Introduction to PLINQ in C# .NET: Parallelising Queries for Performance Gains

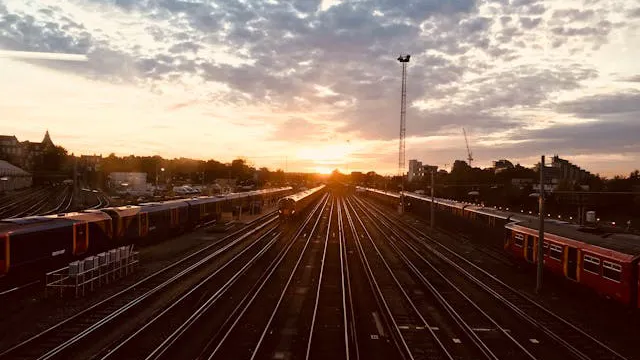
Parallel LINQ (PLINQ) is a powerful feature in C# that enables parallel execution of LINQ queries to leverage multi core processors for improved performance. While PLINQ can significantly speed up data processing in many scenarios, it is not always the best choice. If used incorrectly, it can lead to degraded performance and increased complexity.
In this article, we'll explore what PLINQ is, how it works, when to use it, when to avoid it, and common pitfalls to watch out for.
What is PLINQ?
PLINQ (Parallel LINQ) is a parallelised implementation of LINQ (Language Integrated Query) in C#. It is part of the System.Linq
namespace and allows developers to process collections in parallel by utilising multiple threads.
PLINQ builds on the Task Parallel Library (TPL) and is designed to provide automatic parallelisation while maintaining the declarative syntax of LINQ. The goal of PLINQ is to improve performance by distributing work across multiple CPU cores.
PLINQ automatically partitions the data and processes it using multiple threads. This is done by calling the .AsParallel()
method on an IEnumerable<T>
collection. By default, PLINQ tries to determine whether parallelisation will be beneficial, but you can also force parallel execution.
Basic Example of PLINQ
using System;
using System.Linq;
class Program
{
static void Main()
{
int[] numbers = Enumerable.Range(1, 10).ToArray();
var squaredNumbers = numbers.AsParallel()
.Select(n => n * n)
.ToArray();
Console.WriteLine(string.Join(", ", squaredNumbers));
}
}
In this example, .AsParallel()
enables parallel execution of the Select
operation, distributing the workload across multiple CPU cores.
Forcing Parallel Execution
By default, PLINQ may fall back to sequential execution if it determines that parallelization won't improve performance. You can force parallel execution using the .WithExecutionMode(ParallelExecutionMode.ForceParallelism)
method:
var results = numbers.AsParallel()
.WithExecutionMode(ParallelExecutionMode.ForceParallelism)
.Select(n => ExpensiveComputation(n))
.ToArray();
When to Use PLINQ
PLINQ is useful when:
Processing Large Data Sets: When working with large collections, parallel execution can significantly reduce processing time.
CPU-Bound Operations: Tasks that involve heavy computation (e.g., mathematical calculations, image processing) benefit from PLINQ.
Independent Operations: If each item in the collection can be processed independently, parallel execution can be effective.
Multi Core Environments: Modern CPUs have multiple cores, and PLINQ can help utilise them efficiently.
Example: Processing a Large Data Set in Parallel
var largeNumbers = Enumerable.Range(1, 1_000_000);
var primes = largeNumbers.AsParallel()
.Where(IsPrime)
.ToArray();
static bool IsPrime(int number)
{
if (number < 2) return false;
for (int i = 2; i <= Math.Sqrt(number); i++)
{
if (number % i == 0) return false;
}
return true;
}
This example finds prime numbers in a large dataset using PLINQ, significantly improving performance compared to a sequential LINQ query.
When NOT to Use PLINQ
PLINQ is not always beneficial. Here are cases where you should avoid it:
Small Data Sets: The overhead of parallel execution may outweigh the benefits for small collections.
I/O-Bound Operations: PLINQ is optimised for CPU-bound tasks. If your operation involves database queries, file I/O, or network requests, using PLINQ can degrade performance.
Order Dependent Queries: Parallel execution does not guarantee order unless explicitly specified using
.AsOrdered()
, which can negate performance gains.Stateful or Shared Resources: If your operations modify shared state (e.g., writing to a file, updating a shared variable), race conditions can occur, leading to unpredictable behaviour.
Example: PLINQ Performing Worse Than Sequential LINQ
var names = new List<string> { "Alice", "Bob", "Charlie", "David" };
var greetings = names.AsParallel()
.Select(name => $"Hello {name}")
.ToArray();
For a small list, the overhead of managing parallel threads may result in worse performance than simply using LINQ.
PLINQ Performance Pitfalls & Warnings
While PLINQ can improve performance, improper use can lead to performance degradation and unexpected behaviour:
Threading Overhead: Creating and managing threads incurs an overhead, which can make PLINQ slower than sequential LINQ for small data sets.
Unpredictable Execution Order: PLINQ does not preserve order by default. If order matters, use
.AsOrdered()
, but this may reduce performance.Deadlocks & Race Conditions: If queries involve shared state, unexpected concurrency issues may arise.
Blocking Calls in Parallel Queries: Performing
Task.Delay()
,Thread.Sleep()
, or other blocking calls within a PLINQ query can cause inefficient thread usage.
Example: Handling Order in PLINQ
var orderedResults = numbers.AsParallel()
.AsOrdered()
.Select(n => n * n)
.ToArray();
Using .AsOrdered()
ensures that results appear in the same order as the original sequence, but at the cost of some parallel performance gains.
PLINQ is a valuable tool in .NET for improving performance by parallelising LINQ queries. However, it should be used carefully. Always measure performance before and after implementing PLINQ to ensure that it provides actual benefits.
Things to remember…….
Use PLINQ for CPU-intensive, independent tasks on large datasets.
Avoid PLINQ for small datasets, I/O bound operations, and shared state modifications.
Be mindful of potential race conditions, ordering issues, and performance overhead.
By following these guidelines, you can effectively harness the power of PLINQ to enhance application performance in .NET!
Subscribe to my newsletter
Read articles from Patrick Kearns directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
