How to Build Interactive UI with Next.js and Tailwind CSS in 2025
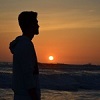
Building interactive and modern UIs has become increasingly easier with tools like Next.js and Tailwind CSS. These technologies have gained widespread popularity among developers due to their simplicity, flexibility, and performance. In this guide, we'll walk through how to combine Next.js (a popular React framework) and Tailwind CSS (a utility-first CSS framework) to create a modern, interactive user interface in 2025.
Why Use Next.js and Tailwind CSS?
Before diving into the actual process, let's briefly explore why Next.js and Tailwind CSS are an excellent combination for building interactive UIs:
Next.js: It's a powerful React framework that offers server-side rendering (SSR), static site generation (SSG), API routes, and built-in optimizations for performance. It simplifies complex tasks like routing, data fetching, and page rendering, making it ideal for building dynamic and high-performing web applications.
Tailwind CSS: A utility-first CSS framework that lets you rapidly build custom designs without writing custom CSS. It comes with a set of low-level utility classes that you can combine to style components, making it highly customizable and efficient.
Setting Up Next.js and Tailwind CSS in 2025
Step 1: Create a New Next.js Project
Start by setting up a new Next.js project. Open your terminal and run the following commands:
bash
# Install the Next.js app
npx create-next-app@latest my-next-tailwind-project
# Change to the project directory
cd my-next-tailwind-project
This will create a new Next.js app with the latest setup.
Step 2: Install Tailwind CSS
Next, install Tailwind CSS in your project. Run the following commands in your terminal:
bash
# Install Tailwind and its dependencies
npm install -D tailwindcss postcss autoprefixer
# Generate the Tailwind config file
npx tailwindcss init -p
This generates both a tailwind.config.js file and a postcss.config.js file.
Step 3: Configure Tailwind in the Project
Now, configure Tailwind CSS. Open the tailwind.config.js file and add the following content to specify where Tailwind should look for classes:
js
module.exports = {
content: [
'./pages/**/*.{js,ts,jsx,tsx}',
'./components/**/*.{js,ts,jsx,tsx}',
],
theme: {
extend: {},
},
plugins: [],
}
Building Interactive UI Components
Step 4: Create a Responsive Navigation Bar
Let's begin by building a simple, responsive navigation bar. This will demonstrate how to use Tailwind CSS classes for layout and style, while also showcasing responsiveness.
In the components folder, create a new file called Navbar.js:
jsx
import React, { useState } from 'react';
const Navbar = () => {
const [isOpen, setIsOpen] = useState(false);
return (
<nav className="bg-blue-500 p-4">
<div className="container mx-auto flex justify-between items-center">
<div className="text-white text-2xl">MyApp</div>
<div className="space-x-4 hidden md:flex">
<a href="#" className="text-white hover:text-blue-200">Home</a>
<a href="#" className="text-white hover:text-blue-200">About</a>
<a href="#" className="text-white hover:text-blue-200">Services</a>
<a href="#" className="text-white hover:text-blue-200">Contact</a>
</div>
<button
className="md:hidden text-white"
onClick={() => setIsOpen(!isOpen)}
\>
{isOpen ? 'Close' : 'Menu'}
</button>
</div>
{isOpen && (
<div className="md:hidden">
<a href="#" className="block text-white py-2 px-4">Home</a>
<a href="#" className="block text-white py-2 px-4">About</a>
<a href="#" className="block text-white py-2 px-4">Services</a>
<a href="#" className="block text-white py-2 px-4">Contact</a>
</div>
)}
</nav>
);
};
export default Navbar;
In this code, we use the hidden md:flex utility class to hide the navigation links on small screens and display them only on medium and larger screens. On mobile, the menu button toggles the visibility of the links.
Step 5: Add Dynamic Content Using Next.js
To make the UI more interactive, let's fetch data from an API and display it. We’ll use Next.js's getServerSideProps to fetch the data on the server and send it to the client.
In your pages/index.js file:
jsx
Copy
import { useEffect, useState } from 'react';
import Navbar from '../components/Navbar';
const Home = ({ data }) => {
const [items, setItems] = useState(data);
return (
<div>
<Navbar />
<main className="p-6">
<h1 className="text-3xl mb-4">Latest Items</h1>
<div className="grid grid-cols-1 sm:grid-cols-2 lg:grid-cols-3 gap-6">
{items.map((item) => (
<div key={item.id} className="bg-white p-4 rounded shadow-md">
<h2 className="text-xl font-bold">{item.name}</h2>
<p>{item.description}</p>
</div>
))}
</div>
</main>
</div>
);
};
export async function getServerSideProps() {
// Simulate fetching data from an API
const data = [
{ id: 1, name: 'Item 1', description: 'Description of Item 1' },
{ id: 2, name: 'Item 2', description: 'Description of Item 2' },
{ id: 3, name: 'Item 3', description: 'Description of Item 3' },
];
return {
props: {
data,
},
};
}
export default Home;
In this example, getServerSideProps fetches the data (simulated here) and passes it as a prop to the Home component. We dynamically display the items using Tailwind CSS for layout and styling.
Conclusion
By combining Next.js with Tailwind CSS, you can quickly build modern, responsive, and interactive user interfaces. Next.js manages the logic and rendering, while Tailwind CSS simplifies styling with its utility-first approach. This results in a streamlined workflow that lets you focus on building engaging web applications with minimal effort. This powerful toolset is widely used in full-stack development course in Delhi, Noida, Mumbai, and other Indian cities, where students learn how to create exceptional user experiences with cutting-edge technologies. Whether you’re building a simple landing page or a complex web app, this combination offers the flexibility and performance required to deliver a top-tier user experience in 2025.
Subscribe to my newsletter
Read articles from Sanjeet Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
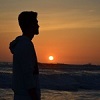
Sanjeet Singh
Sanjeet Singh
I am Sanjeet Singh, an IT professional with experience in the IT sector. I have a broad understanding of Data Analytics and proficiency across multiple layers of software development and testing, from the front end to the back end.