More Classes vs. More Functions: The Eternal Developer Dilemma
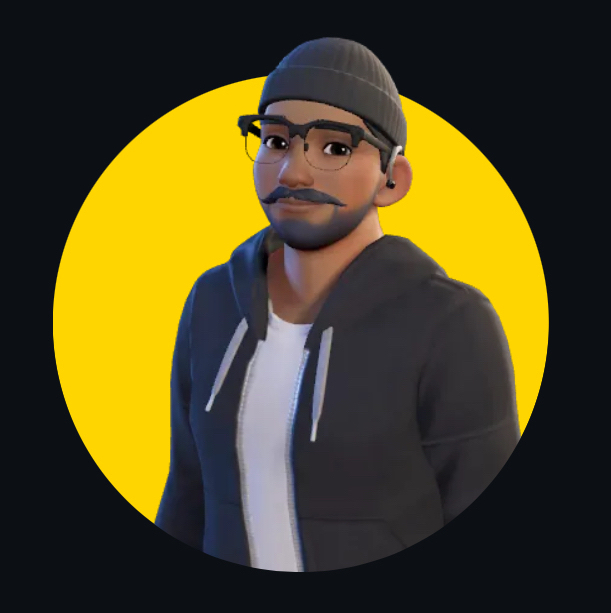
If you've been coding long enough, you've probably faced this classic dilemma: Should I create more classes or just add more functions in an existing class? Itโs like deciding between keeping everything in one big suitcase or neatly organizing stuff into multiple smaller bags.
Neither approach is inherently wrong, but choosing the right one can make your code scalable, maintainable, and readable. So, letโs break it down and understand when to go class-heavy and when to stick to functions.
๐ The Case for More Classes
When to Use More Classes?
When You Have Distinct Entities ๐๏ธ
If your logic can be broken into separate responsibilities, use different classes.
Example: A
UserService
class handling authentication should be separate from anOrderService
handling orders.
Reusability Across Multiple Modules ๐
If the logic needs to be reused across multiple flows, it makes sense to encapsulate it in a separate class.
Example: A
PaymentProcessor
class that multiple checkout systems use.
Encapsulation & Data Hiding ๐
More classes mean better separation of concerns.
Example: A
DatabaseConnector
should not expose direct SQL queries in an unrelatedOrderProcessing
class.
Scalability & Maintainability ๐ง
If functionality is expected to grow significantly, keeping it in separate classes prevents a massive God-class.
Example: A
NotificationService
class can later expand to support SMS, Email, and Push notifications without cluttering another class.
Example: More Classes Approach
public class InternalOrderService {
public Response placeOrderInternal(String productId, int quantity, String warehouseId, String authToken) {
return RestAssured.given()
.header("Authorization", "Bearer " + authToken)
.post("/internal/orders/place");
}
}
public class B2COrderService {
public Response placeOrderB2C(String productId, int quantity, String authToken) {
return RestAssured.given()
.header("Authorization", "Bearer " + authToken)
.post("/b2c/orders/place");
}
}
โ Pros:
Clear separation of internal and external logic.
Easier to debug and extend.
Avoids bloated classes.
โ Cons:
More files to manage.
Might feel over-engineered for small applications.
๐ค The Case for More Functions in Fewer Classes
When to Use More Functions?
When the Functionality is Closely Related ๐
If all functions logically belong together, putting them in the same class makes sense.
Example:
MathUtils
having functions for addition, subtraction, and multiplication.
If the Class Has a Single Responsibility ๐ฏ
If a class is designed to handle a specific task, it should contain all related methods.
Example:
FileHandler
class withreadFile()
,writeFile()
, anddeleteFile()
.
Avoiding Class Explosion ๐ฅ
Overuse of classes can lead to unnecessary complexity.
Example: Instead of separate
UserLogin
,UserLogout
, andUserSession
classes, a singleUserAuth
class may be more practical.
Example: More Functions in One Class Approach
public class OrderPlacementUtils {
public static Response placeOrderInternal(String productId, int quantity, String warehouseId, String authToken) {
return RestAssured.given()
.header("Authorization", "Bearer " + authToken)
.post("/internal/orders/place");
}
public static Response placeOrderB2C(String productId, int quantity, String authToken) {
return RestAssured.given()
.header("Authorization", "Bearer " + authToken)
.post("/b2c/orders/place");
}
}
โ Pros:
Easier to manage fewer files.
Quicker to implement simple functionalities.
Reduces over-engineering.
โ Cons:
Can become bloated over time.
Harder to refactor if more logic is added later.
โ๏ธ The Middle Ground: A Balanced Approach
A pragmatic approach is to start with functions inside a class and split into multiple classes only when necessary. Hereโs a good rule of thumb:
โ Use one class with functions if:
The logic is simple and closely related.
The number of functions is less than 5-6.
โ Create separate classes if:
The logic is becoming too large (> 300-400 lines of code).
Different entities are getting mixed.
๐ฏ The Final Verdict
Scenario | More Classes | More Functions in Fewer Classes |
Reusability | โ Better for reuse across multiple areas | โ If functions are closely related |
Complexity | โ Better for complex, growing applications | โ Better for simple implementations |
Maintainability | โ Easier to modify without breaking other code | โ Fewer files to manage |
Scalability | โ More scalable for expanding logic | โ Good for small to medium applications |
๐ Conclusion: Choose Wisely!
Ultimately, thereโs no universal right answer. The best approach depends on your projectโs needs:
Start with functions inside a class for simplicity.
Refactor into multiple classes as complexity increases.
Follow the Single Responsibility Principle (SRP) to decide when to split.
Every project is different, but with a little foresight, you can strike the perfect balance between maintainability and simplicity. ๐
๐ก What do you think? Do you prefer breaking things into multiple classes or keeping things together in one big class? Letโs discuss in the comments! ๐
Subscribe to my newsletter
Read articles from Himanshu Nikhare directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
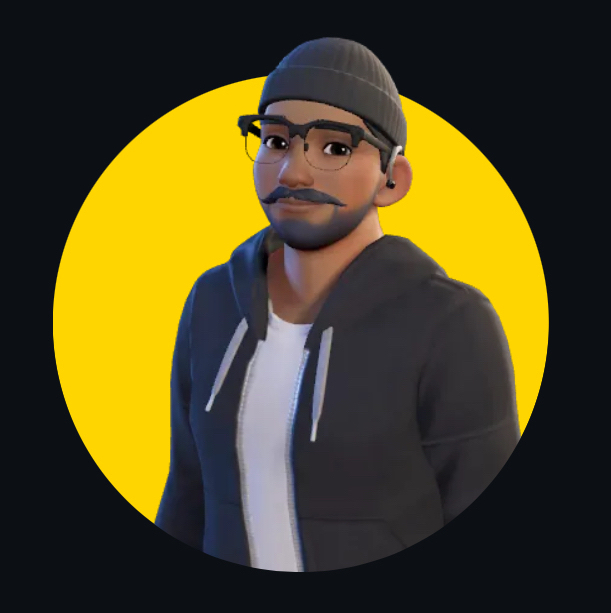
Himanshu Nikhare
Himanshu Nikhare
I am a Senior Software Engineer at BigBasket with over three years of experience in software development. Specializing in QA automation, CI/CD, and mobile automation, I am passionate about creating efficient workflows and sharing knowledge through blogs and articles. I actively contribute to the tech community through my work, open-source projects, and insightful content. Feel free to connect with me on GitHub or LinkedIn to explore my projects and contributions.