("Tommy");
dog.bark(); // Tommy bhow bhow.
console.log(dog.name); // Error: Property 'name' is protected
Abstract Classes
Abstract classes are those classes which cannot be instantiated. It can have abstract methods and have implemented methods. These abstract classes can be used as a base class for inheritance.
A small example of abstract class in typescript:
abstract class Vehicle {
abstract start(): void;
}
// abstract methods are implemented in subclasses
class Car extends Vehicle {
start(): void {
console.log("Car started");
}
}
const v = new Vehicle(); //Cannot instantiate abstract class
Generics
With better Type Safety , typescript provides generics, which is again not available in Javascript.
An example of using generics in Typescript:
Class Box<T>{
content: T
constructor(content:T){
this.content=content
}
}
const numBox = new Box<number>(10) // works nicely
const stringBox= new Box<string>("sadfsdfsdf") // works nicely
Although Javascript is trying to provide a robust system for writing OOP, they have been recently pushing updates in ES2020 and ES2021 , some examples being true private field and method using “#” and adding static fields, Javascript still lags behind Typescript in enforcing OOP.
Some of the reasons along with missing out on above features are :
Typescript catches OOP related mistakes earlly on during compile time
Typescript also prevented unintended mutations of data
Typescript provides better readablity and maintainibilty with interfaces and generics
Typescript lacks true ‘protected’ members
Typescript also allows strict OOP principle unlike Javascript which is too much flexible and might lead to bad design pattern.
In conclusion, Typscript is just not a tool for type safetly it enforces strict OOP principles which Javascript follows very loosely.
Subscribe to my newsletter
Read articles from Rohan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
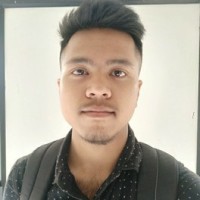
Rohan
Rohan
I am a developer from a small village in North-East India. I am passionate about technology and have a very keen interest in cognition and human-computer interaction. Besides technologies, I love to read, play a lot of sports and am very passionate about offroading.