How to Build a Referral Program with Node.js
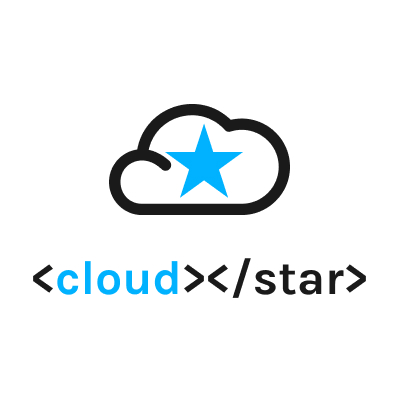
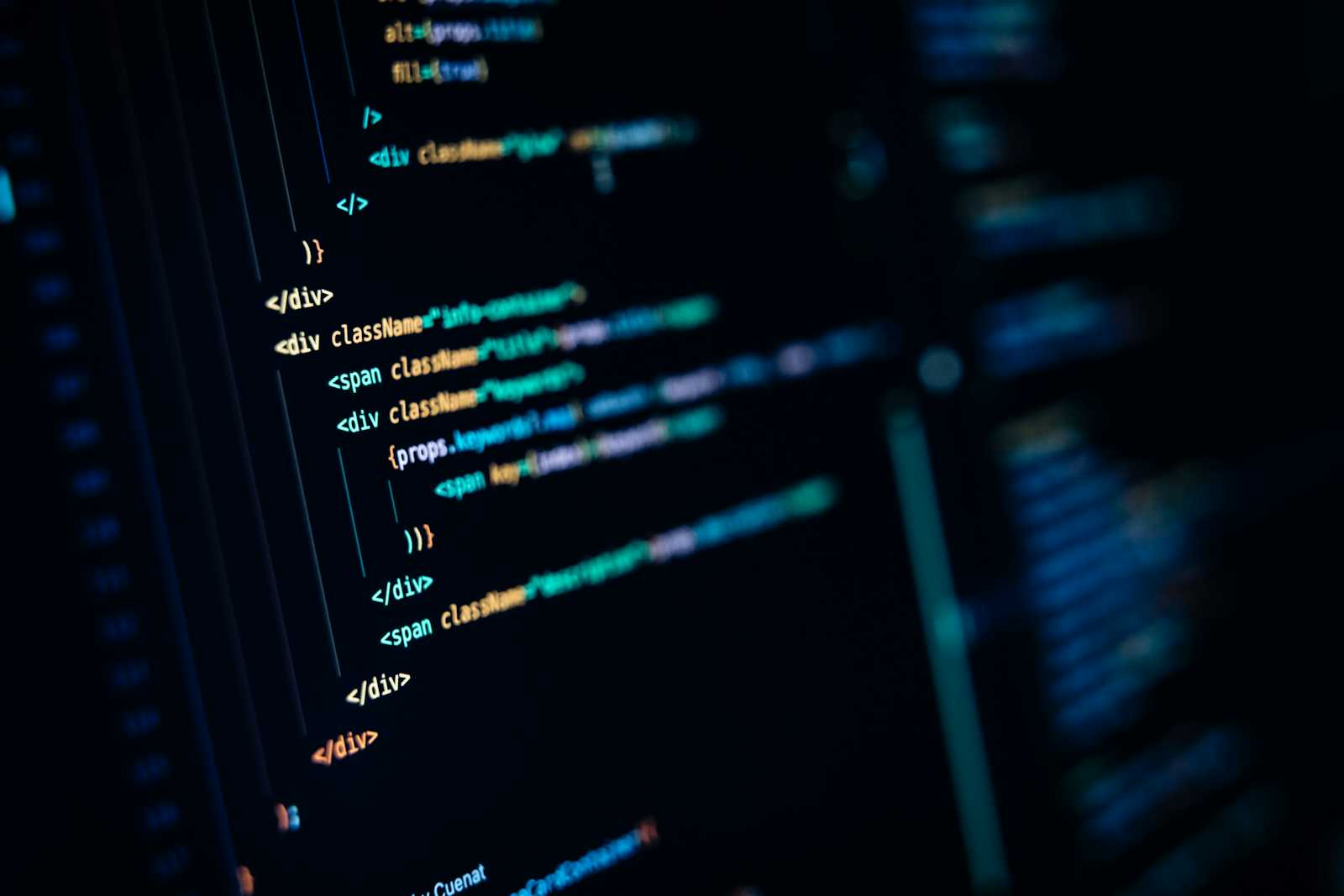
A referral program is a great way to acquire new users and retain existing ones by encouraging them to share your service. In this article, we will walk through building a simple referral system using Node.js, Express, and MongoDB.
Prerequisites
Before we begin, ensure you have the following installed:
Project Setup
First, create a new Node.js project and install the necessary dependencies:
mkdir referral-program
cd referral-program
npm init -y
npm install express mongoose uuid jsonwebtoken dotenv
express
: Web framework for Node.js.mongoose
: MongoDB object modeling tool.uuid
: Generates unique referral codes.jsonwebtoken
: Handles authentication.dotenv
: Loads environment variables.
Create a .env
file for storing environment variables:
PORT=5000
MONGO_URI=your_mongodb_connection_string
JWT_SECRET=your_jwt_secret
Setting Up the Server
Create an index.js
file and set up an Express server:
require('dotenv').config();
const express = require('express');
const mongoose = require('mongoose');
const app = express();
app.use(express.json());
mongoose.connect(process.env.MONGO_URI, {
useNewUrlParser: true,
useUnifiedTopology: true,
}).then(() => console.log('MongoDB Connected'))
.catch(err => console.log(err));
app.listen(process.env.PORT, () => {
console.log(`Server running on port ${process.env.PORT}`);
});
Creating the Referral Model
Create a models/User.js
file to define the user schema:
const mongoose = require('mongoose');
const { v4: uuidv4 } = require('uuid');
const UserSchema = new mongoose.Schema({
name: String,
email: { type: String, unique: true },
referralCode: { type: String, default: uuidv4 },
referredBy: { type: String, default: null },
rewards: { type: Number, default: 0 },
});
module.exports = mongoose.model('User', UserSchema);
Creating Routes
Register a User with a Referral Code
Create a routes/user.js
file:
const express = require('express');
const User = require('../models/User');
const router = express.Router();
router.post('/register', async (req, res) => {
const { name, email, referralCode } = req.body;
try {
const referredBy = referralCode ? await User.findOne({ referralCode }) : null;
const newUser = new User({
name,
email,
referredBy: referredBy ? referredBy.referralCode : null
});
await newUser.save();
if (referredBy) {
referredBy.rewards += 10; // Reward the referrer
await referredBy.save();
}
res.status(201).json({ message: 'User registered successfully', user: newUser });
} catch (err) {
res.status(500).json({ error: err.message });
}
});
module.exports = router;
Integrating the Routes
Update index.js
to use the user routes:
const userRoutes = require('./routes/user');
app.use('/api/users', userRoutes);
Testing the API
Use Postman or cURL to test the API:
Register a user without a referral code:
POST http://localhost:5000/api/users/register { "name": "John Doe", "email": "john@example.com" }
Register a user with a referral code:
POST http://localhost:5000/api/users/register { "name": "Jane Doe", "email": "jane@example.com", "referralCode": "referral_code_of_existing_user" }
Conclusion
Congratulations! You have successfully built a simple referral program with Node.js and MongoDB. This can be expanded by integrating authentication, better reward logic, and frontend integration.
Subscribe to my newsletter
Read articles from Alex Cloudstar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
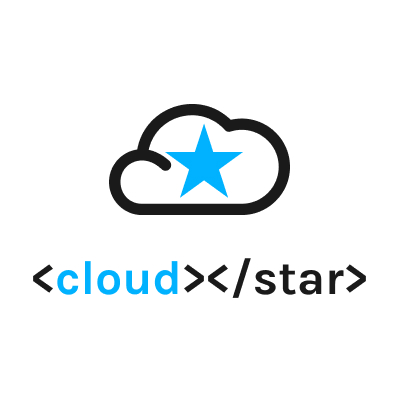
Alex Cloudstar
Alex Cloudstar
Hi there! ๐๐ป I'm a Senior Full-Stack Developer oriented on Javascript. Along my career so far, I worked with different technologies, a few of them are: React JS, React Native, Typescript, Styled Components, Redux, Webpack, Node JS, Express, NestJS, Prisma, AWS. My biggest ability is that I'm a self-learner. This is how I move forward and develop into this incredible career. I give my best on every project I'm working at. Always happy to get a fresh task! If you're interested to know more about me and my career, drop a message and let's talk! Cheers!