Understanding DOM: How JavaScript interacts with HTML

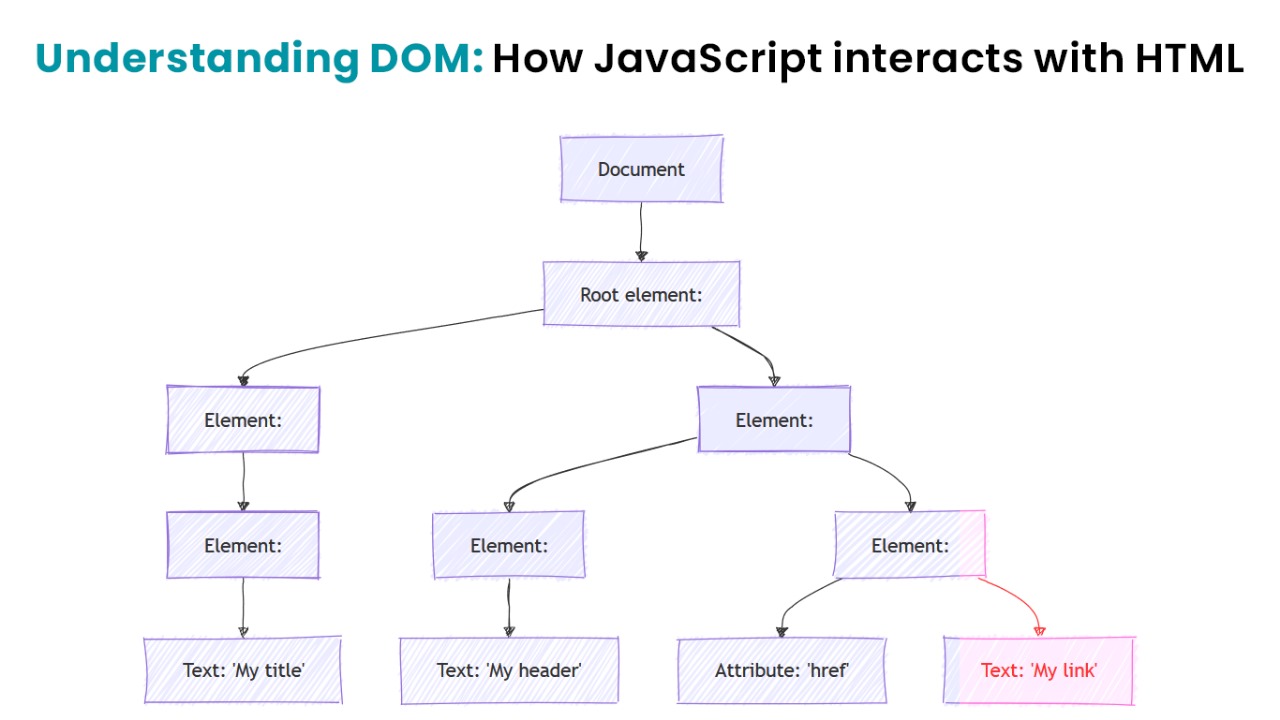
1. Introduction to the DOM
“Document Object Model” or simply DOM is an API that is cross-platform and language-independent that treats an HTML or XML document as a tree-like structure. It represents web pages as a hierarchy of nodes and objects, allowing dynamic access and manipulation of the document’s content, structure, and style
Each branch of the tree ends in a node, and each node contains objects. DOM methods allow programmatic access to the tree. With them, you can change the document's structure, style, or content. Nodes are actually HTML elements but the root node is the document itself. Each element node has a tag name and attributes, and can contain other element nodes or text nodes as children. For example, an HTML document with the following structure
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>DOM Tree Example</title>
<link rel="stylesheet" href="styles.css"> <!-- This represents the <link> (CSS) -->
</head>
<body>
<form>
<label for="name">Name:</label>
<input type="text" id="name" name="name">
<label for="email">Email:</label>
<input type="email" id="email" name="email">
<button type="submit">Submit</button>
</form>
<div>
<h2>Contact Us</h2>
<p>Email: example@example.com</p>
</div>
</body>
</html>
will be represented in DOM tree as:
Document
│
├── <html>
│ ├── <head>
│ │ ├── <title>
│ │ └── <link> (CSS)
│ ├── <body>
│ ├── <form>
│ │ ├── <label> Name:
│ │ ├── <input type="text">
│ │ ├── <label> Email:
│ │ ├── <input type="email">
│ │ ├── <button> Submit
│ ├── <div>
│ │ ├── <h2> Contact Us
│ │ ├── <p> Email: example@example.com
2. How JavaScript Accesses the DOM
The important thing to understand first is that the DOM is not part of the JavaScript language, but is instead a Web API used to build websites. JavaScript can also be used in other contexts. The DOM was designed to be independent of any particular programming language, making the structural representation of the document available from a single, consistent API. Even if most web developers will only use the DOM through JavaScript, implementations of the DOM can be built for any language.
You can start using the DOM right away without any special setup. Simply access the API directly in JavaScript within a script, which is a program executed by the browser. When you write a script, either inline within a <script>
element or as an external file included in a web page, you can instantly use the API for the document
or window
objects. This allows you to manipulate the document itself or any of its descendant elements within the web page.
For example, the following function creates a new h1
element, adds text to that element, and then adds it to the tree for the document:
<html lang="en">
<head>
<script>
// run this function when the document is loaded
window.onload = () => {
// create a <h1></h1> element in an otherwise empty HTML page
const heading = document.createElement("h1");
// create a text to add between tags
const headingText = document.createTextNode("Some Text.");
// append the text to <h1> element
heading.appendChild(headingText);
// append the <h1>Some Text.</h1> to the body of HTML
document.body.appendChild(heading);
};
</script>
</head>
<body></body>
</html>
3. Manipulating HTML elements with JavaScript
To manipulate HTML elements we first need their reference in JavaScript. Elements can be refereed and stored in variables in JavaScript via different ways. To get all the elements in the document with the class content
, we can create a variable and set it equal to document.querySelectorAll('.content')
. Similarly we can get element by their id by document.getElementById(‘<id of that element>‘)
.
let elements = document.querySelectorAll('.content');
let element = document.getElementById('<id of that element>');
Once we have a reference to an element, we can manipulate its content, attributes, and styles dynamically.
Modifying Text Content
We can change the text inside an element using the .textContent
or .innerHTML
properties:
element.textContent = "New text content";
element.innerHTML = "<strong>Bold text</strong>";
Changing Styles
To modify the CSS properties of an element using the .style
property:
element.style.color = "red";
element.style.fontSize = "20px";
Adding and Removing Classes
To dynamically add or remove CSS classes to modify an element's appearance:
element.classList.add("new-class");
element.classList.remove("old-class");
Creating and Appending Elements
We can also create new elements and insert them into the document:
let newElement = document.createElement("p");
newElement.textContent = "This is a new paragraph";
document.body.appendChild(newElement);
4. Traversing and Navigating DOM Tree
Traversing the DOM refers to moving between nodes to access, modify, or manipulate elements dynamically. Traversing the DOM efficiently is crucial for dynamic content manipulation, event handling, and modifying web page elements without unnecessary performance costs. The DOM tree consists of parent, child, and sibling relationships between elements:
Parent Node: The node that contains another node.
Child Nodes: The nodes contained within a parent node.
Sibling Nodes: Nodes that share the same parent.
For example, in an HTML list, <ul>
is the parent, <li>
elements are children, and each <li>
is a sibling of the others. To navigate the DOM following methods are used
Parent Element (moving up the DOM tree)
let parent = element.parentElement;
Child Elements (moving down the DOM tree)
let children = element.children; let firstChild = element.firstElementChild; let lastChild = element.lastElementChild;
Sibling Elements (moving between elements of the same parent)
let next = element.nextElementSibling; let previous = element.previousElementSibling;
5. Dynamically creating element with JavaScript and DOM
The Document Object Model (DOM) allows JavaScript to dynamically create and manipulate elements within an HTML document. This is an example demonstrating on how to generate an unordered list (<ul>
) dynamically when a button is clicked.
Creating an HTML List Dynamically
Example
In this example, we add a new list to the page when a button is clicked.
HTML
<input type="button" value="Generate a List" onclick="generateList()" />
JavaScript
function generateList() {
// Create a <ul> element
const list = document.createElement("ul");
// Creating list items dynamically
for (let i = 1; i <= 3; i++) {
// Create a <li> element and a text node
const listItem = document.createElement("li");
const listItemText = document.createTextNode(`Item ${i}`);
// Append the text node to the <li> element
listItem.appendChild(listItemText);
// Append the <li> element to the <ul>
list.appendChild(listItem);
}
// Append the <ul> element to the <body>
document.body.appendChild(list);
}
Result
After clicking the button, a dynamically generated list will appear on the page:
<ul>
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
</ul>
Explanation
The script follows a structured approach to create and append elements:
Creating the Parent Element
- We first create a
<ul>
element to serve as the container for our list items.
- We first create a
Creating Child Elements
We use a loop to create multiple
<li>
elements.For each
<li>
, a text node is created and added.
Appending Elements to Build the Structure
Each text node is appended to its respective
<li>
element.Each
<li>
is then added to the<ul>
.Finally, the
<ul>
is appended to the<body>
.
Understanding the DOM Structure
The generated DOM structure looks like this:
<ul>
├── <li> Item 1 </li>
├── <li> Item 2 </li>
├── <li> Item 3 </li>
</ul>
This example demonstrates how JavaScript can dynamically generate elements and attach them in a hierarchical manner, following the principles of the DOM tree model.
Conclusion: The Power of JavaScript and the DOM
The Document Object Model (DOM) acts as a connection between JavaScript and HTML, allowing for dynamic changes and real-time updates to web pages. By using the DOM API, developers can easily create, change, and navigate elements, resulting in interactive and responsive web applications.
Understanding how to manipulate the DOM is crucial for front-end development. It enables smooth user interactions, dynamic content changes, and event-driven programming. By following best practices—like reducing unnecessary DOM changes and improving performance—JavaScript developers can create efficient, scalable, and engaging web experiences.
Subscribe to my newsletter
Read articles from Saad Ur Rehman directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
