Exploring How Everything is an Object in JavaScript

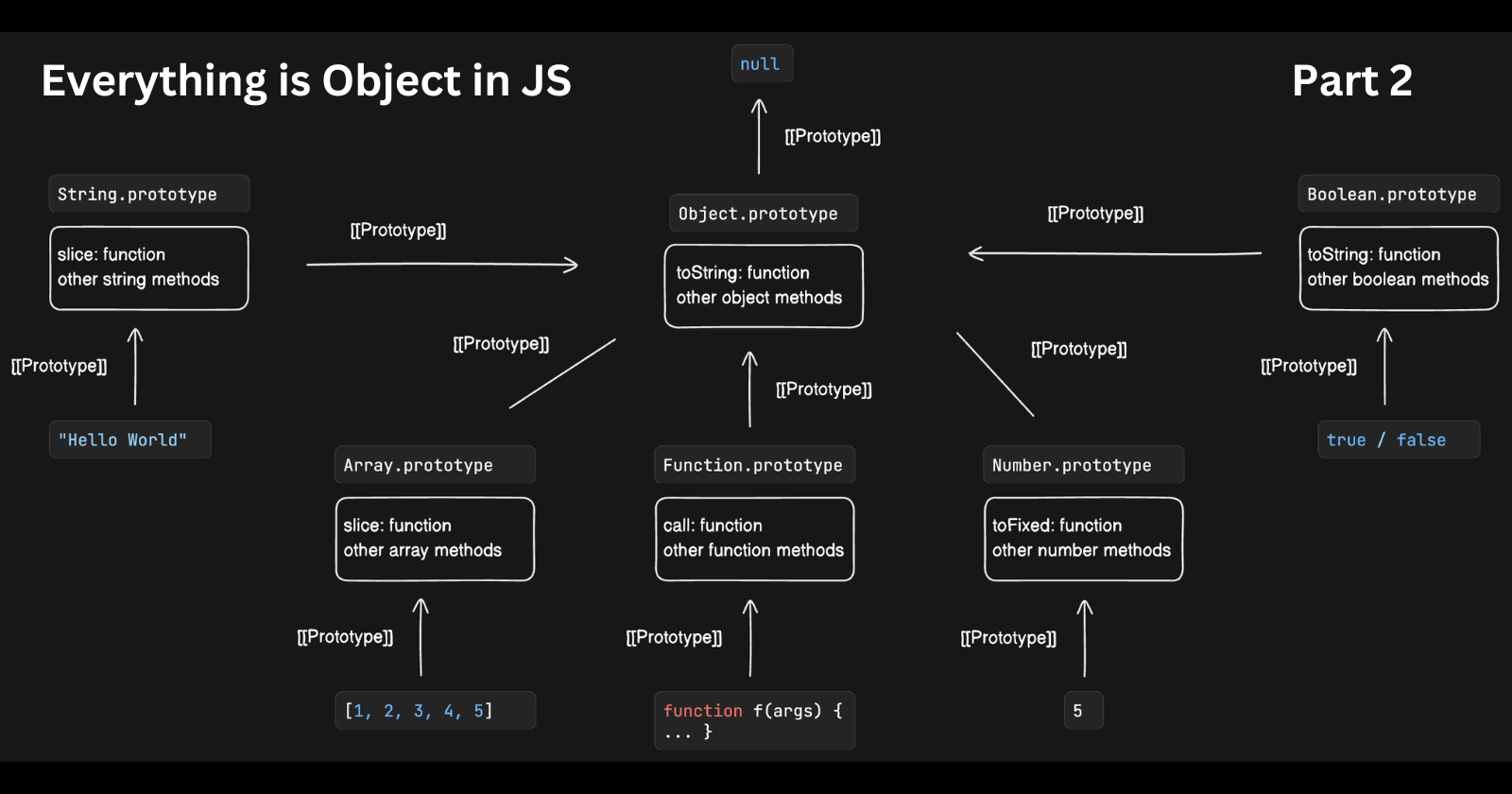
You can read about Part 1 of this article here, which explains about the Prototype mechanism in JS.
Before understanding, How everything is object in JavaScript. We must first understand how many types of data types are there in JavaScript. JavaScript have two types of data types Primitives and Non-Primitive data types.
The Primitive data types are further classified into:
String
Number
Boolean
null
undefined
BigInt
Symbol
The Non-Primitive data types is classified into:
- Objects (like Array, Functions)
Here, an important point to note that in JavaScript Non-Primitives data types are also known as Objects means we use Object name to reference to Non-Primitives data types.
Wrapper Object
The Primitive data types in JavaScript are immutable (that they cannot be changed or there value cannot be changed we can only assign a new value to it) and these are the types that you most regularly interact with but there is one problem that they are values and we cannot access any method on them like we do in the code. For example
const str = "Hello World"
console.log(str.length) // 11
console.log(str.indexOf("l")) // 2
It is also true for other primitive values.
But in the code we are able to access all the properties and methods because the every primitive value except null
and undefined
have a corresponding wrapper object and these objects have methods and properties defined on them while the primitives do not have these properties, but the primitives appear to have methods because JavaScript silently creates a wrapper object when code attempts to access any property of a primitive.
For example, consider the above code only:
const str = "Hello World"
console.log(str.length) // 11
console.log(str.indexOf("l")) // 2
Behind the scene JavaScript perform the following operations:
Create a wrapper
String
object fromstr
, which is equivalent to usingnew String(str)
.const str = "Hello World" It is equivalent to const str = new String("Hello World")
Access the
length
property andindexOf
method from theString
object and computes there value.Access this property from the String object. String {'', anchor: ƒ, at: ƒ, big: ƒ, blink: ƒ, indexOf: f, …}
It now destroy or discarded this
String
object that it had created in the step 1.Return the values calculated in step 2.
Because of these above operations it looks like the primitive values have the property and methods defined on them but it is the wrapper object of the primitive types that these properties and method defined on them. JavaScript internally creates a wrapper object for us so that we can access that property or method and JS delete this wrapper object after it is used and return it’s value. This thing in JavaScript is called as Autoboxing.
And Autoboxing is just same that it is the process where primitive values (like string
, number
, boolean
) are temporarily converted into objects when accessing properties or calling methods on them. This happens automatically behind the scenes.
The Numbers
, Boolean
, BigInt
and Symbol
they all have wrapper object defined on them but null
and undefined
does not have a wrapper object defined on them and it is because of this reason that we cannot access any property and method on null
and undefined
data types.
console.log(null.toString())
// Uncaught TypeError: Cannot read properties of null (reading 'toString')
console.log(undefined.toString())
// Uncaught TypeError: Cannot read properties of undefined (reading 'toString')
Functions are Objects
We already know that Array
and Objects
are objects in JavaScript but to your surprise Functions
are also objects in JavaScript.
For example consider this code:
In this code we are assigning a functions an variable.
function sayHello() {
return "Hello World"
}
sayHello.hello = "I am saying hello";
console.log(sayHello.hello) // I am saying hello
// This sayHello details is only accessible in Firefox browser. For this write sayHello in console
function sayHello()
arguments: null
caller: null
hello: "I am saying hello" // Your define property
length: 0
name: "sayHello"
prototype: Object { … } // .prototype property useful when function is used as constructor
<prototype>: function () // <prototype> represents who is parent of this object
Also consider this code example:
In this code we are assigning a function an method:
function sayHello() {
return "Hello World"
}
sayHello.sayBye = function() {
return "Bye Bye"
}
console.log(sayHello.sayBye()) // Bye Bye
// This sayHello details is only accessible in Firefox browser. For this write sayHello in console
function sayHello()
arguments: null
caller: null
length: 0
name: "sayHello"
prototype: Object { … } // .prototype property useful when function is used as constructor
sayBye: function sayBye() // Your define "sayBye" method.
<prototype>: function () // <prototype> represents who is parent of this object
As you can see that we can assign function an properties and methods just like an object. Also in JavaScript functions can do much more than this as they are First Class Functions
or First Class Objects
or First Class Citizens
this means that they can be:
Assign as a variable
Pass as an argument to another function
Can be return from the another function
Consider these code:
Function assign to an variable.
// Function stored in sayHello variable
const sayHello = function() {
return "Hello World"
}
// Function stored in an array
const array = ["Bye Bye", sayHello]
console.log(sayHello()) // Hello World
console.log(array[0]()) // Hello World
Function pass as an argument to another function.
// Declared "hello" function
function hello() {
return "Hello";
}
// Declared "say" function
function say(func) {
return func()
}
// Passing "hello" function as an argument to "say" function
console.log(say(hello)) // Hello
Functions return from another function.
// Declared "say" function
function say() {
const message = "Hello";
// Return another function from "say"
return function() {
return `I am saying ${message}`;
}
}
// Function that is returned from "say"
const functionReturned = say()
console.log(functionReturned()) // I am saying Hello
Why typeof Function
is ”function”
?
Functions are not just objects in JavaScript, they are special callable Object
, meaning that they have an internal [[call]]
method that typeof
operator will check before returning for type of objects
.
Here, you can see for the object, the typeof
operator check if there is [[call]]
method present in the object and if [[call]]
method is present in the object it returns function
and if not present than return object
.
Now, functions are special object as explained above have there own [[call]]
method so typeof
will return ”function”
for them and for array
as they don’t have there own [[call]]
method so they are not callable object so typeof
will return the ”object”
for them. This is also true for String
, Number
, Boolean
and any other wrapper object as they also don’t have [[call]]
method so typeof
will also return ”object”
for them.
const str = new String("Hello")
const num = new Number(33)
const bool = new Boolean(true)
function sayHello() {
return "Hello"
}
console.log(typeof str) // object
console.log(typeof num) // object
console.log(typeof bool) // object
console.log(typeof sayHello) // function
Here, [[call]]
simply means that we can call an object using the ()
parentheses.
Conclusion
In JavaScript, almost everything is an object, including arrays, functions, and even other built-in types and now you know the reason for this.
Subscribe to my newsletter
Read articles from Ridam Singhal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
