🚀 Day 8: JavaScript Prototypes - Unlocking the Power of Object Inheritance 🔥

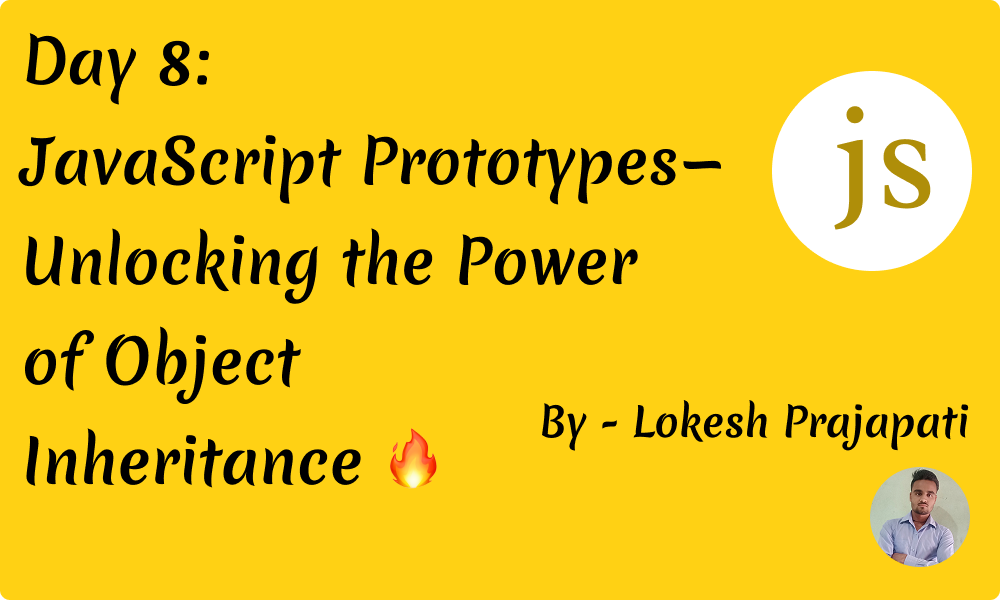
Welcome to Day 8 of our JavaScript Basics to Advanced series! Today, we’re diving deep into one of JavaScript’s core concepts — Prototypes. Understanding prototypes is essential for mastering JavaScript, as they form the foundation of how objects inherit properties and methods.
By the end of this post, you’ll have a solid grasp of prototypes, prototype chains, and how JavaScript’s inheritance model works with real-world examples.
1️⃣ What Are Prototypes in JavaScript?
In JavaScript, objects do not inherit from classes (as in other languages like Java or Python). Instead, they inherit from other objects via something called a prototype.
👉 Every JavaScript object has an internal property called [[Prototype]]
, which points to another object from which it can inherit properties and methods.
Think of it as a blueprint that objects can refer to when they don’t have a property or method of their own.
Example: Prototypes in Action
const person = {
greet: function() {
return "Hello!";
}
};
const user = Object.create(person); // user inherits from person
console.log(user.greet()); // Output: "Hello!"
console.log(user.hasOwnProperty("greet")); // Output: false
🚀 Since user
doesn’t have the greet
method, it looks up its prototype (person) to find it.
2️⃣ The Prototype Chain: How It Works
A prototype chain is the process JavaScript follows to find a property or method on an object. If it doesn’t find the property in the object itself, it moves up the prototype chain until it either finds it or reaches null
(the top of the chain).
Example: Understanding the Prototype Chain
const vehicle = {
wheels: 4,
start: function() {
return "Starting vehicle...";
}
};
const car = Object.create(vehicle);
car.color = "red";
console.log(car.color); // "red" (found on car itself)
console.log(car.wheels); // 4 (inherited from vehicle)
console.log(car.start()); // "Starting vehicle..." (inherited)
console.log(car.hasOwnProperty("wheels")); // false (belongs to prototype)
**Prototype Lookup Process
**1️⃣ JavaScript first checks car
for the property/method.
2️⃣ If it’s not found, it checks car
's prototype (vehicle
).
3️⃣ If still not found, it moves up the chain until null
is reached.
This process is automatic and happens behind the scenes!
3️⃣ Constructor Functions & Prototypes
In JavaScript, we can create multiple objects using constructor functions, and all of them can share the same methods using prototypes, saving memory.
Example: Using Prototypes in a Constructor Function
function Person(name) {
this.name = name;
}
// Adding method to prototype
Person.prototype.sayHello = function() {
return `Hello, my name is ${this.name}`;
};
const user1 = new Person("Alice");
const user2 = new Person("Bob");
console.log(user1.sayHello()); // "Hello, my name is Alice"
console.log(user2.sayHello()); // "Hello, my name is Bob"
💡 Instead of storing sayHello
in every object, we attach it to Person.prototype, so all instances share the same method!
4️⃣ Changing & Overriding Prototypes
You can override prototype methods if you want custom behavior for a particular object.
Example: Overriding a Prototype Method
function Animal(name) {
this.name = name;
}
Animal.prototype.makeSound = function() {
return "Some generic sound";
};
const dog = new Animal("Buddy");
console.log(dog.makeSound()); // "Some generic sound"
// Overriding the prototype method
dog.makeSound = function() {
return "Woof! Woof!";
};
console.log(dog.makeSound()); // "Woof! Woof!"
Here, dog
has its own makeSound
method, so JavaScript doesn’t look up the prototype chain.
5️⃣ The __proto__
vs. prototype
Confusion
JavaScript has two different terms related to prototypes, which can be confusing:
🔹 prototype
Exists only on constructor functions (like
Person.prototype
).Used to define methods that should be shared across all instances.
🔹 __proto__
Exists on all objects and points to their prototype.
It’s used to access the object’s prototype manually but should not be modified directly.
Example: The Difference Between prototype
& __proto__
function Cat(name) {
this.name = name;
}
// Adding a method to the prototype
Cat.prototype.speak = function() {
return "Meow!";
};
const kitty = new Cat("Whiskers");
console.log(kitty.__proto__ === Cat.prototype); // true
console.log(Cat.prototype.speak()); // Error! Cat.prototype has no 'name'
🚨 Rule: Always use Class.prototype.methodName = function() {}
instead of modifying __proto__
directly!
6️⃣ Real-World Use Case of Prototypes
Imagine you’re creating an online store where every product has a name
and price
. You don’t want each product object to store the same methods individually (it wastes memory), so you use prototypes.
Example: Prototypes in an E-commerce App
function Product(name, price) {
this.name = name;
this.price = price;
}
// Adding a method to the prototype
Product.prototype.getDetails = function() {
return `Product: ${this.name}, Price: $${this.price}`;
};
const item1 = new Product("Laptop", 1000);
const item2 = new Product("Phone", 500);
console.log(item1.getDetails()); // "Product: Laptop, Price: $1000"
console.log(item2.getDetails()); // "Product: Phone, Price: $500"
Here, both products share the same getDetails
method instead of creating a separate copy in each instance.
7️⃣ Summary & Key Takeaways
✅ Prototypes allow objects to inherit properties & methods from other objects.
✅ Prototype Chain is the process of looking up properties/methods through the chain.
✅ Constructor Functions use prototypes to share methods efficiently.
✅ prototype
is for defining shared methods, __proto__
is for lookup.
✅ Overriding prototypes can customize object behavior.
✅ Real-world use cases include object sharing in web apps like e-commerce stores.
Understanding prototypes is crucial for mastering JavaScript, especially if you plan to work with Object-Oriented Programming (OOP), React, or Node.js. 🚀
💬 Do you use prototypes in your projects? Have any doubts? Drop your questions in the comments! ⬇️
Subscribe to my newsletter
Read articles from Lokesh Prajapati directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Lokesh Prajapati
Lokesh Prajapati
🚀 JavaScript | React | Shopify Developer | Tech Blogger Hi, I’m Lokesh Prajapati, a passionate web developer and content creator. I love simplifying JavaScript, React, and Shopify development through easy-to-understand tutorials and real-world examples. I’m currently running a JavaScript Basics to Advanced series on Medium & Hashnode, helping developers of all levels enhance their coding skills. My goal is to make programming more accessible and practical for everyone. Follow me for daily coding tips, tricks, and insights! Let’s learn and grow together. 💡🚀 #JavaScript #React #Shopify #WebDevelopment #Coding #TechBlogger #LearnToCode